一、Javascript
1. What is JS
定义 | A scripting language used for client-side web development. |
作用 | an implementation of the ECMAScript standard defines the syntax/characteristics of the language and a basic set of commonly used objects such as Number, Date supported in browsers supports additional objects(Window, Frame, Form, DOM object) |
One Java Script? | Different brands and/or different versions of browsers may support different implementations of JavaScript. – They are not fully compatible. – JScript is the Microsoft version of JavaScript. |
2. What can we do with JS
Create an interactive user interface in a webpage (eg. menu, pop-up alerts, windows) |
Manipulate web content dynamically – Change the content and style of an element – Replace images on a page without page reload – Hide/Show contents |
Generate HTML contents on the fly |
Form validation |
3. The lanuage and features
Validation | Validating forms before sending to server. |
Dynamic writing | Client-side language to manipulate all areas of the browser’s display, whereas servlets and PHPs run on server side. |
Dynamic typing | Interpreted language (interpreter in browser): dynamic typing. |
Event-handling | Event‐Driven Programming Model: event handlers. |
Scope and Functions | Heavyweight regular expression capability |
Arrays and Objects | Functional object‐oriented language with prototypical inheritance (class free), which includes concepts of objects, arrays, prototypes |
4. Interpreting JS
Commands are interpreted where they are found | – start with tag, then functions in <head> or in separate .js file |
– static JavaScript is run before is loaded | |
– output placed in HTML page • [document.write() ... dynamic scripting] • <SCRIPT> tags inside <BODY> |
二、 Validation
<HTML>
<HEAD>
<SCRIPT>
<!--验证表单数据-->
function validate(){
if (document.forms[0].elements[0].value==“”) {
alert(“please enter your email”);
<!--alert弹出一个警告框,提示用户输入他们的电子邮件地址-->
return false;
}
return true;
}
</SCRIPT>
</HEAD>
<!--FORM定义了一个表单-->
<!--method="get":使用GET方法来发送表单数据,将数据附加到URL的查询字符串中-->
<!--action="someURL":表单数据发送到的目标URL-->
<!--onSubmit="return validate()":在提交表单时调用函数"validate()"进行验证-->
<!--如果验证函数返回false,则表单不会被提交-->
<BODY>
<FORM method=“get” action=“someURL” onSubmit=“return validate()”>
<!--文本输入框使用<input>标签创建-->
<!--type="text":输入框的类型为文本,这个名称将在提交表单时用于标识输入框的值-->
<!--name="email":输入框的名称为"email"-->
<!--placeholder="":显示在输入框内的占位符文本,提示用户输入电子邮件地址-->
<input type=text name=“email”>enter your email<br>
<!--提交按钮使用<input>标签创建-->
<!--type="submit":指定按钮的类型为提交按钮-->
<!--value="send":按钮上显示的文本为"send"-->
<!--用户在文本输入框中输入完电子邮件地址并点击提交按钮时,表单将被提交,并调用函数"validate()"进行验证-->
<input type=submit value=“send”>
</FORM>
</BODY>
</HTML>
<html>
<head>
<script>
var greeting=“Hello”;
</script>
</head>
<body>
<script>
<!-- document.write()将带有 magenta 颜色的 "Hello" 和 "world!" 输出到页面中-->
<!--向页面写入一个 <font> 标签,并设置字体颜色为 magenta-->
document.write(“<FONT COLOR=‘magenta’>”);
<!--输出换行符 <br> 和文字 "world!"。通过 </FONT> 关闭之前打开的 <font> 标签-->
document.write(greeting);
document.write(“<br>world!</FONT>”);
</script>
</body>
</html>
<HTML>
<HEAD>
<!--type="text/javascript"是 <script> 属性之一,指定嵌入的脚本语言类型,此处为 JavaScript-->
<SCRIPT type="text/javascript">
function updateOrder() {
const cakePrice = 1;
var noCakes = document.getElementById("cake").value;
document.getElementById("total").value = "£" + cakePrice*noCakes;
}
function placeOrder(form){ form.submit(); }
</SCRIPT>
</HEAD>
<BODY>
<FORM method="get" action="someURL">
<H3>Cakes Cost £1 each</H3>
<input type=text name="cakeNo" id="cake"
onchange="updateOrder()">enter no. cakes<br>
<input type=text name="total" id="total">Total Price<br>
<input type=button value="send" onClick="placeOrder(this.form)">
</FORM>
</BODY>
</HTML>
三、 Dynamic Typing
1. Type is defined by literal value you assign to it
2. Implicit conversion between types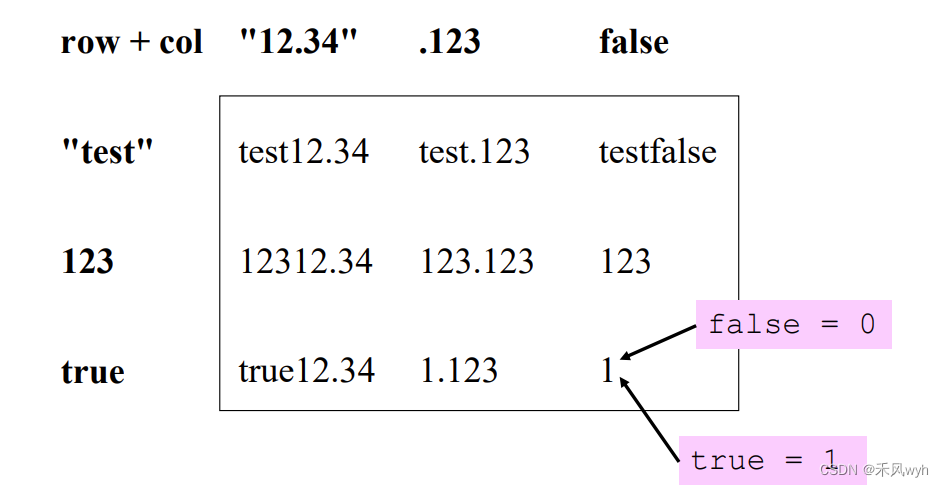
"string1"+"string2" = "string1string2" //string的拼接
10 + "string2"="10string2" //将int类型的10,转换成string
true + "string2" = "truestring2" //将Boolean类型转换为string
.123 + "string2" = ".123string2" //将float类型转换为string
10 + .123 = 10.123 //将int转换为float,进行数值加减
true + .123 = 1.123 //将Boolean类型转换为float,进行数值加减
false + 10 = 10 //将Boolean类型转换为int,进行数值加减
false + true = 1 //进行逻辑运算
a = 1; b = “20”;
c = a + b;//"120"
c = b + a;//"201"
// prefers numeric conversion with minus (and prefers string conversion with +).
c = a + (b-0);//21
// The empty string, forcing conversion into a string
c = a + “”;//"1"
x = 7; y = 4;
sum = x + y;//11
document.writeln(“x + y = ” + sum + “”); //11
document.writeln(“x + y = ” + x+y + “”); //74
document.writeln(“x + y = ” + (x+y) + “”); //括号中的表达式被当作数值相加运算,11
3. Quotes within strings&writeln
Quotes within strings | document.write(“He said, \"That’s mine\" ”); |
document.write(‘She said, "No it\'s not" '); | |
• Beware of splitting over lines | |
writeln()-new lines (avoid-not expected) | writeln: adds a return character after the text string |
<br>:get a line break on the page | |
using headers:document.write(“<H1>Top level header”<H1>); | |
document.write(‘<br>’): get a line break |
4. Comparison Operators
== and != do | perform type conversion before comparison “5”==5 is true |
=== and !== | do not perform type conversion “5”===5 is false |
|
5. Explicit Conversion Functions
eval() | takes a string parameter and evaluates it |
| |
parseInt() | returns the first integer in a string; if no integer returns NaN. |
| |
parseFloat() |
|
isNaN() | returns true if input is not a number |
| |
Concatenation issue with using +: implicit string conversion |
|
<HTML>
<HEAD>
<SCRIPT type="text/javascript">
function updateOrder() {
const cakePrice = 1;
const bunPrice = 0.5;
var noCakes = document.getElementById("cake").value;
var noBuns = document.getElementById("bun").value;
document.getElementById("count").value = parseInt(noCakes, 10)+parseInt(noBuns, 10);
document.getElementById("total").value = "£" + (cakePrice*noCakes +
bunPrice*noBuns);
}
function placeOrder(form) { form.submit(); }
</SCRIPT>
</HEAD>
<BODY>
<FORM method="get" action="someURL">
<H3>Cakes Cost £1 each; Buns 50p each</H3>
<input type=text name="cakeNo" id="cake" onchange="updateOrder()">enter no. cakes<br>
<input type=text name="bunNo" id="bun" onchange="updateOrder()">enter no. buns<br>
<input type=text name="count" id="count">number of units<br>
<input type=text name="total" id="total">Total Price<br>
<input type=button value="send" onClick="placeOrder(this.form)">
</FORM>
</BODY>
</HTML>
四、Event-handling
1. Event-Diven Programming
Event-Diven Programming | Web browser generates an event whenever anything ‘interesting’ occurs. |
registers an event handler | |
event handlers | <input type=text name=“phone” ___>enter phone no. |
focus event … onfocus event handler | |
blur event … onblur event handler | |
change event … onchange event handler | |
user types a char … onkeyup event handler | |
event handlers as HTML element attributes | <input type=“button” value=“push” onclick=validate()>
– Calls
validate()
function defined in
<head>
.
– Case insensitive (HTML):
onClick=validate()
.
– Can then force form to
submit
, e.g.
document.forms[0].submit();
|
Main version used in course examples: <form action=“someURL” onSubmit=“return validate()”> // textboxes
<input type=“submit” value=“send”> //
If
onSubmit=validate() [
omit ‘
return
’], this will still send the user input to the server side even if it fails the validation.
|
2. Feedback (Forms and JavaScript, Event Handling)
write the HTML: user guidance, 2 textboxes, button (not ‘submit’) |
– name the elements
|
getting the button |
|
Where and How to write JavaScript functions |
|
Global window object |
|
3. Dynamic Writing
Document Object Model & Empty String |
• API for HTML and XML documents:
-Provides
structural representation of a document
, so its content and visual presentation can be modified.
-Connects web pages to scripts or programming languages.
• Navigating to
elements
and accessing user input:
textboxes
,
textareas
...
|
| |
focus() and modifying the value |
|
Modifying the HTML page to notify |
|
<html>
<head>
<script type=“text/javascript”>
function validate() {
if (document.forms[0].usrname.value==“”) {
alert(“please enter name”);
document.forms[0].usrname.focus();
document.forms[0].usrname.value=“please enter name”;
document.getElementById(“a”).innerHTML=“please enter name above”;
}
}
</script>
</head>
<body>
<font face=“arial”>
<h1>Please enter details</h1>
<form>
Name <input type=“text” name=“usrname”> <br>
<font color=“red”> <p id="a"> </p></font>
<font face=“arial”>
Email <input type=“text” name=“email”> <br><br>
<input type=“button” value=“Send” onclick=“validate()”>
</form>
</body>
</html>
五、 Arrays and Objects
1. Strings & Intervals
Useful string functions for validation |
if (ph.charAt(i)>=‘A’ && ph.charAt(i) <= ‘Z’)
//
character at index
i
of this variable
if (ph.length < 9) //
the length of this variable
if (ph.substring(0, 1) == ‘,’) //
substring,
could first iterate through user input to extract all non-digits, then use substring to extract area code.
if (ph.indexOf('@') == 0) //
index of character (charAt
) ,
emails never start with
@
| |
setInterval()
|
| |
Handling Delays | setTimeout() | delay before execution |
setInterval() | interval between execution | |
clearInterval() | ||
clearTimeout() | ||
setTimeout(myFunc(), 1) | delay 1 ms | |
setTimeout(myFunc(),1000) | delay 1 second | |
myVar = setInterval(myFunc(), 1000) | ||
myVar = setInterval(myFunc(), 1000) |
2. JavaScript and Functions
JavaScript functions are objects | can be stored in objects, arrays and variables |
can be passed as arguments or provided as return values | |
can have methods (= function stored as property of an object) | |
can be invoked | |
call a function | (Local call) directly: var x = square(4); |
(Callback) in response to something happening: called by the browser, not by developer’s code: events; page update function in Ajax |
3. Arrays and Objects
Array Literal |
list = [“K”, “J”, “S”];
var list = [“K”, 4, “S”]; // mixed array
var emptyList = [];
|
var myArray = new Array(length);
// array constructor
employee = new Array(3);
employee[0] = “K”; employee[1] = “J”;
employee[2] = “S”;
document.write(employee[0]);
|
new Object() /
/A new blank object with no properties or methods.
employee = new Object();
employee[0] = “K”;
|
var a = new Array();
new Array(value0, value1, ...);
employee = new Array(“K”, “J”, “S”);
document.write(employee[0]);
| |
Sparse Arrays |
new Array(); // Length is
0
initially; automatically extends when new elements are initialised.
|
employee = new Array();
employee[5] = “J”; //
length:
6
employee[100] = “R”; //l
ength :
101
|
length is found as
employee.length
No array bounds errors.
| ||
Associative array or object |
|
|
4. Scope and local functions
html><head><title>Functions </title>
<script>
y = 6;
function square(x) {
var y = x*x; // if didn’t include var, then y is the global y
return y;
}
</script></head>
<body>
<script>
document.write(“The square of ” + y + “ is ”);//36
document.write(square(y));
document.write(“<P>y is ” + y);//6
</script>
</body></html>
JavaScript and Scope | C‐like languages have block scope:declare at first point of use |
JavaScript has function scope:variables defined within a function are visible everywhere in the function | |
declare at the start/top of the function body. | |
Inner functions can access actual parameters and variables (not copies) of their outer functions. |
六、Cookies
作用 |
allow you to store information between browser sessions, and
you can set their expiry date
|
默认 | browser session |
包含 | A name-value pair containing the actual data. |
An expiry date after which it is no longer valid. | |
The domain and path of the server it should be sent to. | |
Storing a cookie in a JavaScript program |
document.cookie = “version=” + 4;
document.cookie = “version=” +
encodeURIComponent(document.lastModified);
|
• Stores the name-value pair for the browser session.
• A cookie name cannot include semicolons,commas, or whitespace
– Hence the use of
encodeURIComponent(value)
.
– Must remember to
decodeURIComponent()
when reading the saved cookie.
| |
document.cookie = "version=" +
encodeURIComponent(document.lastModified) +
" ; max-age=" + (60*60*24*365);
| |
•
only
the name-value pair is stored in the name-value list associated with
document.cookie
.
• Attributes are stored by the system.
|
//A Cookie to remember the number of visits
<html>
<head>
<title>How many times have you been here before?</title>
<script type="text/javascript">
expireDate = new Date();
expireDate.setMonth(expireDate.getMonth()+6);
hitCt = parseInt(cookieVal("pageHit"));
hitCt++;
document.cookie= "pageHit=" + hitCt + ";expires=" + expireDate.toGMTString();
function cookieVal(cookieName) {
thisCookie = document.cookie.split("; ");
for (i=0; i<thisCookie.length; i++) {
if (cookieName == thisCookie[i].split("=")[0])
return thisCookie[i].split("=")[1];
}
return 0;
}
</script>
</head>
<body bgcolor="#FFFFFF">
<h2>
<script language="Javascript" type="text/javascript">
document.write("You have visited this page " + hitCt + " times.");
</script>
</h2>
</body>
</html>
七、PROBLEMS WITH USER INPUT
1. A Cautionary Tale: SQL Statements
Enter phone number to retrieve address |
– '
||'a'='a
– No validation
|
Name=value pair, PhoneNumber=‘||’a’=‘a, passed to server | Server side: access/update database information using SQL statements. |
• SELECT * FROM table_name WHERE phone=‘parameter’ | – SELECT * FROM table_name WHERE phone=‘02078825555’ – SELECT * FROM table_name WHERE phone=‘asdsdaEN3’
–
SELECT * FROM table_name WHERE phone=‘
’||’a’=‘a
’
• i.e.
SELECT * FROM table_name WHERE
phone=‘’ OR ‘a’=‘a’
|
2. Further Examples of Event Handling
Buttons | submitting to, e.g. CGI: <INPUT TYPE=“submit” value=“Send”> |
onClick: <INPUT TYPE=“button” onClick=“doFunc()”> | |
Text Boxes | <INPUT TYPE=“text” onFocus=“typeText()” onBlur=“typeNothing()”> |
When the document is first loaded |
<BODY οnlοad=doSomething()>
or
(inside
<script>
tags):
window.οnlοad=“doSomething”
|
//First set up array containing help text
<HTML>
<HEAD>
<TITLE>Forms</TITLE>
<SCRIPT>
var helpArray = [“Enter your name in this input box.”,“Enter your email address in this input box, \in the format user@domain.”,“Check this box if you liked our site.”,“In this box, enter any comments you would \like us to read.”,“This button submits the form to the \server-side script”,“This button clears the form”,“This TEXTAREA provides context-sensitive help. \Click on any input field or use the TAB key to \get more information about the input field.”];
function helpText(messageNum) {
document.myForm.helpBox.value = helpArray[messageNum];
}
function formSubmit() {
window.event.returnValue = false;
if (confirm(“Are you sure you want to submit?”))
window.event.returnValue = true;
// so in this case it now performs the action
}
function formReset() {
window.event.returnValue = false;
if (confirm(“Are you sure you want to reset?”))
window.event.returnValue = true;
}
</SCRIPT>
</HEAD>
<BODY>
<FORM NAME = “myForm” ONSUBMIT = “formSubmit()”
ACTION=http://localhost/cgi-bin/mycgi ONRESET = “return formReset()”>
Name: <INPUT TYPE = “text” NAME = “name” ONFOCUS = “helpText(0)” ONBLUR = “helpText(6)”><BR>
Email: <INPUT TYPE = “text” NAME = “email” ONFOCUS = “helpText(1)” ONBLUR = “helpText(6)”><BR>
Click here if you like this site
<INPUT TYPE = “checkbox” NAME = “like” ONFOCUS = “helpText(2)” ONBLUR = “helpText(6)”><BR>
Any comments?<BR>
<TEXTAREA NAME = “comments” ROWS = 5 COLS = 45 ONFOCUS = “helpText(3)” ONBLUR = “helpText(6)”></TEXTAREA><BR>
<INPUT TYPE = “submit” VALUE = “Submit” ONFOCUS = “helpText(4)” ONBLUR = “helpText(6)”>
<INPUT TYPE = “reset” VALUE = “Reset” ONFOCUS = “helpText(5)” ONBLUR = “helpText(6)”>
<TEXTAREA NAME = “helpBox” STYLE = “position: absolute; right:0; top: 0” ROWS = 4 COLS = 45>
This TEXTAREA provides context-sensitive help. Click on any input field or use the TAB key to get more information about the input field.</TEXTAREA>
</FORM>
</BODY>
</HTML>
//Element objects & ONLOAD
<HTML>
<HEAD>
<TITLE>Object Model</TITLE>
<SCRIPT>
function start() {
alert(pText.innerText);
pText.innerText = “Thanks for coming.”;
}
</SCRIPT>
</HEAD>
<BODY ONLOAD = “start()”>
<P ID = “pText”>Welcome to our Web page!</P>//Changes when alert box is clicked.
//pText.innerText or document.getElementById(“pText”).innerHTML
</BODY>
</HTML>
//More inner text – from Microsoft (Altering Text)
<P ID=oPara>Here's the text that will change.</P>
<BUTTON onclick=“oPara.innerText=‘WOW! It changed!’”>Change text</BUTTON>
<BUTTON onclick=“oPara.innerText=‘And back again’”>Reset</BUTTON>