哈希存储
基础哈希存储(哈希冲突明显)
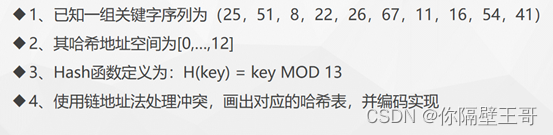
main.c
#include<stdio.h>
#include<stdlib.h>
#include"fun.h"
int main(int argc, const char *argv[])
{
int arr[N]={25,51,8,22,26,67,11,16,54,41};
datatype hash[P];
init_hash(hash);
for(int i=0;i<N;i++)
{
insert_hash(hash,arr[i]);
}
show_hash(hash);
search_hash(hash,22);
return 0;
}
fun.c
初始化哈希表
/初始化哈希表
void init_hash(datatype *hash)
{
for(int i=0;i<P;i++)
{
hash[i]='\0';
}
printf("初始化成功\n");
}
将元素存入哈希表
int insert_hash(datatype *hash,int x)
{
int index = x%P;
hash[index] = x;
return 0;
}
定义查看哈希表函数
void show_hash(datatype *hash)
{
for(int i=0;i<P;i++)
{
printf("%d\t:",i);
printf("%d\n",hash[i]);
}
printf("\n");
}
定义哈希查找函数
void search_hash(datatype *hash,int key)
{
int index = key%P;
if(key==hash[index])
{
printf("%d下标为%d\n",hash[index],index);
printf("您要查找的数据再表中\n");
}
}
fun.h
#ifndef __FUN_H__
#define __FUN_H__
#define N 10
#define P 13
typedef int datatype;
datatype hash[N];
void init_hash(datatype *hash);
int insert_hash(datatype *hash,int x);
void show_hash(datatype *hash);
void search_hash(datatype *hash,int key);
#endif
链地址法处理哈希冲突
main.c
#include<stdio.h>
#include<stdlib.h>
#include"hash.h"
int main(int argc, const char *argv[])
{
int arr[N]={25,51,8,22,26,67,11,16,54,41};
Node *hash[P];
init_hash(hash);
for(int i=0;i<N;i++)
{
insert_hash(hash,arr[i]);
}
show_hash(hash);
search_hash(hash,22);
return 0;
}
hash.c
初始化哈希表
void init_hash(Node *hash[])
{
for(int i=0;i<P;i++)
{
hash[i]=NULL;
}
printf("初始化成功\n");
}
将元素存入哈希表
int insert_hash(Node *hash[],int x)
{
int index = x%P;
Node *q = (Node*)malloc(sizeof(Node));
if(q==NULL)
{
printf("申请失败\n");
return -1;
}
q->data = x;
q->next = NULL;
q->next = hash[index];
hash[index] = q;
return 0;
}
定义查看哈希表函数
void show_hash(Node *hash[])
{
for(int i=0;i<P;i++)
{
printf("%d:",i);
Node *q = hash[i];
while (q!=NULL)
{
printf("%d->",q->data);
q=q->next;
}
printf("NULL\n");
}
}
定义哈希查找函数
void search_hash(Node *hash[],int key)
{
int index = key%P;
Node*q=hash[index];
while(q!=NULL &&q->data!=key)
{
q=q->next;
}
if(NULL==q)
{
printf("fail\n");
}else
{
printf("您要查找的数据再表中\n");
}
}
hash.h
#ifndef __hash_h__
#define __hash_h__
#define N 10
#define P 13
typedef int datatype;
typedef struct Node
{
datatype data;
struct Node *next;
}Node;
void init_hash(Node *hash[]);
int insert_hash(Node *hash[],int x);
void show_hash(Node *hash[]);
void search_hash(Node *hash[],int key);
#endif
排序方法
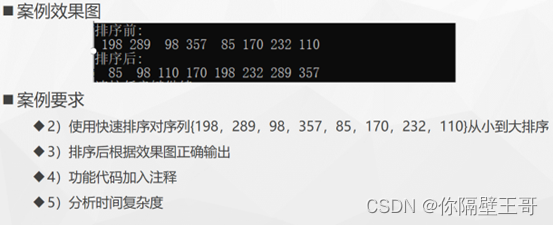
冒泡排序
#include<stdio.h>
void show(int *arr)
{
for(int i=0;i<8;i++)
{
printf("%d\t",arr[i]);
}
printf("\n");
}
int main(int argc, const char *argv[])
{
int temp,count=0;
int arr[8]={198,289,98,357,85,170,232,110};
for(int i=0;i<7;i++)
{
count=0;
for(int j=0;j<7-i;j++)
{
if(arr[j]>arr[j+1])
{
temp=arr[j];arr[j]=arr[j+1];arr[j+1]=temp;
count++;
}
}
if(count==0)
{
break;
}
}
show(arr);
return 0;
}
选择排序
#include<stdio.h>
void show(int *arr)
{
for(int i=0;i<8;i++)
{
printf("%d\t",arr[i]);
}
printf("\n");
}
int main(int argc, const char *argv[])
{
int min,count,temp=0;
int arr[8]={198,289,98,357,85,170,232,110};
for(int i=0;i<8;i++)
{
arr[min]=arr[i];
for(int j=i+1;j<8;j++)
{
if(arr[j]<arr[min])
{
min=j;
}
}
temp=arr[i];arr[i]=arr[min];arr[min]=temp;
}
show(arr);
return 0;
}
插入排序
#include<stdio.h>
void show(int *arr)
{
for(int i=0;i<8;i++)
{
printf("%d\t",arr[i]);
}
printf("\n");
}
int main(int argc, const char *argv[])
{
int i,j;
int temp;
int arr[8]={198,289,98,357,85,170,232,110};
for(i=1; i<8; i++)
{
int temp = arr[i];
for(j=i; j>0&&temp<arr[j-1]; j--)
{
arr[j]=arr[j-1];
}
arr[j] = temp;
}
show(arr);
return 0;
}
快速排序
int part(int *arr, int low, int high)
{
int x = arr[low];
while(low<high)
{
while(arr[high]>=x && low<high)
{
high--;
}
arr[low] = arr[high];
while(arr[low]<=x && low<high)
{
low++;
}
arr[high] = arr[low];
}
arr[low] = x;
return low;
}
void quick_sort(int *arr, int low, int high)
{
if(low < high)
{
int mid = part(arr, low, high);
quick_sort(arr, low, mid-1);
quick_sort(arr, mid+1, high);
}
}