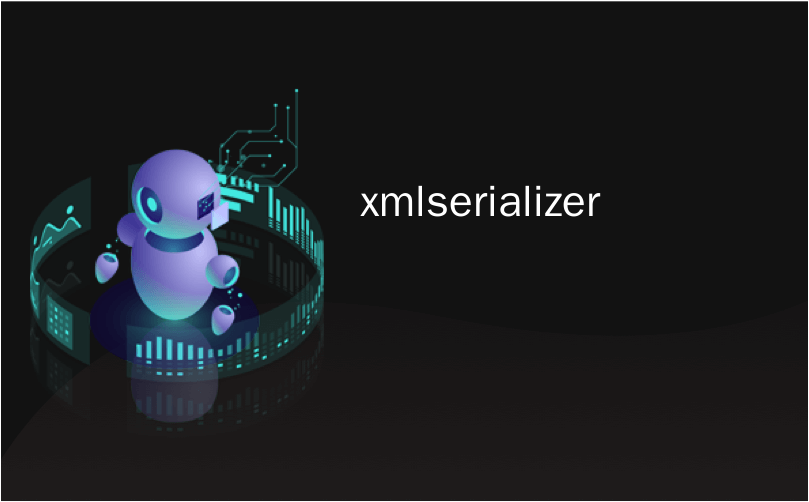
xmlserializer
Given a class like this:
给定这样的课程:
public class Student
{
public int SSN = 555555555;
public decimal GPA = 0.1;
public bool cool = false;
public DateTime birthday = DateTime.Now;
public long Height = 80;
}
The XmlSerializer will output XML like this:
XmlSerializer将输出XML,如下所示:
<Student>
<SSN>555555555</SSN>
<GPA>0.1</GPA>
<cool>false</cool>
<birthday>2005-09-01T13:25:41.4964384-07:00</birthday>
<Height>60</Height>
</Student>
<学生> <SSN> 555555555 </ SSN> <GPA> 0.1 </ GPA> <cool>假</ cool> <生日> 2005-09-01T13:25:41.4964384-07:00 </ birthday> <Height> 60 </ Height> </学生>
As you'd expect. Note the standard 8601 DateTime and the word "false" for the boolean element "cool."
如您所料。 注意标准的8601 DateTime和布尔元素“ cool”的单词“ false”。
However, what if you want to output "bar" for false and "foo" for true? Here's one way that we've built into some code generation templates. The trick is that the actual boolean that the developer accesses is marked as XmlIgnore, and a parallel string value is marked with and [XmlElement] attribute that changes the name of the serialized element.
但是,如果要输出false的“ bar”和输出true的“ foo”怎么办? 这是我们内置到某些代码生成模板中的一种方法。 技巧是将开发人员访问的实际布尔值标记为XmlIgnore,并使用和[XmlElement]属性标记一个并行字符串值,该属性会更改序列化元素的名称。
1 using System;
2 using System.Xml;
3 using System.Collections;
4 using System.Xml.Serialization;
5 using System.IO;
6
7 public class SerTest
8 {
9 public static void Main(string[] args)
10 {
11 Student[] students = new Student[2];
12
13 students[0] = new Student();
14 students[0].SSN = 555555555;
15 students[0].GPA = 0.1M;
16 students[0].Height = 60;
17 students[0].cool = false;
18
19 students[1] = new Student();
20 students[1].SSN = 555554444;
21 students[1].GPA = 3.1M;
22 students[1].Height = 160;
23 students[1].cool = true;
24
25
26 XmlSerializer mySerializer = new
27 XmlSerializer(typeof(Student[] ));
28
29 StreamWriter myWriter = new StreamWriter("Students.xml");
30 mySerializer.Serialize(myWriter, students);
31
32 myWriter.Close();
33 StreamReader myReader = new StreamReader("Students.xml");
34 Student[] somefolks = (Student[])mySerializer.Deserialize(myReader);
35 }
36 }
37
38 public class Student
39 {
40 public int SSN;
41
42 public decimal GPA;
43
44 //It is assumed that the values "FOO" and "BAR" are put here by
45 // a code generator, so it's not that big of a deal that they are
46 // duplicated in this code. Just trying to cover all bases.
47 [XmlIgnore]
48 public bool cool
49 {
50 get
51 {
52 if (coolStringValue == null || coolStringValue.Length == 0) return false;
53
54 if (CaseInsensitiveComparer.DefaultInvariant.Compare(coolStringValue,"FOO") == 0)
55 {
56 return true;
57 }
58 else if (CaseInsensitiveComparer.DefaultInvariant.Compare(coolStringValue,"BAR") == 0)
59 {
60 return false;
61 }
62 throw new ApplicationException(coolStringValue + "
is neither FOO (true) nor BAR (false). This is an invalid state for this boolean.");
63 }
64 set
65 {
66 coolStringValue = (value ? "FOO" : "BAR" );
67 }
68 }
69 [XmlElementAttribute(ElementName="cool",DataType="string")]
70 public string coolStringValue = "bar";
71
72 public DateTime birthday = DateTime.Now;
73
74 public long Height = 80;
75 }
This code listing outputs this XML:
此代码清单输出此XML:
<?xml version="1.0" encoding="utf-8"?>
<ArrayOfStudent xmlns:xsd="http://www.w3.org/2001/XMLSchema"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance">
<Student>
<SSN>555555555</SSN>
<GPA>0.1</GPA>
<cool>BAR</cool>
<birthday>2005-09-01T13:35:31.0041088-07:00</birthday>
<Height>60</Height>
</Student>
<Student>
<SSN>555554444</SSN>
<GPA>3.1</GPA>
<cool>FOO</cool>
<birthday>2005-09-01T13:35:31.0041088-07:00</birthday>
<Height>160</Height>
</Student>
</ArrayOfStudent>
<?xml版本=“ 1.0”编码=“ utf-8”?> <ArrayOfStudent xmlns:xsd =“ http://www.w3.org/2001/XMLSchema ” xmlns:xsi =“ http://www.w3.org/2001/XMLSchema-instance ”> <学生> <SSN> 555555555 </ SSN> <GPA> 0.1 </ GPA> <cool> BAR </ cool> <生日> 2005-09-01T13:35:31.0041088-07:00 </ birthday> <Height> 60 </ Height> </学生> <学生> <SSN> 555554444 </ SSN> <GPA> 3.1 </ GPA> <cool> FOO </ cool> <生日> 2005-09-01T13:35:31.0041088-07:00 </ birthday> <Height> 160 </ Height> </学生> </ ArrayOfStudent>
翻译自: https://www.hanselman.com/blog/making-the-xmlserializer-output-alternate-values-for-simple-types
xmlserializer