一、实验内容
使用IPAddress类、IPEndPoint类、IPHostEntry类 、DNS类的方法,显示中央电视台所有服务器的IP地址信息和本机主机名及相关IP地址。
二、效果图预览
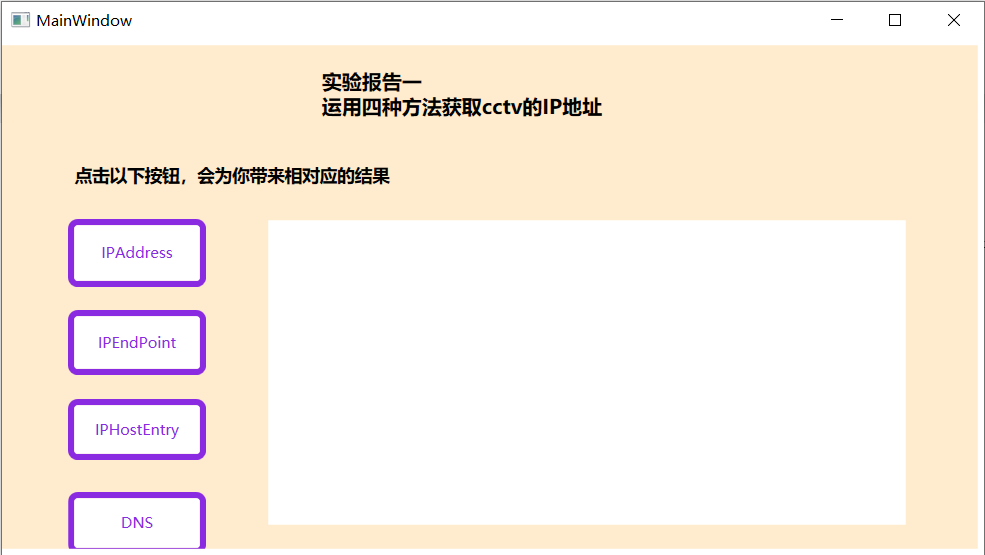
三.代码
以下为主窗口代码
<Window x:Class="实验报告1.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:实验报告1"
mc:Ignorable="d"
Title="MainWindow" Height="450" Width="800">
<Window.Resources>
<Color x:Key="SolidColorBrushColor1">#FF92E9F0</Color>
<Color x:Key="SolidColorBrushColor2">#FF8F15DD</Color>
</Window.Resources>
<Border BorderThickness="5" BorderBrush="White" >
<Grid Background="BlanchedAlmond" Margin="-5,0,0,0" >
<Grid.Resources>
<Style TargetType="TextBox">
<Setter Property="Foreground" Value="Black"/>
<Setter Property="Background" Value="BlanchedAlmond"/>
<Setter Property="BorderBrush" Value="BlanchedAlmond"/>
</Style>
<Style TargetType="Button">
<Setter Property="Foreground" Value="BlueViolet"/>
<Setter Property="Background" Value="White"/>
<Setter Property="BorderBrush" Value="BlueViolet"/>
<Setter Property="BorderThickness" Value="5"/>
<Style.Resources>
<Style TargetType="{x:Type Border}">
<Setter Property="CornerRadius" Value="5"/>
</Style>
</Style.Resources>
</Style>
</Grid.Resources>
<Button Content="IPAddress" HorizontalAlignment="Left" Margin="53,139,0,0" VerticalAlignment="Top" Height="54" Width="110" Click="IPAddress"/>
<Button Content="IPEndPoint" HorizontalAlignment="Left" Margin="53,212,0,0" VerticalAlignment="Top" Height="52" Width="110" Click="IPEndPoint" />
<Button Content="IPHostEntry" HorizontalAlignment="Left" Margin="53,283,0,0" VerticalAlignment="Top" Height="49" Width="110" Click="IPHostEntry"/>
<Button Content="DNS" HorizontalAlignment="Left" Margin="53,357,0,0" VerticalAlignment="Top" Height="50" Width="110" Click="DNS"/>
<TextBox x:Name="textbox1" TextWrapping="Wrap"
Background="White" Foreground="Black" FontSize="13" Text="" Margin="212,139,57,18" />
<Label Content="实验报告一
运用四种方法获取cctv的IP地址" Margin="156,10,215,335" Width="424" HorizontalContentAlignment="Center" VerticalContentAlignment="Center" FontSize="16" FontWeight="Bold"/>
<Label Content="点击以下按钮,会为你带来相对应的结果" HorizontalAlignment="Left" Margin="53,90,0,0" VerticalAlignment="Top" Height="33" Width="281" FontWeight="Bold" FontSize="14"/>
</Grid>
</Border>
</Window>
以下为cs部分代码
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Net.Sockets;
using System.Text;
using System.Threading.Tasks;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Data;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Imaging;
using System.Windows.Navigation;
using System.Windows.Shapes;
namespace 实验报告1
{
/// <summary>
/// MainWindow.xaml 的交互逻辑
/// </summary>
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
private void IPAddress(object sender, RoutedEventArgs e)
{
textbox1.Text = null;
StringBuilder sb = new StringBuilder();
sb.AppendLine("获取cctv的所有IP地址:");
try
{
IPAddress[] ips = Dns.GetHostAddresses("www.cctv.com");
foreach (IPAddress ip in ips)
{
sb.AppendLine(ip.ToString());
}
textbox1.Text = sb.ToString();
}
catch(Exception ex)
{
MessageBox.Show(ex.Message,"获取失败");
}
}
private void IPEndPoint(object sender, RoutedEventArgs e)
{
textbox1.Text = null;
textbox1.Text = "本次不使用该按钮";
}
private void IPHostEntry(object sender, RoutedEventArgs e)
{
textbox1.Text = null;
StringBuilder sb = new StringBuilder();
sb.AppendLine("获取cctv的所有IP地址:");
try
{
IPAddress[] ips = Dns.GetHostAddresses("www.cctv.com");
foreach (IPAddress ip in ips)
{
sb.AppendLine(ip.ToString());
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message, "获取失败");
}
string hostname = Dns.GetHostName();
sb.AppendLine();
sb.AppendLine("本机主机名:"+hostname);
IPHostEntry me = Dns.GetHostEntry(hostname);
foreach(IPAddress ip in me.AddressList)
{
if(ip.AddressFamily==AddressFamily.InterNetwork)
{
sb.AppendLine("ipv4:"+ip.ToString());
}
else if (ip.AddressFamily == AddressFamily.InterNetworkV6)
{
sb.AppendLine("ipv6:" + ip.ToString());
}
}
textbox1.Text=sb.ToString();
}
private void DNS(object sender, RoutedEventArgs e)
{
textbox1.Text = null;
textbox1.Text = "上面均在使用本类,故不做多余代码设计!";
}
}
}
四、待解决的问题
1.Label随着页面的大小变化而变化,无法固定在一个地方
2.Label内部字体会因为窗口没有放大而存在遮挡,不能随窗口大小变化而变化
3.textbox,textblock存在着很明显的遮挡现象,如果在其周围添加诸如label标签,会直接挡住(此问题多半为上一个问题的延伸),且这两个控件也存在很明显的随窗口大小变化的问题
如果是采用嵌套关系,如在textbox控件外加一个Border控件,那么该如何有限选中border控件而不是优先选中textbox控件?(重点解决,不解决容易 心肌梗塞)
五、实验感悟及获得的小技巧
block的字体颜色 foreground
block的背景颜色 background
block的边框怎么设置
好看的颜色合集
白杏仁色:BlanchedAlmond
蓝色暴力:Blueviolet
textbox可以设置圆角,但直接加border会更加简单,但是border和stackpanel等都只能有一个子控件(待商榷)
如果要建立两个控件之间的联系,务必加上x:Name的标识符,然后用该标识符去指当该控件
在wpf窗口界面,单击控件,右上角有一个电灯泡,点击电灯泡,可以直接操控控件