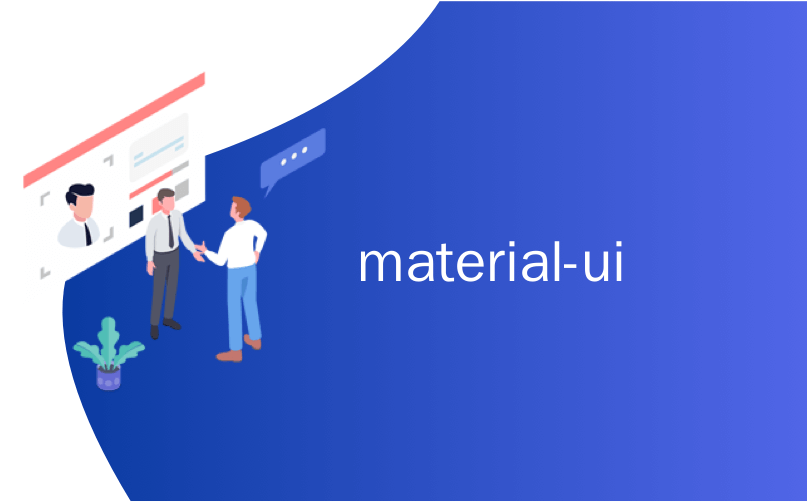
material-ui
这篇文章介绍了如何使用材料设计指南来创建天气应用。 材质设计是一组视觉设计,UI交互,动作等规则。 这些规则可帮助开发人员设计和创建Android应用。
这篇文章想描述我们如何使用Weatherlib作为天气层和Material设计规则来创建天气应用。 我们不仅要为原生支持Material Design的Android 5 Lollipop开发此应用,还希望支持4.x Kitkat之类的Android早期版本。 因此,我们将引入appCompat v7库,即使在先前的Android版本中,该库也可以帮助我们实现Material Design。
我们想编写一个具有扩展工具栏的应用程序,其中包含有关位置和当前天气的一些信息以及有关温度,天气图标,湿度,风和压力的一些基本天气信息。 最后,我们将得到如下图所示的图片:
Android项目设置
我们要做的第一件事是配置项目,以便我们可以使用Weatherlib,尤其是appCompat v7。 我们可以打开build.graddle并添加以下行:
dependencies {
compile fileTree(dir: 'libs', include: ['*.jar'])
compile 'com.android.support:appcompat-v7:21.0.+'
compile 'com.mcxiaoke.volley:library:1.0.6@aar'
compile 'com.survivingwithandroid:weatherlib:1.5.3'
compile 'com.survivingwithandroid:weatherlib_volleyclient:1.5.3'
}
现在我们已经正确设置了项目,我们可以开始定义应用布局。
应用布局:材质设计
如前所述,我们想在扩展工具栏上使用工具栏。 工具栏是操作栏的综合,可以给我们更多的控制权。 与紧密绑定到Actvitiy的操作栏不同,工具栏可以放置在View层次结构内的任何位置。
因此,我们的布局将分为三个主要区域:
- 工具栏区域
- 天气图标和温度
- 其他天气数据
布局如下图所示:
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".WeatherActivity"
android:orientation="vertical">
<android.support.v7.widget.Toolbar
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/my_toolbar"
android:layout_height="128dp"
app:popupTheme="@style/ActionBarPopupThemeOverlay"
android:layout_width="match_parent"
android:background="?attr/colorPrimary"
android:paddingLeft="72dp"
android:paddingBottom="16dp"
android:gravity="bottom"
app:titleTextAppearance="@style/Toolbartitle"
app:subtitleTextAppearance="@style/ToolbarSubtitle"
app:theme="@style/ThemeOverlay.AppCompat.Light"
android:title="@string/location_placeholder"
/>
....
</RelativeLayout>
如您所见,我们使用了工具栏。 如指南中所述,我们将工具栏的高度设置为等于128dp,此外,我们使用原色作为背景。 原色在colors.xml中定义。 您可以参考材料设计颜色准则以获取更多信息。 我们应该定义至少三种不同的颜色:
- 原色,由500标识
- 700标识的主要深色
- 应当是主要操作按钮等的强调颜色
工具栏的背景色设置为原色。
<resources>
<color name="primaryColor_500">#03a9f4</color>
<color name="primaryDarkColor_700">#0288d1</color>
....
</resources>
此外,工具栏内的左侧填充和底部填充是根据准则定义的。 最后,我们添加菜单项,就像使用操作栏一样。 主要结果如下所示:
如您所见,工具栏的背景等于原色。
搜索城市:带有材料设计的弹出窗口
我们可以使用弹出窗口让用户输入位置。 弹出窗口非常简单,它由一个用于输入数据的EditText和一个简单的ListView组成,该ListView显示与插入EditText中的模式匹配的城市。 我不会介绍如何在weatherlib中搜索城市,因为我已经介绍了它。 结果显示在这里:
弹出布局如下所示:
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical" android:layout_width="match_parent"
android:layout_height="match_parent"
>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="@string/dialog.city.header"
style="@style/Theme.AppCompat.Dialog"/>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="8sp"
android:text="@string/dialog.city.pattern"/>
<EditText
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
android:id="@+id/ptnEdit"/>
<ListView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/cityList"
android:clickable="true"/>
</LinearLayout>
创建和处理对话框的代码如下所示:
private Dialog createDialog() {
AlertDialog.Builder builder = new AlertDialog.Builder(this);
LayoutInflater inflater = this.getLayoutInflater();
View v = inflater.inflate(R.layout.select_city_dialog, null);
builder.setView(v);
EditText et = (EditText) v.findViewById(R.id.ptnEdit);
....
et.addTextChangedListener(new TextWatcher() {
....
@Override
public void onTextChanged(CharSequence s, int start, int before, int count) {
if (count > 3) {
// We start searching
weatherclient.searchCity(s.toString(), new WeatherClient.CityEventListener() {
@Override
public void onCityListRetrieved(List<City> cities) {
CityAdapter ca = new CityAdapter(WeatherActivity.this, cities);
cityListView.setAdapter(ca);
}
});
}
}
});
builder.setPositiveButton("Accept", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
dialog.dismiss();
// We update toolbar
toolbar.setTitle(currentCity.getName() + "," + currentCity.getCountry());
// Start getting weather
getWeather();
}
});
builder.setNegativeButton("Cancel", new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
dialog.dismiss();
}
});
return builder.create();
}
需要注意的重要一点是在第33行,根据用户选择的城市设置工具栏标题,然后获取当前天气。 这段代码的结果如下所示:
天气库:天气
要获取当前天气,我们使用Weatherlib:
private void getWeather() {
weatherclient.getCurrentCondition(new WeatherRequest(currentCity.getId()),
new WeatherClient.WeatherEventListener() {
@Override
public void onWeatherRetrieved(CurrentWeather currentWeather) {
// We have the current weather now
// Update subtitle toolbar
toolbar.setSubtitle(currentWeather.weather.currentCondition.getDescr());
tempView.setText(String.format("%.0f",currentWeather.weather.temperature.getTemp()));
pressView.setText(String.valueOf(currentWeather.weather.currentCondition.getPressure()));
windView.setText(String.valueOf(currentWeather.weather.wind.getSpeed()));
humView.setText(String.valueOf(currentWeather.weather.currentCondition.getHumidity()));
weatherIcon.setImageResource(WeatherIconMapper.getWeatherResource(currentWeather.weather.currentCondition.getIcon(), currentWeather.weather.currentCondition.getWeatherId()));
setToolbarColor(currentWeather.weather.temperature.getTemp());
}
....
}
您会注意到,在第8行,我们根据当前天气设置工具栏字幕,而在第15行,我们根据当前温度更改工具栏颜色。 作为工具栏的背景色,我们使用了准则中显示的原色。
- 源代码可从@github获得。
翻译自: https://www.javacodegeeks.com/2014/11/develop-android-weather-app-with-material-design.html
material-ui