I've been orbiting around F# - but not really jumping in - for a while now. In fact, I looked at F# in 2008 almost 6 years ago and more recently talked to Richard Minerich and Phillip Trelford on Hanselminutes Podcast #311 "Why F#?"
我一直在绕F#轨道飞行-但现在还没有真正跳进去-已经有一段时间了。 实际上,我在大约6年前的2008年看过F#,最近在Hanselminutes Podcast#311 “为什么使用F#?”上与Richard Minerich和Phillip Trelford进行了交谈。
Last week I looked at using ScriptCS, literally C# as a Scripting language, to drive browser automation. Today I'm exploring a make system called FAKE. It's F# Make, a build automation system similar to Make (which is 38 years old next month!) or Rake (which uses Ruby).
上周,我研究了使用ScriptCS (实际上是C#作为脚本语言)来驱动浏览器自动化。 今天,我正在探索一个名为FAKE的make系统。 它是F#Make ,它是一个类似于Make(下个月已经38岁!)或Rake(使用Ruby)的构建自动化系统。
Fake is a Domain Specific Language that you can use without knowing F#, but if and when you outgrow it you can keep heading down the F# road. In all cases you've got all of .NET at your command.
Fake是一种特定于域的语言,您可以在不了解F#的情况下使用它,但是如果您不再使用它,则可以继续前进。 在所有情况下,您都可以使用所有.NET。
Here's their Hello World example, a basic deploy script:
这是他们的Hello World示例,一个基本的部署脚本:
#r "tools/FAKE/tools/FakeLib.dll" // include Fake lib
open Fake
Target "Test" (fun _ ->
trace "Testing stuff..."
)
Target "Deploy" (fun _ ->
trace "Deploy stuff..."
)
"Test" // define the dependencies
==> "Deploy"
Run "Deploy"
Note that Deploy depends on Test.
请注意,部署取决于测试。
FAKE uses F# but you can use it go build whatever. These are some C# OSS projects that use FAKE to build themselves:
FAKE使用F#,但是您可以使用它来构建任何东西。 以下是一些使用FAKE自己构建的C#OSS项目:
Octokit.NET by GitHub
GitHub的Octokit .NET
NSubstitute (https://github.com/nsubstitute/NSubstitute/pull/138 WIP)
NSubstitute( https://github.com/nsubstitute/NSubstitute/pull/138 WIP)
FAKE isn't new, it's actually been around for 4 years with 55 contributors so far! It works not only on .NET but also on Linux and Mac under Mono - it works everywhere. Because it's all .NET you can use it on your Continuous Integration Servers like TeamCity or Travis CI.
FAKE并不是什么新鲜事物,到目前为止,它已经有55位贡献者了4年了! 它不仅可以在.NET上运行,还可以在Mono下Linux和Mac上运行-随处都可以使用。 因为都是.NET,所以您可以在诸如TeamCity或Travis CI之类的持续集成服务器上使用它。
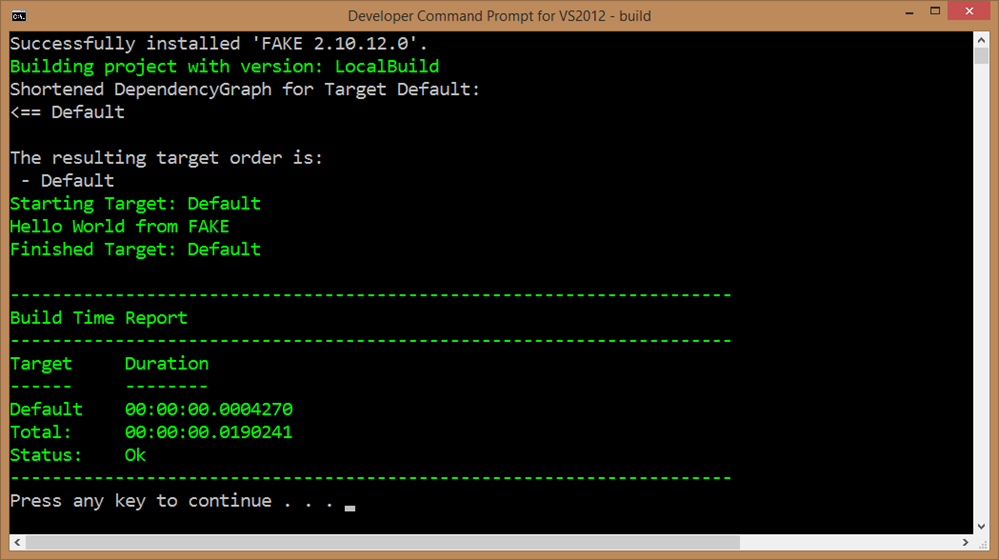
FAKE入门 (Getting Started with FAKE)
Check out their Calculator sample, a .NET project you'll extend to build itself with FAKE. Just download the zip, unblock it, and unzip. Then run build.bat from a developer command prompt.
查看他们的Calculator示例,这是一个.NET项目,您将对其进行扩展以使用FAKE构建自身。 只需下载zip文件,然后将其解锁并解压缩。 然后从开发人员命令提示符运行build.bat。
The build.net is a bootstrapper. It could be powershell or a shell script if you like, of course.
build.net是一个引导程序。 当然,它可以是powershell或shell脚本。
@echo off
cls
"tools\nuget\nuget.exe" "install" "FAKE" "-OutputDirectory" "tools" "-ExcludeVersion"
"tools\FAKE\tools\Fake.exe" build.fsx
pause
This batch file uses NuGet to get FAKE, just as npm install restores node_modules, or gem gets ruby libraries. Then it calls Fake on the build.fsx file. Follow their Getting Started instructions to slowly expand the responsibilities of the build.fsx file.
这个批处理文件使用NuGet来获取FAKE,就像npm install恢复node_modules一样,或者gem获取ruby库一样。 然后,在build.fsx文件上调用Fake。 按照他们的入门指南逐步扩展build.fsx文件的职责。
FAKE has a lot of Helpers in their API documentation. Hundreds, and there's a whole community making others that you can call upon. For example, the built in FileHelper has things like CleanDir to remove files and subdirs.
FAKE在其API文档中有很多帮助程序。 数百个社区组成了您可以呼吁的其他社区。 例如,内置的FileHelper具有CleanDir之类的功能,可以删除文件和子目录。
Here we Clean before we build by making Clean a dependency of Default. BuildDir here is a property that's shared.
在这里,我们通过将Clean设置为Default的依赖项来进行清理。 BuildDir是共享的属性。
// include Fake lib
#r "tools/FAKE/tools/FakeLib.dll"
open Fake
// Properties
let buildDir = "./build/"
// Targets
Target "Clean" (fun _ ->
CleanDir buildDir
)
Target "Default" (fun _ ->
trace "Hello World from FAKE"
)
// Dependencies
"Clean"
==> "Default"
// start build
RunTargetOrDefault "Default"
Jumping to the end of the tutorial, the syntax gets a little more tricky, butu once you get the |> format, it makes sense.
跳到本教程的结尾,语法变得有些棘手,但是一旦获得|>格式,它就会变得有意义。
Some cool things to note and file away as interesting at in the script below.
在下面的脚本中,一些很有趣的事情要注意并归档,以免引起兴趣。
- the use of !! to include files 指某东西的用途 !! 包含文件
- the use of -- to exclude a file spec after a !! operator 使用-排除!!之后的文件规范算子
- Zip is built-in as a helper and zips up the results of the build. Zip是作为帮助程序内置的,并压缩构建结果。
- the options passed into NUnit 传递给NUnit的选项
- The dependency chain 依赖链
- #r for referencing .NET DLLs #r用于引用.NET DLL
// include Fake lib
#r "tools/FAKE/tools/FakeLib.dll"
open Fake
RestorePackages()
// Properties
let buildDir = "./build/"
let testDir = "./test/"
let deployDir = "./deploy/"
// version info
let version = "0.2" // or retrieve from CI server
// Targets
Target "Clean" (fun _ ->
CleanDirs [buildDir; testDir; deployDir]
)
Target "BuildApp" (fun _ ->
!! "src/app/**/*.csproj"
|> MSBuildRelease buildDir "Build"
|> Log "AppBuild-Output: "
)
Target "BuildTest" (fun _ ->
!! "src/test/**/*.csproj"
|> MSBuildDebug testDir "Build"
|> Log "TestBuild-Output: "
)
Target "Test" (fun _ ->
!! (testDir + "/NUnit.Test.*.dll")
|> NUnit (fun p ->
{p with
DisableShadowCopy = true;
OutputFile = testDir + "TestResults.xml" })
)
Target "Zip" (fun _ ->
!! (buildDir + "/**/*.*")
-- "*.zip"
|> Zip buildDir (deployDir + "Calculator." + version + ".zip")
)
Target "Default" (fun _ ->
trace "Hello World from FAKE"
)
// Dependencies
"Clean"
==> "BuildApp"
==> "BuildTest"
==> "Test"
==> "Zip"
==> "Default"
// start build
RunTargetOrDefault "Default"
You can do virtually anything and there's a great community out there to help.
您几乎可以做任何事情,这里有一个很棒的社区可以提供帮助。
Here's a more complex dependency chain with an optional parameter:
这是带有可选参数的更复杂的依赖项链:
// Build order
"Clean"
==> "BuildApp"
==> "BuildTest"
==> "FxCop"
==> "NUnitTest"
=?> ("xUnitTest",hasBuildParam "xUnitTest") // runs the target only if FAKE was called with parameter xUnitTest
==> "Deploy"
There's a rich FAKE world out there with support for Octopus Deploy, all Unit Test systems, Xamarin's xpkg format and much more. Thanks to Steffan Forkmann for helping me explore this. ;)
支持章鱼部署,所有单元测试系统,Xamarin的xpkg格式以及更多其他功能,有一个丰富的FAKE世界。 感谢Steffan Forkmann帮助我探索这一点。 ;)
相关链接 (Related Links)
翻译自: https://www.hanselman.com/blog/exploring-fake-an-f-build-system-for-all-of-net