本篇内容: 对气泡(Popup)和菜单(Menu)学习
一、知识储备
1. 气泡(Popup)
- 就是绑定在组件上显示的气泡提醒弹窗。
- 分为两种系统气泡(PopupOptions)和自定义气泡(CustomPopupOptions)。
- 系统气泡常见设置
Button('弹窗')
.onClick(() => {
this.handlePopup = !this.handlePopup;
})
.bindPopup(this.handlePopup, { //绑定气泡,当参数this.handlePopup为true时,显示气泡
message: '这是个弹窗',
onStateChange: e => {
if (!e.isVisible) { //监听气泡的可见状态
this.handlePopup = false;
}
},
primaryButton: { //设置气泡的按钮
value: '确定',
action: () => {
this.handlePopup = !this.handlePopup;
}
},
secondaryButton: {
value: '取消',
action: () => {
this.handlePopup = !this.handlePopup;
}
}
})
@State isShow: boolean = false;
@State tips: string = '我是提示';
@Builder popupBuilder() {
Row({ space: 2 }) {
Image($r('app.media.app_icon')).width(20).height(20).margin(5)
Text(this.tips).fontSize(18)
}.width(200).height(36).padding(8)
}
build() {
Column() {
Button('自定义气泡喽')
.onClick(() => {
this.isShow = !this.isShow;
})
.bindPopup(this.isShow, {
builder: this.popupBuilder, //自定义的气泡
placement: Placement.Bottom, //气泡弹出在绑定组件的下方
popupColor: 0x2600c250,
onStateChange: e => {
if (e.isVisible) {
this.isShow = false;
}
}
})
}.height('50%')
}
2.菜单
Button('系统菜单')
.bindMenu([{
value: '出生',
action: () => {
this.tips = '出生'
}
}, {
value: '上学',
action: () => {
this.tips = '上学'
}
}])
- 自定义菜单,常用函数
- 自定义菜单,常用组件menu menuItem menuItemGroup
- 自定义组件属性绑定
Button('自定义组件')
.bindMenu(this.MyMenu)
@Builder customMenu() { //子菜单
Menu() {
MenuItem({ content: '出生', labelInfo: 'birthday' })
MenuItem({ content: '上学', labelInfo: 'school' })
}
}
@Builder mainMenu() { //主菜单
Menu() {
MenuItem({ startIcon: $r('app.media.icon'), content: '生命周期' })
MenuItem({ endIcon: $r('app.media.app_icon'), content: '菜单' }).enabled(false)
MenuItem({
startIcon: $r('app.media.app_icon'),
content: '前后图标菜单',
endIcon: $r('app.media.icon'),
builder: this.customMenu
})
MenuItemGroup({ header: '小标题' }) {
MenuItem({ content: '生命周期' })
.selectIcon(true)
.selected(this.selected)
.onChange((selected) => {
console.error('选中状态: ' + selected);
})
MenuItem({
startIcon: $r('app.media.app_icon'),
content: '前后图标菜单',
endIcon: $r('app.media.icon'),
builder: this.customMenu
})
}
}
}
build() {
Column() {
Button('自定义组件')
.bindMenu(this.mainMenu)
.bindContextMenu(this.mainMenu(), ResponseType.RightClick)
}.width('100%')
}
二、效果一览
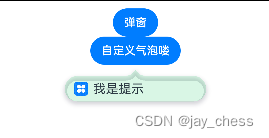
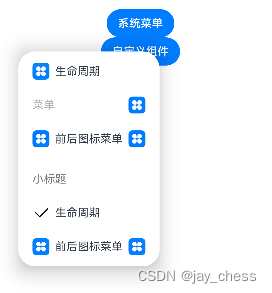
三、源码剖析
@Entry
@Component
struct MyMenu {
build() {
Column() {
SystemMenu()
CustomMenu()
}
}
}
@Component
struct CustomMenu {
@State selected: boolean = true
@Builder customMenu() { //子菜单
Menu() {
MenuItem({ content: '出生', labelInfo: 'birthday' })
MenuItem({ content: '上学', labelInfo: 'school' })
}
}
@Builder mainMenu() { //主菜单
Menu() {
MenuItem({ startIcon: $r('app.media.icon'), content: '生命周期' })
MenuItem({ endIcon: $r('app.media.app_icon'), content: '菜单' }).enabled(false)
MenuItem({
startIcon: $r('app.media.app_icon'),
content: '前后图标菜单',
endIcon: $r('app.media.icon'),
builder: this.customMenu
})
MenuItemGroup({ header: '小标题' }) {
MenuItem({ content: '生命周期' })
.selectIcon(true)
.selected(this.selected)
.onChange((selected) => {
console.error('选中状态: ' + selected);
})
MenuItem({
startIcon: $r('app.media.app_icon'),
content: '前后图标菜单',
endIcon: $r('app.media.icon'),
builder: this.customMenu
})
}
}
}
build() {
Column() {
Button('自定义组件')
.bindMenu(this.mainMenu)
.bindContextMenu(this.mainMenu(), ResponseType.RightClick)
}.width('100%')
}
}
@Component
struct SystemMenu {
@State tips: string = '';
build() {
Column() {
Text(this.tips)
.fontSize(33)
.fontColor(Color.Pink)
.textAlign(TextAlign.Center)
.width('100%')
.padding(10)
Button('系统菜单')
.bindMenu([{
value: '出生',
action: () => {
this.tips = '出生'
}
}, {
value: '上学',
action: () => {
this.tips = '上学'
}
}])
}
}
}
import thermal from '@ohos.thermal'
@Entry
@Component
struct MyPopup {
build() {
Column() {
Toast()
MyToast()
}
}
}
@Component
struct Toast {
@State handlePopup: boolean = false;
build() {
Column() {
Button('弹窗')
.onClick(() => {
this.handlePopup = !this.handlePopup;
})
.bindPopup(this.handlePopup, { //绑定气泡,当参数this.handlePopup为true时,显示气泡
message: '这是个弹窗',
onStateChange: e => {
if (!e.isVisible) { //监听气泡的可见状态
this.handlePopup = false;
}
},
primaryButton: { //设置气泡的按钮
value: '确定',
action: () => {
this.handlePopup = !this.handlePopup;
}
},
secondaryButton: {
value: '取消',
action: () => {
this.handlePopup = !this.handlePopup;
}
}
})
}.width('100%').padding({ top: 10 })
}
}
@Component
struct MyToast {
@State isShow: boolean = false;
@State tips: string = '我是提示';
@Builder popupBuilder() {
Row({ space: 2 }) {
Image($r('app.media.app_icon')).width(20).height(20).margin(5)
Text(this.tips).fontSize(18)
}.width(200).height(36).padding(8)
}
build() {
Column() {
Button('自定义气泡喽')
.onClick(() => {
this.isShow = !this.isShow;
})
.bindPopup(this.isShow, {
builder: this.popupBuilder, //自定义的气泡
placement: Placement.Bottom, //气泡弹出在绑定组件的下方
popupColor: 0x2600c250,
onStateChange: e => {
if (e.isVisible) {
this.isShow = false;
}
}
})
}.height('50%')
}
}