在Android应用中,Canvas和Paint是两个绘图的基本类,使用这两个类几乎可以完成所有的绘制工作。
Canvas:画布,2D图形系统最核心的一个类,作为参数传入onDraw()方法,完成绘制工作,该类提供了各种绘制方法,用于绘制不同的图形,例如点、直线、矩形、圆、文本、颜色、位图等。
Paint:画笔,用于设置绘制的样式、颜色等信息。
Canvas类常用方法
方法 | 描述 |
---|
drawArc (RectF oval, float startAngle, float sweepAngle, boolean useCenter, Paint paint) | 绘制弧形 |
drawBitmap (Bitmap bitmap, float left, float top, Paint paint) | 绘制位图 |
drawCircle (float cx, float cy, float radius, Paint paint) | 绘制圆形 |
drawLine (float startX, float startY, float stopX, float stopY, Paint paint) | 绘制一条线 |
drawPoint (float x, float y, Paint paint) | 绘制一个点 |
drawRect (float left, float top, float right, float bottom, Paint paint) | 绘制矩形 |
drawText (String text, float x, float y, Paint paint | 绘制字符串 |
Paint类常用方法
方法 | 描述 |
---|
setAlpha(int a) | 设置透明度 |
setARGB(int a, int r, int g, int b) | 绘制颜色 |
setColor(int color) | 设置颜色 |
setShader(Shader shader) | 设置渲染效果 |
setShadowLayer(float radius, float dx, float dy, int color) | 设置阴影 |
setStrokeWidth(float width) | 设置画笔粗细 |
setStyle(Paint.Style style) | 设置画笔风格 |
Shader类的子类
子类 | 构造方法 | 描述 |
---|
BitmapShader | BitmapShader(Bitmap bitmap,Shader.TileMode tileX, Shader.TileMode tileY) | 使用位图平铺的渲染效果 |
LinearGradient | LinearGradient(float x0, float y0, float x1, float y1, int[] colors, float[ ] positions, Shader.TileMode tile)- - LinearGradient(float x0, float y0, float x1, float y1, int color0, int color1, Shader.TileMode tile) | 使用线性渐变来渲染图形 |
RadialGradient | RadialGradient (float x, float y, float radius, int[] colors, float[] positions, Shader.TileMode tile)-RadialGradient(float x, float y, float radius, int color0, int color1, Shader.TileMode tile) | 使用圆形渐变来渲染图形 |
SweepGradient | SweepGradient(float cx, float cy, int[] colors, float[] positions) - - - - - - - - - - - - - - - SweepGradient(float cx, float cy, int color0, int color1) | 使用角度渐变来渲染图形 |
ComposeShader | ComposeShader(Shader shaderA, Shader shaderB, PorterDuff.Mode mode) - - ComposeShader(Shader shaderA, Shader shaderB, Xfermode mode) | 使用组合效果来渲染图形 |
Path类常用方法
方法 | 描述 |
---|
addCircle(float x, float y, float radius, Path.Direction dir) | 为路径添加一个圆形轮廓 |
addRect(float left, float top, float right, float bottom, Path.Direction dir) | 为路径添加一个矩形轮廓 |
close() | 将目前的轮廓闭合,即连接起点和终点 |
lineTo(float x, float y) | 从最后一个点到点(x,y)之间画一条线 |
moveTo(float x, float y) | 设置下一个轮廓的起点 |
PathEffect类的子类
子类 | 方法 | 描述 |
---|
CornerPathEffect | CornerPathEffect(float radius) | 使用圆角来代替尖角,从而对图形尖锐的边角进行平滑处理 |
DashPathEffect | DashPathEffect(float[] intervals, float phase) | 创建一个虚线的轮廓(短横线/小圆点) |
DiscretePathEffect | DiscretePathEffect(float segmentLength, float deviation) | 与DashPathEffect相似,但是添加了随机性,需要指定每一段的长度和与原始路径的偏离度 |
PathDashPathEffect | PathDashPathEffect(Path shape, float advance, float phase, PathDashPathEffect. Style style) | 定义一个新的路径,并将其用作原始路径的轮廓标记 |
SumPathEffect | SumPathEffect(PathEffect first, PathEffect second) | 添加两种效果,将两种效果结合起来 |
ComposePathEffect | ComposePathEffect(PathEffect outerpe, PathEffect innerpe) | 在路径上先使用第一种效果,再在此基础上应用第二种效果 |
package com.file.file
import android.app.Activity
import android.content.Context
import android.graphics.Canvas
import android.graphics.Color
import android.graphics.ComposePathEffect
import android.graphics.CornerPathEffect
import android.graphics.DashPathEffect
import android.graphics.DiscretePathEffect
import android.graphics.Paint
import android.graphics.Path
import android.graphics.PathDashPathEffect
import android.graphics.PathEffect
import android.graphics.RadialGradient
import android.graphics.RectF
import android.graphics.Shader
import android.graphics.SumPathEffect
import android.os.Bundle
import android.view.View
public class MainActivity extends Activity {
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState)
setContentView(new MyView(this))
}
class MyView extends View
{
float phase1=0
float phase2=0
Path path
public MyView(Context context) {
super(context)
path=new Path()
path.moveTo(0, 0)
for (int i = 1
{
//生成15个点,随机生成它们的Y座标。并将它们连成一条Path
path.lineTo(i * 30, (float) Math.random() * 20)
}
}
protected void onDraw(Canvas canvas) {
super.onDraw(canvas)
//设置画布为白色
canvas.drawColor(Color.WHITE)
//设置画笔样式
Paint paint=new Paint()
//抗锯齿
paint.setAntiAlias(true)
paint.setColor(Color.BLUE)
paint.setStyle(Paint.Style.STROKE)
paint.setStrokeWidth(2.5f)
//绘制圆形
canvas.drawCircle(35, 35, 25, paint)
//绘制椭圆
RectF r1=new RectF(10, 70, 60, 100)
canvas.drawOval(r1, paint)
//绘制矩形
canvas.drawRect(10,110,60,140,paint)
//绘制圆角矩形
RectF r2=new RectF(10, 150, 60, 180)
canvas.drawRoundRect(r2, 15, 15, paint)
//绘制路径为三角形的图形
Path path1=new Path()
path1.moveTo(10, 230)
path1.lineTo(60, 230)
path1.lineTo(35, 190)
path1.close()
canvas.drawPath(path1, paint)
//重新设置画笔样式
paint.setColor(Color.YELLOW)
paint.setStyle(Paint.Style.FILL)
//重新绘制以上图形的另一种样式
canvas.drawCircle(95, 35, 25, paint)
RectF r3=new RectF(70, 70, 120, 100)
canvas.drawOval(r3, paint)
canvas.drawRect(70,110,120,140,paint)
RectF r4=new RectF(70, 150, 120, 180)
canvas.drawRoundRect(r4, 15, 15, paint)
Path path2=new Path()
path2.moveTo(70, 230)
path2.lineTo(120, 230)
path2.lineTo(95, 190)
path2.close()
canvas.drawPath(path2, paint)
//设置渲染效果
Shader myShader=new RadialGradient(0, 0, 25,
new int[]{Color.GREEN,Color.RED},
null,Shader.TileMode.REPEAT)
paint.setShader(myShader)
paint.setShadowLayer(15, 5, 5, Color.BLACK)
//再次绘制图形
canvas.drawCircle(155, 35, 25, paint)
RectF r5=new RectF(130, 70, 180, 100)
canvas.drawOval(r5, paint)
canvas.drawRect(130,110,180,140,paint)
RectF r6=new RectF(130, 150, 180, 180)
canvas.drawRoundRect(r6, 15, 15, paint)
Path path3=new Path()
path3.moveTo(130, 230)
path3.lineTo(180, 230)
path3.lineTo(155, 190)
path3.close()
canvas.drawPath(path3, paint)
//取消渲染效果
paint.setShader(null)
//取消阴影
paint.setShadowLayer(0, 0, 0, 0)
//设置文字大小
paint.setTextSize(20)
//设置文字颜色
paint.setColor(Color.BLACK)
//绘制字符串
canvas.drawText(getResources().getString(R.string.circle), 225, 45, paint)
canvas.drawText(getResources().getString(R.string.oval), 215, 90, paint)
canvas.drawText(getResources().getString(R.string.rect), 215, 135, paint)
canvas.drawText(getResources().getString(R.string.round_rect), 195, 170, paint)
canvas.drawText(getResources().getString(R.string.triangle), 210, 220, paint)
//使用PathEffect
paint.setStyle(Paint.Style.STROKE)
PathEffect[] pathEffects=new PathEffect[8]
//不添加PathEffect
pathEffects[0]=null
//添加CornerPathEffect效果
pathEffects[1]=new CornerPathEffect(5)
//添加DashPathEffect效果
pathEffects[2]=new DashPathEffect(new float[]{2,8,15,2}, phase1)
//添加DiscretePathEffect效果
pathEffects[3]=new DiscretePathEffect(1.5f,5)
//添加PathDashPathEffect效果
Path path5=new Path()
path5.addRect(0 , 0, 5, 5, Path.Direction.CCW)
pathEffects[4]=new PathDashPathEffect(path5, 2.0f, phase1, PathDashPathEffect.Style.ROTATE)
//添加SumPathEffect效果
pathEffects[5]=new SumPathEffect(pathEffects[3], pathEffects[4])
//添加ComposePathEffect效果
pathEffects[6]=new ComposePathEffect(pathEffects[2], pathEffects[3])
//对比ComposePathEffect和SumPathEffect
pathEffects[7]=new SumPathEffect(pathEffects[2], pathEffects[3])
//移动画布
canvas.translate(0, 50)
for (int i = 0
paint.setPathEffect(pathEffects[i])
canvas.drawPath(path1, paint)
canvas.translate(60, 0)
}
canvas.translate(-240, 55)
for (int i = 4
paint.setPathEffect(pathEffects[i])
canvas.drawPath(path1, paint)
canvas.translate(60, 0)
}
canvas.translate(-230, 250)
PathEffect pathEffect=new DashPathEffect(new float[]{15,10,5,10}, phase2)
paint.setPathEffect(pathEffect)
canvas.drawPath(path, paint)
phase2+=1
//重绘
invalidate()
}
}
}
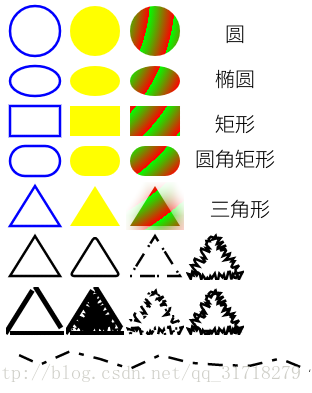