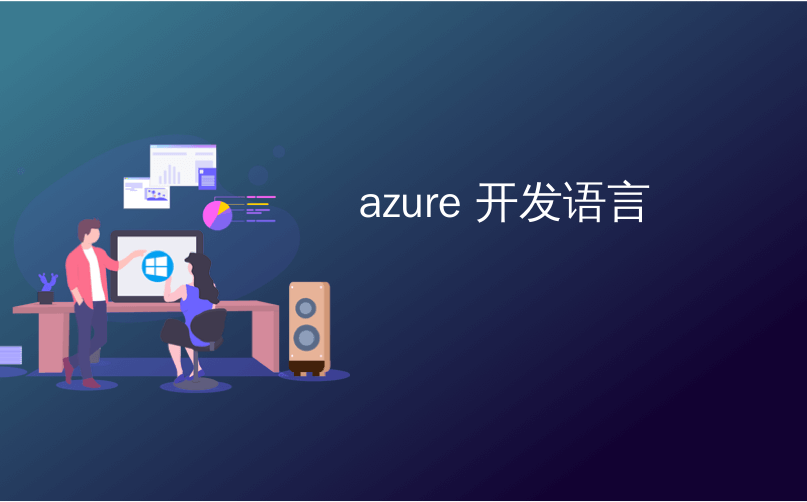
azure 开发语言
I was hanging out with Miguel de Icaza in New York a few weeks ago and he was sharing with me his ongoing love affair with a NoSQL Database called Azure DocumentDB. I've looked at it a few times over the last year or so and though it was cool but I didn't feel like using it for a few reasons:
几周前,我在纽约与Miguel de Icaza闲逛,他与我分享了他与NoSQL数据库Azure Azure的恋爱关系。 在过去的一年左右的时间里,我已经看过几次了,虽然它很酷,但是由于某些原因,我不喜欢使用它:
- Can't develop locally - I'm often in low-bandwidth or airplane situations 无法在本地开发-我经常在低带宽或飞机情况下
- No MongoDB support - I have existing apps written in Node that use Mongo 不支持MongoDB-我已经在Node中编写了使用Mongo的应用程序
- No .NET Core support - I'm doing mostly cross-platform .NET Core apps没有.NET Core支持-我主要在做跨平台的.NET Core应用程序
Miguel told me to take a closer look. Looks like things have changed! DocumentDB now has:
Miguel告诉我仔细看看。 看起来情况已经变了! DocumentDB现在具有:
Free local DocumentDB Emulator - I asked and this is the SAME code that runs in Azure with just changes like using the local file system for persistence, etc. It's an "emulator" but it's really the essential same core engine code. There is no cost and no sign in for the local DocumentDB emulator.
免费的本地DocumentDB模拟器-我问过,这是在Azure中运行的SAME代码,只是进行了一些更改,例如使用本地文件系统进行持久性等。它是一个“模拟器”,但实际上是必不可少的相同核心引擎代码。 本地DocumentDB仿真器没有费用,也无需登录。
-
MongoDB protocol support - This is amazing. I literally took an existing Node app, downloaded MongoChef and copied my collection over into Azure using a standard MongoDB connection string, then pointed my app at DocumentDB and it just worked. It's using DocumentDB for storage though, which gives me MongoDB协议支持-太神奇了。 我实际上使用了一个现有的Node应用程序,下载了MongoChef,然后使用标准的MongoDB连接字符串将我的集合复制到Azure中,然后将我的应用程序指向DocumentDB,它就可以正常工作。 它使用DocumentDB进行存储,这给了我
-
Better Latency更好的延迟
-
Turnkey global geo-replication (like literally a few clicks)交钥匙全局地理复制(如单击几下一样)
-
A performance SLA with <10ms read and <15ms write (Service Level Agreement)读取时间<10ms和写入时间<15ms的性能SLA(服务水平协议)
-
Metrics and Resource Management like every Azure Service像每个Azure服务一样的指标和资源管理
MongoDB protocol support - This is amazing. I literally took an existing Node app, downloaded MongoChef and copied my collection over into Azure using a standard MongoDB connection string, then pointed my app at DocumentDB and it just worked. It's using DocumentDB for storage though, which gives me MongoDB协议支持-太神奇了。 我实际上使用了一个现有的Node应用程序,下载了MongoChef,然后使用标准的MongoDB连接字符串将我的集合复制到Azure中,然后将我的应用程序指向DocumentDB,它就可以正常工作。 它使用DocumentDB进行存储,这给了我 -
DocumentDB .NET Core Preview SDK that has feature parity with the .NET Framework SDK.
具有与.NET Framework SDK相同功能的DocumentDB .NET Core Preview SDK。
There's also Node, .NET, Python, Java, and C++ SDKs for DocumentDB so it's nice for gaming on Unity, Web Apps, or any .NET App...including Xamarin mobile apps on iOS and Android which is why Miguel is so hype on it.
还有用于DocumentDB的Node,.NET,Python,Java和C ++ SDK,因此非常适合在Unity,Web Apps或任何.NET App上进行游戏...包括iOS和Android上的Xamarin移动应用程序,这就是Miguel如此大肆宣传的原因在上面。
Azure DocumentDB本地快速入门 (Azure DocumentDB Local Quick Start)
I wanted to see how quickly I could get started. I spoke with the PM for the project on Azure Friday and downloaded and installed the local emulator. The lead on the project said it's Windows for now but they are looking for cross-platform solutions. After it was installed it popped up my web browser with a local web page - I wish more development tools would have such clean Quick Starts. There's also a nice quick start on using DocumentDB with ASP.NET MVC.
我想看看我能多快开始。 我在Azure星期五与该项目的项目经理进行了交谈,并下载并安装了本地模拟器。 该项目的负责人说,现在是Windows,但是他们正在寻找跨平台的解决方案。 安装后,它会弹出带有本地网页的Web浏览器-我希望更多的开发工具具有如此简洁的“快速入门”。 将DocumentDB与ASP.NET MVC结合使用也有一个不错的快速入门。
NOTE: This is a 0.1.0 release. Definitely Alpha level. For example, the sample included looks like it had the package name changed at some point so it didn't line up. I had to change "Microsoft.Azure.Documents.Client": "0.1.0" to "Microsoft.Azure.DocumentDB.Core": "0.1.0-preview" so a little attention to detail issue there. I believe the intent is for stuff to Just Work. ;)
注意:这是一个0.1.0版本。 绝对是Alpha级。 例如,所包含的示例看起来在某个时候更改了软件包名称,因此没有对齐。 我不得不将“ Microsoft.Azure.Documents.Client”:“ 0.1.0”更改为“ Microsoft.Azure.DocumentDB.Core”:“ 0.1.0-preview”,所以稍微注意那里的细节问题。 我相信目的是为了使工作正常。 ;)
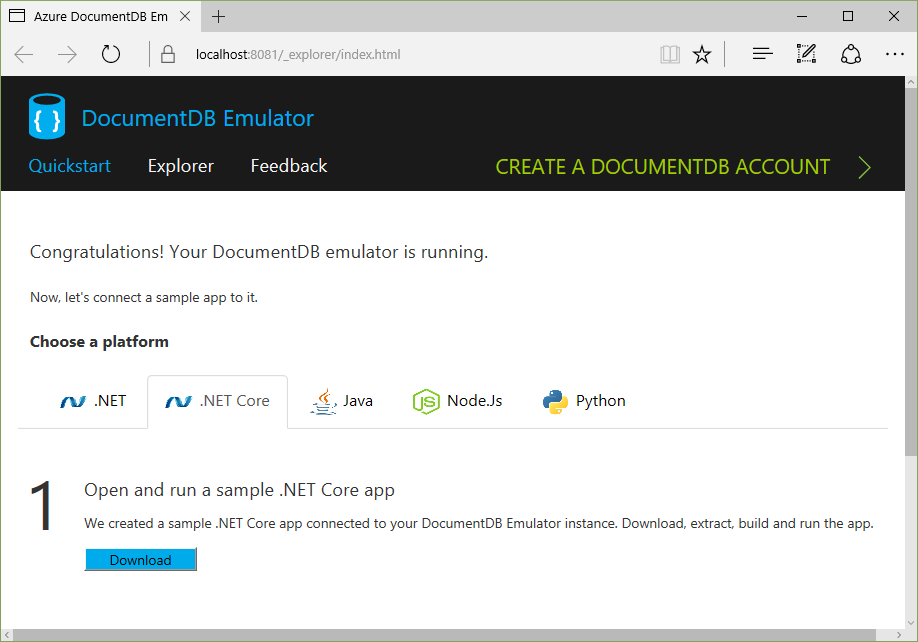
The sample app is a pretty standard "ToDo" app:
该示例应用程序是一个非常标准的“ ToDo”应用程序:
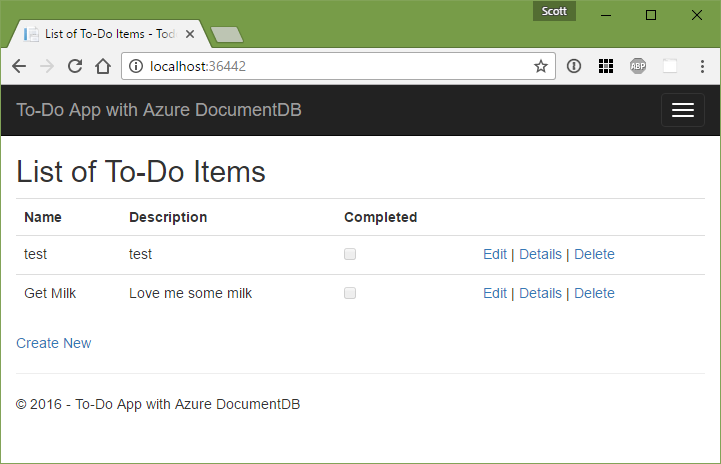
The local Emulator also includes a web-based local Data Explorer:
本地仿真器还包括一个基于Web的本地Data Explorer:
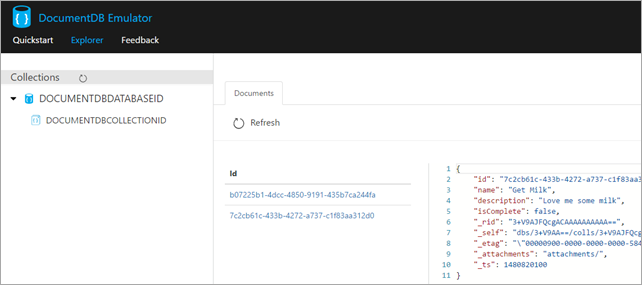
A Todo Item is really just a POCO (Plain Old CLR Object) like this:
待办事项实际上就是这样的POCO(普通旧CLR对象):
namespace todo.Models
{
using Newtonsoft.Json;
public class Item
{
[JsonProperty(PropertyName = "id")]
public string Id { get; set; }
[JsonProperty(PropertyName = "name")]
public string Name { get; set; }
[JsonProperty(PropertyName = "description")]
public string Description { get; set; }
[JsonProperty(PropertyName = "isComplete")]
public bool Completed { get; set; }
}
}
The MVC Controller in the sample uses an underlying repository pattern so the code is super simple at that layer - as an example:
样本中的MVC控制器使用基础存储库模式,因此该层的代码非常简单-例如:
[ActionName("Index")]
public async Task<IActionResult> Index()
{
var items = await DocumentDBRepository<Item>.GetItemsAsync(d => !d.Completed);
return View(items);
}
[HttpPost]
[ActionName("Create")]
[ValidateAntiForgeryToken]
public async Task<ActionResult> CreateAsync([Bind("Id,Name,Description,Completed")] Item item)
{
if (ModelState.IsValid)
{
await DocumentDBRepository<Item>.CreateItemAsync(item);
return RedirectToAction("Index");
}
return View(item);
}
The Repository itself that's abstracting away the complexities is itself not that complex. It's like 120 lines of code, and really more like 60 when you remove whitespace and curly braces. And half of that is just initialization and setup. It's also DocumentDBRepository<T> so it's a generic you can change to meet your tastes and use it however you'd like.
提取复杂性的资源库本身并不那么复杂。 这就像120行代码,而当删除空白和花括号时,实际上更像是60行。 其中一半只是初始化和设置。 它也是DocumentDBRepository <T>,因此它是通用名称,您可以更改它以满足自己的喜好,并根据需要使用它。
The only thing that stands out to me in this sample is the loop in GetItemsAsync that's hiding potential paging/chunking. It's nice you can pass in a predicate but I'll want to go and put in some paging logic for large collections.
在此示例中,对我唯一突出的是GetItemsAsync中的循环,该循环隐藏了潜在的分页/分块。 可以传入一个谓词很好,但是我想为大型集合输入一些分页逻辑。
public static async Task<T> GetItemAsync(string id)
{
try
{
Document document = await client.ReadDocumentAsync(UriFactory.CreateDocumentUri(DatabaseId, CollectionId, id));
return (T)(dynamic)document;
}
catch (DocumentClientException e)
{
if (e.StatusCode == System.Net.HttpStatusCode.NotFound){
return null;
}
else {
throw;
}
}
}
public static async Task<IEnumerable<T>> GetItemsAsync(Expression<Func<T, bool>> predicate)
{
IDocumentQuery<T> query = client.CreateDocumentQuery<T>(
UriFactory.CreateDocumentCollectionUri(DatabaseId, CollectionId),
new FeedOptions { MaxItemCount = -1 })
.Where(predicate)
.AsDocumentQuery();
List<T> results = new List<T>();
while (query.HasMoreResults){
results.AddRange(await query.ExecuteNextAsync<T>());
}
return results;
}
public static async Task<Document> CreateItemAsync(T item)
{
return await client.CreateDocumentAsync(UriFactory.CreateDocumentCollectionUri(DatabaseId, CollectionId), item);
}
public static async Task<Document> UpdateItemAsync(string id, T item)
{
return await client.ReplaceDocumentAsync(UriFactory.CreateDocumentUri(DatabaseId, CollectionId, id), item);
}
public static async Task DeleteItemAsync(string id)
{
await client.DeleteDocumentAsync(UriFactory.CreateDocumentUri(DatabaseId, CollectionId, id));
}
I'm going to keep playing with this but so far I'm pretty happy I can get this far while on an airplane. It's really easy (given I'm preferring NoSQL over SQL lately) to just through objects at it and store them.
我将继续玩这个游戏,但到目前为止,我很高兴我能在飞机上走得这么远。 确实很简单(鉴于我最近更喜欢NoSQL而不是SQL)只是遍历对象并存储它们。
In another post I'm going to look at RavenDB, another great NoSQL Document Database that works on .NET Core that s also Open Source.
在另一篇文章中,我将介绍RavenDB , RavenDB是另一个出色的NoSQL文档数据库,可在.NET Core上使用,该数据库也是开源的。
Sponsor: Big thanks to Octopus Deploy! Do you deploy the same application multiple times for each of your end customers? The team at Octopus have taken the pain out of multi-tenant deployments. Check out their latest 3.4 release
赞助商:非常感谢章鱼部署! 您是否为每个最终客户多次部署相同的应用程序? Octopus的团队已摆脱了多租户部署的痛苦。 查看他们最新的3.4版本
翻译自: https://www.hanselman.com/blog/nosql-net-core-development-using-an-local-azure-documentdb-emulator
azure 开发语言