获取文件夹下的文件名、大小信息
可根据自己的需要选择选择按钮输出。默认排序按照修改日期排序,可选择按照文件大小升序或降序列排列,效果图如下所示(图为选中显示名称+字节显示大小+默认修改时间排序)
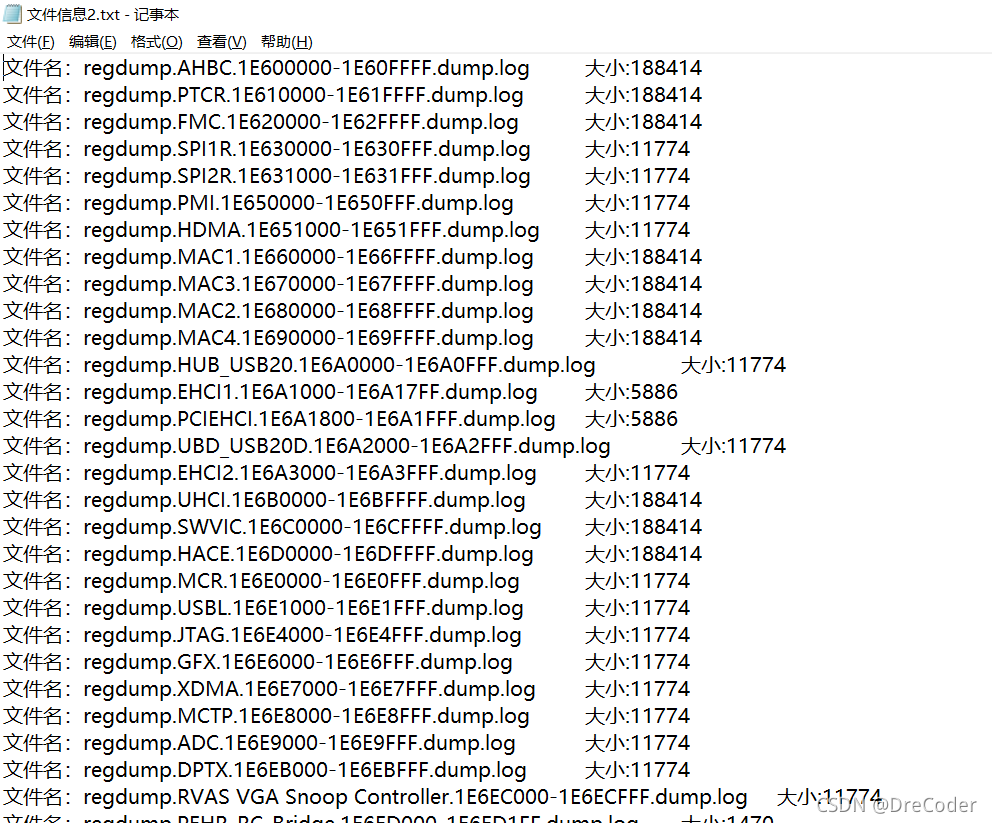
附件下载地址 https://download.csdn.net/download/xya644/24417354
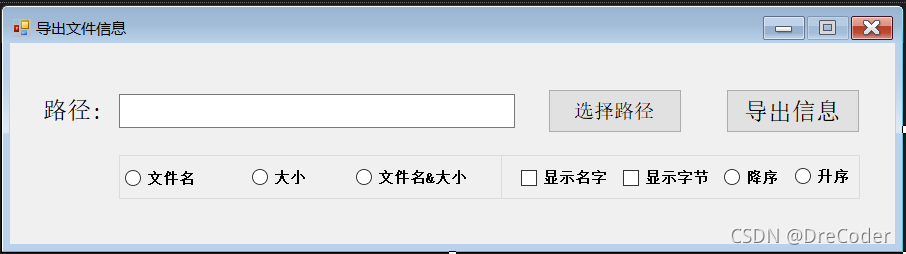
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.IO;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace 获取文件大小并保存在指定路径下
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
System.IO.FileInfo fileInfo = null;//用于判断文件大小
/// <summary>
/// 获取文件大小
/// </summary>
/// <param name="filePath">文件路径</param>
/// <returns></returns>
private long GetFileSize(string filePath)
{
try
{
fileInfo = new System.IO.FileInfo(filePath);
}
catch
{
return 0;
}
if (fileInfo != null && fileInfo.Exists)
{
return fileInfo.Length;
}
else
{
return 0;
}
}
string btnPathStr = "";
static string SelectedPath = System.IO.Directory.GetCurrentDirectory();
private void button1_Click(object sender, EventArgs e)
{
try
{
FolderBrowserDialog folderDlg = new FolderBrowserDialog();
folderDlg.SelectedPath = SelectedPath;
if (folderDlg.ShowDialog() == DialogResult.OK)
{
btnPathStr = folderDlg.SelectedPath;
SelectedPath = btnPathStr;
}
textBox1.Text = btnPathStr;
}
catch
{
MessageBox.Show("打开路径出错");
}
}
List<long> mylist = new List<long>();
Dictionary<string, long> keyValuePairs2 = null;
private void button3_Click(object sender, EventArgs e)
{
try
{
List<string> list = getDirFileNameStr();
for (int i = 0; i < list.Count; i++)
{
long size = GetFileSize(@textBox1.Text + "\\" + list[i]);
mylist.Add(size);
}
if (rbxJiang.Checked)
{
AnaFileDicInfo(list, @textBox1.Text);
Dictionary<string, long> keyValuePairs = dic;
var temp = from pair in keyValuePairs orderby pair.Value descending select pair;
keyValuePairs2 = temp.ToDictionary<KeyValuePair<string, long>, string, long>(pair => pair.Key, pair => pair.Value);
}
else if (rbxSheng.Checked)
{
AnaFileDicInfo(list, @textBox1.Text);
Dictionary<string, long> keyValuePairs = dic;
var temp = from pair in keyValuePairs orderby pair.Value ascending select pair;
keyValuePairs2 = temp.ToDictionary<KeyValuePair<string, long>, string, long>(pair => pair.Key, pair => pair.Value);
}
else
{
AnaFileDicInfo(list, @textBox1.Text);
}
SaveFile();
}
catch
{
MessageBox.Show("处理信息出错", "系统提示");
}
}
private List<string> getDirFileNameStr()
{
List<string> list = new List<string>();
if (textBox1.Text == null)
return null;
string dirPath = btnPathStr; //文件夹路径`
DirectoryInfo mydir = new DirectoryInfo(dirPath);
FileInfo[] files = mydir.GetFiles();
System.IO.FileInfo ftmp;
for (int i = 0; i < files.Length - 1; i++)
for (int j = i + 1; j < files.Length; j++)
if (files[i].LastWriteTime > files[j].LastWriteTime)
{
ftmp = files[i];
files[i] = files[j];
files[j] = ftmp;
}
foreach (FileInfo info in files)
{
list.Add(info.Name);
}
return list;
}
Dictionary<string, long> dic = new Dictionary<string, long>();
private void AnaFileDicInfo(List<string> tempList, string path)
{
dic.Clear();//防止添加相同的值造成报错
for (int i = 0; i < tempList.Count; i++)
{
dic.Add(tempList[i], GetFileSize(@path + "\\" + tempList[i]));
}
}
private string GetLength(long ByteLength)
{
if (ByteLength < 1024)
{
return string.Format(ByteLength.ToString() + 'B');
}
else if (ByteLength > 1024 && ByteLength <= Math.Pow(1024, 2))
{
return string.Format("{0:N2}KB",(ByteLength / 1024.0));
}
else if (ByteLength > Math.Pow(1024, 2) && ByteLength <= Math.Pow(1024, 3))
{
return string.Format("{0:N2}M",(ByteLength / 1024.0 / 1024.0));
}
else
{
return string.Format("{0:N2}GB",(ByteLength / 1024.0 / 1024.0 / 1024.0));
}
}
private string GetShowStr(Dictionary<string,long> dic1)
{
string str = "";
if (cbxShowNameStr.Checked)
{
if (rbfile.Checked)
{
foreach (var item in dic1)
{
//string tempSrt = GetLength(item.Value);
str += string.Format("文件名:{0}\r\n", item.Key);
}
}
else if (rbSize.Checked)
{
if (cbxByte.Checked)
{
foreach (var item in dic1)
{
str += string.Format("大小:{0}\r\n",item.Value);
}
}
else
{
foreach (var item in dic1)
{
string tempSrt = GetLength(item.Value);
str += string.Format("大小:{0}\r\n",tempSrt);
}
}
}
else if (rbFileAndSize.Checked)
{
if (cbxByte.Checked)
{
foreach (var item in dic1)
{
str += string.Format("文件名:{0} \t 大小:{1}\r\n", item.Key,item.Value);
}
}
else
{
foreach (var item in dic1)
{
string tempSrt = GetLength(item.Value);
str += string.Format("文件名:{0} \t 大小:{1}\r\n", item.Key, tempSrt);
}
}
}
}
else {
if (rbfile.Checked)
{
foreach (var item in dic1)
{
//string tempSrt = GetLength(item.Value);
str += string.Format("{0}\r\n", item.Key);
}
}
else if (rbSize.Checked)
{
if (cbxByte.Checked)
{
foreach (var item in dic1)
{
str += string.Format("{0}\r\n",item.Value);
}
}
else {
foreach (var item in dic1)
{
string tempSrt = GetLength(item.Value);
str += string.Format("{0}\r\n", tempSrt);
}
}
}
else if (rbFileAndSize.Checked)
{
if (cbxByte.Checked)
{
foreach (var item in dic1)
{
str += string.Format("{0} \t {1}\r\n",item.Key,item.Value);
}
}
else
{
foreach (var item in dic1)
{
string tempSrt = GetLength(item.Value);
str += string.Format("{0} \t {1}\r\n", item.Key, tempSrt);
}
}
}
}
return str;
}
private void SaveFile()
{
SaveFileDialog sfd = new SaveFileDialog();
sfd.Filter = "文本文件|*.txt";
sfd.InitialDirectory = SelectedPath;
sfd.FileName = "文件信息";
if (sfd.ShowDialog() == DialogResult.Cancel)
return;
var path = sfd.FileName;
SelectedPath = path;
string writeStr = "";
if (File.Exists(@path))
{
File.Delete(@path);
}
if (rbxJiang.Checked ||rbxSheng.Checked)
{
writeStr = GetShowStr(keyValuePairs2);
}
else
{
writeStr = GetShowStr(dic);
}
try
{
FileStream fwrite = new FileStream(path, FileMode.OpenOrCreate, FileAccess.Write);
byte[] data = System.Text.Encoding.Default.GetBytes(writeStr);
using (fwrite)
{
fwrite.Write(data, 0, data.Length);
}
MessageBox.Show("保存成功","系统提示");
}
catch
{
MessageBox.Show("保存失败","系统提示");
}
}
private void Form1_Load(object sender, EventArgs e)
{
rbFileAndSize.Checked = true;
cbxShowNameStr.Checked = true;
}
}
}