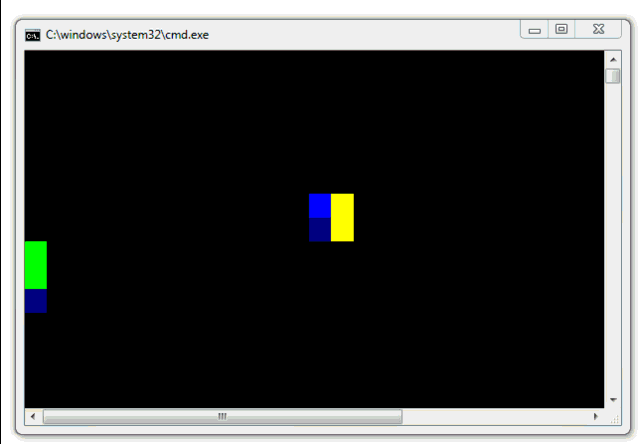
In August I purchased and reviewed the Microsoft Touch Mouse. I still use my Microsoft Arc Mouse more than the touch, initially due to what I felt was dodgy scrolling performance on the Touch Mouse, as I mentioned in my review. Still, I've kept it in my backpack and I use the Touch Mouse perhaps a few times a month and have kept the software up to date in case there's some software changes made to improve performance.
八月份,我购买并审查了Microsoft Touch Mouse 。 我仍然在使用Microsoft Arc Mouse而不是使用触摸,最初是因为我认为在Touch Mouse上滚动功能不稳定,正如我在评论中提到的那样。 尽管如此,我仍将其放在背包中,并且每月可能会使用几次Touch Mouse,并保持软件为最新版本,以防为了改进性能而进行一些软件更改。
I can happily say that they've changed something and the scrolling performance is WAY better. I can finally get 1 to 2 pixel precision with it while scrolling in my browsers. The other nice feature is the "three finger swipe up" which gives you effectively a Windows version of the Mac Expose window switcher view.
我可以高兴地说,他们做了一些更改,滚动性能更好。 在浏览器中滚动时,最终可以达到1到2像素的精度。 另一个不错的功能是“三指向上滑动”,可以有效地为您提供Windows版本的Mac Expose窗口切换器视图。
Today I noticed while catching up on Long Zheng's excellent blog that the Touch Mouse Sensor SDK is available for download. Per their site:
今天,当我在Long Zheng的精彩博客中发消息时,注意到可以下载Touch Mouse Sensor SDK。 根据他们的站点:
"The Microsoft Touch Mouse supports multitouch gestures via an integrated sensor. The Touch Mouse Sensor API SDK is a small library intended to enable students and researchers to experiment directly with Touch Mouse sensor output.
“ Microsoft触摸鼠标通过集成的传感器支持多点触摸手势。触摸鼠标传感器API SDK是一个小型库,旨在使学生和研究人员可以直接使用触摸鼠标传感器输出进行实验。
Using this SDK, you can create your own applications consuming the 13×15 sensor image. The SDK includes C# and C++ samples demonstrating how to read and manipulate sensor data"
使用此SDK,您可以使用13×15传感器图像创建自己的应用程序。 该SDK包含C#和C ++示例,演示了如何读取和操作传感器数据”
OK, so what can we do with this thing? It comes with a number of samples to get the bitmap from the sensor and process it. It includes examples in both C# and C++. Because I don't have C++ on this machine I got an error opening that solution, but you can just remove that project and still build the samples.
好,那我们该怎么办? 它带有许多样本,可以从传感器获取位图并进行处理。 它包括C#和C ++中的示例。 因为我的计算机上没有C ++,所以打开该解决方案时出现错误,但是您可以删除该项目并仍然构建示例。
You don't need to do your work in a graphical application, although it's nice to visualize this data of course. First sample is a console app, that tells you were the center of mass of your big fat finger is.
尽管可视化这些数据当然很不错,但是您无需在图形应用程序中进行工作。 第一个示例是一个控制台应用程序,它告诉您您的大手指的重心在哪里。
The smarts are in the Microsoft.Research.TouchMouseSensor namespace. You implement a callback function that the mouse effectively calls whenever something interesting happens. It looks like this:
这些智能控件位于Microsoft.Research.TouchMouseSensor命名空间中。 您实现了一个回调函数,只要发生有趣的事情,鼠标就会有效地调用它。 看起来像这样:
/// <summary>
/// Function receiving callback from mouse.
/// </summary>
/// <param name="pTouchMouseStatus">Values indicating status of mouse.</param>
/// <param name="pabImage">Bytes forming image, 13 rows of 15 columns.</param>
/// <param name="dwImageSize">Size of image, assumed to always be 195 (13x15).</param>
static void TouchMouseCallbackFunction(ref TOUCHMOUSESTATUS pTouchMouseStatus,
byte[] pabImage,
int dwImageSize)
{
...
}
As you can see, the image size is 13x15, always. You get the raw bytes that represent the image, 195 bytes long.
如您所见,图像大小始终为13x15。 您得到代表图像的原始字节,长195个字节。
The first thing I was surprised to see was just HOW MUCH of the surface area of the mouse is covered and how high the resolution is. I was thinking, "13x15? That's so small, how could it tell me anything useful with such a limited structure?" Well, that's how clueless I was.
我惊讶地发现的第一件事就是鼠标的表面积有多大以及分辨率有多高。 我当时在想:“ 13x15?太小了,它怎么能告诉我在如此有限的结构下有用的东西?” 好吧,那就是我的无知。
Of course, each of these bytes is giving a number between 0 and 255 so a lot of information can be extrapolated between two pixels with different values and you can get a pretty detailed picture of what's going on. How much? Well, before I move on to a graphical example, why not abuse ASCII as much as we can?
当然,这些字节中的每个字节都给出一个介于0到255之间的数字,因此可以在两个具有不同值的像素之间推断出很多信息,并且可以详细了解正在发生的事情。 多少? 好吧,在继续介绍图形示例之前,为什么不尽可能多地滥用ASCII?
First, I'll output just the hex values with this code, then show what it looks like when I move my figures around the surface of the mouse.
首先,我将使用此代码仅输出十六进制值,然后显示将图形围绕鼠标表面移动时的外观。
Console.SetWindowSize(50,16);
Console.SetCursorPosition(0, 0);
StringBuilder sb = new StringBuilder(300);
for (Int32 y = 0; y < pTouchMouseStatus.m_dwImageHeight; y++)
{
// Iterate over columns.
for (Int32 x = 0; x < pTouchMouseStatus.m_dwImageWidth; x++)
{
// Get the pixel value at current position.
int pixel = pabImage[pTouchMouseStatus.m_dwImageWidth * y + x];
if (pixel > 0)
sb.AppendFormat("#{0:X2}", pixel);
else
sb.Append(" ");
}
sb.Append("\n");
}
Console.Write(sb.ToString());
Here's the result, animated.
这是动画的结果。
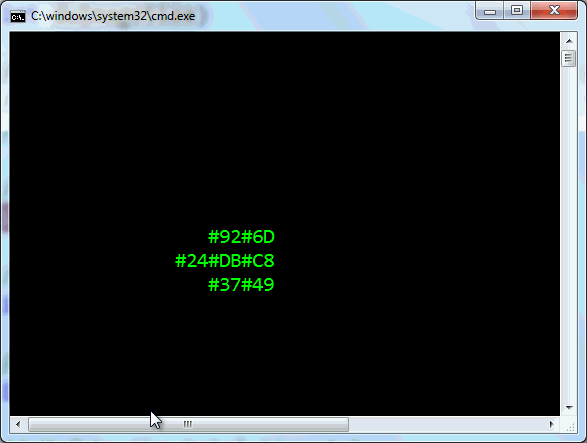
Cool. But what about with color? I could use the default Console stuff and set the colors, or I could get direct access to the Console, use the Win32 APIs and what not, but I could also use Tim Sneath's ConsoleEx class that's on NuGet thanks to Anthony Mastrean! First, I'll "install-package ConsoleEx" to bring it in.
凉。 但是颜色呢? 我可以使用默认的Console东西并设置颜色,或者我可以直接访问Console,使用Win32 API,但不能,但是我也可以使用Tim Sneath在NuGet上的ConsoleEx类,这要归功于Anthony Mastrean ! 首先,我将“安装软件包ConsoleEx ”将其引入。
How about a mouse touch heat-map with color? I thought about building a whole "find closest color" map and trying to map 255 different colors, but then I just did this.
带有颜色的鼠标触摸热图怎么样? 我考虑过要构建一个完整的“查找最接近的颜色”贴图,并尝试绘制255种不同的颜色,但是后来我做到了。
if (pixel > 230)
Console.BackgroundColor = ConsoleColor.Red;
else if (pixel > 128)
Console.BackgroundColor = ConsoleColor.Yellow;
else if (pixel > 90)
Console.BackgroundColor = ConsoleColor.Green;
else if (pixel > 45)
Console.BackgroundColor = ConsoleColor.Blue;
else if(pixel > 15)
Console.BackgroundColor = ConsoleColor.DarkBlue;
else
Console.BackgroundColor = ConsoleColor.Black;
ConsoleEx.WriteAt(x*2, y, " ");
And the result is this:
结果是这样的:
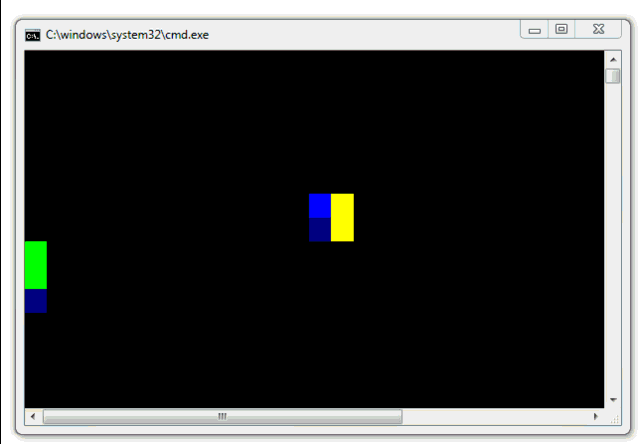
You can do the same thing in WPF, of course, with better bitmap support, but seriously, with a Console-based heatmap, who needs graphics? ;)
当然,在WPF中,您可以通过更好的位图支持来执行相同的操作,但实际上,使用基于控制台的热图,谁需要图形? ;)
OK, fine, here's the sample code that takes the results of the TouchMouseSensorEventArgs and creates a grayscale image.
好的,这是示例代码,该示例代码获取TouchMouseSensorEventArgs的结果并创建灰度图像。
void TouchMouseSensorHandler(object sender, TouchMouseSensorEventArgs e)
{
// We're in a thread belonging to the mouse, not the user interface
// thread. Need to dispatch to the user interface thread.
Dispatcher.Invoke((Action<TouchMouseSensorEventArgs>)SetSource, e);
}
void SetSource(TouchMouseSensorEventArgs e)
{
// Convert bitmap from memory to graphic form.
BitmapSource source =
BitmapSource.Create(e.Status.m_dwImageWidth, e.Status.m_dwImageHeight,
105, 96,
PixelFormats.Gray8, null, e.Image, e.Status.m_dwImageWidth);
// Show bitmap in user interface.
SensorImage.Source = source;
}
And the result:
结果:
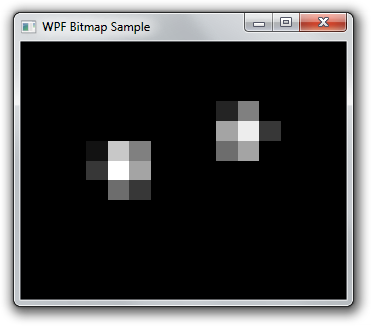
One way to look at this is as if the surface of the mouse is a tiny Xbox Kinect. What kinds of gestures could I recognize and hook up to do stuff? Control WIndows, do custom stuff inside my application, browser, or whatever. Any ideas?
一种查看方式是,鼠标的表面好像是一个很小的Xbox Kinect。 我可以识别出哪些手势并进行操作? 控制窗口,在我的应用程序,浏览器或其他任何东西中执行自定义操作。 有任何想法吗?
The downloads are here:
下载在这里:
Enjoy!
请享用!