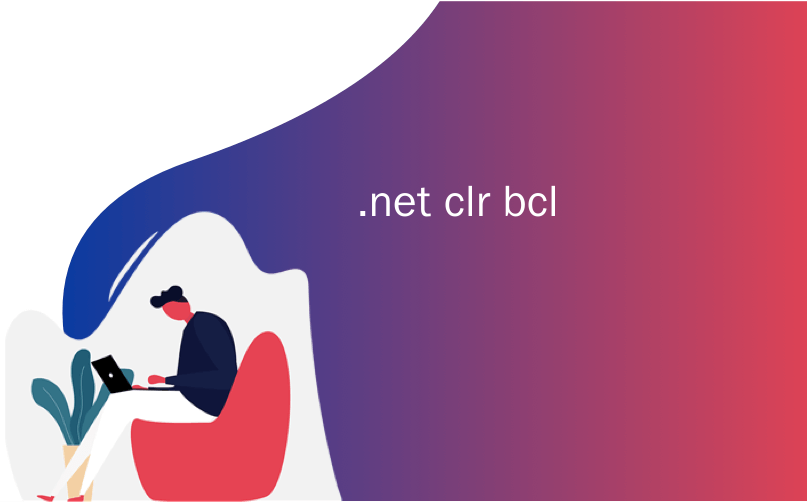
.net clr bcl
Just got a great tweet from Jeremiah Morrill about .NET 4.
Jeremiah Morrill刚刚获得了有关.NET 4的推文。
I love a challenge! In my first Whirlwind Tour post on ASP I mentioned how COM Interop and Office Interop was fun with 4.
我喜欢挑战! 在我的第一篇关于ASP的Whirlwind Tour帖子中,我提到了COM Interop和Office Interop如何与4一起玩。
I've done a lot of COM Interop with C# and a LOT of Office Automation. Once upon a time, I worked at a company called Chrome Data, and we created a Fax Server with a Digiboard. Folks would call into a number, and the person who took the order would pick the make/model/style/year of the car and "instantly" fax a complete report about the vehicle. It used VB3, SQL Server 4.21 and Word 6.0 and a magical thing called "OLE Automation."
我已经用C#和很多Office Automation完成了很多COM Interop。 曾几何时,我在一家名为Chrome Data的公司工作,我们用Digiboard创建了一个传真服务器。 市民会拨打电话,接单的人会选择汽车的品牌/型号/样式/年份,并立即“传真”有关该车辆的完整报告。 它使用了VB3,SQL Server 4.21和Word 6.0,以及一个神奇的东西,叫做“ OLE Automation”。
Fast forward 15 years and I sent an email to Mads Torgerson, a PM on C# that said:
快进15年了,我给C#经理PMs Torgerson发送了一封电子邮件,其中说:
I’m doing a sample for a friend where I’m simply spinning through an Automation API over a Word Doc to get and change some CustomDocumentProperties.
我正在为一个朋友做一个示例,在该示例中,我只是通过Word Doc上的Automation API进行旋转以获取和更改一些CustomDocumentProperties。
I’m really surprised at how current C# sucks at this. Of course, it makes sense, given all the IDispatch code in Word, but still. Dim != var as they say. Fix it!
我对当前的C#在这方面的表现感到非常惊讶。 当然,考虑到Word中的所有IDispatch代码,这还是有道理的,但仍然如此。 他们说的是Dim!= var。 修理它!
Well, everything except "fix it!" is true. I added that just now. ;) I did a post on this a while back showing how scary the C# code was. This is/was somewhere where Visual Basic truly excels. I vowed to only use VB for Office Automation code after this fiasco.
好吧,除了“修复它”之外的所有东西! 是真的。 我刚才添加了。 ;)我前一段时间对此发表了一篇文章,展示了C#代码的可怕程度。 这是/曾经是Visual Basic真正擅长的地方。 在此惨败之后,我发誓只将VB用于Office Automation代码。
If you want to melt your brain, check out the old code. No joke. I've collapsed the block because it's too scary. See the "ref missings"? The reflection? The Get/Sets? Scandalous!
如果您想使自己的大脑融化,请查看旧代码。 不是开玩笑。 我已将其折叠,因为它太吓人了。 看到“裁判失踪”? 反思? 获取/设置? 太丑了!
{
ApplicationClass WordApp = new ApplicationClass();
WordApp.Visible = true;
object missing = System.Reflection.Missing.Value;
object readOnly = false;
object isVisible = true;
object fileName = Path.Combine(AppDomain.CurrentDomain.BaseDirectory, @"..\..\..\NewTest.doc");
Microsoft.Office.Interop.Word.Document aDoc = WordApp.Documents.Open(
ref fileName,ref missing,ref readOnly,ref missing,
ref missing,ref missing,ref missing,ref missing,
ref missing,ref missing,ref missing,ref isVisible,
ref missing,ref missing,ref missing,ref missing);
aDoc.Activate();
string propertyValue = GetCustomPropertyValue(aDoc, "CustomProperty1");
SetCustomPropertyValue(aDoc, "CustomProperty1", "Hanselman");
foreach (Range r in aDoc.StoryRanges)
{
r.Fields.Update();
}
}
public string GetCustomPropertyValue(Document doc, string propertyName)
{
object oDocCustomProps = doc.CustomDocumentProperties;
Type typeDocCustomProps = oDocCustomProps.GetType();
object oCustomProp = typeDocCustomProps.InvokeMember("Item",
BindingFlags.Default |
BindingFlags.GetProperty,
null, oDocCustomProps,
new object[] { propertyName });
Type typePropertyValue = oCustomProp.GetType();
string propertyValue = typePropertyValue.InvokeMember("Value",
BindingFlags.Default |
BindingFlags.GetProperty,
null, oCustomProp,
new object[] { }).ToString();
return propertyValue;
}
public void SetCustomPropertyValue(Document doc, string propertyName, string propertyValue)
{
object oDocCustomProps = doc.CustomDocumentProperties;
Type typeDocCustomProps = oDocCustomProps.GetType();
typeDocCustomProps.InvokeMember("Item",
BindingFlags.Default |
BindingFlags.SetProperty,
null, oDocCustomProps,
new object[] { propertyName, propertyValue });
}
Fast forward to C# under .NET 4.
在.NET 4下快进至C#。
var WordApp = new ApplicationClass();
WordApp.Visible = true;
string fileName = @"NewTest.doc";
Document aDoc = WordApp.Documents.Open(fileName, ReadOnly: true, Visible: true);
aDoc.Activate();
string propertyValue = aDoc.CustomDocumentProperties["FISHORTNAME"].Value;
aDoc.CustomDocumentProperties["FISHORTNAME"].Value = "HanselBank";
string newPropertyValue = aDoc.CustomDocumentProperties["FISHORTNAME"].Value
foreach (Range r in aDoc.StoryRanges)
{
foreach (Field b in r.Fields)
{
b.Update();
}
}
See how all the crap that was originally reflection gets dispatched dynamically? But that's not even that great an example.
看看原来反射的所有废话是如何动态分配的? 但这还不是一个很好的例子。
使用C#4的Word和Excel自动化 (Word and Excel Automation with C# 4)
That's just an example from Jonathan Carter and Jason Olson that gets running processes (using LINQ, woot) then makes a chart in Excel, then puts the chart in Word.
这只是乔纳森·卡特( Jonathan Carter)和杰森·奥尔森( Jason Olson)的一个示例,该示例获取正在运行的进程(使用LINQ,woot),然后在Excel中制作图表,然后将图表放入Word中。
using System;
using System.Diagnostics;
using System.Linq;
using Excel = Microsoft.Office.Interop.Excel;
using Word = Microsoft.Office.Interop.Word;
namespace One.SimplifyingYourCodeWithCSharp
{
class Program
{
static void Main(string[] args)
{
GenerateChart(copyToWord: true);
}
static void GenerateChart(bool copyToWord = false)
{
var excel = new Excel.Application();
excel.Visible = true;
excel.Workbooks.Add();
excel.get_Range("A1").Value2 = "Process Name";
excel.get_Range("B1").Value2 = "Memory Usage";
var processes = Process.GetProcesses()
.OrderByDescending(p => p.WorkingSet64)
.Take(10);
int i = 2;
foreach (var p in processes)
{
excel.get_Range("A" + i).Value2 = p.ProcessName;
excel.get_Range("B" + i).Value2 = p.WorkingSet64;
i++;
}
Excel.Range range = excel.get_Range("A1");
Excel.Chart chart = (Excel.Chart)excel.ActiveWorkbook.Charts.Add(
After: excel.ActiveSheet);
chart.ChartWizard(Source: range.CurrentRegion,
Title: "Memory Usage in " + Environment.MachineName);
chart.ChartStyle = 45;
chart.CopyPicture(Excel.XlPictureAppearance.xlScreen,
Excel.XlCopyPictureFormat.xlBitmap,
Excel.XlPictureAppearance.xlScreen);
if (copyToWord)
{
var word = new Word.Application();
word.Visible = true;
word.Documents.Add();
word.Selection.Paste();
}
}
}
}
Notice the named parameters in C#, like 'Title: "whatever"' and "copyToWord: true"?
注意C#中的命名参数,例如'Title:“ whatever”'和“ copyToWord:true”?
PIA不再代表* ss中的痛苦-类型等效和嵌入式互操作程序集 (PIAs no long stand for Pain in the *ss - Type Equivalence and Embedded Interop Assemblies)
Primary Interop Assemblies are .NET assemblies that bridge the gap between a .NET app and a COM server. They are also a PIA, ahem. When there's articles about your technology on the web called "Common Pitfalls With ______" you know there's trouble.
主互操作程序集是.NET程序集,它们弥合了.NET应用程序和COM服务器之间的空白。 他们也是PIA,哎呀。 当网络上有关于您的技术的文章称为“ ______的常见陷阱”时,您就知道有麻烦了。
Typically you reference these Interop assemblies in Visual Studio and they show up, predictably, as references in your assembly. Here's a screenshot with the project on the right, and the assembly under Reflector on the left.
通常,您在Visual Studio中引用这些Interop程序集,并且可以预见地将它们显示为程序集中的引用。 这是一个截图,右边是项目,左边是Reflector下的程序集。
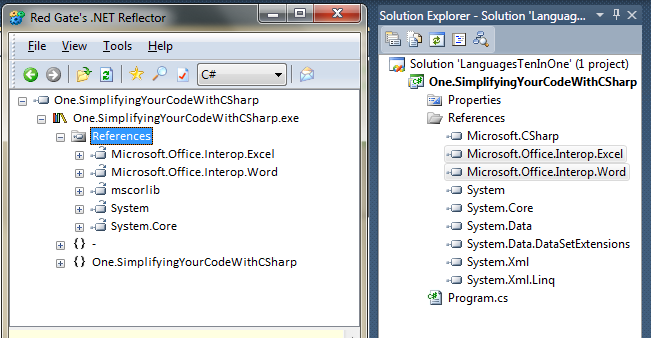
This means that those PIAs better be deployed on the end user's machine. Some PIAs can be as large as 20 megs or more! That's because for each COM interface, struct, enum, etc, there is a managed equivalent for marshalling data. That sucks, especially if I just want to make a chart and I'm not using any other types. It's great that Office has thousands of types, but don't make me carry them all around.
这意味着最好将这些PIA部署在最终用户的计算机上。 一些PIA可能高达20兆或更大! 这是因为对于每个COM接口,结构,枚举等,都有用于编组数据的托管等效项。 太糟糕了,特别是如果我只是想制作图表并且不使用任何其他类型的话。 Office有成千上万种类型,这很好,但不要让我随身携带它们。
However, now I can click on Properties for these references and click Embed Interop Types = true. Now, check the screenshot. The references are gone and new types have appeared.
但是,现在我可以单击这些引用的属性,然后单击Embed Interop Types = true。 现在,检查屏幕截图。 引用不见了,出现了新的类型。
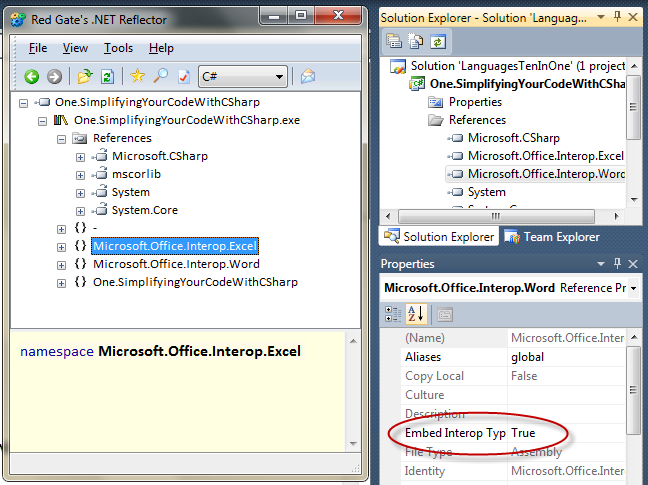
Just the types I was using are now embedded within my application. However, since the types are equivalent, the runtime handles this fact and we don't have to do anything.
现在,我所使用的类型已嵌入到我的应用程序中。 但是,由于类型是等效的,因此运行时可以处理此事实,我们无需执行任何操作。
This all adds up to Office and COM Interop that is actually fun to do. Ok, maybe not fun, but better than a really bad paper cut. Huh, Jeremiah? ;)
所有这些加起来实际上使Office和COM Interop变得很有趣。 好吧,也许不好玩,但是比真正糟糕的剪纸要好。 耶利米吗? ;)
.net clr bcl