C# 调用C++ 非托管代码
将C++代码打包为DLL文件
- 工具
- Visual Studio 2019
创建C++ DLL 工程
一、定义宏(必须)
//SuperDLLS.h
// 符号视为是被导出的。
#ifdef SUPERDLLS_EXPORTS
#define SUPERDLLS_API __declspec(dllexport)
#else
#define SUPERDLLS_API __declspec(dllimport)
#endif
二、定义要导出的函数
//SuperDLLS.h
EXTERN_C SUPERDLLS_API int Add(int x, int y);
EXTERN_C SUPERDLLS_API float Sub(float x, float y);
EXTERN_C SUPERDLLS_API double Mul(double x, double y);
EXTERN_C SUPERDLLS_API long Division(long x, long y);
三、实现要导出的函数
//SuperDLLS.cpp
int Add(int x, int y)
{
return x + y;
}
float Sub(float x, float y)
{
return x - y;
}
double Mul(double x, double y)
{
return x * y;
}
long Division(long x, long y)
{
return x / y;
}
四、定义要导出的C++类(不能直接导出使用,需要使用一个类包装器)
创建并定义头文件(SuperDLLS.h)
//SuperDLLS.h
class SuperDLLS {
public:
SuperDLLS(void);
int AddC(int x, int y);
float SubC(float x, float y);
double MulC(double x, double y);
long DivisionC(long x, long y);
};
创建并定义CPP文件(SuperDLLS.cpp)
SuperDLLS::SuperDLLS()
{
cout << "constructor new SuperDLLS" << endl;
}
int SuperDLLS::AddC(int x, int y)
{
return x + y;
}
float SuperDLLS::SubC(float x, float y)
{
return x - y;
}
double SuperDLLS::MulC(double x, double y)
{
return x * y;
}
long SuperDLLS::DivisionC(long x, long y)
{
return x / y;
}
五、将需要导出的类包装起来供外部调用
创建并定义头文件(SuperDLLSWrapper.h)
// SuperDLLSWrapper.h
#include "SuperDLLS.h"
namespace SuperDLLSWrapper {
extern "C" SUPERDLLS_API int __stdcall AddC(int x, int y);
extern "C" SUPERDLLS_API float __stdcall SubC(float x, float y);
extern "C" SUPERDLLS_API double __stdcall MulC(double x, double y);
extern "C" SUPERDLLS_API long __stdcall DivisionC(long x, long y);
}
创建并定义cpp文件(SuperDLLSWrapper.cpp)
#include "pch.h"
#include "SuperDLLSWrapper.h"
namespace SuperDLLSWrapper {
SuperDLLS* csuperdll = new SuperDLLS();
int AddC(int n1, int n2) {
return csuperdll->AddC(n1, n2);
}
float SubC(float n1, float n2) {
return csuperdll->SubC(n1, n2);
}
double MulC(double n1, double n2) {
return csuperdll->MulC(n1, n2);
}
long DivisionC(long n1, long n2) {
return csuperdll->DivisionC(n1, n2);
}
}
六、生成时,请根据自己的调用平台的架构打包DLL,否者识别不了(x64请打x64 DLL,x86 请打x86 DLL)
创建C#端测试代码
一、创建一个控制台应用程序,并将上一步生成的DLL 拷贝至当前目录下
- 使用工具
- Visual Studio Code
- C# 版本
- .Net Core 3.1
二、创建一个链接类,用于读取DLL中的函数信息
创建并定义SampleClassWrapper.cs类如下
using System;
using System.Runtime.InteropServices;
public static class SampleClassWrapper
{
[DllImport(@"SUPERDLLS.dll")]
public static extern int Add(int n1, int n2);
[DllImport(@"SUPERDLLS.dll")]
public static extern float Sub(float n1, float n2);
[DllImport(@"SUPERDLLS.dll")]
public static extern double Mul(double x, double y);
[DllImport(@"SUPERDLLS.dll")]
public static extern long Division(long n1, long n2);
[DllImport(@"SUPERDLLS.dll")]
public static extern int AddC(int n1, int n2);
[DllImport(@"SUPERDLLS.dll")]
public static extern float SubC(float n1, float n2);
[DllImport(@"SUPERDLLS.dll")]
public static extern double MulC(double n1, double n2);
[DllImport(@"SUPERDLLS.dll")]
public static extern long DivisionC(long n1, long n2);
}
测试代码如下:
using System;
using System.Reflection;
using System.Collections.Generic;
using System.IO;
using System.Net;
using System.Runtime.Serialization.Formatters.Binary;
class Program
{
static void Main(string[] args)
{
Console.WriteLine(SampleClassWrapper.Add(10000, 2));
Console.WriteLine(SampleClassWrapper.Sub(1.29544f, 2.43358874f));
Console.WriteLine(SampleClassWrapper.Mul(12.0005, 59.98775));
Console.WriteLine(SampleClassWrapper.Division(336644, 3355555555));
Console.WriteLine(SampleClassWrapper.AddC(10000, 2));
Console.WriteLine(SampleClassWrapper.SubC(1.29544f, 2.43358874f));
Console.WriteLine(SampleClassWrapper.MulC(12.0005, 59.98775));
Console.WriteLine(SampleClassWrapper.DivisionC(336644, 3355555555));
}
}
输出代码如下:
更多内容,欢迎关注:
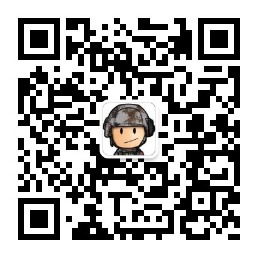