LATCH描述符简介
Learned Arrangements of Three Patch Codes(LATCH)是2015年CVPR关于二值化描述符优化变种二值特征描述子。经典二值描述子LBP等主要通过计算特征点局部窗口内n个邻域点对进行比较值来形成bit串,形成bit串后通过计算汉明码来提升计算速度。相关二值改进描述子使用滤波算法来降低局部邻域噪声的干扰,但是也一定程度降低局部邻域的唯一性。LATCH作者提出计算窗口内像素块比较值来形成bit串,同时作者提出如何定位像素块方法。当然,LATCH描述符匹配性能要高于先前的二值描述特征,低于非二值描述特征;计算耗时高于二值化特征,低于非二值化特征。因此,LATCH描述符处于一个折中方案策略,感觉速度不如ORB、AKAZE等算法,性能不如SIFT、SURF等算法。不过,可能通过组合特征+描述符会发挥其作用。相关具体细节参考论文LATCH或者作者官网。
OpenCV代码LATCH匹配:
// LATCH_match.cpp : 定义控制台应用程序的入口点。
#include "stdafx.h"
#include <iostream>
#include "opencv2/opencv_modules.hpp"
#ifdef HAVE_OPENCV_XFEATURES2D
#include <opencv2/features2d.hpp>
#include <opencv2/xfeatures2d.hpp>
#include <opencv2/imgcodecs.hpp>
#include <opencv2/opencv.hpp>
#include <vector>
// If you find this code useful, please add a reference to the following paper in your work:
// Gil Levi and Tal Hassner, "LATCH: Learned Arrangements of Three Patch Codes", arXiv preprint arXiv:1501.03719, 15 Jan. 2015
using namespace std;
using namespace cv;
const float inlier_threshold = 2.5f; // Distance threshold to identify inliers
const float nn_match_ratio = 0.8f; // Nearest neighbor matching ratio
int main(void)
{ //-- 待匹配图像对读取
Mat img1 = imread("..//LATCH_match//images//img1.png", IMREAD_GRAYSCALE);
Mat img2 = imread("..//LATCH_match//images//img3.png", IMREAD_GRAYSCALE);
//-- 读取同质矩阵
Mat homography;
FileStorage fs("..//LATCH_match//images//H1to3p.xml", FileStorage::READ);
fs.getFirstTopLevelNode() >> homography;
//-- 初始化特征点与描述子
vector<KeyPoint> kpts1, kpts2;
Mat desc1, desc2;
Ptr<cv::ORB> orb_detector = cv::ORB::create(10000);
// opencv-contrib xfeatures2d 初始化LATCH描述符
Ptr<xfeatures2d::LATCH> latch = xfeatures2d::LATCH::create();
/**
@param images Image set.
@param keypoints Input collection of keypoints. Keypoints for which a descriptor cannot be
computed are removed. Sometimes new keypoints can be added, for example: SIFT duplicates keypoint
with several dominant orientations (for each orientation).
@param descriptors Computed descriptors. In the second variant of the method descriptors[i] are
descriptors computed for a keypoints[i]. Row j is the keypoints (or keypoints[i]) is the
descriptor for keypoint j-th keypoint.
*/
//CV_WRAP virtual void compute(InputArrayOfArrays images,
// CV_OUT CV_IN_OUT std::vector<std::vector<KeyPoint> >& keypoints,
// OutputArrayOfArrays descriptors);
///** Detects keypoints and computes the descriptors */
//CV_WRAP virtual void detectAndCompute(InputArray image, InputArray mask,
// CV_OUT std::vector<KeyPoint>& keypoints,
// OutputArray descriptors,
// bool useProvidedKeypoints = false);
orb_detector->detect(img1, kpts1);
latch->compute(img1, kpts1, desc1);
//latch->detectAndCompute();
orb_detector->detect(img2, kpts2);
latch->compute(img2, kpts2, desc2);
BFMatcher matcher(NORM_HAMMING);
vector< vector<DMatch> > nn_matches;
matcher.knnMatch(desc1, desc2, nn_matches, 2);
vector<KeyPoint> matched1, matched2, inliers1, inliers2;
vector<DMatch> good_matches;
for (size_t i = 0; i < nn_matches.size(); i++) {
DMatch first = nn_matches[i][0];
float dist1 = nn_matches[i][0].distance;
float dist2 = nn_matches[i][1].distance;
if (dist1 < nn_match_ratio * dist2) {
matched1.push_back(kpts1[first.queryIdx]);
matched2.push_back(kpts2[first.trainIdx]);
}
}
for (unsigned i = 0; i < matched1.size(); i++) {
Mat col = Mat::ones(3, 1, CV_64F);
col.at<double>(0) = matched1[i].pt.x;
col.at<double>(1) = matched1[i].pt.y;
col = homography * col;
col /= col.at<double>(2);
double dist = sqrt(pow(col.at<double>(0) - matched2[i].pt.x, 2) +
pow(col.at<double>(1) - matched2[i].pt.y, 2));
if (dist < inlier_threshold) {
int new_i = static_cast<int>(inliers1.size());
inliers1.push_back(matched1[i]);
inliers2.push_back(matched2[i]);
good_matches.push_back(DMatch(new_i, new_i, 0));
}
}
Mat res;
drawMatches(img1, inliers1, img2, inliers2, good_matches, res);
imwrite("latch_result.png", res);
double inlier_ratio = inliers1.size() * 1.0 / matched1.size();
cout << "LATCH Matching Results" << endl;
cout << "*******************************" << endl;
cout << "# Keypoints 1: \t" << kpts1.size() << endl;
cout << "# Keypoints 2: \t" << kpts2.size() << endl;
cout << "# Matches: \t" << matched1.size() << endl;
cout << "# Inliers: \t" << inliers1.size() << endl;
cout << "# Inliers Ratio: \t" << inlier_ratio << endl;
cout << endl;
imshow("result", res);
waitKey(0);
return 0;
}
#else
int main()
{
std::cerr << "OpenCV was built without xfeatures2d module" << std::endl;
return 0;
}
#endif
LATCH示例匹配结果
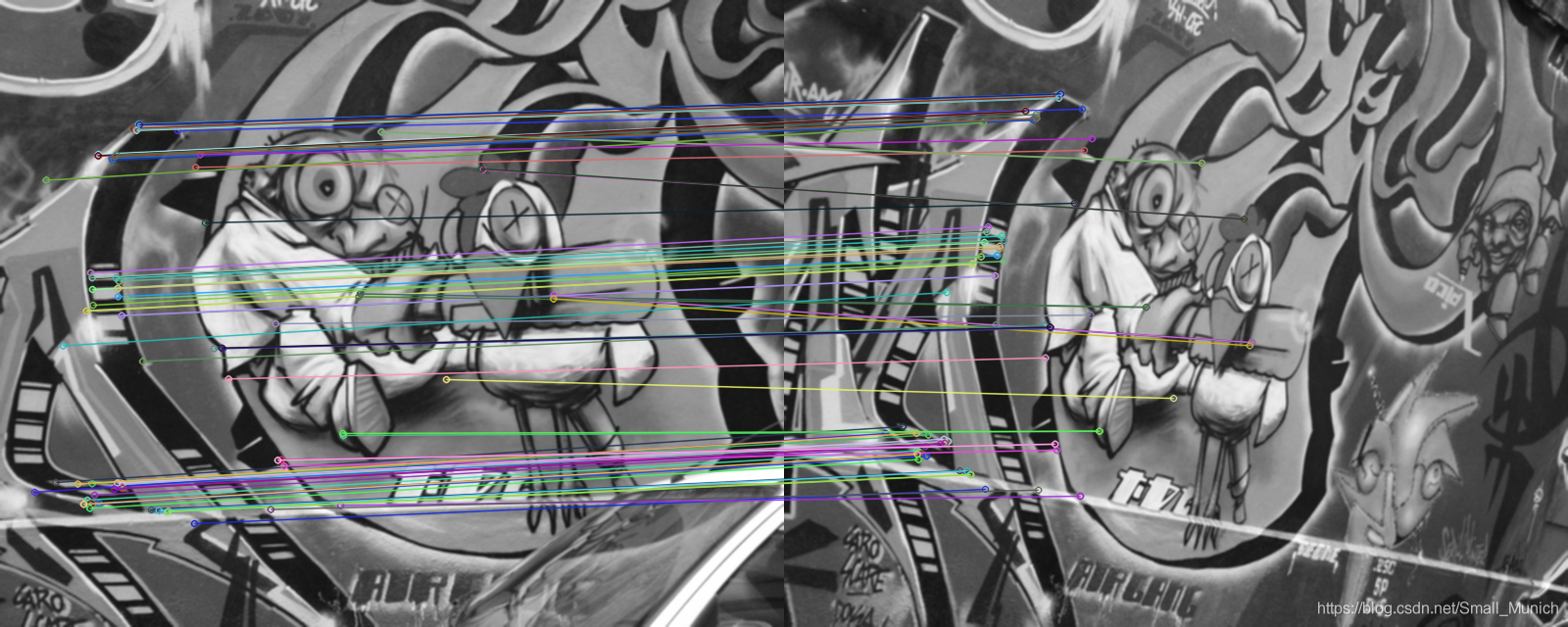
参考文献
https://arxiv.org/pdf/1501.03719.pdf
https://gilscvblog.wordpress.com/
https://docs.opencv.org/4.0.0-beta/