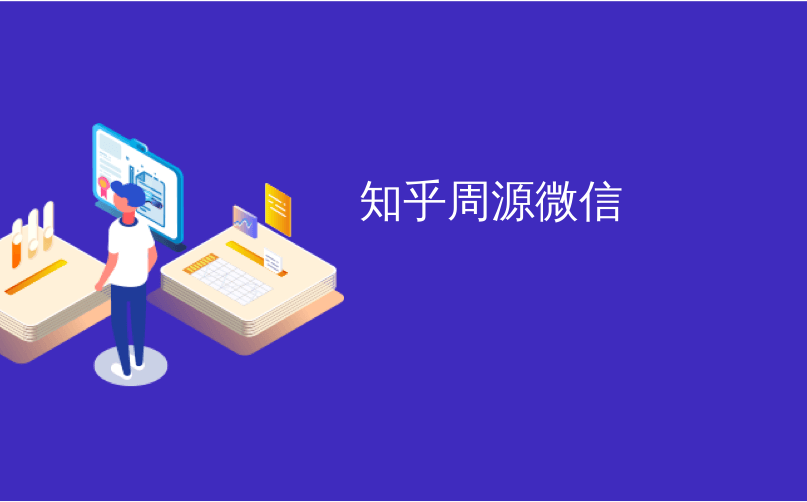
知乎周源微信
Dear Reader, I present to you nineteenth in a infinite number of posts of "The Weekly Source Code."
At Mix, Clint Rutkas and I were messing around writing a plugin model for one of his apps. We were prototyping and I typed up this typical-looking plugin style code:
在Mix上, Clint Rutkas和我正在为他的一个应用程序编写一个插件模型。 我们正在制作原型,我输入了这个看起来很典型的插件样式代码:
string[] filesToTest = Directory.GetFiles(Path.Combine(AppDomain.CurrentDomain.BaseDirectory, "plugins"), "*.dll");
foreach (string file in filesToTest)
{
Assembly a = Assembly.LoadFrom(file);
foreach (Type t in a.GetTypes())
{
if (!t.IsAbstract && t.BaseType == typeof(MyBaseClass))
{
myListOfInstances.Add((MyBaseClass)Activator.CreateInstance(t, credentials));
}
}
}
It's pretty straightforward and has little (read: no) error handling. It spins through the DLLs in the /plugins folder, loads them each (this should be more explicit rather than a blanket load of all dlls in a folder), then looks for any types that are derived from MyBaseClass that aren't abstract, then instantiates them and puts them in a list.
它非常简单,几乎没有(未读)错误处理。 它遍历/ plugins文件夹中的DLL,分别加载它们(这应该更明确,而不是对文件夹中所有dll进行全面加载),然后查找从MyBaseClass派生的不是抽象的任何类型,然后实例化它们并将它们放在列表中。
I'm going to quote Jeff Moser's very good blog post "What does it take to be a grandmaster" in both this post and the next one I write. It's that good. Seriously, go read it now, I'll wait here.
在这篇文章和我写的下一篇文章中,我都会引用Jeff Moser的非常好的博客文章“成为一名大师大师需要做什么” 。 就是那样认真地说,现在就去读吧,我在这里等。
Jeff invokes Anders Heilsberg (Father of C# and LINQ) and:
Jeff调用Anders Heilsberg(C#和LINQ之父),并且:
"Anders' recent statement that future versions of C# will be about getting the programmer to declare "more of the what, and less of the how" with respect to how a result gets computed. A side effect of this is that your "chunks" tend to be more efficient for the runtime, and more importantly, your brain."
“ Anders最近发表声明说,未来的C#版本将使程序员声明“如何计算结果,而对方法则更少” 。这样做的副作用是您的“块”往往对运行时更有效,更重要的是对您的大脑而言。”
I'm going to repeat part I bolded. I like Programming Languages that allow me to declare "more of the what, and less of the how." This how we tried to design the system at my last job and I automatically mentally migrate towards systems that encourage this kind of thinking.
我将重复我加粗的部分。 我喜欢允许我声明“更多内容,而更少内容”的编程语言。 这是我们在上一份工作中尝试设计系统的方式,并且我自动地心智迁移到鼓励这种思维的系统。
So, back to the foreach loops above. My good friend (and fellow baby sign language fan) Kzu came by while Clint and I were working and suggested we turn this increasingly complex procedure into a LINQ query.
因此,回到上面的foreach循环。 我的好朋友(和婴儿手语爱好者) Kzu在Clint和我正在工作的时候来了,建议我们将这个日益复杂的过程转换为LINQ查询。
After a few tries, here's what we came up with.
经过几次尝试,这就是我们想到的。
myListOfInstances =
(from file in Directory.GetFiles(Path.Combine(AppDomain.CurrentDomain.BaseDirectory, "plugins"), "*.dll")
let a = Assembly.LoadFrom(file)
from t in a.GetTypes()
where !t.IsAbstract && t.BaseType == typeof(MyBaseClass)
select (MyBaseClass)Activator.CreateInstance(t, credentials))
.ToList();
This is all one line, with some whitespace for readability. It says the same thing as the first chunk of code, but for some minds, it might be easier to read. This isn't a very good example of LINQ shining, from my point of view even though I like reading it. Certainly there's nowhere (I can see) for me to put error handling code that catches a bad load or bad cast in this example. There are, however, a LOT of places within that single line that one could set a breakpoint.
这都是一行,带有一些空格以提高可读性。 它和第一段代码说的一样,但是对于某些人来说,它可能更容易阅读。 从我的角度来看,这不是LINQ闪光的一个很好的例子,尽管我喜欢阅读它。 当然,在此示例中,没有地方(我可以看到)放置错误处理代码来捕获不良负载或不良转换。 但是,在那一行中有很多地方可以设置断点。
A better example would be like the one Jeff uses:
一个更好的例子就像Jeff使用的那样:
var primes = new int[] { 2,3,5,7,11,13,17,19,13,29,31,37,41 };
var primeSum = 0;
for (int i = 0; i < primes.Length; i++)
{
primeSum += primes[i];
}
...or even...
...甚至...
var primes = new int[] { 2,3,5,7,11,13,17,19,13,29,31,37,41 };
var primeSum = 0;
foreach (int i in primes)
{
primeSum += i;
}
...could be more easily written and read like:
...可能更容易编写和阅读,例如:
var primes = new int[] { 2,3,5,7,11,13,17,19,13,29,31,37,41 };
var primeSum = primes.Sum();
where .Sum() is part of LINQ as Arrays are IEnumerable.
其中.Sum()是LINQ的一部分,因为Array是IEnumerable的。
I totally recommend you go get the free, no-installer LINQPad that comes with 200 examples from C# in a Nutshell.
我完全建议您从Nutshell中获得免费的,无需安装的LINQPad ,其中随附C#中的200个示例。
Here are a few examples that I think really typify pretty code; certainly an improvement over the standard procedural code I'd usually write:
以下是一些我认为确实代表漂亮代码的示例。 肯定比我通常编写的标准过程代码有所改进:
var names = new[] { "Tom", "Dick", "Harry" }.Where(n => n.Length >= 4);
Here's onen that does quite a few things to a list in a fairly clean syntax:
这里的onon以相当干净的语法对列表做了很多事情:
string[] names = { "Tom", "Dick", "Harry", "Mary", "Jay" };
var results =
(
from n in names
where n.Contains("a") // Filter elements
orderby n.Length // Sort elements
select n.ToUpper() // Translate each element (project)
).ToList();
What LINQ-style queries have you written that feel more what than how?
您编写了什么LINQ风格的查询,感觉比实际的还要多?
Related, ahem, LINQS
相关的,啊,LINQS
Get namespaces from an XML Document with XPathDocument and LINQ to XML
Improving LINQ Code Smell with Explicit and Implicit Conversion Operators
翻译自: https://www.hanselman.com/blog/the-weekly-source-code-19-linq-and-more-what-less-how
知乎周源微信