Lec0 :
1,数组
1.1创建数组
double[] myList; int n = 100; myList = new double[n];
myList是数组,myList[i]是元素double,数组索引从0开始
1.2数组方法与默认数值
arrayRefVar.length 返回长度
0 for the numeric primitive data types, '\u0000' for char types, and false for boolean types, and null for reference types
1.3数组应用
*交换同组内元素:double temp = myList[i]; myList[i] = myList[j]; myList[j] = temp;
*元素左移:double temp = myList[0];
for (int i = 1; i < myList.length; i++) { myList[i - 1] = myList[i]; }
*元素右移:double temp = myList[myList.length - 1];
for(int i=myList.length-1; i>0; i--) myList[i] = myList[i-1]; myList[0] = temp;
*遍历:In general, the syntax is
for (elementType value: arrayRefVar) { // Process the value }
*复制数组(注意=)
System.arraycopy(sourceArray, src_pos, targetArray, tar_pos, length);
例,把a全复制到b:System.arraycopy(a, 0, b, 0, a.length);
*调用数组:调用方法需要数组时,要么写数组名称,要么新建一个数组(数组没有显式引用变量,称为anonymous array)
调用方法内改变调用数组后,方法外的被调用数组会改变数值。但是传递实际值的原始类型值参数不会被方法改变。
1.4 Binary Search
The binary search first compares the key with the element in the middle of the array.
方法调用 java.util.Arrays.binarySearch(list, target Value))
1.5 Selection Sort
Selection sort finds the smallest number in the list and places it first. It then finds the smallest number in the remaining list and places it second, and so on until the list contains only a single number.(找最小值放前面,然后找除它之外的最小值,依次放好)
1.6 Insertion Sort
The insertion sort algorithm sorts a list of values by repeatedly inserting an unsorted element into a sorted sublist until the whole list is sorted.(从最后一位开始,一直往前移直到放到比它小的数后面)
java.util.Arrays.sort(list);
Lec 1
1. Object:
An object has a unique state and behavior:
the state of an object consists of a set of data fields (properties) with their current values
the behavior of an object is defined by a set of instance methods
2. Class
In Java classes are templates that define objects of the same type
A Java class uses: non-static/instance variables to define data fields non-static/instance methods to define behavior
class提供默认的constructors,或者可以自定义constructors,用于构造实例
Unified Modeling Language (UML) Class Diagram
3. 调用
Referencing the object’s data: objectRefVar.data
Invoking the object’s method: objectRefVar.methodName(arguments)
4. Static vs. Non-static variables
4.1Static variables and constants: 被类里所有实例共享
global variables for the entire class: for all objects instances of this class
eg :static final double PI = 3.141592;
4.2Non-static/instance variables are date fields of objects:
eg :System.out.println(myCircle.radius);
5. Default values
5.1 Java assigns no default value to a local variable inside a method.
5.2 Data fields have default values
6. Variables of Primitive Data Types and Object Types
6.1原始数据类型可以直接赋值,对象类型要new
6.2两个原始数据类型变量可以=,去交换数据。 两个对象类型变量=(i=j)意味着指向同一个地址的数据(j).
The object previously referenced by c1 is no longer referenced, it is called garbage . Garbage is automatically collected by the JVM, a process called garbage collection
7.两个class:Date和Random
7.1Date :java.util.Date date = new java.util.Date(); System.out.println(date.toString());
7.2Random :Random random1 = new Random(3);
for (int i = 0; i < 10; i++) System.out.print(random1.nextInt(1000) + " ");
8. Visibility Modifiers and Accessor/Mutator Methods
8.1 public (+ in UML) The class, data, or method is visible to any class in any package.
-radius: double -numberOfObjects: int
+Circle() +Circle(radius: double)
8.2 private (- in UML) The data or methods can be accessed only by the declaring class - To protect data!
注:getField (accessors) and setField (mutators) methods are used to read and modify private properties.
8.3The private modifier restricts access to within a class
The default modifier restricts access to within a package
public – unrestricted access
未标注的可被在同一个包里的不同类调用,public可以被任何人调用,private只能在该类里调用。
9. ttl里的getter和setter
9.1因为有些变量是私有的,所以外部类不能直接访问到这个变量。相反,外部代码必须调用getter()和setter()才能读取或更新变量。
eg : public String getName(){
return this.name;}
public void setName(String newName){
this.name = newName;}
(getter是获取变量值,所以要有return。setter是设置新变量值,所以调用的时候要有对应的新值)
9.2 String ,int ,char等等可以直接用上面的set和get。对于原始数据类型(int,char,double ...)Java将一个基元的值复制到了另一个而不是复制对象引用。而对该String对象的每次更改都会导致创建一个新的String对象。
9.3 集合类型的get和set:不要在setter直接复制引用到内部变量,不要在getter方法中返回原始对象的引用。相反,它应该返回原始对象的副本。(简单来说就是外包出去)
private List<String> listTitles;
public void setListTitles(List<String> titles) {
// this.listTitles = titles;
this.listTitles = new ArrayList<String>(titles);
}
public List<String> getListTitles() {
// return this.listTitles;
return new ArrayList<String>(this.listTitles);
}
public int[] getScores() {
int[] copy = new int[this.scores.length];
System.arraycopy(this.scores, 0, copy, 0, copy.length);
return copy;
}
public void setScores(int[] scr) {
this.scores = new int[scr.length];
System.arraycopy(scr, 0, this.scores, 0, scr.length);
}
Lec 2.1
1. Immutable Objects and Classes
1.1 Immutable object: the contents of an object cannot be changed once the object is created.
1.2 Its class is called an immutable class.
1.3 An immutable class: ①. It must mark all data fields private! ②. Provide no mutator (set) methods! ③. Provide no accessor methods that would return a reference to a mutable data field object(这里换句话说,不可变类的访问方法不应该返回对私有数据字段对象的引用)
eg: A类里只有private变量且没有任何set变量的方法,因此该类和对象无法更改
2. Scope of Variables and Default values
2.1 The scope of a local variable starts from its declaration and continues to the end of the block. So a local variable must be initialized explicitly.
2.2 Data Field Variables can be declared anywhere inside a class. 静态和实例作用于该类所以Initialized with default values.
3. The this Keyword
3.1 The this keyword is the name of a reference that refers to an object itself
3.2 Common uses : ①引用者隐藏自身(setter里的 this. var = var)
②To enable a constructor to invoke another constructor of the same class as the first statement in the constructor. 举个例子就是,一个学生类里有两个类似构造,其中第二种构造本质是调用第一种构造方法。
public Person(String name, int age) {
this.name = name;
this.age = age;
}
public Person(String name) {
this(name, 0); // 在这里使用this关键字来调用构造函数1,并传入默认的年龄值
}
4. Calling Overloaded Constructors
上面呢
public double getArea() { return this.radius * this.radius * Math.PI; }此处this可省略
5. Class Abstraction and Encapsulation
5.1 Class Abstraction = separate class implementation from the use of the class (API)抽象类会有描述让你知道怎么用
5.2 Class encapsulation = The user does not need to know how the class is implemented (private fields and only the public API is used).
6. 几个类实现就不展开了,只贴一个数栈实现
7. The String Class in detail(看一眼就行,不愧是detail)
7.1 Constructing Strings : String newString = new String(stringLiteral);
String message = "Welcome to Java";
7.2 A String object is immutable; its contents cannot be changed .
7.3 Interned Strings : String interning is a method of storing only one copy of each distinct compile-time constant/explicit string in the source code stored in a string intern pool.
简单来说就是直接给a,b字符串赋相同的值“阿米诺斯”,它们指向的是同一个内部字符串对象,a==b。但是如果你new了一个c并赋值“阿米诺斯”,c指向的是堆中新建的对象,c!=a和b。
7.4 String Class methods :
*字符串比较
①equals(Object object):a.equals( b )比较的是内容,而不是指向对象是否一致,所以上面6.3的a.equals(c) == true
②compareTo(Object object): Returns an integer greater than 0, equal to 0, or less than 0 to indicate whether this string is greater than, equal to, or less than s1.
*String Length, Characters, and Combining Strings
①length(): int ——>Returns the number of characters
②charAt(index: int): char——>Returns the character at the specified index
③concat(s1: String): String——>Returns a new string(s2,s1的结合)
*Retrieving Individual Characters in a String
①message.charAt(index)→ char 注意:索引从0开始,空格也占索引
*Extracting Substrings
①+subString(beginIndex: int): String
②subString(beginIndex: int, endIndex: int): String 返回从begin到end-1的str
*Finding a Character or a Substring
*
8. Regular Expressions :
替换,拆分,查找匹配可用正则表达式
eg :String s = "a+b$#c".replaceAll("[$+#]", "NNN");用NNN代替这几个符号
[\\d]{3}-[\\d]{2}-[\\d]{4} 匹配xxx-xx-xxxx的数字
[1-9][\\d]*[02468]匹配以非0开头,偶数结尾的数
String[] s3 = "Java;HTML:Perl,".split("[;:,]"); => [“Java”, “HTML”, “Perl”]
9. Command-Line Parameters
javac Calculator.java //编译Java源文件Calculator.java
java Calculator 1 + 2 //运行已经编译好的Calculator类,并给参数1,2
3 //结果
10. StringBuilder and StringBuffer
10.1 The StringBuilder/StringBuffer classes are alternatives to the String class,但是比str更灵活,你可以在缓冲区变更字符串。
10.2 ①StringBuffer is synchronized i.e. thread safe. It means two threads can't call the methods of StringBuffer simultaneously.
②StringBuilder is non-synchronized i.e. not thread safe. It means two threads can call the methods of StringBuilder simultaneously.
10.3 StringBuilder有各种append,insert,delect,toString方法(太多了,不粘了)
11. The Character Class
可以与其他character比较相同,大小,也可以去判断该character的数据类型。
equals和string的一样,compareTo类似
12. Designing Classes
12.1 Coherence: A class should describe a single entity
Separating responsibilities: A single entity with too many responsibilities can be broken into several classes to separate responsibilities
12.2 Reuse: Classes are designed for reuse!
12.3Place the data declaration before the constructor, and place constructors before methods.
Provide a public no-arg constructor and override the equals method and the toString method (returns a String) whenever possible.
Lec 2.2
1.Superclasses and Subclasses
1.1 Inheritance is the mechanism of basing a sub-class on extending another super-class
eg : public abstract class GeometricObject {...}
public class Circle extends GeometricObject {...}
2. Declaring a Subclass
2.1 在子类调用父类的方法时,使用super()或者super.method()
2.2 继承super的无参数构造函数可以隐藏super()。但是如果子类使用this去调用了另一个自己的构造函数,就要在最后一个被调用的构造函数里加上super()。
注:在继承链中构造一个类的实例,一定要顺着链去调用super的构造函数,注意sub的构造有隐藏的super()去调用父类的无参构造函数
2.3 Calling Superclass Methods with super
eg :父类里有public String toString(),子类可以public String toString() { return "Circle " + super.toString(); }去方法overriding
2.4 Method overloading
overloading is the ability to create multiple methods of the same name, but with different signatures and implementations。发生在同一个类中,允许定义多个同名方法,参数列表不同。在编译时,根据参数类型,顺序等匹配方法
2.5 Method overriding
要求与super has the same method signature。子类可以重新定义(覆盖)父类中的方法,方法名、参数列表和返回类型都保持一致。在运行时,动态绑定最具体的重载方法实现
3. The Object Class
3.1 所有类的superclass
3.2 toString() :The default Object implementation returns a string consisting of a class name of which the object is an instance, the @ ("at") sign, and a number representing this object
4. Polymorphism, Dynamic Binding and Generic Programming
4.1 Polymorphism: an object of a subtype can be used wherever its supertype value is required.它允许不同的对象对同一个消息做出不同的响应。例如对于函数m,不同的对象做m的参数会给出不同的输出。
4.2 Dynamic binding: the Java Virtual Machine determines dynamically at runtime which implementation is used by the method。在运行时确定对象的类型,顺着继承关系找该对象调用方法的实现是在哪里,以便调用相应的方法。
eg :即使编译时myDog是Animal,但是运行时它是Dog类,所以调Dog里的sound()
class Animal {
void sound() {
System.out.println("Some sound");
}
}
class Dog extends Animal {
void sound() {
System.out.println("阿米诺斯");
}
}
public class Main {
public static void main(String[] args) {
Animal myDog = new Dog();
myDog.sound(); // 调用的是Dog类中重写的方法,输出: 阿米诺斯 }
}
4.3 Generic programming: polymorphism allows methods to be used generically for a wide range of object arguments. 如果方法参数是super,则可传递sub的任意对象,方法的实现是动态决定的。
5. Casting Objects
5.1 Casting can be used to convert an object of one class type to another within an inheritance hierarchy。
还是上面的例子,m(new Dog()) 等同于 Animal myDog = new Dog(); m(myDog)
5.2 Explicit Casting :Dog dog = myDog 会出现编译错误,因为对象animal不一定是dog。这时我们需要显式转换 Dog dog = (Dog)myDog
5.3 instanceof :可以判断对象是不是某类的实例 myDog isinstanceof Dog
5.4 equals() : 比较两个对象 this == obj。可以根据需要去override
6. The ArrayList Class
6.1 ArrayList can be used to store an unlimited finite number of objects
6.2 创建 java.util.ArrayList<String> cityList=new java.util.ArrayList<String>();
java.util.ArrayList cityList = new java.util.ArrayList();
6.3 Custom stack :自定义堆栈,可以按需存放任何obj
7. The protected Modifier
7.1 A protected data or a protected method in a public class can be accessed by any class in the same package or its subclasses, even if the subclasses are in a different package
private, default (if no modifier is used), protected, public
从左到右,可行性增加。私有的只能该类下,默认的同包即可,公共大家都能用
7.2 UML Class Diagram
Visibility: ①+ = public ②- = private ③~ = default/package ④# = protected ⑤underlined = static
7.3 A Subclass Cannot Weaken the Accessibility
子类可以override父类的protected方法,将其变为public方法,但是不会改变父类方法的可访问性。
子类可以继承父类的static方法,但是不能override。如果子类重新定义了父类的static方法,子类中的方法隐藏了父类中的同名方法。下图的ref实例最后调用的是父类里的方法
class Parent {
static void staticMethod() {
System.out.println("Parent's static method");
}
}
class Child extends Parent {
static void staticMethod() {
System.out.println("Child's static method");
}
}
public class Main {
public static void main(String[] args) {
Parent.staticMethod(); // 输出: "Parent's static method"
Child.staticMethod(); // 输出: "Child's static method"
Parent ref = new Child();
ref.staticMethod(); // 输出: "Parent's static method"
}
}
与重载不同,重载的非静态方法这时在调用时会动态绑定,根据调用者的对象调用对应方法,例如ref就应该调用子类里的方法。
8. The final Modifier
8.1 A final variable is a constant
8.2 A final method cannot be overridden by its subclasses
A final class cannot be extended
Lec 3 Abstract Classes and Interfaces
1. Abstract Classes and Abstract Methods
1.1 The # sign indicates protected modifier,abstract classes and methods are italicized or have the annotation <abstarct>
public abstract class GeometricObject {
private java.util.Date dateCreated;
protected GeometricObject() {
dateCreated = new java.util.Date();
}
protected GeometricObject(String color, boolean filled) {
dateCreated = new java.util.Date();
this.color = color;
this.filled = filled;
}
public abstract double getArea();
public abstract double getPerimeter();
}
public class Rectangle extends GeometricObject {
public Rectangle() {
// super();
}
public Rectangle(double width, double height) {
this();
this.width = width;
this.height = height;
}
public double getArea() {
return width * height;
}
public double getPerimeter() {
return 2 * (width + height);
}
}
1.2 An abstract method can only be contained in an abstract class.
1.3 Subclasses of abstract classes :
①In a nonabstract (a.k.a., concrete) subclass extended from an abstract super-class, all the abstract methods MUST be implemented.
②In an abstract subclass extended from an abstract super-class, we can choose:
to implement the inherited abstract methods
OR to postpone the constraint to implement the abstract methods to its nonabstract subclasses.
abstract class A{
abstract void m();
}
abstract class B extends A{
}
public class C extends B {
void m(){
}
}
1.4 A subclass can be abstract even if its superclass is concrete.
1.5 A subclass can override a method from its concrete superclass to define it abstract
① useful when we want to force its subclasses to implement that method。
② the implementation of the method in the superclass is invalid in the subclass
子类的抽象与父类无关,可以强制让子类或子类方法抽象
1.6 It is possible to define an abstract class that contains no abstract methods. ==>This class is used as a base class for defining new subclasses.
1.7 An object cannot be created from abstract class抽象类无法new出实例,但是我们依旧会写构造函数。因为可以让子类的构造函数通过constructor chaining去调用抽象类的构造函数super()
An abstract class can be used as a data type: GeometricObject c = new Circle(2);
eg : 构造一个数组 GeometricObject[] geo = new GeometricObject[10];数组中每一个元素初始都是null,直到被具体对象初始化geo[0] = new Circle(); ......
1.8 The abstract Calendar class and its GregorianCalendar subclass
① java.util.Date 实例可以表示毫秒精度的特定瞬间
② java.util.Calendar是抽象基类,可以从Data对象里提取年月日小时等等
③ java.util.GregorianCalendar是Calendar的现代公历子类
1.9 GregorianCalendar构造 :
① new GregorianCalendar() 构建当前时间
② new GregorianCalendar(year, month, date) 月比较特殊,1-12月对应0-11的参数
Calendar 方法 :
① get(int field) :从calendar对象里提取具体的year,month,date,minute...
2. Interfaces
2.1 An interface is a class-like construct that contains only abstract methods and constants.
UML图中对象虚线和三角形去连接接口,接口和方法都是斜体。
2.2 接口目的是specify behavior for objects,allows multiple inheritance
2.3 Define an Interface :
public interface InterfaceName { // constant declarations; // method signatures; }
public interface Edible {
public abstract String howToEat();
}
class Chicken extends Animal implements Edible {
public String howToEat() {
return "Chicken: Fry it";
} }
2.4 Omitting Modifiers in Interfaces :
① 在接口中所有数据字段都是public static final
② 在接口中所有方法都是public abstract
注:使用InterfaceName.dataName 去访问数据字段
2.5 Each interface is compiled into a separate bytecode file just like a regular class.
接口使用和抽象类一样,要么是 a data type for a variable 要么是as the result of casting
2.6 The Comparable Interface :java里string,data等类实现了该接口,以定义对象的自然顺序,这些对象都是instanceof comparable。方法是compareTo(Obj),经过compareTo返回的是Comparable类型的数据,所以需要cast it to String or the other explicitly
2.7 The Cloneable Interface :
① Marker Interface: is an empty interface (does not contain constants or methods)
② 实现该接口的对象可以用clone()去克隆自己,返回的是Object类型的对象,所以需要自己去cast。 我们也可以在类里override this method去自定义
Calendar calendarCopy = (Calendar)(calendar.clone());
public Object clone() {
try {
return super.clone();
}catch (CloneNotSupportedException ex) {
return null;
}
}
eg:calendar 克隆了一个calendarCopy。calendar != calendarCopy,因为引用不同
calendar.equals(calendarCopy) is true,因为比较的是内容
③ Shallow Copy 如果字段属于引用类型,则复制对象的引用而不是其内容,导致共享一个引用指向的内容。
④ Deep copying 是通过构建自定义clone()来不仅复制对象本身,还会手动去递归地复制对象所引用的所有内容,包括引用对象的内容,以确保拷贝后的对象和原对象完全独立,不共享引用对象。例如这里就是手动复制建造日期
public Object clone() { // deep copy
try {
House h = (House)(super.clone());
h.whenBuilt = (Date)(whenBuilt.clone());
return h;
}catch (CloneNotSupportedException ex) {
return null;
}
}
3. 接口,抽象类,类比较
3.1 接口里数据字段必须是常量,抽象类里则可能是可变数据字段
3.2 接口无构造函数,抽象类有
3.3 接口方法只有signature没有实现,抽象类里可以有具体方法
3.4 继承里接口可以拓展任意数量的接口,接口没有根,一个类可以实现任意数量的接口。
但是有可能Conflicting interfaces,因为两个接口的常量不同值,或者方法有相同的signature却返回数据类型不一致。
3.5 使用规范
① Strong is-a:比如父母与儿女,Should be modeled using class inheritance
② Weak is-a (or is-kind-of):比如物体有质量,Should be modeled using interfaces
③ 当有类继承限制时,可以用多接口继承
4.Wrapper Classes
4.1 Primitive data types in Java ➔ Better performance
4.2 Each primitive type has a wrapper class.
The wrapper classes do not have no-arg constructors. The instances of all wrapper classes are immutable
4.3 Each wrapper class overrides the toString and equals methods defined in the Object class.因为这些类都实现了Comparable,和Comparable的compareTo方法
4.4 The Number Class :Each numeric wrapper class extends the abstract Number class
① 方法doubleValue, floatValue, intValue, longValue(都是抽象方法), shortValue, and byteValue
② byteValue and shortValue are not abstract, which simply return (byte)intValue() and (short)intValue(), respectively。剩下的抽象方法都在数字包装类里被实现了
4.5 ① 可以用原始数据类型或者数值字符串去构造数字包装对象
② 每个数字包装类都有常量MAX_VALUE and MIN_VALUE
③ 每个数字包装类都有静态方法valueOf(String s),并且也有overloaded parsing methods
eg: Double doubleObject = Double.valueOf("12.4");
double d = Double.parseDouble("12.4");
④ 原始类型和包装自动转换
5. Arrays ,BigInteger and BigDecimal
5.1 数组是对象类的实例
If A is a subclass of B, every instance of A[] is an instance of B[]
eg : new Calendar[10] instanceof Object[] ===> true
int值可以赋值给double,但是int[] and double[]是不兼容的,不能把int[]赋值给double[],因为这俩就不是类。
5.2 Java用Comparable接口提供static sort方法去对对象数组排序
5.3 BigInteger can represent an integer of any size
BigDecimal has no limit for the precision (as long as it’s finite=terminates)
Both are immutable,extend the Number class and implement the Comparable interface.
Lec4 Generics
1.1 warning :
compiling errors better than runtime errors
看到了课件例子,顺便写一下int≠Integer,一个是数据类型一个是对象,一个可直接赋值初始化一个要么构造要么初始化赋值,一个在栈内存一个在堆内存,一个只是类型一个能调用方法。Integer.valueOf()返回的是基本数据类型 int ; Integer.parseInt()返回的是一个 Integer 对象;不管是int还是Integer都不是str能强转的。
1.2 Generics
1.2.1Generics是什么
Generics is the capability to parameterize types. 具体来说你可以定义一个使用泛型类型的类或方法,然后由编译器使用具体类型进行替换。举个例子,你可以定义一个泛型的栈类,用来存储泛型类型的元素。然后,你可以使用这个泛型类创建:
- 一个用于存储字符串的栈对象
- 一个用于存储数字的栈对象
在这个泛型类中,字符串和数字是具体的类型,它们会替换泛型类型的位置。
1.2.2 Generics好处
① The key benefit of generics is to enable errors to be detected at compile time rather than at runtime(泛型类/方法只需要一次实现就可以来处理不同类型的数据,实现了代码的重用)
② Most important advantage: If you attempt to use the class or method with an incompatible object, a compile error occurs
1.2.3 Generic Types
下面的代码里 <T>represents a formal generic type, which can be replaced later with an actual concrete type This is called Generic Instantiation
package java.lang;
public interface Comparable<T> {
public int compareTo(T o)
}
Comparable<Date> c = new Date();
System.out.println(c.compareTo("red"));//Compiler error
//声明了该泛型可以接受 Date 类型的对象,但在调用 compareTo 方法时却传递了一个字符串 "red",这与声明的数据类型不符。
1.2.4 Generic ArrayList Method
java.util.ArrayList:①add(o: E) : void
②get(index: int) : E
③set(index: int, o: E) : E
No Casting Needed,避免了casting
ArrayList<Double> list = new ArrayList<Double>();
list.add(5.5); // 5.5 is automatically boxed/
// converted to new Double(5.5)
list.add(3.0); // 3.0 is automatically boxed/
// converted to new Double(3.0)
Double doubleObject = list.get(0);
// No casting is needed
注:generic types must be reference types rather than a primitive type such as int, double, or char!<Integer>对 <int>错。但是可以往ArrayList<Integer>里加int,java会帮你自动封装
1.2.5 Generic Classes
① Declaring Generic Classes
public class GenericStack<E> {
private java.util.ArrayList<E> list = new java.util.ArrayList<E>();
public E peek() {
return list.get(getSize() - 1);
}
@Override // Java annotation: also used at compile time to
public String toString() { // detect override errors
return "stack: " + list.toString();
}
}
② Using Generic Classes
public static void main(String[] args){
GenericStack<Integer> s1;
s1 = new GenericStack<>();
s1.push(1);}
1.2.6 Generic Static Methods
To declare a generic method, you place the generic type immediately after the keyword static in the header
public static <E> void print(E[] list) {...}
GenericMethods1.<String>print(s3);
// OR simply:
print(s3);
1.2.7 Bounded Generic Types
A generic type can be specified as a subtype of another type
Circle and Rectangle extend GeometricObject,只能使用GeometricObject的子类
public static <E extends GeometricObject> boolean equalArea(E object1, E object2) {
return object1.getArea() == object2.getArea();
}
Rectangle rectangle = new Rectangle(2, 2);
Circle circle = new Circle(2);
equalArea(rectangle, circle));
1.3 Sorting an Array of Objects
public static <E extends Comparable<E>> void
genericSelectionSort(E[] list) {
E currentMin;}
GenericSelectionSort.<Integer>genericSelectionSort(intArray);
The generic type <E extends Comparable<E>> has two meanings:
First, it specifies that E must be a subtype of Comparable
Second, it specifies that the elements to be compared are of the E type as well
1.4 Raw Type and Backward Compatibility
1.4.1 Raw Type and Backward Compatibility是什么
A generic class or interface used without specifying a concrete type, called a raw type, enables backward compatibility with earlier versions of Java.让旧版本代码可以在新版本上运行,则新版本有向后兼容性
eg : ArrayList list = new ArrayList() ≈ ArrayList<Object> list = new ArrayList<Object>();
1.4.2 Raw Type is Unsafe, how to make it safe
public static Comparable max1(Comparable o1, Comparable o2) {
if (o1.compareTo(o2) > 0)
return o1;
else
return o2;
}
max1("Welcome", 23);
上面是错误代码No compilation error. But Runtime Error!
public static <E extends Comparable<E>> E max2(E o1, E o2) {...}
max2("Welcome", 23);
此时因为可以声明泛型接受的对象类型,所以是Compiler Error
1.5 Wildcards
1.5.1 Integer is a subclass of Number, but GenericStack<Integer> is not a subclass of GenericStack<Number>
1.5.2 You can use unbounded wildcards, bounded wildcards, or lower-bound wildcards to specify a range for a generic type: 先看例子好理解
① ? :unbounded wildcard: any class
② ? extends T : bounded wildcard: any subclass of T (including T)
③ ? super T :lower bound wildcard: any superclass of T (including T)
eg: <? extends Number>is a wildcard type that represents Number or a subtype of Number . So GenericStack<Integer> is a subclass of GenericStack<? extends Number>
public static <T> void add(GenericStack<? extends T> stack1, GenericStack<T> stack2) {...}
GenericStack<String> stack1 = new GenericStack<String>();
GenericStack<Object> stack2 = new GenericStack<Object>();
add(stack1, stack2);//stack1可以是声明类型的子或者其本身,stack2只能是声明类型本身
1.6 Erasure and Restrictions on Generics
1.6.1 Erasure on Generics
① Generics are implemented in Java using an approach called type erasure:编译器在编译代码时会使用通用类型信息,之后会删除。因此运行时不会获得通用类型信息,并且一旦编译器认为可以安全使用泛型,它会把通用类型信息转为raw type Object(a--->b)
② 无论实例的参数类型如何,这些实例共享一个通用类。
eg:GenericStack<Integer> and GenericStack<String> are two types, but there is only one class GenericStack loaded into the JVM
System.out.println(stack1 instanceof GenericStack); True!
System.out.println(stack1 instanceof GenericStack<Integer>); Compile errors!!!
1.6.2 Restrictions on Generics
Because generic types are erased at runtime, there are certain restrictions:
① Cannot Create an Instance of a Generic Type (i.e., new E()) E is not available at runtime
② Generic Array Creation is Not Allowed (i.e. new E[100], E[] elements = new E[capacity];)
③ 由于静态方法、静态字段和类初始化器都属于类本身且在实例化之前就存在的,所有实例共享该类和类里方法,而泛型类型是在类实例化时确定的,所以不能直接在静态上下文中引用泛型类型参数。It is illegal to refer to a generic type parameter for a class in a static method, field, or initializer.
public class DataStructure<E> {
public static void m(E o1) { // Illegal
}
public static E o1; // Illegal
static {
E o2; // Illegal
}
}
④ Exception Classes Cannot be Generic. Because a generic class may not extend java.lang.Throwable
eg: public class MyException extends Exception { … }因为运行时通用类型信息不存在
1.7 Use Case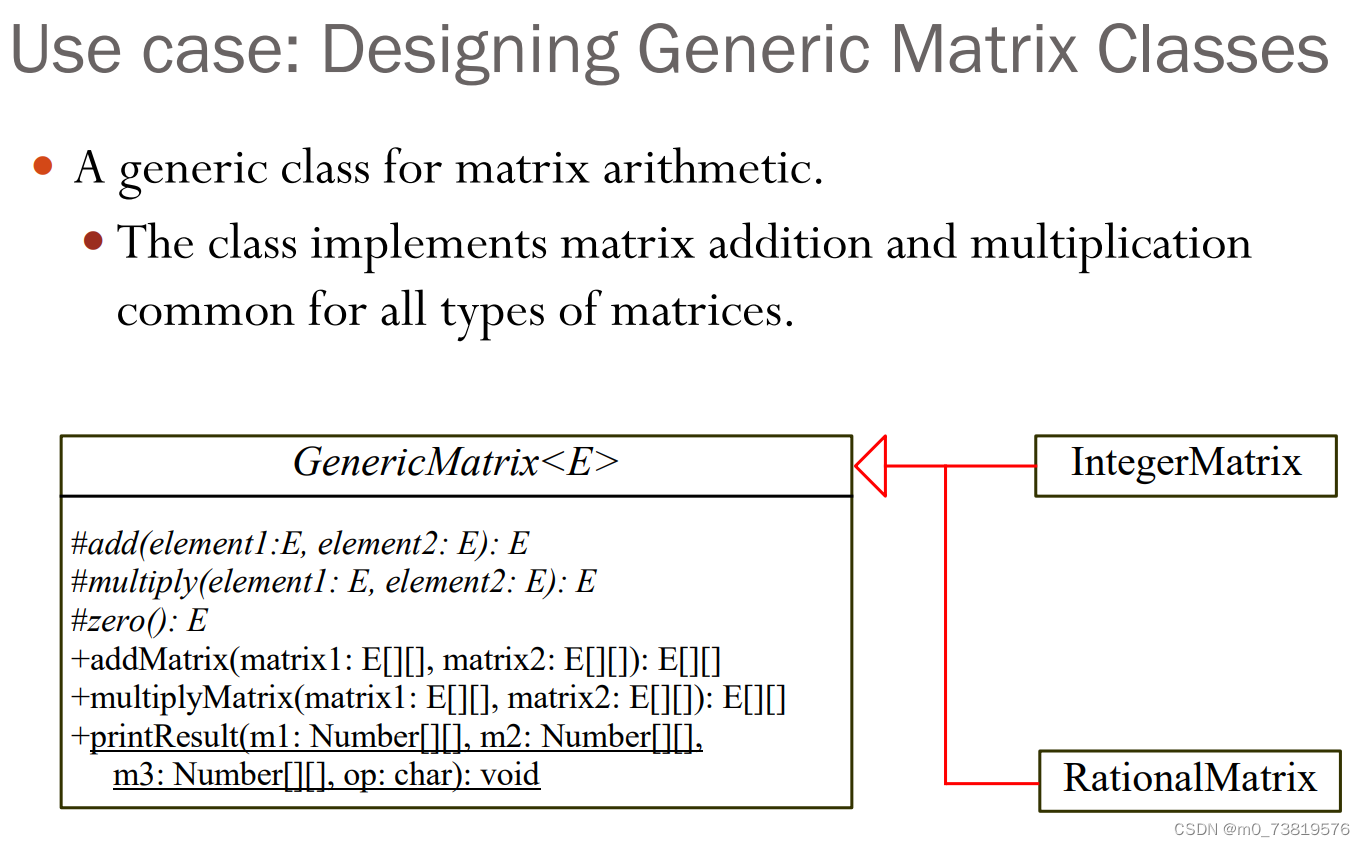
Lec7
1.Set
1.1 Basic
Set interfaceis a sub-interface of Collection
It extends the Collection, but does not introduce new methods or constants.
However, the Set interface stipulates that an instance of Set contains no duplicate elements So,no two elements e1and e2 can be in the set such that e1.equals(e2) is true
1.2 实例
类:HashSet, LinkedHashSet, or TreeSet
The concrete classes that implement Set must ensure that no duplicate elements can be added to the set :
①HastSet & LinkedHashSet use: hashcode() + equals()
②TreeSet use: compareTo() or Comparable
2.HashSet
2.1 Creation 无参空集
create an empty hash set using its no-arg const
Set<String> set = new HashSet<>();
①The first diamond operation ("<>") is called a type parameter or generic type.指定了HashSet要存储的元素类型
②The 2nd diamond operation ("<>"),the compiler infersthe generic type from the context
③The parentheses("()") is used for calling the constructor of the HashSet class.这里是无参构造,创造一个空集.
2.2 Creation from an existing collection
List<String> list = Arrays.asList("Apple");
HashSet<String> hashSet = new HashSet<>(list);
2.3 Creation with the specified initial capacity or initial capacity
int initialCapacity = 16;
float loadFactor = 0.75f;
HashSet<String> hashSet1 = new HashSet<>(initialCapacity);
HashSet<String> hashSet2 = new HashSet<>(initialCapacity, loadFactor);
Loadfactor rangesfrom 0.0 to 1.0, measuring how full the set is allowed to be before its capacity is increased (doubled; x2)
E.g., the capacity is 16 and load factor is 0.75, when the size reaches 12(16*0.75 = 12) the capacity will be doubled to 32 (16x2)
2.4 Method
因为实现或者拓展了接口,抽象类。So,all the declared methods(e.g., add(), remove(), etc) can be called in a set instance.
2.5 Method: add()
Adding elementsto set. But a hash set is unordered (because of hashing, W13)
A hash set is non-duplicated.A hash set use hashcode() and equals() to check duplication
2.6 迭代Enhanced for loop
Collection interface extends the Iterable interface , so the elements in a set are iterable
格式:for (declaration : expression) { // Statements}
for(String s: set) {
System.out.print(s.toUpperCase() + " "); }
①Declaration: the part where you declare a variable that will hold an element of the array or collection you're iterating over
②Expression: the collection or array you want to iterate over; the target
③Enhanced for loop is used because a hash set is unordered without index (No [i])
2.7 迭代forEach()
①A default method in the Iterable interface
②格式:Set.forEach(e -> System.out.print())
Set.forEach(e -> System.out.print(e.toUpperCase() + " "))
③e is the parameter passed to the lambda expression. It represents the current element of the set
④-> is the lambda arrow which separates the parameters of the lambda expression from its body
2.8 Other Common Method
remove(); size();contains();addAll();removeAll();
3. LinkedHashSet
3.1 Basic
LinkedHashSet extends HashSet with a linked list implementation that supports an ordering of the elements in the set .所以上面的创建与方法也适用于LinkedHashSet
Significant Difference: The elements in a LinkedHashSet can be retrieved in the order in which they were inserted into the set
Set<String> set = new LinkedHashSet<>();
set.add("London");
System.out.printin(set);
for (String element: set)
System.out.print(element.toLowerCase() + "");
4. TreeSet
4.1 Basic
①TreeSet is a concrete class that implements the SortedSet and NavigableSet interfaces ②SortedSet is asub-interface of Set. The elements in the set are sorted ③NavigableSet extends SortedSet to provide navigation methods : lower(e), floor(e),...
4.2 Creation
①Empty tree set without arguement, which will be sorted in ascending order according to the natural ordering of its element.无参构造,元素自然升序排序
TreeSet<String> treeSet = new TreeSet<>();
②Tree set with other collections, being sorted by the natural ordering.用其他集合构造
TreeSet treeSet = new TreeSet<>(list);
③Tree set with customized comparator,where we can define the orders自定义的Comparator来定义元素的顺序
TreeSet treeSet = new TreeSet<>(Comparator.reverseOrder());
④Tree set with the same elements and the same ordering asthe specified sorted set构造相同TreeSet
// If we already have a setsorted according to a specific rule
SortedSet originalSet = new TreeSet<>(String.CASE_INSENSITIVE_ORDER);
// We take this way to create another tree set with same elements and same ordering
TreeSet copiedSet = new TreeSet<>(originalSet);
4.3 add()
与HashSet相同,无duplicted element
Instead of hashcode() and equals(), this is because the “built-in” compareTo() in String and Integer and other wrapper classesin Java's standard library. The difference is due to the bottomed data structure
4.4 Common Method in SortedSet
first();last();
headSet(); 查找小于等于给定toElement的元素
tailSet();查找大于等于给定toElement的元素
4.5 Common Method in Navigable
lower();严格小于给定元素的最大元素
higher();严格大于给定元素的最小元素
floor();小于等于给定元素的最大元素
ceiling();大于等于给定元素的最小元素
pollFirst();读取并删除第一个元素
pollLast();读取并删除最后一个元素
5. Performance of Sets and Lists
①Sets are more efficient than lists for storing nonduplicate elements
②Lists are useful for accessing elements through the index
③Sets do not support indexing because the elements in a set are unordered
6. Map
6.1 Basics
A map is a container object thatstores a collection of key/value pairs.
It enablesfast retrieval, deletion, and updating of the pair through the key. A map storesthe values along with the keys.
The keys can be any objects.A map can't contain duplicate keys. Each key maps to one value.键值对,靠键索引,键不能重复,一键一值
6.2 Three Types
HashMap, LinkedHashMap, and TreeMap.
三种map确保键非重复:hashcode() and equals() (for HashMap and LinkedHashMap) as well asthe compareTo()/Comparator (for TreeMap).
三种map都是map接口的具体实现,并且TreeMap additionally implements SortedMap and NavigableMa
6.3 Creation
①格式:Map<? extends K, ? extends V>
②构造函数使用smallmap :Map<X,Y>其中X,Y是K,V的子类
Map<String, Integer> smallmap = new HashMap<>();
Map<Object, Number> largerMap = new HashMap<>(smallMap);
③X=K,Y=V时②也适用
Map<Integer, String> smallMap = new HashMap<>();
Map<Integer, String> largerMap = new HashMap<>(smallMap);
6.4 LinkedHashMap
①与LinkedHashSet相似,it extends HashMap with a linked-list implementation that supports retreiving elementsin the insertion order
②带参构造(initialCapacity: int, loadFactor: float, accessOrder: boolean) initialCapacity和loadFactor都和LinkedHashSet里性质一样。accessOrder是true时retreive elements in the order in which they were last accessed, from least recently to most recently accessed (access order).
Map<Integer, String> smallMap = new HashMap<>(16,0.75f,true);
6.5 Method
①get(): Returns the value to which the specified key is mapped
②forEach():Performs the given action for each entry in this map until all entries have been processed or the action throws an exception.
void forEach(BiConsumer<? super K, ? super V> -> action)不返回,只执行action
Map<String, Integer> hashmap = new HashMap<>();
Hap<String, Integer> treemap = new TreeMap<>(hashMap);
treemap.forEach()
(name,age) -> System.out.println(name + "" + age + "");)
name和age是声明参数String和Integer的名称
Lecture8 Developing Efficient Algorithms
1. Algorithms(定义都是int202和上学期学过的玩意,可看可不看)
1.1 definition
An algorithm is a finite sequence of well-defined, computer-implementable instructions, typically to solve a class of problems or to perform a computation.
1.2 Algorithm analysis
It is the process of finding the computational complexity of algorithms, that is: the execution time and the storage growth rate, or other resources needed to execute them.
1.3 Big O Notation
a "theoretical" approach was developed to analyze algorithms independent of specific inputs and computers (i.e., system load or hardware: CPU speed, memory, multiple cores, etc.)
This approach approximates the effect of a change on the size of the input in the execution time, i.e., the Growth Rate
2. Big O Notation
2.1 线性搜索
If the key is not in the array, it requires n comparisons ;If the key is in the array, it requires "on average" n/2 comparisons.
This algorithm grows at a linear rate . The growth rate has an order of magnitude growth rate of n. So the complexity of the linear search algorithm is O(n), pronounced as “order of n”
2.2 Best, Worst, Average Cases
①An input that results in the shortest execution time is called the best-case input
②An input that results in the longest execution time is called the worst-case input
③An average-case analysis attempts to determine the average amount of time among all possible inputs of the same size
平均是最理想的,最坏最容易获得且普遍使用
2.3 两个忽略
①Ignoring Multiplicative Constants :eg 3n => O(n)
②Ignoring Non-Dominating Terms :eg n-1 => O(n)
2.4 Input size and constant time
如果输入大小与运行时间无关,改算法称为constant time,O(1)
2.5 Useful Mathematic Summations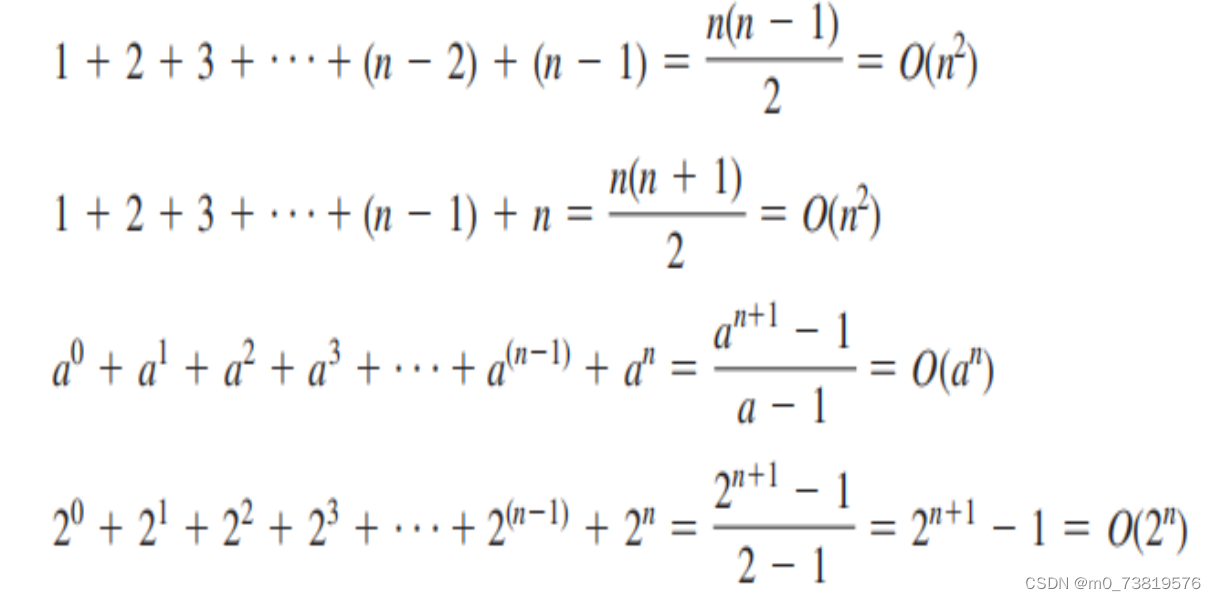
3. Determining Big-O : Repetition
3.1 Simple Loop
对k = k + 5循环n次的Time Complexity : T(n) = (a constant c) * n = cn = O(n)
3.2 Nested Loops
①T(n) = (a constant c) * n * n = c = O(
)
for (i = 1; i <= n; i++) {
for (j = 1; j <= n; j++) {
k = k + i + j; }}
②T(n) = c + 2c + 3c + 4c + … + nc = cn(n+1)/2 = (c/2) + (c/2)n = O(
)
for (i = 1; i <= n; i++) {
for (j = 1; j <= i; j++) {
k = k + i + j; }}
③T(n) = 20 * c * n = O(n)
for (i = 1; i <= n; i++) {
for (j = 1; j <= 20; j++) {
k = k + i + j; }}
4. Determining Big-O : Sequence
取增长率更大的,忽略小的
T(n) = c *10 + 20 * c * n = O(n)
for (j = 1; j <= 10; j++) {
k = k + 4; }
for (i = 1; i <= n; i++) {
for (j = 1; j <= 20; j++) {
k = k + i + j; }}
5. Determining Big-O : Selection
T(n) = test time + worst-case (if, else) = O(n) + O(n) = O(n)
if (list.contains(e))
System.out.println(e);
else
for (Object t: list)
System.out.println(t);
6. Determining Big-O : Logarithmic time
result = 1;
for (int i = 1; i <= n; i++) result *= a;
原为O(n), 令n = k = log2 n,此时result可以视为n个a相乘,那我们让两个a在一次运行时相乘
result = a;
for (int i = 1; i <= k; i++) result = result * result;
优化后T(n) = k = log n = O(log n)
7. Binary Search
An algorithm with the O(log n) time complexity is called a logarithmic algorithm and it exhibits a logarithmic growth rate
The base of the log is 2, but the base does not affect a logarithmic growth rate, so it can be omitted
输入每变大一倍,比较数多一; 输入数平方,比较数乘二
8. Selection Sort
8.1 定义
Selection sort finds the smallest element in the list and swaps it with the first element . It then finds the smallest element remaining and swaps it with the first element in the remaining list, and so on until the remaining list contains only one element left to be sorted.
8.2 复杂度
T(n)= (n-1) + (n-2) +...+ 1 + c(n-1) = + n/2 = O(
)
9. Quadratic Time
9.1 Definition
An algorithm with the O() time complexity is called a quadratic algorithm
9.2 Insertion Sort
①Definition:The insertion sort algorithm sorts a list of values by repeatedly inserting an unsorted element into a sorted sublist until the whole list is sorted.
②复杂度计算:To insert the first element, we need 1 comparison and at most 1 swap To insert the last element, we need n−1 comparisons and at most n−1 swaps . c denotes the total number of other operations such as assignments and additional comparisons in each iteration
10. Exponential complexity
10.1 Towers of Hanoi
10.2 复杂度
An algorithm with O() time complexity is called an exponential algorithm, and it exhibits an exponential growth rate.
10.3 Recursive Fibonacci Numbers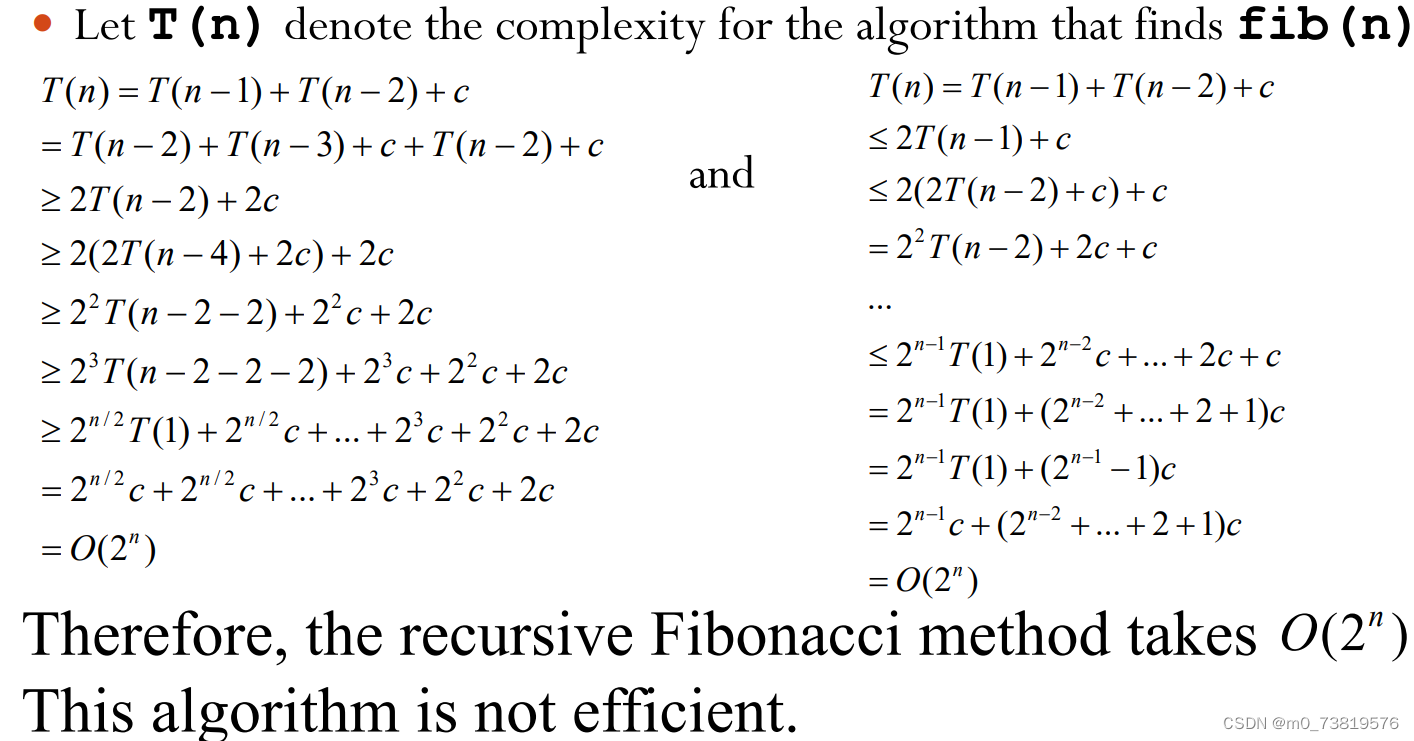
每次递归调用自身,所以计算得出复杂度是2^n; 非递归的简化版可以让复杂度为O(n)
if (n == 0)
return 0;
else if (n == 1 || n == 2)
return 1;
int f0 = 0; // For fib(0)
int f1 = 1; // For fib(1)
int f2 = 1; // For fib(2)
for (int i = 3;i <= n;i++){
f0 = f1;
f1 = f2;
f2 = f0 + f1; }
11. Common Recurrence Relations And Growth Functions
11.1 Recurrence Relations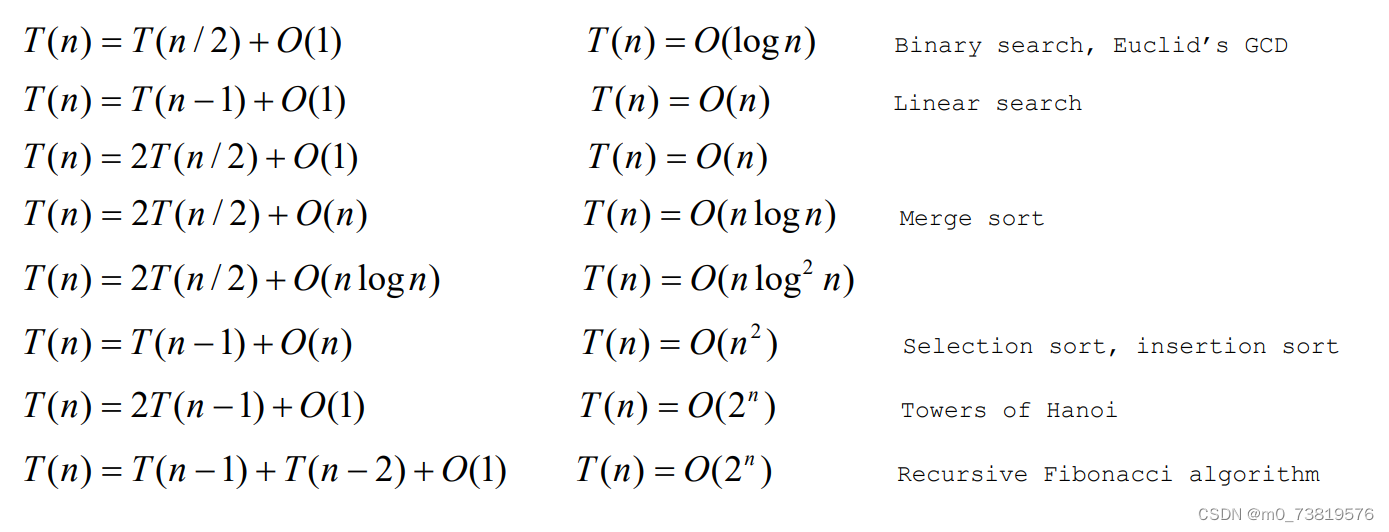
11.2 Comparing Growth Functions
12. Algorithm Techniques
12.1 Definition
Techniques for designing and implementing algorithm designs are called algorithm design patterns
eg:
①Brute-force or exhaustive search
②Divide and conquer:一个实例分为多个小实例,小实例的解和为问题的解
③Dynamic programming:将小问题的结果暂存,并可以被其他问题使用,避免重复计算
④Greedy algorithms:局部最优解
⑤Backtracking:逐步建立多个解决方案,并在确定无法有效解决问题时放弃它们
12.2 Dynamic programming
非递归的斐波那契额就是一个例子,因为subproblems overlap所以不能只使用递归
The key idea behind dynamic programming is to solve each subprogram only once and store the results for subproblems for later use
12.3 Divide-and-Conquer
与动态编程不同,分而治之的subproblems don’t overlap.
找距离最近的两点:
①按照x递增顺序排序,x相同的按照y递增排序,得到列表x。
②找到列表x中间的点mid,将列表分为大小相同的两个S1和S2(mid在S1),递归找出S1和S2的分别最小距离d1,d2。令d = min(d1,d2)
③找到S1与S2的各自一点在[mid' x – d, mid' x + d]的范围里最近的,记为d3。比较d3与d,找到距离最小的一对点。 伪代码:
④复杂度分析:① sorting为O(n log n) ,因为Merge Sort对点排序
② 为2T(n/2),因为通过递归二分的方式,每轮操作将列表分成两半,因此需要 O(log n) 轮,而每一轮的操作时间复杂度是 O(n)。
③ 为O(n),因为在有序列表中查找范围内的点所需的操作是线性的。
总复杂度是T(n) = O(n log n)+2T(n/2) + O(n) = O(n logn)
12.4 Backtracking: Eight Queens
棋盘每行有一个皇后,每个皇后不能相互攻击到,使用递归解决
Assign j to queens[i] to denote that a queen is placed in row i and column j
从第一行开始搜索, k = 0 ,其中 k 是当前行的索引。算法会按该皇后的可能攻击路线去检查 j = 0、1、......、7 行的第 j 列是否可能有皇后。如果成功,则进行下一行,直到最后一行皇后也被找到;如果不成功,则backtracks上一行,并在上一行的下一列中找到新的位置;如果在第一行仍不成功,则该问题无解
Lecture9 Sorting
1. Bubble Sort
1.1定义
重复遍历列表,比较列表每对元素,如果顺序有误,则调换
The pass through the list is repeated until no swaps are needed, which indicates that the list is sorted. If no swap takes place in a pass, all the elements are already sorted.
1.2代码
boolean needNextPass = true;
for (int k = 1; k < list.length && needNextPass; k++) {
// Array may be sorted and next pass not needed
needNextPass = false;
// Perform the kth pass
for (int i = 0; i < list.length - k; i++)
if (list[i] > list[i + 1]) {
// swap list[i] with list[i + 1];
needNextPass = true; // Next pass still needed
}}
1.3时间复杂度
①Best case :algorithm needs just the first pass to find that the array is already sorted. Since the number of comparisons is n - 1 in the first pass, the best case time for a bubble sort is O(n).
②Time complexity (i.e., Worse case) :( n-1)+ (n-2)+...+1 --->O( )
2. Merge Sort
2.1 定义
Merge sort is a divide and conquer algorithm.
将未排序的列表分为n个,每个子列表有一个元素(也被视为已排序)。重复合并这些子列表,直到剩下一个最终列表
2.2 代码
public static void mergeSort(int[] list) {
if (list.length > 1) {
// Merge sort the first half
int[] firstHalf = new int[list.length / 2];
System.arraycopy(list,0,firstHalf,0,list.length / 2);
mergeSort(firstHalf);
// Merge sort the second half
int secondHalfLength = list.length - list.length / 2;
int[] secondHalf = new int[secondHalfLength];
System.arraycopy(list,list.length / 2,secondHalf,0,secondHalfLength);
mergeSort(secondHalf);
// Merge firstHalf with secondHalf into list
merge(firstHalf, secondHalf, list);
}}
public static void merge(int[] list1, int[] list2, int[] temp){
int current1 = 0; // Current index in list1
int current2 = 0; // Current index in list2
int current3 = 0; // Current index in temp
while (current1 < list1.length && current2 < list2.length) {
if (list1[current1] < list2[current2])
temp[current3++] = list1[current1++];
else
temp[current3++] = list2[current2++];
}
while (current1 < list1.length)temp[current3++] = list1[current1++];
while (current2 < list2.length)temp[current3++] = list2[current2++];
}
2.3 时间复杂度
T (n) = T(n/2) + T(n/2)+ mergetime
根据代码,每次合并列表时需要比较n-1次,和n次移动将元素放入temp里
所以T (n) = 2T(n/2) + 2n-1 = 2nlogn +1 = O(nlogn)
3. Quick Sort
3.1 定义
在数组中选择一个元素,称为中枢pivot,让数组分为两部分。前半部分都小于等于中枢,后半部分大于中枢。递归的先对前半部分使用快速排序算法,再对后半部分使用,最后两个部分和中枢合并为最终列表。
3.2 代码
public static void quickSort(int[] list) {
quickSort(list, 0, list.length - 1);}
public static void quickSort(int[] list, int first, int last) {
if (last > first) {
int pivotIndex = partition(list, first, last);
quickSort(list, first, pivotIndex - 1);
quickSort(list, pivotIndex + 1, last);
}}
public static int partition(int[] list, int first, int last) {
int pivot = list[first]; // Choose the first element as pivot
int low = first + 1; // Index for forward search
int high = last; // Index for backward search
while (high > low) {
// Search forward from left
while (low <= high && list[low] <= pivot)
low++;
// Search backward from right
while (low <= high && list[high] > pivot)
high--;
// Swap two elements in the list
if (high > low) {
int temp = list[high];
list[high] = list[low];
list[low] = temp;
}
}
// Account for duplicated elements:
while (high > first && list[high] >= pivot)
high--;
// Swap pivot with list[high]
if (pivot > list[high]) {
list[first] = list[high];
list[high] = pivot;
return high;
} else return first;
}
3.3 时间复杂度
① Worst Case: To partition an array of n elements, it takes n-1 comparisons and n moves in the worst case. So, the time required for partition is O(n)
In the worst case, each time the pivot divides the array into one big subarray with the other empty. 所以比上一次大小减一,因此
(n-1) + (n-2) +... +1 = O(n^2)
② Best-Case Time Complexity: 每次中枢把数组平分成大小相同的两个
T (n) = T(n/2) + T(n/2) + n = O(nlogn)
③ Average-Case Time : the sizes of the two parts are very close => the average time is also O(n log n)
3.4 与merge比较
都采用分而治之,merge的大部分时间用于合并,从最坏结果来看merge优于quick,平均情况下两者差别不大
但是merge需要临时数组存储两个子列表,quick不需要额外空间,quick节省空间
4. Heap Sort: Binary tree
4.1 Complete Binary Tree
A binary tree is complete if every level of the tree is full except that the last level may not be full and all the leaves on the last level are placed left-most.
4.2 Binary Heap
A binary heap is a binary tree with the following properties:
It is a complete binary tree, and each node is greater than or equal to any of its children
4.3 Heap Sort
Heap sort uses a binary heap: it first adds all the elements to a heap and then removes the largest elements successively to obtain a sorted list.
4.4 Storing a Heap
A heap can be stored in an ArrayList or an array if the heap size is known in advance
For a node at position i, its left child is at position 2i+1 and its right child is at position 2i+2, and its parent is at index (i-1)/2 (integer division)向下取整
4.4.1 Adding Elements to the Heap
To add a new node to a heap, first add it to the end of the heap and then rebuild the tree with this algorithm:假设新添加的节点为当前节点(current node),当前节点的值大于其父节点的值时,交换当前节点和其父节点的值,然后当前节点上移至其父节点的位置。重复以上步骤,直到当前节点的值不再大于其父节点的值,或者当前节点已经成为根节点(没有父节点)
4.4.2 Removing the Root and Rebuild the Heap
根元素最大,最先移除根元素,移除后需要重建树并保持堆属性:
让最后一个节点取代根节点,选根节点为当前节点(current node)。当前节点有子节点并且值小于其子节点的值时,交换当前节点和其子节点的值,然后当前节点下移至其子节点的位置。重复以上步骤,直到当前节点的值不再小于其子节点的值。
4.5 The Heap Class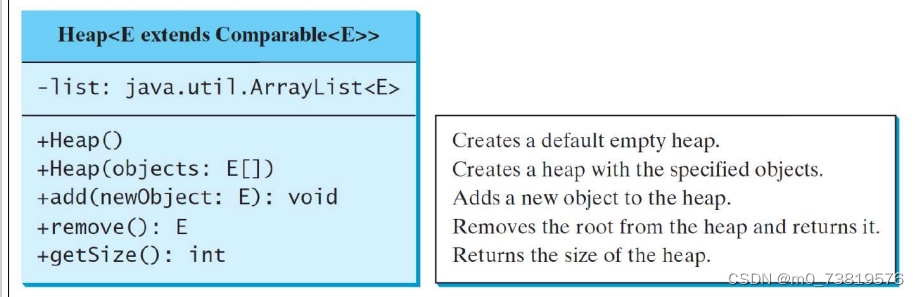
public E remove() {
if (list.size() == 0) return null;
E removedObject = list.get(0);
list.set(0, list.get(list.size() - 1));
list.remove(list.size() - 1);
int currentIndex = 0;
while (currentIndex < list.size()) {
int leftChildIndex = 2 * currentIndex + 1;
int rightChildIndex = 2 * currentIndex + 2;
// Find the maximum between two children
if (leftChildIndex >= list.size())
break; // The tree is a heap
int maxIndex = leftChildIndex;
if (rightChildIndex < list.size())
if (list.get(maxIndex).compareTo(list.get(rightChildIndex)) < 0)
maxIndex = rightChildIndex;
// Swap if the current node is less than the maximum
if (list.get(currentIndex).compareTo(list.get(maxIndex)) < 0) {
E temp = list.get(maxIndex);
list.set(maxIndex, list.get(currentIndex));
list.set(currentIndex, temp);
currentIndex = maxIndex;
}
else
break; // The tree is a heap}
return removedObject;
}
public int getSize() {
return list.size();
}}
4.6 时间复杂度
①令h表示含有n个元素的堆的高度,因为堆都是完全二叉树,所以第k层有2^(k-1)个节点,最后一层h有最少一个,最多2^(h-1)个节点。因此总节点个数n为
1+2+...+2^(h-2)<n<=1+2+...+2^(h-2)+2^(h-1)
2^(h-1)<n<=2^h-1
h-1<log(n+1)<=h
Thus, log(n + 1) ≤ h < log(n + 1) + 1,堆高为O(logn)
②添加方法追踪的是从叶子到根的路径,因此添加一个新元素最多需要h=logn步,添加n个元素的数组总共需要O(nlogn)
③删除方法同理追踪的是从根到叶子的路径,删除一个最多需要h=logn步,删除一整个堆需要O(nlogn)
④Heap Sort Time: O(n log n)
4.7 与merge比较
不需要额外空间,heap sort更省空间
5. Insertion Sort
5.1 定义
sorts a list of values by repeatedly inserting an unsorted element into a sorted sublist until the whole list is sorted. eg:在已排序的2,5,9中插入未排序的4
5.2 代码
public static void insertionSort(int[] list) {
for (int i = 1; i < list.length; i++) {
int currentElement = list[i];
int k;
for (k = i - 1; k >= 0 && list[k] > currentElement; k--)
list[k + 1] = list[k];
// Insert the current element into list[k + 1]
list[k + 1] = currentElement;
}}
5.3 时间复杂度
To insert the first element, we need 1 comparison and at most 1 swap. To insert the last element, we need n−1 comparisons and at most n−1 swaps . c denotes the total number of other operations such as assignments and additional comparisons in each iteration.
T(n) = (2+c) +(2x2+c)+...+(2x(n-1)+c) = 2(1+2+...+(n-2))+c(n-1)
=n^2-n+cn-c = O(n^2)
Lec10 Graphs and Applications
1. 图术语
vertices, edges, directed/ undirected, weighted/unweighted, connected graphs, loops, parallel edges, simple graphs, cycles, subgraphs and spanning tree
2. Representing
2.1 Representing Vertices
String[],List<String>,
In all these representations the vertices can be conveniently labeled using the indexes 0, 1, 2, …, n-1, for a graph for n vertices.
2.2 Representing Edges:
①Edge Array,eg:int[][] edges = {...}
②Edge Objects,eg:
public class Edge {
int u, v;
public Edge(int u, int v) {
this.u = u;
this.v = v;
}…
}
List<Edge> list = new ArrayList();
③Adjacency Matrix, use dimensional N * N matrix,eg:int[][] adjacencyMatrix = {...}
④Adjacency Vertex List, 表每点的邻点eg:List[] neighbors = new List[12]; OR
List> neighbors = new ArrayList();
⑤Adjacency Edge List,eg:List[] neighbors = new List[12]; Edge(0, 1);List<ArrayList<Edge>> neighbors = new ArrayList();
2.2 Modeling Graphs
The Graph interface defines the common operations for a graph
An abstract class named AbstractGraph that partially implements the Graph interface
Two concrete class WeightedGraph,UnweightedGraph
3. Graph Traversals
3.1 定义
Graph traversal is the process of visiting each vertex in the graph exactly once.
There are two popular ways: depth-first search, breadth-first traversal
3.2 Depth-First Search
①The depth-first search of a graph starts from a vertex in the graph and visits all vertices in the graph as far as possible before backtracking
public Tree dfs(int v) {
int[] parent = new int[vertices.size()];
for (int i = 0; i < parent.length; i++)
parent[i] = -1; // Initialize parent[i] to -1
// Mark visited vertices (default false)
boolean[] isVisited = new boolean[vertices.size()];
List<Integer> searchOrder = new ArrayList();
// Recursively search
dfs(v, parent, isVisited, searchOrder);
// Return the search tree
return new Tree(v, parent, searchOrder);
}
/** Recursive method for DFS search */
private void dfs(int u, int[] parent, boolean[] isVisited,List<Integer> searchOrder){
// Store the visited vertex
searchOrder.add(u);
isVisited[u] = true; // Vertex v visited
for (Edge e : neighbors.get(u))
if (!isVisited[e.v]) { //e.v代表边e连接的另一个顶点的索引
parent[e.v] = u; // The parent of vertex e.v is u
dfs(e.v, parent, isVisited, searchOrder); // Recursive search
}
}
②复杂度 O(|E| + |V|)
③Applications of the DFS:
Detecting whether a graph is connected(从任意点查询,如果找到的点数量等于V,则图为连通图)
Detecting whether there is a path between two vertices AND find it
Finding all connected components. A connected component is a maximal connected subgraph in which every pair of vertices are connected by a path
3.3 Breadth-First Search
①步骤:The first level consists of the starting vertex (root) . Each next level consists of the vertices adjacent to the vertices in the preceding level
@Override /** Starting bfs search from vertex v */
public Tree bfs(int v) {
List<Integer> searchOrder = new ArrayList();
int[] parent = new int[vertices.size()];
for (int i = 0; i < parent.length; i++)
parent[i] = -1; // Initialize parent[i] to -1
// list used as a queue
java.util.LinkedList<Integer> queue = new java.util.LinkedList();
queue.offer(v); // Enqueue v
boolean[] isVisited = new boolean[vertices.size()];
isVisited[v] = true; // Mark it visited
while (!queue.isEmpty()) {
int u = queue.poll(); // Dequeue to u
searchOrder.add(u); // u searched
for (Edge e: neighbors.get(u))
if (!isVisited[e.v]) {
queue.offer(e.v); // Enqueue v
parent[e.v] = u; // The parent of w is u
isVisited[e.v] = true; // Mark it visited
}
}
return new Tree(v, parent, searchOrder);
}
②Applications of the BFS:Detecting whether a graph is connected;Detecting whether there is a path between two vertices;Finding a shortest path between two vertices;Finding all connected components ; Detect whether there is a cycle(if a node was seen before, then there is a cycle);Testing whether a graph is bipartite(点可以分为两个独立集合,在同一集合里点之间不存在边)
4. Weighted Graphs
4.1 Representing Weighted Graphs
①Edge Array:int[][] edges = {{0, 1, 2}} 点,点,权重
②Weighted Adjacency Matrices:Integer[][] adjacencyMatrix = { {null, 2, null, 8, null } }表示点到点的距离
③Edge Adjacency Lists:
java.util.List[] list = new java.util.List[5]; list[0] 包括WeightedEdge(0, 1, 2) ,WeightedEdge(0, 3, 8)
OR List> list = new java.util.ArrayList();
5. Minimum Spanning Trees
5.1 定义
A minimum spanning tree(connects all the vertices together without any cycles) is a spanning tree with the minimum total weight
5.2 Prim’s Minimum Spanning Tree Algorithm
从选定顶点开始构建 MST。每一步,我们都会在当前树中没有的顶点上增加一条边,从而 "grow "这棵树,我们会选择一条 "attachment cost "最小的边,同时确保不会与已选的边形成循环。
5.3 Shortest Path
Find a shortest path between two vertices in the graph . The shortest path between two vertices is a path with the minimum total weight
In order to find a shortest path from vertex s to vertex v, Dijkstra’s algorithm finds the shortest path from s to all vertices.简单来说就是从初始点开始,一步步找没有连接的顶点的权重最小的边更新,然后同步把所有点的cost更新,父点更新。最终找到最短距离与MST
Lec11 Binary Search Trees
1. Binary Trees
1.1 Representing Binary Trees
A binary tree can be represented using a set of linked nodes, 每个节点包含一个element和两个名为左右的link
class TreeNode<E> {
E element;
TreeNode<E> left;
TreeNode<E> right;}
1.2 Representing Non-Binary Trees
A non-binary tree (like a file system) can be represented using a set of linked nodes
An ArrayList can also be used (instead of an array) to represent non-binary trees:
class TreeNode<E> {
E element;
TreeNode<E>[] children;}
class TreeNode<E> {
E element;
ArrayList<TreeNode<E>> children;}
1.3 Binary Search Trees
A special type of binary trees, called binary search tree is a binary tree with no duplicate elements,左子树都小于父点,右子树都大于父点
public class BST<E extends Comparable<E>> extends AbstractTree<E> {
protected TreeNode<E> root;
1.4 Inserting an Element to a BST
① BST is empty, create a root node with the new element
② insert the element into a leaf:初始化根点,插入值小于就放左子树并递归的找插入的父点;插入值大于就放右子树并递归的找插入的父点
Node<E> current = root, parent = null;
while (current != null)
if (element < current.element) {
parent = current;
current = current.left;
} else if (element > current.element) {
parent = current;
current = current.right;
} else return false; // Duplicate node not inserted
// Create the new node and attach it to the parent node
if (element < parent.element)
parent.left = new TreeNode(element);
else
parent.right = new TreeNode(element);
return true; // Element inserted }
同样的死路和代码也可以去作为Searching an Element in a Binary Search Tree
1.5 Tree Traversal
Tree traversal is the process of visiting each node in the tree exactly once
① preorder:根左右
② inorder:左根右
③ postorder:左右根
④ depth-first:level by level,从根开始左往右,一层层找
⑤ breadth-first:branch by branch from left to right,和preorder一样
1.6 The Tree Interface
The Tree interface defines common operations for trees
public interface Tree<E> extends Iterable<E>{...}
public abstract class AbstractTree<E> implements Tree<E> {
@Override /** Preorder traversal from the root */
public void preorder() {}}
1.7 The BST Class
A concrete binary tree class BST extends AbstractTree
public class BST<E extends Comparable<E>> extends AbstractTree<E> {
protected TreeNode<E> root;
public static class TreeNode<E extends Comparable<E>> {
protected E element;
protected TreeNode<E> left;
protected TreeNode<E> right;
public TreeNode(E e) {
element = e;
}
protected void preorder(TreeNode<E> root) {
if (root == null) return;
System.out.print(root.element + " ");
preorder(root.left);
preorder(root.right);
}
}
1.8 Deleting Elements in a Binary Search Tree
It need to first locate the node that contains the element and also its parent node
There are two cases to consider:
Case 1: The current node does not have a left child。这时Simply connect the parent with the right child of the current node
Case 2: The current node has a left child。让rightMost指向被删节点的左子树的最大点,parentOfRightMost指向rightMost的父点(rightMost没有右子点,因为它是最大)。被删点与rightMost交换,将parentOfRightMost与rightMost的左子树或者节点连接,删除被删点。
public boolean delete(E e) {
// Locate the node to be deleted and also locate its parent node
TreeNode<E> parent = null;
TreeNode<E> current = root;
//search the elemnet代码省略了
if (current == null)
return false; // Element is not in the tree
// Case 1: current has no left child
if (current.left == null) {
// Connect the parent with the right child of the current node
if (parent == null) {
root = current.right;
} else {
if (e.compareTo(parent.element) < 0)
parent.left = current.right;
else
parent.right = current.right;
}
} else {
// Case 2: The current node has a left child
TreeNode<E> parentOfRightMost = current;
TreeNode<E> rightMost = current.left;
while (rightMost.right != null) {
parentOfRightMost = rightMost;
rightMost = rightMost.right; // Keep going to the right
}
// Replace the element in current by the element in rightMost代码省略
size--; return true;}
1.9 Binary Tree Time Complexity
The time complexity for the inorder, preorder, and postorder traversals is O(n), since each node is traversed only once
The time complexity for search, insertion and deletion is the height of the tree . In the worst case, the height of the tree is O(n)
1.10 Iterators
The Tree interface extends java.lang.Iterable. Since BST is a subclass of AbstractTree and AbstractTree implements Tree, BST is a subtype of Iterabl
eg:The iterator method for Tree returns an instance of InorderIterator
Iterator的实例被构造出来时,current value is initialized to 0. The hasNext() method checks whether current is still in the range of list;next() method returns the current element and moves current to point to the next element in the list; The remove() method removes the current element from the tree and a new list is created - current does not need to be changed.
@Override /** Get the current element and move to the next */
public E next() {
return list.get(current++);
}
@Override /** Remove the current element */
public void remove() {
BST.this.delete(list.get(current)); // Delete the current element
list.clear(); // Clear the list
inorder(); // Rebuild the list
}
1.11 Data Compression: Huffman Coding
Huffman coding compresses data by using fewer bits to encode more frequently occurring characters.
The left and right edges of any node are assigned a value 0 or 1 ; Each character is a leaf in the tree;The code for a character consists of the edge values in the path