1. 链表从指定节点后方插入新节点(126.9.6)
#include <stdio.h>
struct Test
{
int data;
struct Test *next;
};
void insertFromBehind(struct Test *head,int data,struct Test *new)
{
struct Test *p=head;
while(p != NULL){
if(p->data==data){
new->next=p->next;
p->next=new;
}
p=p->next;
}
}
void printLink(struct Test *head)
{
struct Test *point;
point=head;
while(point != NULL){
printf("%d ",point->data);
point=point->next;
}
putchar('\n');
}
int getLinkTotalNodeNum(struct Test *head)
{
int cnt=0;
struct Test *p=head;
while(p != NULL){
cnt++;
p=p->next;
}
return cnt;
}
int searchLink(struct Test *head,int data)
{
while(head != NULL){
if(head->data==data){
return 1;
}
head=head->next;
}
return 0;
}
int main()
{
int i;
int array[]={1,2,3};
for(i=0;i<sizeof(array)/sizeof(array[0]);i++){
printf("%d ",array[i]);
}
putchar('\n');
struct Test t1={1,NULL};
struct Test t2={2,NULL};
struct Test t3={3,NULL};
struct Test t4={4,NULL};
struct Test t5={5,NULL};
struct Test t6={6,NULL};
t1.next=&t2;
t2.next=&t3;
t3.next=&t4;
t4.next=&t5;
t5.next=&t6;
printf("use t1 to print three nums:\n");
printLink(&t1);
struct Test new={100,NULL};
puts("after insert behind:");
insertFromBehind(&t1,6,&new);
printLink(&t1);
return 0;
}
2. 链表从指定节点前方插入新节点(127.9.7)
#include <stdio.h>
struct Test
{
int data;
struct Test *next;
};
struct Test* insertFromFor(struct Test *head,int data,struct Test *new)
{
struct Test *p=head;
if(p->data==data){
new->next=p;
return new;
}
while(p->next != NULL){
if(p->next->data==data){
new->next=p->next;
p->next=new;
printf("insert ok\n");
return head;
}
p=p->next;
}
printf("no data insert %d\n",data);
return head;
}
void insertFromBehind(struct Test *head,int data,struct Test *new)
{
struct Test *p=head;
while(p != NULL){
if(p->data==data){
new->next=p->next;
p->next=new;
}
p=p->next;
}
}
void printLink(struct Test *head)
{
struct Test *point;
point=head;
while(point != NULL){
printf("%d ",point->data);
point=point->next;
}
putchar('\n');
}
int getLinkTotalNodeNum(struct Test *head)
{
int cnt=0;
struct Test *p=head;
while(p != NULL){
cnt++;
p=p->next;
}
return cnt;
}
int searchLink(struct Test *head,int data)
{
while(head != NULL){
if(head->data==data){
return 1;
}
head=head->next;
}
return 0;
}
int main()
{
int i;
int array[]={1,2,3};
for(i=0;i<sizeof(array)/sizeof(array[0]);i++){
printf("%d ",array[i]);
}
putchar('\n');
struct Test t1={1,NULL};
struct Test t2={2,NULL};
struct Test t3={3,NULL};
struct Test t4={4,NULL};
struct Test t5={5,NULL};
struct Test t6={6,NULL};
t1.next=&t2;
t2.next=&t3;
t3.next=&t4;
t4.next=&t5;
t5.next=&t6;
struct Test *head=&t1;
printf("use t1 to print three nums:\n");
printLink(head);
struct Test new={100,NULL};
puts("after insert behind:");
insertFromBehind(head,6,&new);
printLink(head);
struct Test new2={122,NULL};
head=insertFromFor(head,1,&new2);
puts("after insert head forward:");
printLink(head);
struct Test new3={66,NULL};
head=insertFromFor(head,2,&new3);
puts("after insert forward:");
printLink(head);
struct Test new4={66,NULL};
head=insertFromFor(head,20,&new3);
puts("after insert forward:");
printLink(head);
return 0;
}
3. 链表删除指定节点(128.9.8)
- gdb用法
gcc test.c -g
gdb a.out
run
q
y
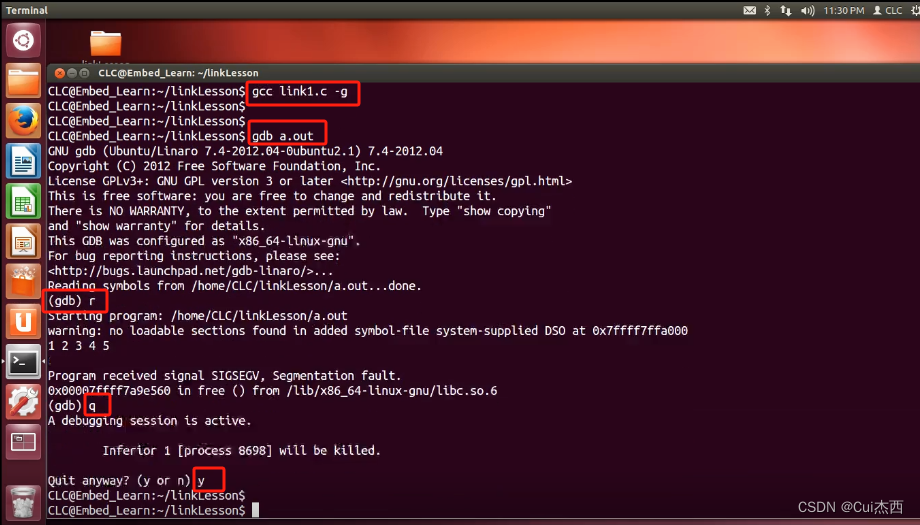
#include <stdio.h>
#include <stdlib.h>
struct Test
{
int data;
struct Test *next;
};
void printLink(struct Test *head)
{
struct Test *point;
point=head;
while(point != NULL){
printf("%d ",point->data);
point=point->next;
}
putchar('\n');
}
struct Test* deleteNode(struct Test *head,int data)
{
struct Test *p=head;
if(p->data==data){
head=head->next;
return head;
}
while(p->next != NULL){
if(p->next->data==data){
p->next=p->next->next;
return head;
}
p=p->next;
}
return head;
}
int main()
{
struct Test *head=NULL;
struct Test t1={1,NULL};
struct Test t2={2,NULL};
struct Test t3={3,NULL};
struct Test t4={4,NULL};
struct Test t5={5,NULL};
struct Test t6={6,NULL};
head=&t1;
t1.next=&t2;
t2.next=&t3;
t3.next=&t4;
t4.next=&t5;
t5.next=&t6;
printLink(head);
head=deleteNode(head,1);
printLink(head);
head=deleteNode(head,3);
printLink(head);
return 0;
}
4. 链表动态创建之头插法(129.9.9)
#include <stdio.h>
#include <stdlib.h>
struct Test
{
int data;
struct Test *next;
};
struct Test* insertFromHead(struct Test *head)
{
struct Test *new;
while(1){
new=(struct Test*)malloc(sizeof(struct Test));
if(new == NULL){
printf("Memory allocation failed.\n");
return head;
}
printf("input your new node data:\n");
scanf("%d",&(new->data));
if(new->data == 0){
printf("0 quit\n");
return head;
}
if(head == NULL){
head=new;
}else{
new->next=head;
head=new;
}
}
}
void printLink(struct Test *head)
{
struct Test *point;
point=head;
while(point != NULL){
printf("%d ",point->data);
point=point->next;
}
putchar('\n');
}
int main()
{
struct Test *head=NULL;
head=insertFromHead(head);
printLink(head);
return 0;
}
5. 头插法优化补充(130.9.10)
#include <stdio.h>
#include <stdlib.h>
struct Test
{
int data;
struct Test *next;
};
struct Test* insertFromHead(struct Test *head,struct Test *new)
{
if(head == NULL){
head=new;
}else{
new->next=head;
head=new;
}
return head;
}
struct Test* creatClink(struct Test *head)
{
struct Test *new;
while(1){
new=(struct Test*)malloc(sizeof(struct Test));
if(new == NULL){
printf("Memory allocation failed.\n");
return head;
}
printf("input your new node data:\n");
scanf("%d",&(new->data));
if(new->data == 0){
printf("0 quit\n");
free(new);
return head;
}
head=insertFromHead(head,new);
}
}
void printLink(struct Test *head)
{
struct Test *point;
point=head;
while(point != NULL){
printf("%d ",point->data);
point=point->next;
}
putchar('\n');
}
int main()
{
struct Test *head=NULL;
head=creatClink(head);
printLink(head);
struct Test t1={520,NULL};
head=insertFromHead(head,&t1);
printLink(head);
return 0;
}
6. 尾插法创建链表(131.9.11)
#include <stdio.h>
#include <stdlib.h>
struct Test
{
int data;
struct Test *next;
};
struct Test* insertFromHead(struct Test *head,struct Test *new)
{
if(head == NULL){
head=new;
}else{
new->next=head;
head=new;
}
return head;
}
struct Test* creatClink(struct Test *head)
{
struct Test *new;
while(1){
new=(struct Test*)malloc(sizeof(struct Test));
if(new == NULL){
printf("Memory allocation failed.\n");
return head;
}
printf("input your new node data:\n");
scanf("%d",&(new->data));
if(new->data == 0){
printf("0 quit\n");
free(new);
return head;
}
head=insertFromHead(head,new);
}
}
struct Test* insertFromBehind(struct Test *head,struct Test *new)
{
struct Test *p=head;
if(p == NULL){
head=new;
return head;
}
while(p->next != NULL){
p=p->next;
}
p->next=new;
return head;
}
struct Test* creatClink2(struct Test *head)
{
struct Test *new;
while(1){
new=(struct Test*)malloc(sizeof(struct Test));
if(new == NULL){
printf("Memory allocation failed.\n");
return head;
}
printf("input your new node data:\n");
scanf("%d",&(new->data));
if(new->data == 0){
printf("0 quit\n");
free(new);
return head;
}
head=insertFromBehind(head,new);
}
}
void printLink(struct Test *head)
{
struct Test *point;
point=head;
while(point != NULL){
printf("%d ",point->data);
point=point->next;
}
putchar('\n');
}
int main()
{
struct Test *head=NULL;
head=creatClink2(head);
printLink(head);
struct Test t1={520,NULL};
head=insertFromHead(head,&t1);
struct Test t2={250,NULL};
head=insertFromBehind(head,&t2);
printLink(head);
return 0;
}