栈
实现代码
#include <iostream>
using namespace std;
template <typename T>
class MyStack
{
private:
T *bottom;
T *top;
T *end;
public:
MyStack(int size=1)
{
bottom=new T[size];
top=bottom;
end=bottom+size;
}
~MyStack()
{
delete []bottom;
end=bottom=top=nullptr;
}
MyStack (const MyStack &other)
{
int len = other.top-other.bottom;
int size=other.end-other.bottom;
this->bottom=new T[size];
mencpy(this->bottom,other.bottom,len*sizeof(T));
this->top = this->bottom+len;
this->end = this->bottom+size;
}
MyStack& operator=(const MyStack &other)
{
int len = other.last-other.first;
int size=other.end-other.first;
this->first=new T[size];
mencpy(this->first,other.first,len*sizeof(T));
this->last = this->first+len;
this->end = this->first+size;
return *this;
}
bool empty()
{
return this->bottom == this->top;
}
bool full()
{
return this->top == this->end;
}
void expend()
{
int size=this->end-this->bottom;
T* temp = new T[2*size];
memcpy(temp,this->bottom,size*sizeof(T));
delete []bottom;
bottom =temp;
top=bottom+size;
end=bottom+2*size;
}
void push(const T value)
{
if(this->full())
{
this->expend();
}
*top=value;
top++;
}
T& pop()
{
if(this->empty())
{
throw -1;
}
top--;
return *top;
}
T& getTop() const
{
return *(top-1);
}
int size()
{
return end-bottom;
}
int len()
{
return top-bottom;
}
void show()
{
for(int i=0;i<len();i++)
{
cout<<bottom[i]<<" ";
}
cout<<endl;
}
};
int main()
{
MyStack<int> s;
for(int i=1;i<=10;i++)
{
s.push(i);
cout<<"栈容量:"<<s.size()<<endl;
}
cout<<"栈元素:";
s.show();
try {
cout<<"出栈:"<<s.pop()<<endl;
cout<<"出栈:"<<s.pop()<<endl;
cout<<"出栈:"<<s.pop()<<endl;
} catch (int e) {
if(e==-1)
{
cout<<"栈空,出栈失败"<<endl;
}
}
cout<<"栈元素:";
s.show();
return 0;
}
运行结果
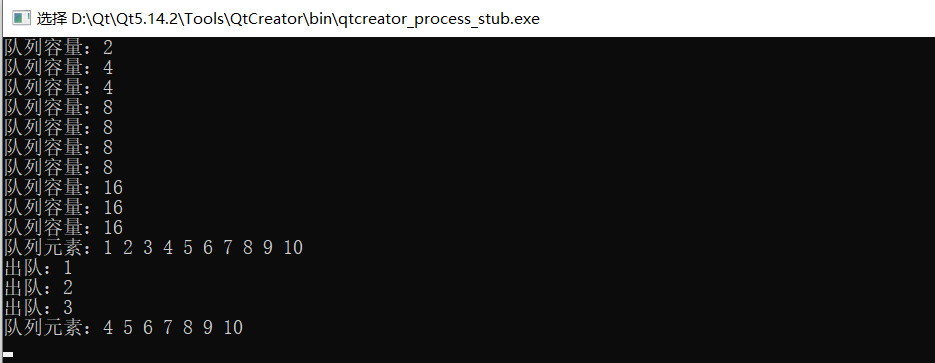
队列
实现代码
#include <iostream>
using namespace std;
template <typename T>
class MyQueue
{
private:
int front;
int rear;
T *first;
int size;
public:
MyQueue(int size=1)
{
first=new T[size];
rear=front=0;
this->size=size;
}
~MyQueue()
{
delete []first;
size=front=rear=0;
}
MyQueue (const MyQueue &other)
{
this->first=new T[other.size];
this->size=other.size;
mencpy(this->first,other.first,size*sizeof(T));
this->rear = other.rear;
this->front = other.front;
}
MyQueue& operator=(const MyQueue &other)
{
this->first=new T[other.size];
this->size=other.size;
mencpy(this->first,other.first,size*sizeof(T));
this->rear = other.rear;
this->front = other.front;
return *this;
}
bool empty()
{
return this->front == this->rear;
}
bool full()
{
return this->front == (this->rear+1)%size;
}
void expend()
{
T* temp = new T[2*size];
memcpy(temp,this->first,size*sizeof(T));
delete []first;
first =temp;
size=2*size;
}
void push(const T value)
{
if(this->full())
{
this->expend();
}
first[rear]=value;
rear=(rear+1)%size;
}
T& pop()
{
if(this->empty())
{
throw -1;
}
int temp=front;
front=(front+1)%size;
return first[temp];
}
T& getfront() const
{
return first[front];
}
T& getrear() const
{
return first[rear];
}
int getSize()
{
return this->size;
}
int len()
{
return (rear-front+size)%size;
}
void show()
{
for(int i=front;i<front+len();i++)
{
cout<<first[i]<<" ";
}
cout<<endl;
}
};
int main()
{
MyQueue<int> q;
for(int i=1;i<=10;i++)
{
q.push(i);
cout<<"队列容量:"<<q.getSize()<<endl;
}
cout<<"队列元素:";
q.show();
try {
cout<<"出队:"<<q.pop()<<endl;
cout<<"出队:"<<q.pop()<<endl;
cout<<"出队:"<<q.pop()<<endl;
} catch (int e) {
if(e==-1)
{
cout<<"队列为空,出队失败"<<endl;
}
}
cout<<"队列元素:";
q.show();
return 0;
}
运行结果
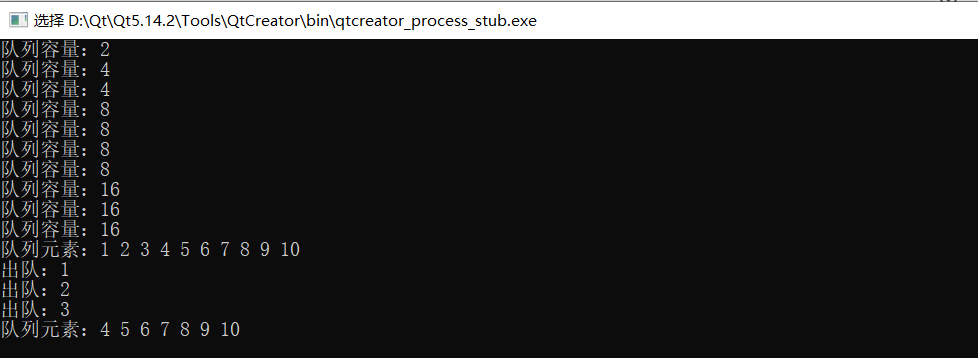