文章目录
1 说明
本文介绍 Odin Inspector 插件中条件特性的使用方法。
2 条件特性
2.1 DisableIf / EnableIf
如果解析的字符串计算结果为指定值,则禁用 / 启用 Inspector 窗口中的属性更改。
string condition
检查值条件的解析字符串,如成员名称、表达式等。
object optionalValue
要检查的值。
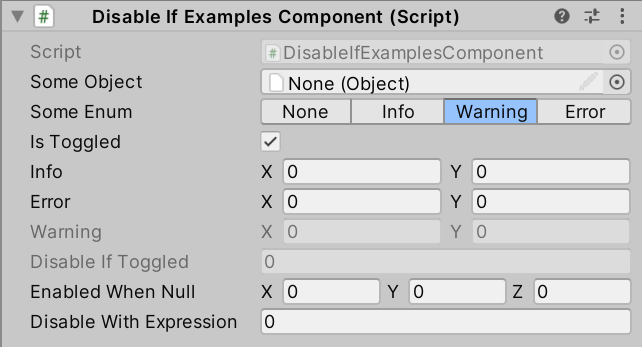
// DisableIfExamplesComponent.cs
using Sirenix.OdinInspector;
using UnityEngine;
public class DisableIfExamplesComponent : MonoBehaviour
{
public UnityEngine.Object SomeObject;
[EnumToggleButtons]
public InfoMessageType SomeEnum;
public bool IsToggled;
[DisableIf("SomeEnum", InfoMessageType.Info)]
public Vector2 Info;
[DisableIf("SomeEnum", InfoMessageType.Error)]
public Vector2 Error;
[DisableIf("SomeEnum", InfoMessageType.Warning)]
public Vector2 Warning;
[DisableIf("IsToggled")]
public int DisableIfToggled;
[DisableIf("SomeObject")]
public Vector3 EnabledWhenNull;
[DisableIf("@this.IsToggled && this.SomeObject != null || this.SomeEnum == InfoMessageType.Error")]
public int DisableWithExpression;
}
2.2 DisableIn / EnableIn / ShowIn / HideIn
当该对象所在的脚本挂载在何种预制体上,其修饰的对象禁止 / 允许在 Inspector 窗口中的更改 / 显示。
PrefabKind prefabKind
预制体的种类。
None
所有预制体,都不满足条件。
InstanceInScene
场景中的预制体实例。
InstanceInPrefab
嵌套在其他预制体中的预制件实例。
Regular
常规预制体。
Variant
预制体资产。
NonPrefabInstance
非预制体或场景中的游戏对象实例。
PrefabInstance = InstanceInPrefab | InstanceInScene
常规预制体的实例,以及场景中或嵌套在其他预制体中的预制体。
PrefabAsset = Variant | Regular
常规预制体和预制体资产。
PrefabInstanceAndNonPrefabInstance = PrefabInstance | NonPrefabInstance
预制体以及非预制实例。
All = PrefabInstanceAndNonPrefabInstance | PrefabAsset
所有预制体。
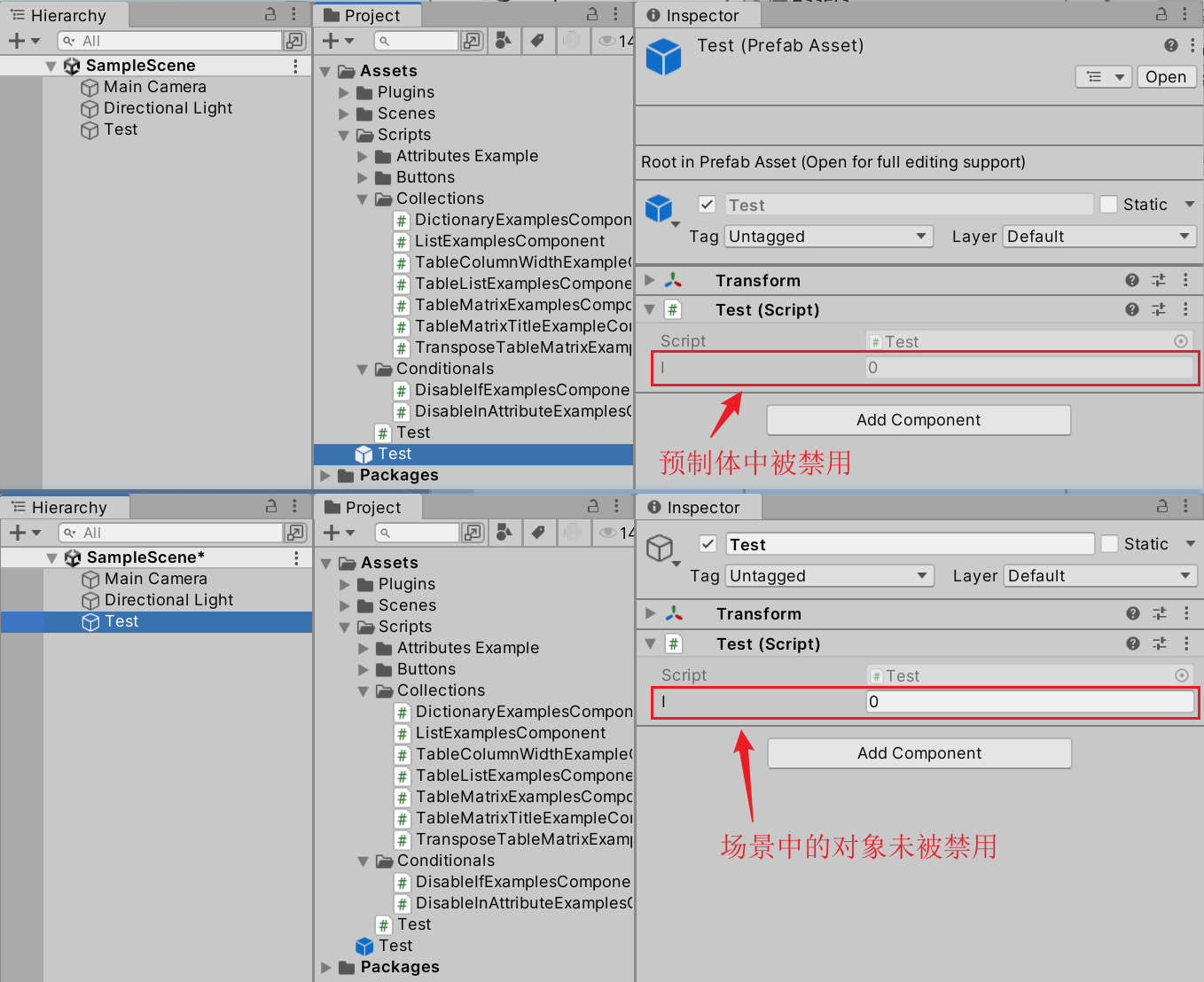
using System.Collections;
using System.Collections.Generic;
using Sirenix.OdinInspector;
using UnityEngine;
public class Test : MonoBehaviour
{
[DisableIn(PrefabKind.PrefabAsset)]
public int i;
}
2.3 DisableInEditorMode / HideInEditorMode
在不处于播放模式时禁用 / 隐藏对象。只希望在播放模式下可编辑 / 显示时,可使用此选项。
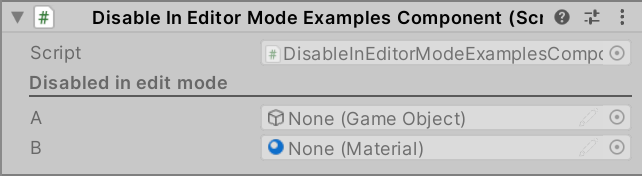
// DisableInEditorModeExamplesComponent.cs
using Sirenix.OdinInspector;
using UnityEngine;
public class DisableInEditorModeExamplesComponent : MonoBehaviour
{
[Title("Disabled in edit mode")]
[DisableInEditorMode]
public GameObject A;
[DisableInEditorMode]
public Material B;
}
2.4 DisableInInlineEditors / ShowInInlineEditors / HideInInlineEditors
如果对象被 InlineEditor 特性绘制,则该对象在 Inspector 窗口中禁用更改 / 显示 / 隐藏。
补充 InlineEditor 特性:将继承 UnityEngine.Object 的类(如 ScriptableObject)的详细信息显示在 Inspector 窗口。
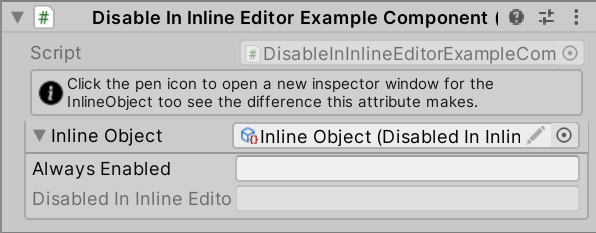
数据结构类:
using UnityEngine;
#nullable disable
namespace Sirenix.OdinInspector.Editor.Examples
{
public class DisabledInInlineEditorScriptableObject : ScriptableObject
{
public string AlwaysEnabled;
[DisableInInlineEditors]
public string DisabledInInlineEditor;
}
}
挂载的脚本:
// DisableInInlineEditorExampleComponent.cs
using Sirenix.OdinInspector;
using UnityEngine;
#if UNITY_EDITOR // Editor namespaces can only be used in the editor.
using Sirenix.OdinInspector.Editor.Examples;
#endif
public class DisableInInlineEditorExampleComponent : MonoBehaviour
{
#if UNITY_EDITOR // DisabledInInlineEditorScriptableObject is an example type and only exists in the editor
[InfoBox("Click the pen icon to open a new inspector window for the InlineObject too see the difference this attribute makes.")]
[InlineEditor(Expanded = true)]
public DisabledInInlineEditorScriptableObject InlineObject;
#endif
#if UNITY_EDITOR // Editor-related code must be excluded from builds
[OnInspectorInit]
private void CreateData() {
InlineObject = ExampleHelper.GetScriptableObject<DisabledInInlineEditorScriptableObject>("Inline Object");
}
[OnInspectorDispose]
private void CleanupData() {
if (InlineObject != null) Object.DestroyImmediate(InlineObject);
}
#endif
}
2.5 DisableInPlayMode / HideInPlayMode
在处于播放模式时禁用 / 隐藏对象。只希望在编辑模式下可编辑 / 显示时,可使用此选项。
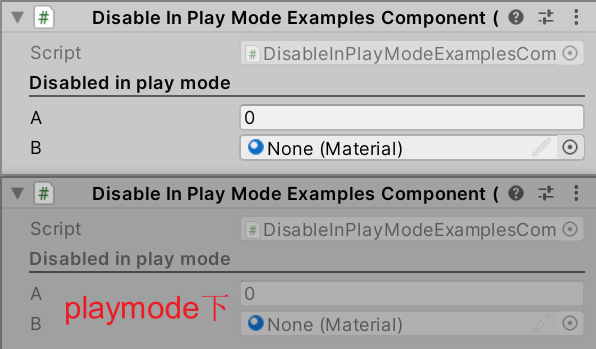
// DisableInPlayModeExamplesComponent.cs
using Sirenix.OdinInspector;
using UnityEngine;
public class DisableInPlayModeExamplesComponent : MonoBehaviour
{
[Title("Disabled in play mode")]
[DisableInPlayMode]
public int A;
[DisableInPlayMode]
public Material B;
}
2.6 ShowIf / HideIf
如果解析的字符串计算结果为指定值,则在 Inspector 窗口中显示 / 隐藏该对象。
string condition
检查值条件的解析字符串,如成员名称、表达式等。
object optionalValue
要检查的值。
bool animate = true
状态改变时是否启用滑入和滑出动画显示。
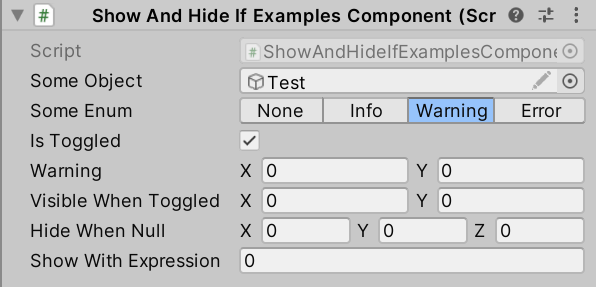
// ShowAndHideIfExamplesComponent.cs
using Sirenix.OdinInspector;
using UnityEngine;
public class ShowAndHideIfExamplesComponent : MonoBehaviour
{
// Note that you can also reference methods and properties or use @expressions. You are not limited to fields.
public UnityEngine.Object SomeObject;
[EnumToggleButtons]
public InfoMessageType SomeEnum;
public bool IsToggled;
[ShowIf("SomeEnum", InfoMessageType.Info)]
public Vector3 Info;
[ShowIf("SomeEnum", InfoMessageType.Warning)]
public Vector2 Warning;
[ShowIf("SomeEnum", InfoMessageType.Error)]
public Vector3 Error;
[ShowIf("IsToggled")]
public Vector2 VisibleWhenToggled;
[HideIf("IsToggled")]
public Vector3 HiddenWhenToggled;
[HideIf("SomeObject")]
public Vector3 ShowWhenNull;
[ShowIf("SomeObject")]
public Vector3 HideWhenNull;
[EnableIf("@this.IsToggled && this.SomeObject != null || this.SomeEnum == InfoMessageType.Error")]
public int ShowWithExpression;
}
2.7 ShowIfGroup / HideIfGroup
满足某个条件,则显示 / 隐藏指定组。
string path
指定组的路径。
object value
与路径末尾的标签重名的属性等于该值时,则满足条件。
bool animate = true
状态改变时是否启用滑入和滑出动画显示。
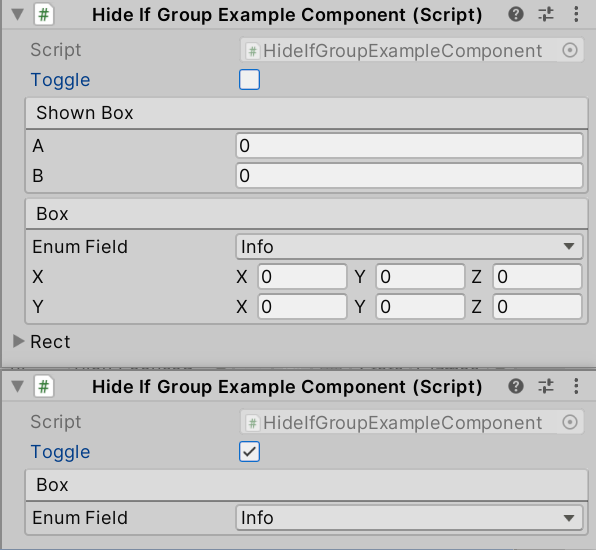
// HideIfGroupExampleComponent.cs
using Sirenix.OdinInspector;
using UnityEngine;
public class HideIfGroupExampleComponent : MonoBehaviour
{
public bool Toggle = true;
[HideIfGroup("Toggle")]
[BoxGroup("Toggle/Shown Box")]
public int A, B;
[BoxGroup("Box")]
public InfoMessageType EnumField = InfoMessageType.Info;
[BoxGroup("Box")]
[HideIfGroup("Box/Toggle")]
public Vector3 X, Y;
// Like the regular If-attributes, HideIfGroup also supports specifying values.
// You can also chain multiple HideIfGroup attributes together for more complex behaviour.
[HideIfGroup("Box/Toggle/EnumField", Value = InfoMessageType.Info)]
[BoxGroup("Box/Toggle/EnumField/Border", ShowLabel = false)]
public string Name;
[BoxGroup("Box/Toggle/EnumField/Border")]
public Vector3 Vector;
// HideIfGroup will by default use the name of the group,
// but you can also use the MemberName property to override this.
[HideIfGroup("RectGroup", Condition = "Toggle")]
public Rect Rect;
}