C++中对文件操作需要包含头文件<fstream >
文件类型分为两种:
1.文本文件:文件以文本的ASCII码形式存储在计算机中2.二进制文件:文件以文本的二进制形式存储在计算机中,用户一般不能直接读懂它们
操作文件的三大类:
1.ofstream: 写操作
2. ifstream:读操作
3. fstream :读写操作文件打开方式:
打开方式 解释 ios:in 为读文件而打开文件 ios:out 为写文件而打开文件 ios:ate 初始位置:文件尾 ios:app 追加方式写文件 ios:trunc 如果文件存在先删除,再创建 ios:binary 二进制方式
文本文件
1、写文件
#include <iostream> using namespace std; #include <string> #include <fstream> //文本文件 写文件 void test01() { //1、包含头文件 fstream //2、创建流对象 ofstream ofs; //指定打开方式 ofs.open("Test.txt", ios::out); //4、写内容 ofs << "姓名:张三" << endl; ofs << "性别:男" << endl; ofs << "年龄:18" << endl; //5、关闭文件 ofs.close(); } int main() { test01(); system("pause"); return 0; }
2、读文件
#include <iostream> using namespace std; #include <string> #include <fstream> //读文件 void test01() { //1、包含头文件 //2、创建流对象 ifstream ifs; //3、打开文件 并且判断是否打开成功 ifs.open("test.txt", ios::in); if (!ifs.is_open()) { cout << "文件打开失败" << endl; return; } //4、读数据 //第一种 /*char buf[1024] = { 0 }; while (ifs >> buf) { cout << buf << endl; }*/ //第二种 /*char buf[1024] = { 0 }; while (ifs.getline(buf,sizeof(buf))){ cout << buf << endl; }*/ //3、第三种 /*string buf; while (getline(ifs, buf)) { cout << buf << endl; }*/ //4、第四种 char c; while ((c = ifs.get()) != EOF) { //EOF end of file cout << c; } //5、关闭文件 ifs.close(); } int main() { test01(); system("pause"); return 0; }
二进制文件
1、写文件
#include <iostream> using namespace std; #include <string> #include <fstream> class Person { public: char m_Name[64]; int m_Age; }; void test01() { //1、包含头文件 //2、创建流对象 ofstream ofs("person.txt", ios::out | ios::binary); //3、打开文件 //ofs.open("person.txt", ios::out | ios::binary); //4、写文件 Person p = { "张三",18 }; ofs.write((const char*)&p, sizeof(Person)); //5、关闭文件 ofs.close(); } int main() { test01(); system("pause"); return 0; }
2、读文件
#include <iostream> using namespace std; #include <string> #include <fstream> class Person { public: char m_Name[64]; int m_Age; }; void test01() { //1、包含头文件 //2、创建流对象 ifstream ifs; //3、打开文件 判断文件是否打开成功 ifs.open("person.txt", ios::in | ios::binary); if (!ifs.is_open()) { cout << "文件打开失败" << endl; return; } //4、读文件 Person p; ifs.read((char*)&p, sizeof(Person)); cout << "姓名:" << p.m_Name << " 年龄:" << p.m_Age << endl; //5、关闭文件 ifs.close(); } int main() { test01(); system("pause"); return 0; }
文件操作(个人学习笔记黑马学习)
最新推荐文章于 2024-11-05 15:56:29 发布
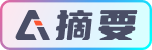