import java.awt.Color;
import java.awt.Graphics;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import javax.swing.JFrame;
import javax.swing.JPanel;public class PlatformGame extends JPanel implements KeyListener {
private int playerX, playerY;
private int monsterX, monsterY;
private int platformY;
private boolean isJumping;
private boolean isGameOver;
private boolean isGameWon;
private int level;
private int monsterSpeed;public PlatformGame() {
JFrame frame = new JFrame("Platform Game");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(600, 400);
frame.addKeyListener(this);
frame.add(this);
frame.setVisible(true);
playerX = 50;
playerY = 300;
monsterX = 400;
monsterY = 280;
platformY = 350;
isJumping = false;
isGameOver = false;
isGameWon = false;
level = 1;
monsterSpeed = 2;
}@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
if (!isGameOver && !isGameWon) {
g.setColor(Color.BLUE);
g.fillRect(playerX, playerY, 20, 20);
g.setColor(Color.RED);
g.fillRect(monsterX, monsterY, 30, 30);
g.setColor(Color.GREEN);
g.fillRect(0, platformY, 600, 50);
} else {
g.setColor(Color.BLACK);
if (isGameOver) {
g.drawString("Game Over", 280, 180);
} else {
g.drawString("Congratulations! You Won!", 240, 180);
}
}
}public void jump() {
if (!isJumping && !isGameOver && !isGameWon) {
isJumping = true;
Thread jumpThread = new Thread(new Runnable() {
@Override
public void run() {
for (int i = 0; i < 100; i++) {
playerY -= 2;
checkCollision();
checkPlatform();
checkWin();
repaint();
try {
Thread.sleep(10);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
for (int i = 0; i < 100; i++) {
playerY += 2;
checkCollision();
checkPlatform();
checkWin();
repaint();
try {
Thread.sleep(10);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
isJumping = false;
}
});
jumpThread.start();
}
}public void moveMonster() {
if (!isGameOver && !isGameWon) {
Thread monsterThread = new Thread(new Runnable() {
@Override
public void run() {
while (!isGameOver && !isGameWon) {
monsterX += monsterSpeed;
if (monsterX <= 0 || monsterX >= 570) {
monsterSpeed *= -1;
}
try {
Thread.sleep(10);
} catch (InterruptedException e) {
e.printStackTrace();
}
checkCollision();
repaint();
}
}
});
monsterThread.start();
}
}public void checkCollision() {
if (playerX + 20 >= monsterX && playerX <= monsterX + 30 && playerY + 20 >= monsterY && playerY <= monsterY + 30) {
isGameOver = true;
}
}public void checkPlatform() {
if (playerY >= platformY) {
platformY -= 50;
monsterY -= 50;
level++;
if (level % 5 == 0) {
moveMonster();
}
}
}public void checkWin() {
if (platformY <= 0) {
isGameWon = true;
}
}@Override
public void keyPressed(KeyEvent e) {
int key = e.getKeyCode();
if (key == KeyEvent.VK_SPACE) {
jump();
}
}@Override
public void keyReleased(KeyEvent e) {
}@Override
public void keyTyped(KeyEvent e) {
}public static void main(String[] args) {
new PlatformGame();
}
}
由Java实现的一个平台小游戏
最新推荐文章于 2024-10-01 09:56:52 发布
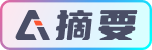