1 图像处理、计算机视觉、Opencv
1.1 图像处理
图像处理是用计算机对图像进行分析,以达到所需结果的技术。图像处理包括三部分:
- 图像压缩
- 增强与复原
- 匹配、描述、识别
1.2 计算机视觉
计算机视觉可以看做是如何使用人工系统模拟人的视觉。
1.3 opencv简介及组成
opencv是一个开源发行的跨平台计算机视觉库,它实现了图像处理和计算机视觉方面的很多通用算法。
组成模块:
(1)【calib3d】———Calibration(校准)和3D这两个词的组合缩写。这个模块主要是相机校准和三维重建相关的内容,包括基本的多视角几何算法、单个立体摄像头标定,物体姿态估计,立体相似性算法,3D信息的重建等。
(2)【contrib】———Contribute/Experimental Stuf的缩写。该模块包含了一些不太稳定的可选功能,比如人脸识别、立体匹配、人工视网膜模型等技术。
(3)【core】———核心功能模块,包含如下内容:
OpenCV基本数据结构
动态数据结构
绘图函数
数组操作相关函数
辅助功能与系统函数和宏
与OpenGL的互操作
(4)【imgproc】———Image 和Process 这两个单词的缩写组合,图像处理模块。包含如下内容:
线性和非线性的图像滤波
图像的几何变换
其他的图像变换
直方图相关
结构分析和形状描述
运动分析和对象跟踪
特征检测
目标检测等内容
(5)【feature2d】———2D功能框架。包含如下内容:
特征检测和描述
特征检测器(Fearure Detectors) 通用接口
描述符提取器(Description Extractors) 通用接口
描述符匹配器(Description Eatchers) 通用接口
通用描述符(Generic Description)匹配器通用接口
关键点绘制函数和匹配功能绘制函数
(6)【flann】———Fast Library For Approximate Nearest Neighbors,高维的近似近邻快速搜索算法库,包含以下两个部分:
快速近似最近邻搜索
聚类
(7)【GPU】———运用GPU加速的计算机视觉模块。
(8)【highgui】———高层GUI图形用户界面,包含媒体的输入输出、视频捕捉、图像和视频的解码编码、图形交互界面的接口等内容。
(9)【legacy】———一些已经废弃的代码库,保留下来作为向下兼容,包含如下内容:
运动分析
期望最大化
直方图
平面细分(C API)
特征检测和描述(Feature Detection and Description)
描述符提取器(Description Dxtractor)的通用接口
通用描述符(Generic Description Matchers)的常用接口
匹配器
(10)【ml】———Machine Learning,机器学习模块, 基本上是统计模型和分类算法,包含如下内容:
统计模型 (Statistical Models)
一般贝叶斯分类器 (Normal Bayes Classifier)
K-近邻 (K-NearestNeighbors)
支持向量机 (Support Vector Machines)
决策树 (Decision Trees)
提升(Boosting)
梯度提高树(Gradient Boosted Trees)
随机树 (Random Trees)
超随机树 (Extremely randomized trees)
期望最大化 (Expectation Maximization)
神经网络 (Neural Networks)
MLData
(11)【nonfree】———一些具有专利的算法模块 ,包含特征检测和GPU相关的内容。最好不要商用。
(12)【objdetect】———目标检测模块,包含Cascade Classification(级联分类)和Latent SVM这两个部分。
(13)【ocl】———即OpenCL-accelerated Computer Vision,运用OpenCL加速的计算机视觉组件模块。
(14)【photo】———Computational Photography,包含图像修复和图像去噪两部分。
(15)【stitching】———images stitching,图像拼接模块,包含如下部分:
拼接流水线
特点寻找和匹配图像
估计旋转
自动校准
图片歪斜
接缝估测
曝光补偿
图片混合
(16)【superres】———SuperResolution,超分辨率技术的相关功能模块。
(17)【ts】———opencv测试相关代码,不用去管。
(18)【video】———视频分析组件,该模块包括运动估计,背景分离,对象跟踪等视频处理相关内容。
(19)【Videostab】———Video stabilization,视频稳定相关的组件
核心模块:
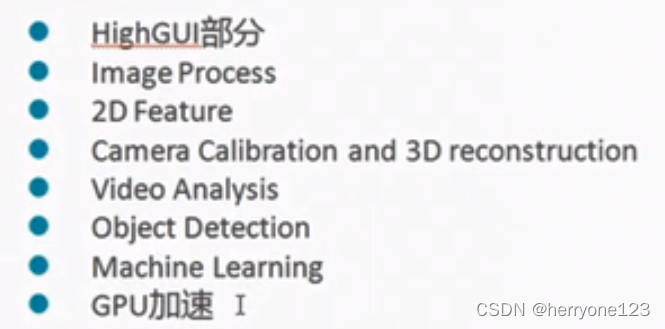
2 opencv基本应用
2.1 读图片并显示
#include <iostream>
#include <opencv2/opencv.hpp>
#include <opencv2/highgui.hpp>
using namespace cv;
using namespace std;
int main()
{
//read image
Mat testImage = imread("girl.jpg");
Mat gray = imread("girl.jpg" , IMREAD_GRAYSCALE);
if (!testImage.data) //check for invalid input
{
cout << "could not find the image" << endl;
return -1;
}
//wirte iamges
// imwrite("lenaGray.jpg",gray);
int myRow = testImage.rows - 1;
int myCol = testImage.cols - 1;
Vec3b pixel = testImage.at<Vec3b>(myRow,myCol);
cout<<"pixel value(R,G,B):"<<(int)pixel[0]<<","<<(int)pixel[1] << "," <<(int)pixel[2]<<endl;
//show image
imshow("opencv test", testImage);
imshow("gray image",gray);
waitKey(0);
return 0;
}
2.2 读取视频摄像头
需要opencv较低版本才能打开摄像头,测试opencv 4.3版本能打开摄像头,但是无显示。
#include <iostream>
#include <opencv2\opencv.hpp>
using namespace cv;
int main()
{
VideoCapture cap(0);//打开默认相机
//cap.open(0);
if (!cap.isOpened())
return -1;
namedWindow("Vidio", 1);
Mat frame;
while (1)
{
cap >> frame;
if (!frame.empty())
{
imshow("vidio", frame);
}
else
{
// cout << "can not find frame" << endl;
}
//if (waitKey(30) >= 0) break;
waitKey(30);
}
cap.release();
return 0;
}
2.3 用户界面操作
#include <iostream>
#include <opencv2/opencv.hpp>
#include <opencv2/highgui.hpp>
using namespace cv;
using namespace std;
int main()
{
//read image
Mat testImage = imread("girl.jpg");
Mat grayImage = imread("girl.jpg", IMREAD_GRAYSCALE);
if (!testImage.data) //check for invalid input
{
cout << "could not find the image" << endl;
return -1;
}
namedWindow("TestImage",WINDOW_GUI_NORMAL);
namedWindow("GrayImage", WINDOW_AUTOSIZE);
//move windows
moveWindow("TestImage", 10,10);
moveWindow("GrayImage", 520, 10);
//show windows
imshow("TestImage", testImage);
imshow("GrayImage", grayImage);
resizeWindow("TestImage",512,512);
waitKey(0);
//destory the windows
destroyWindow("TestImage");
destroyWindow("GrayImage");
return 0;
}
2.4 鼠标和滑块的使用
#include <iostream>
#include <opencv2/opencv.hpp>
#include <opencv2/highgui.hpp>
using namespace cv;
using namespace std;
//create a variable to save the position
int blurAmount = 15;
//trackbar callback function
static void onChange(int pos,void * userInput);
//mouse callback
static void onMouse(int event,int x,int y,int ,void * userInput);
//mouse callback
int main()
{
//read image
Mat testImage = imread("girl.jpg");
//create windows
namedWindow("TestImage",WINDOW_GUI_NORMAL);
//move windows
moveWindow("TestImage", 10,10);
//create a trackbar
createTrackbar("TestImage", "TestImage", &blurAmount, 30 , onChange ,&testImage);//为窗口添加trackbar 最大设置为30
setMouseCallback("TestImage", onMouse, &testImage);
//call to change to init
onChange(blurAmount,&testImage);
waitKey(0);
//destory the windows
destroyWindow("TestImage");
return 0;
}
// trackbar call back function 回调函数将基本的模糊滤镜应用于图像
static void onChange(int pos,void* userData)
{
if (pos < 0) return;//滑块为0 不使用过滤
Mat imgBlur;
//get the pointer input image
Mat* img = (Mat*)userData;
//apply a blur filer
blur(*img, imgBlur ,Size(pos,pos));
//show the result
imshow("TestImage", imgBlur);
}
//mouse callback
static void onMouse(int event,int x,int y,int ,void* userInput)
{
if (event != EVENT_LBUTTONDOWN)
{
return;
}
//get the pointer input image
Mat* img = (Mat*)userInput;
//draw circle
circle(*img,Point(x,y),10,Scalar(0,255,0), 3);
//call on change to get blurred image
onChange(blurAmount, img);
}
参考文献:
【1】OpenCV基本架构分析:OpenCV基本架构分析_博客主页-CSDN博客_opencv结构