import random
import sqlite3
class Role:
def __init__(self, name, level, exp, health):
self.name = name
self.level = level
self.exp = exp
self.health = health
def attack(self, opponent):
attack_points = random.randint(5, 20) + self.level
print("{} 对 {} 造成了 {} 点伤害。".format(self.name, opponent.name, attack_points))
opponent.health -= attack_points
def is_alive(self):
return self.health > 0
class Monster:
def __init__(self, name, level, exp, health):
self.name = name
self.level = level
self.exp = exp
self.health = health
def attack(self, opponent):
attack_points = random.randint(5, 15) + self.level
print("{} 对 {} 造成了 {} 点伤害。".format(self.name, opponent.name, attack_points))
opponent.health -= attack_points
def is_alive(self):
return self.health > 0
roles = [
Role("孙悟空", 1, 0, 100),
Role("猪八戒", 1, 0, 120),
Role("沙僧", 1, 0, 80)
]
monsters = [
Monster("黑熊精", 1, 10, 80),
Monster("白骨精", 3, 30, 150),
Monster("铁扇公主", 5, 50, 200),
Monster("红孩儿", 8, 80, 300),
Monster("黄袍怪", 10, 100, 400)
]
conn = sqlite3.connect('game.db')
def create_table():
conn.execute('''CREATE TABLE IF NOT EXISTS ROLES
(ID INTEGER PRIMARY KEY AUTOINCREMENT,
NAME TEXT NOT NULL,
LEVEL INT NOT NULL,
EXP INT NOT NULL,
HEALTH INT NOT NULL);''')
conn.execute('''CREATE TABLE IF NOT EXISTS MONSTERS
(ID INTEGER PRIMARY KEY AUTOINCREMENT,
NAME TEXT NOT NULL,
LEVEL INT NOT NULL,
EXP INT NOT NULL,
HEALTH INT NOT NULL);''')
conn.execute('''CREATE TABLE IF NOT EXISTS MONSTERS
(ID INTEGER PRIMARY KEY AUTOINCREMENT,
NAME TEXT NOT NULL,
LEVEL INT NOT NULL,
EXP INT NOT NULL,
HEALTH INT NOT NULL);''')
conn.commit()
def save_role(role):
sql = '''INSERT INTO ROLES (NAME, LEVEL, EXP, HEALTH)
VALUES (?, ?, ?, ?)'''
conn.execute(sql, (role.name, role.level, role.exp, role.health))
conn.commit()
def save_monster(monster):
sql = '''INSERT INTO MONSTERS (NAME, LEVEL, EXP, HEALTH)
VALUES (?, ?, ?, ?)'''
conn.execute(sql, (monster.name, monster.level, monster.exp, monster.health))
conn.commit()
def load_role(name):
cursor = conn.execute("SELECT * from ROLES WHERE NAME = ?", (name,))
row = cursor.fetchone()
if row is None:
return None
else:
role = Role(row[1], row[2], row[3], row[4])
return role
def load_monster(name):
cursor = conn.execute("SELECT * from MONSTERS WHERE NAME = ?", (name,))
row = cursor.fetchone()
if row is None:
return None
else:
monster = Monster(row[1], row[2], row[3], row[4])
return monster
def fight(role, monster):
print("{} 开始对战 {}。".format(role.name, monster.name))
while role.is_alive() and monster.is_alive():
role.attack(monster)
if not monster.is_alive():
print("{} 打败了 {},获得了 {} 点经验值".format(role.name, monster.name, monster.exp))
role.exp += monster.exp
if role.exp >= role.level * 50:
print("{} 升级了!当前等级为 {}。".format(role.name, role.level + 1))
role.level += 1
role.exp = 0
save_role(role)
break
monster.attack(role)
if not role.is_alive():
print("{} 被 {} 打败了!".format(role.name, monster.name))
break
def main():
create_table()
for role in roles:
exist_role = load_role(role.name)
if exist_role is None:
save_role(role)
for monster in monsters:
exist_monster = load_monster(monster.name)
if exist_monster is None:
save_monster(monster)
for role in roles:
print("{} 进入了游戏。".format(role.name))
for i in range(5):
monster = random.choice(monsters)
print("{} 遇到了 {} 等级为 {} 的怪物。".format(role.name, monster.name, monster.level))
fight(role, monster)
if __name__ == "__main__":
main()
conn.close()
故事设计类
最新推荐文章于 2024-08-24 14:37:29 发布
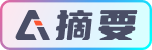