一:普通饼图(动态)
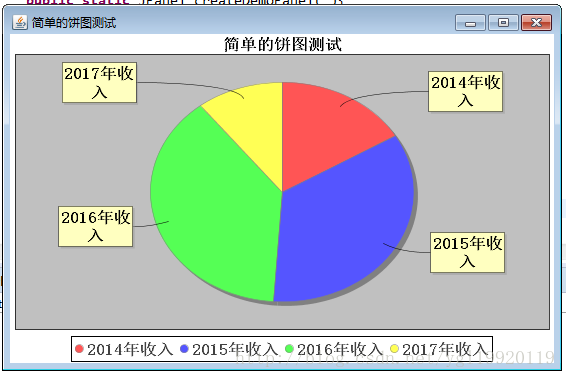
package test;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.Random;
import javax.swing.JPanel;
import javax.swing.Timer;
import org.jfree.chart.ChartFactory;
import org.jfree.chart.ChartPanel;
import org.jfree.chart.JFreeChart;
import org.jfree.chart.plot.PiePlot;
import org.jfree.chart.title.TextTitle;
import org.jfree.data.general.DefaultPieDataset;
import org.jfree.data.general.PieDataset;
import org.jfree.ui.ApplicationFrame;
import org.jfree.ui.RefineryUtilities;
public class PieChartTest extends ApplicationFrame {
/**
* serialVersionUID = 24654896134861354L;
*/
private static final long serialVersionUID = 24654896134861354L;
public static Timer timer = null;
public static DefaultPieDataset dataset = null;
static PiePlot pieplot = null;
public static final Font font = new Font("宋体", Font.BOLD, 16);
public PieChartTest( String title ){
super( title );
setContentPane(createDemoPanel( ));
}
private static PieDataset createDataset( ){
dataset = new DefaultPieDataset( );
dataset.setValue( "2014年收入" , new Double( new Random().nextInt(100) ) );
dataset.setValue( "2015年收入" , new Double( new Random().nextInt(100) ) );
dataset.setValue( "2016年收入" , new Double( new Random().nextInt(100) ) );
dataset.setValue( "2017年收入" , new Double( new Random().nextInt(100) ) );
return dataset;
}
private static JFreeChart createChart( PieDataset dataset ){
JFreeChart chart = ChartFactory.createPieChart(
"简单的饼图测试",
dataset,
true,
true,
false);
return chart;
}
private static JFreeChart chart = null;
public static JPanel createDemoPanel( ){
chart = createChart(createDataset( ) );
pieplot = (PiePlot) chart.getPlot();
pieplot.setLabelFont(font);
TextTitle texttitle = chart.getTitle();
texttitle.setFont(font);
pieplot.setNoDataMessage("No data available");
pieplot.setCircular(false);
pieplot.setLabelGap(0.02);
chart.getLegend().setItemFont(font);
return new ChartPanel( chart );
}
public static void main( String[ ] args ){
PieChartTest demo = new PieChartTest( "简单的饼图测试" );
demo.setSize( 560 , 367 );
RefineryUtilities.centerFrameOnScreen( demo );
demo.setVisible( true );
refresh();
}
public static void refresh(){
int delay = 2000;
ActionListener taskTimer = new ActionListener() {
public void actionPerformed(ActionEvent evt) {
if(pieplot!=null){
pieplot.setDataset(createDataset());
}
}
};
timer = new Timer(delay,taskTimer);
timer.start();
}
}
二:取出饼图中的一块
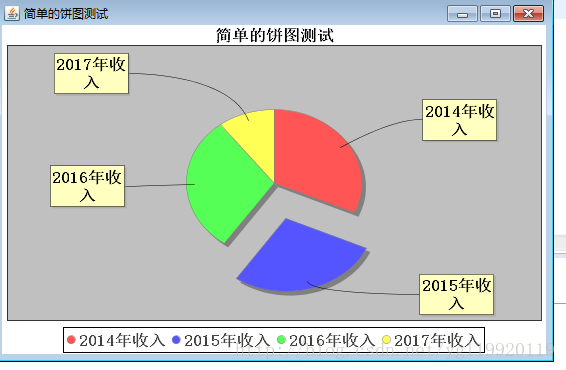
package test;
import java.awt.Font;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.Random;
import javax.swing.JPanel;
import javax.swing.Timer;
import org.jfree.chart.ChartFactory;
import org.jfree.chart.ChartPanel;
import org.jfree.chart.JFreeChart;
import org.jfree.chart.plot.PiePlot;
import org.jfree.chart.title.TextTitle;
import org.jfree.data.general.DefaultPieDataset;
import org.jfree.data.general.PieDataset;
import org.jfree.ui.ApplicationFrame;
import org.jfree.ui.RefineryUtilities;
public class PieChartTest extends ApplicationFrame {
/**
* serialVersionUID = 24654896134861354L;
*/
private static final long serialVersionUID = 24654896134861354L;
public static Timer timer = null;
public static DefaultPieDataset dataset = null;
static PiePlot pieplot = null;
public static final Font font = new Font("宋体", Font.BOLD, 16);
public PieChartTest( String title ){
super( title );
setContentPane(createDemoPanel( ));
}
private static PieDataset createDataset( ){
dataset = new DefaultPieDataset( );
dataset.setValue( "2014年收入" , new Double( new Random().nextInt(100) ) );
dataset.setValue( "2015年收入" , new Double( new Random().nextInt(100) ) );
dataset.setValue( "2016年收入" , new Double( new Random().nextInt(100) ) );
dataset.setValue( "2017年收入" , new Double( new Random().nextInt(100) ) );
return dataset;
}
private static JFreeChart createChart( PieDataset dataset ){
JFreeChart chart = ChartFactory.createPieChart(
"简单的饼图测试",
dataset,
true,
true,
false);
return chart;
}
private static JFreeChart chart = null;
public static JPanel createDemoPanel( ){
chart = createChart(createDataset( ) );
pieplot = (PiePlot) chart.getPlot();
pieplot.setLabelFont(font);
TextTitle texttitle = chart.getTitle();
texttitle.setFont(font);
pieplot.setExplodePercent("2015年收入", 0.5);
pieplot.setNoDataMessage("No data available");
pieplot.setCircular(false);
pieplot.setLabelGap(0.02);
chart.getLegend().setItemFont(font);
return new ChartPanel( chart );
}
public static void main( String[ ] args ){
PieChartTest demo = new PieChartTest( "简单的饼图测试" );
demo.setSize( 560 , 367 );
RefineryUtilities.centerFrameOnScreen( demo );
demo.setVisible( true );
refresh();
}
public static void refresh(){
int delay = 2000;
ActionListener taskTimer = new ActionListener() {
public void actionPerformed(ActionEvent evt) {
if(pieplot!=null){
pieplot.setDataset(createDataset());
}
}
};
timer = new Timer(delay,taskTimer);
timer.start();
}
}