wordpress文本框
If you’ve ever used WordPress to build a website for yourself or a client, or you work for a company whose website is powered by WordPress, you would have seen and used meta boxes.
如果您曾经使用WordPress为自己或客户建立网站,或者为一家以WordPress为动力的公司工作,那么您会看到并使用过meta框。
In the past, we’ve covered adding custom meta boxes to WordPress. In this article, we’ll go two steps further explaining their relationship and integration with post types, including how to use the data saved in a meta box in the WordPress front-end.
过去,我们已经介绍了向WordPress添加自定义元框 。 在本文中,我们将走两步,进一步说明它们与帖子类型的关系和集成,包括如何使用保存在WordPress前端的元框中的数据。
将元框添加到帖子类型屏幕 (Adding Meta Boxes to Post Types Screen)
Most (if not all) of the PHP functions, their parameters and Action
hooks that are handy in creating meta boxes have been covered by Narayan Prusty.
Narayan Prusty涵盖了大多数(如果不是全部)PHP函数,它们的参数和在创建元框时很方便的Action
挂钩。
To add a meta box to the any post type editing screen, the add_meta_box()
is used and subsequently hooked to the add_meta_boxes
action.
要将元框添加到任何帖子类型编辑屏幕,请使用add_meta_box()
,然后将其挂钩到add_meta_boxes
操作。
The code below adds a metabox to the post
edit screen. Take note of global_notice_meta_box_callback
, the function that is called to display the form field(s) in the meta box. We’ll come to that later.
下面的代码将一个metabox添加到post
编辑屏幕。 注意global_notice_meta_box_callback
,该函数被调用以在meta框中显示表单字段。 我们稍后再讨论。
function global_notice_meta_box() {
add_meta_box(
'global-notice',
__( 'Global Notice', 'sitepoint' ),
'global_notice_meta_box_callback',
'post'
);
}
add_action( 'add_meta_boxes', 'global_notice_meta_box' );
To add a meta box to a number of post types screens – post
, page
and a book
custom post type; create an array of the post types, iterate over the array and use add_meta_box()
to add the meta box to them.
向多个帖子类型屏幕添加元框- post
, page
和book
自定义帖子类型; 创建一个帖子类型的数组,遍历该数组,然后使用add_meta_box()
向其中添加元框。
function global_notice_meta_box() {
$screens = array( 'post', 'page', 'book' );
foreach ( $screens as $screen ) {
add_meta_box(
'global-notice',
__( 'Global Notice', 'sitepoint' ),
'global_notice_meta_box_callback',
$screen
);
}
}
add_action( 'add_meta_boxes', 'global_notice_meta_box' );
To add a meta box to all existing post types and those to be created in future, use get_post_types()
to get an array of the post types and then replace the value of $screen
above with it.
要将元框添加到所有现有的帖子类型以及将来要创建的帖子类型,请使用get_post_types()
获取帖子类型的数组,然后将上面的$screen
值替换为它。
function global_notice_meta_box() {
$screens = get_post_types();
foreach ( $screens as $screen ) {
add_meta_box(
'global-notice',
__( 'Global Notice', 'sitepoint' ),
'global_notice_meta_box_callback',
$screen
);
}
}
add_action( 'add_meta_boxes', 'global_notice_meta_box' );
Adding a meta box to all existing and new post types can also be done by leaving out the third ($screen
) argument like so:
也可以通过省略第三个( $screen
)参数来将元框添加到所有现有和新的帖子类型中,如下所示:
function global_notice_meta_box() {
add_meta_box(
'global-notice',
__( 'Global Notice', 'sitepoint' ),
'global_notice_meta_box_callback'
);
}
add_action( 'add_meta_boxes', 'global_notice_meta_box' );
A meta box can also be restricted to a post type (book
in this example) by appending the post type name to add_meta_boxes
action hook as follows:
元框也可以被限制在一个柱类型( book
通过附加交类型名称在此实例中) add_meta_boxes
动作钩如下:
function global_notice_meta_box() {
add_meta_box(
'global-notice',
__( 'Global Notice', 'sitepoint' ),
'global_notice_meta_box_callback'
);
}
add_action( 'add_meta_boxes_book', 'global_notice_meta_box' );
Among the array argument used by register_post_type()
for customizing a custom post type is the register_meta_box_cb
in which its value is a callback function that is called when setting up the meta boxes.
register_post_type()
用于自定义自定义帖子类型的数组参数包括register_meta_box_cb
,其中其值是设置元框时调用的回调函数。
Say we created a book
custom post type with the following code:
假设我们使用以下代码创建了book
自定义帖子类型:
function book_cpt() {
$args = array(
'label' => 'Books',
'public' => true,
'register_meta_box_cb' => 'global_notice_meta_box'
);
register_post_type( 'book', $args );
}
add_action( 'init', 'book_cpt' );
Adding the add_meta_box()
function definition for creating a meta box inside a global_notice_meta_box
PHP function (value of register_meta_box_cb
above) will add the meta box to the book
custom post type edit screen.
添加add_meta_box()
的内部创建一个元包函数定义global_notice_meta_box
PHP函数(价值register_meta_box_cb
以上)将元框添加到book
自定义后类型编辑画面。
And again, here is our example global_notice_meta_box
function.
再次,这是我们的示例global_notice_meta_box
函数。
function global_notice_meta_box() {
add_meta_box(
'global-notice',
__( 'Global Notice', 'sitepoint' ),
'global_notice_meta_box_callback'
);
}
So far, we’ve learned the various ways of registering or adding meta boxes to WordPress. We are yet to create the global_notice_meta_box_callback
function that will contain the form field of our meta box.
到目前为止,我们已经学习了向WordPress注册或添加元框的各种方法。 我们尚未创建global_notice_meta_box_callback
函数,该函数将包含我们的元框的表单字段。
Below is the code for the global_notice_meta_box_callback
function that will include a text area field in the meta box.
下面是global_notice_meta_box_callback
函数的代码,该函数将在meta框中包含一个文本区域字段。
function global_notice_meta_box_callback( $post ) {
// Add a nonce field so we can check for it later.
wp_nonce_field( 'global_notice_nonce', 'global_notice_nonce' );
$value = get_post_meta( $post->ID, '_global_notice', true );
echo '<textarea style="width:100%" id="global_notice" name="global_notice">' . esc_attr( $value ) . '</textarea>';
}

The save_post
action hook handles saving the data entered into the text area when the post is saved as draft or published.
当帖子另存为草稿或发布时, save_post
操作钩子将保存输入到文本区域的数据的保存。
/**
* When the post is saved, saves our custom data.
*
* @param int $post_id
*/
function save_global_notice_meta_box_data( $post_id ) {
// Check if our nonce is set.
if ( ! isset( $_POST['global_notice_nonce'] ) ) {
return;
}
// Verify that the nonce is valid.
if ( ! wp_verify_nonce( $_POST['global_notice_nonce'], 'global_notice_nonce' ) ) {
return;
}
// If this is an autosave, our form has not been submitted, so we don't want to do anything.
if ( defined( 'DOING_AUTOSAVE' ) && DOING_AUTOSAVE ) {
return;
}
// Check the user's permissions.
if ( isset( $_POST['post_type'] ) && 'page' == $_POST['post_type'] ) {
if ( ! current_user_can( 'edit_page', $post_id ) ) {
return;
}
}
else {
if ( ! current_user_can( 'edit_post', $post_id ) ) {
return;
}
}
/* OK, it's safe for us to save the data now. */
// Make sure that it is set.
if ( ! isset( $_POST['global_notice'] ) ) {
return;
}
// Sanitize user input.
$my_data = sanitize_text_field( $_POST['global_notice'] );
// Update the meta field in the database.
update_post_meta( $post_id, '_global_notice', $my_data );
}
add_action( 'save_post', 'save_global_notice_meta_box_data' );
To put the data that would be entered into the meta box text area to use, we’ll display the data before the post content that it’s saved against is displayed.
要将要输入的数据放入元框文本区域中使用,我们将在显示针对其保存的帖子内容之前显示数据。
function global_notice_before_post( $content ) {
global $post;
// retrieve the global notice for the current post
$global_notice = esc_attr( get_post_meta( $post->ID, '_global_notice', true ) );
$notice = "<div class='sp_global_notice'>$global_notice</div>";
return $notice . $content;
}
add_filter( 'the_content', 'global_notice_before_post' );
代码说明 (Code Explanation)
First, we created a global_notice_before_post
function hooked into the_content
filter with a $content
parameter which contains the post content.
首先,我们创建了一个global_notice_before_post
勾搭成函数the_content
与过滤$content
包含了帖子内容的参数。
Inside the function, we include the global $post
variable that contains the WP_Post
object of the current post that is being viewed.
在函数内部,我们包含全局$post
变量,该变量包含正在查看的当前帖子的WP_Post
对象。
The global notice saved against a given post is retrieved by get_post_meta
and saved to $global_notice
variable.
通过给定帖子保存的全局通知由get_post_meta
检索并保存到$global_notice
变量中。
The notice is then wrapped in a div
and saved to the $notice
variable.
然后将该通知包装在div
并保存到$notice
变量中。
And finally, $notice
which holds the global notice is concatenated to $content
which is the actual post content.
最后, $notice
持有全球通知串接到$content
是实际的文章内容。
Below is a screenshot of a post with the global notice before the post content.
以下是帖子的屏幕截图,其中帖子内容之前有全局注意事项。
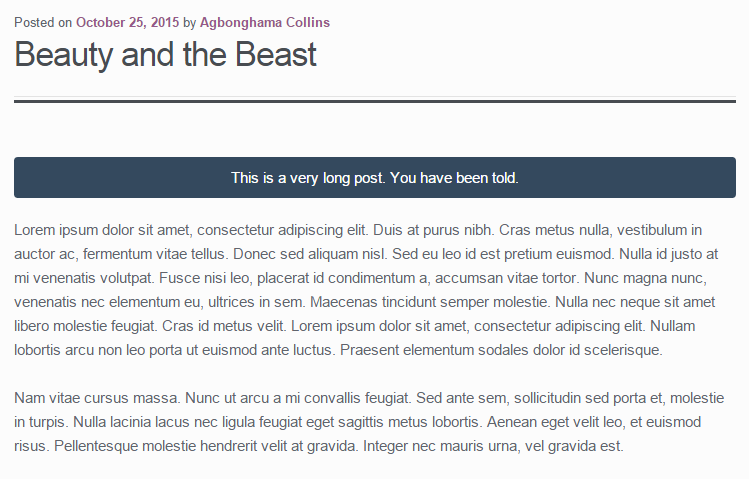
摘要 (Summary)
In this tutorial, we learned a number of ways to register meta boxes to WordPress administrative screens and how to restrict them to post types.
在本教程中,我们学习了多种将元框注册到WordPress管理屏幕的方法,以及如何将它们限制为发布类型。
We also reviewed how to add form fields to a meta box and how to save data entered into it when a post is saved or published.
我们还回顾了如何将表单字段添加到元框,以及如何保存或发布帖子时保存输入到其中的数据。
Finally, we covered how to put into practical use the data entered into a meta box.
最后,我们介绍了如何将输入到meta框中的数据投入实际使用。
In a future article we’ll cover how to add a contextual help tab to the post types administrative screens.
在以后的文章中,我们将介绍如何在帖子类型管理屏幕中添加上下文帮助选项卡。
If you have any questions or contributions, we’d love to hear them in the comments.
如果您有任何问题或贡献,我们很乐意在评论中听到。
翻译自: https://www.sitepoint.com/adding-meta-boxes-post-types-wordpress/
wordpress文本框