前言
初识pygame:pie游戏
pygame游戏库使得如下功能成为可能:绘制图形、获取用户输入、执行动画
以及使用定时器让游戏按照稳定的帧速率运行。
使用pygame库;
以一定字体打印文本;
使用循环来重复动作;
绘制圆、矩形、线条和户型;
创建pie游戏;
【----帮助Python学习,以下所有学习资料文末免费领!----】
从哪里获得pygame库:http://www.pygame.org/download.shtml
我现在使用的Python2.7和pygame1.9
书中使用的环境是Python3.2和pygame1.9
现在不在Python3的环境下安装上pip工具导致环境无法一致
pygame库的初始化工作:
import pygame
from pygame.locals import *
pygame.init()
创建一个(600,500)大小的屏幕
screen=pygame.display.set_mode((600,500))
screen同时被赋值为<Surface(600x500x32 SW)>
这是一个有用的值,所以用screen变量存储。
打印文本
1、创建字体对象
myfont=pygame.font.Font(None,60)
None:使用默认字体
60:字体大小
2、创建一个可以使用screen.blit()绘制的平面
textimage=myfont.render(“Hello Python”,True,(255,255,255))
render需要三个参数,需要被显示的字符串、是否抗锯齿True/False、颜色
3、将textimage交给screen.blit()进行绘制
screen.blit(textimage,(100,100))
screen.blit()需要两个参数,绘制的对象及其(左上角顶点)坐标
背景填充
screen.fill((0,0,0))
screen.fill()需要给出背景颜色
刷新显示
screen.display.update()
一般配合while循环使用
while循环
通过while循环可以进行事件处理和持续的屏幕刷新
while True:
for event in pygame.event.get():
if event.type in (QUIT,KEYDOWN):
sys.exit()
screen.display.update()
绘制圆形
pygame.draw.circle(screen,color,position,radius,width)
color (0,0,0)给定颜色
radius圆半径
position (0,0)给定圆心坐标
width线条宽度
绘制矩形
pygame.draw.rect(screen,color,position,width)
position (pos_x,pos_y,100,100)给定左上角顶点的坐标、长和宽
绘制线条
pygame.draw.line(screen,color,(0,0),(100,100),width)
(0,0)(100,100)负责给定线段的两个端点
绘制弧形
start_angle=math.radians(0)
end_angle=math.radians(90)
position=x-radius,y-radius,radius*2,radius*2
#x,y表示弧形所在的圆的圆心坐标,radius表示半径
pygame.draw.arc(screen,color,position,start_angle,end_angle,width)
start_angle起始角度 指向正右侧的半径开始逆时针旋转就是0到360
end_angle结束角度
两段值得学习的示例
1、绘制移动矩形
#!/usr/bin/python
import sys
import random
from random import randint
import pygame
from pygame import *
pygame.init()
screen=pygame.display.set_mode((600,500))
pygame.display.set_caption(“Drawing Rectangles”)
pos_x=300
pos_y=250
vel_x=2
vel_y=1
color=100,100,100
while True:
for event in pygame.event.get():
if event.type in (QUIT,KEYDOWN):
sys.exit()
screen.fill((0,0,200))
pos\_x +=vel\_x
pos\_y +=vel\_y
if pos\_x>500 or pos\_x<0:
vel\_x=-vel\_x
rand1=randint(0,255)
rand2=randint(0,255)
rand3=randint(0,255)
color=rand1,rand2,rand3
if pos\_y>400 or pos\_y<0:
vel\_y=-vel\_y
rand1=randint(0,255)
rand2=randint(0,255)
rand3=randint(0,255)
color=rand1,rand2,rand3
width=0
pos=pos\_x,pos\_y,100,100
pygame.draw.rect(screen,color,pos,width)
pygame.display.update()
2、pie游戏
#!/usr/bin/python
#init
import sys
import math
import pygame
from pygame.locals import *
pygame.init()
screen=pygame.display.set_mode((600,500))
pygame.display.set_caption(“The Pie Game-Press 1,2,3,4”)
myfont=pygame.font.Font(None,60)
color=200,80,60
width=4
x=300
y=250
radius=200
position=x-radius,y-radius,radius*2,radius*2
piece1=False
piece2=False
piece3=False
piece4=False
while True:
for event in pygame.event.get():
if event.typeQUIT:
sys.exit()
elif event.typeKEYUP:
if event.keypygame.K_ESCAPE:
sys.exit()
elif event.keypygame.K_1:
piece1=True
elif event.keypygame.K_2:
piece2=True
elif event.keypygame.K_3:
piece3=True
elif event.key==pygame.K_4:
piece4=True
screen.fill((0,0,200))
#draw the four numbers
textimage1=myfont.render("1",True,color)
screen.blit(textimage1,(x+radius/2-20,y-radius/2))
textimage2=myfont.render("2",True,color)
screen.blit(textimage2,(x-radius/2,y-radius/2))
textimage3=myfont.render("3",True,color)
screen.blit(textimage3,(x-radius/2,y+radius/2-20))
textimage4=myfont.render("4",True,color)
screen.blit(textimage4,(x+radius/2-20,y+radius/2-20))
#draw arc,line
if piece1:
start\_angle=math.radians(0)
end\_angle=math.radians(90)
pygame.draw.arc(screen,color,position,start\_angle,end\_angle,width)
pygame.draw.line(screen,color,(x,y),(x+radius,y),width)
pygame.draw.line(screen,color,(x,y),(x,y-radius),width)
if piece2:
start\_angle=math.radians(90)
end\_angle=math.radians(180)
pygame.draw.arc(screen,color,position,start\_angle,end\_angle,width)
pygame.draw.line(screen,color,(x,y),(x,y-radius),width)
pygame.draw.line(screen,color,(x,y),(x-radius,y),width)
if piece3:
start\_angle=math.radians(180)
end\_angle=math.radians(270)
pygame.draw.arc(screen,color,position,start\_angle,end\_angle,width)
pygame.draw.line(screen,color,(x,y),(x-radius,y),width)
pygame.draw.line(screen,color,(x,y),(x,y+radius),width)
if piece4:
start\_angle=math.radians(270)
end\_angle=math.radians(360)
pygame.draw.arc(screen,color,position,start\_angle,end\_angle,width)
pygame.draw.line(screen,color,(x,y),(x,y+radius),width)
pygame.draw.line(screen,color,(x,y),(x+radius,y),width)
#if success,display green
if piece1 and piece2 and piece3 and piece4:
color=0,255,0
pygame.display.update()
挑战
1、绘制椭圆
#!/usr/bin/python
import sys
import pygame
from pygame.locals import *
pygame.init()
screen=pygame.display.set_mode((600,500))
pygame.display.set_caption(“Drawing Ellipse”)
while True:
for event in pygame.event.get():
if event.type in (QUIT,KEYDOWN):
sys.exit()
screen.fill((0,255,0))
color=255,0,255
position=100,100,400,300
width=8
pygame.draw.ellipse(screen,color,position,width)
pygame.display.update()
这个题目就是让你认识一下pygame.draw.ellipse()函数的相关使用。
该函数和pygame.draw.rect()函数使用方式十分相似。
2、随机的绘制1000个线条
#!/usr/bin/python
import random
from random import randint
import sys
import pygame
from pygame import *
pygame.init()
screen=pygame.display.set_mode((800,600))
pygame.display.set_caption(“Drawing Line”)
screen.fill((0,80,0))
color=100,255,200
width=2
for i in range(1,1001):
pygame.draw.line(screen,color,(randint(0,800),randint(0,600)),(randint(0,800),randint(0,600)),width)
while True:
for event in pygame.event.get():
if event.type in (QUIT,KEYDOWN):
sys.exit()
pygame.display.update()
通过这个题目理解了如果绘制图形和刷新显示都在循环中时,while True循环每次都会绘
制图形并刷新显示。
调用pygame模块中的randint()函数。
而在while True循环外绘制图形,则图形绘制完成之后保持不变。刷新显示的是一个已经绘制好
的图形。如果大家对Python感兴趣的话,可以加一下我们的学习交流抠抠群哦:649,825,285,免费领取一套学习资料和视频课程哟~
3、修改矩形程序,使矩形碰到屏幕边界是,矩形会改变颜色
#!/usr/bin/python
import sys
import random
from random import randint
import pygame
from pygame import *
pygame.init()
screen=pygame.display.set_mode((600,500))
pygame.display.set_caption(“Drawing Rectangles”)
pos_x=300
pos_y=250
vel_x=2
vel_y=1
rand1=randint(0,255)
rand2=randint(0,255)
rand3=randint(0,255)
color=rand1,rand2,rand3
while True:
for event in pygame.event.get():
if event.type in (QUIT,KEYDOWN):
sys.exit()
screen.fill((0,0,200))
pos\_x +=vel\_x
pos\_y +=vel\_y
if pos\_x>500 or pos\_x<0:
vel\_x=-vel\_x
rand1=randint(0,255)
rand2=randint(0,255)
rand3=randint(0,255)
color=rand1,rand2,rand3
if pos\_y>400 or pos\_y<0:
vel\_y=-vel\_y
rand1=randint(0,255)
rand2=randint(0,255)
rand3=randint(0,255)
color=rand1,rand2,rand3
width=0
pos=pos\_x,pos\_y,100,100
pygame.draw.rect(screen,color,pos,width)
pygame.display.update()
这里需要用到random模块。在每次碰到屏幕边界时,不仅改变矩形的运动方向,而且使用随机数改变矩形的颜色。
也可以先将color设置为定值,可以少写三行代码。
最后给大家介绍一个完整的python学习路线,内容是从入门到进阶,既有思维导图,也有经典书籍,还有配套视频,给那些想学习python以及数据分析的小伙伴们一点帮助!
一、Python入门
下面这些内容是Python各个应用方向都必备的基础知识,想做爬虫、数据分析或者人工智能,都得先学会他们。任何高大上的东西,都是建立在原始的基础之上。打好基础,未来的路会走得更稳重。
包含:
计算机基础
python基础
Python入门视频600集:
观看零基础学习视频,看视频学习是最快捷也是最有效果的方式,跟着视频中老师的思路,从基础到深入,还是很容易入门的。
二、Python爬虫
爬虫作为一个热门的方向,不管是在自己兼职还是当成辅助技能提高工作效率,都是很不错的选择。
通过爬虫技术可以将相关的内容收集起来,分析删选后得到我们真正需要的信息。
这个信息收集分析整合的工作,可应用的范畴非常的广泛,无论是生活服务、出行旅行、金融投资、各类制造业的产品市场需求等等,都能够借助爬虫技术获取更精准有效的信息加以利用。
Python爬虫视频资料
三、数据分析
清华大学经管学院发布的《中国经济的数字化转型:人才与就业》报告显示,2025年,数据分析人才缺口预计将达230万。
这么大的人才缺口,数据分析俨然是一片广阔的蓝海!起薪10K真的是家常便饭。
四、数据库与ETL数仓
企业需要定期将冷数据从业务数据库中转移出来存储到一个专门存放历史数据的仓库里面,各部门可以根据自身业务特性对外提供统一的数据服务,这个仓库就是数据仓库。
传统的数据仓库集成处理架构是ETL,利用ETL平台的能力,E=从源数据库抽取数据,L=将数据清洗(不符合规则的数据)、转化(对表按照业务需求进行不同维度、不同颗粒度、不同业务规则计算进行统计),T=将加工好的表以增量、全量、不同时间加载到数据仓库。
五、机器学习
机器学习就是对计算机一部分数据进行学习,然后对另外一些数据进行预测与判断。
机器学习的核心是“使用算法解析数据,从中学习,然后对新数据做出决定或预测”。也就是说计算机利用以获取的数据得出某一模型,然后利用此模型进行预测的一种方法,这个过程跟人的学习过程有些类似,比如人获取一定的经验,可以对新问题进行预测。
机器学习资料:
六、Python高级进阶
从基础的语法内容,到非常多深入的进阶知识点,了解编程语言设计,学完这里基本就了解了python入门到进阶的所有的知识点。
到这就基本就可以达到企业的用人要求了,如果大家还不知道去去哪找面试资料和简历模板,我这里也为大家整理了一份,真的可以说是保姆及的系统学习路线了。
但学习编程并不是一蹴而就,而是需要长期的坚持和训练。整理这份学习路线,是希望和大家共同进步,我自己也能去回顾一些技术点。不管是编程新手,还是需要进阶的有一定经验的程序员,我相信都可以从中有所收获。
一蹴而就,而是需要长期的坚持和训练。整理这份学习路线,是希望和大家共同进步,我自己也能去回顾一些技术点。不管是编程新手,还是需要进阶的有一定经验的程序员,我相信都可以从中有所收获。
资料领取
上述这份完整版的Python全套学习资料已经上传网盘,朋友们如果需要可以微信扫描下方二维码输入“领取资料” 即可自动领取
或者
【点此链接】领取
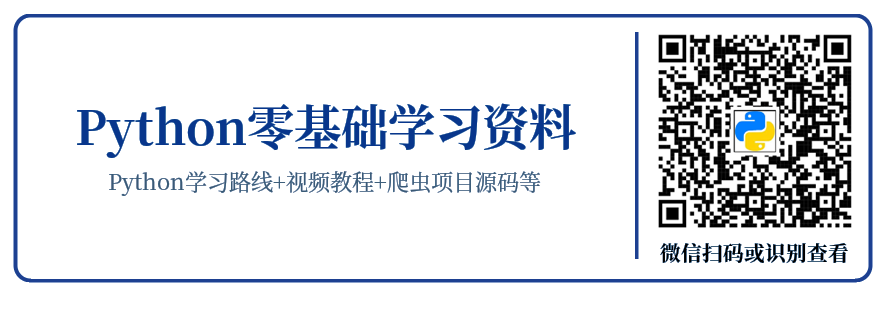
好文推荐
了解python的前景:https://blog.csdn.net/SpringJavaMyBatis/article/details/127194835
了解python的兼职副业:https://blog.csdn.net/SpringJavaMyBatis/article/details/127196603