Crashing Robots
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 9887 | Accepted: 4220 |
Description
In a modernized warehouse, robots are used to fetch the goods. Careful planning is needed to ensure that the robots reach their destinations without crashing into each other. Of course, all warehouses are rectangular, and all robots occupy a circular floor space with a diameter of 1 meter. Assume there are N robots, numbered from 1 through N. You will get to know the position and orientation of each robot, and all the instructions, which are carefully (and mindlessly) followed by the robots. Instructions are processed in the order they come. No two robots move simultaneously; a robot always completes its move before the next one starts moving.
A robot crashes with a wall if it attempts to move outside the area of the warehouse, and two robots crash with each other if they ever try to occupy the same spot.
A robot crashes with a wall if it attempts to move outside the area of the warehouse, and two robots crash with each other if they ever try to occupy the same spot.
Input
The first line of input is K, the number of test cases. Each test case starts with one line consisting of two integers, 1 <= A, B <= 100, giving the size of the warehouse in meters. A is the length in the EW-direction, and B in the NS-direction.
The second line contains two integers, 1 <= N, M <= 100, denoting the numbers of robots and instructions respectively.
Then follow N lines with two integers, 1 <= Xi <= A, 1 <= Yi <= B and one letter (N, S, E or W), giving the starting position and direction of each robot, in order from 1 through N. No two robots start at the same position.
Figure 1: The starting positions of the robots in the sample warehouse
Finally there are M lines, giving the instructions in sequential order.
An instruction has the following format:
< robot #> < action> < repeat>
Where is one of
and 1 <= < repeat> <= 100 is the number of times the robot should perform this single move.
The second line contains two integers, 1 <= N, M <= 100, denoting the numbers of robots and instructions respectively.
Then follow N lines with two integers, 1 <= Xi <= A, 1 <= Yi <= B and one letter (N, S, E or W), giving the starting position and direction of each robot, in order from 1 through N. No two robots start at the same position.
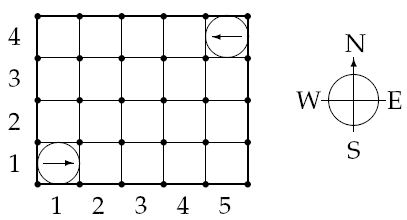
Figure 1: The starting positions of the robots in the sample warehouse
Finally there are M lines, giving the instructions in sequential order.
An instruction has the following format:
< robot #> < action> < repeat>
Where is one of
- L: turn left 90 degrees,
- R: turn right 90 degrees, or
- F: move forward one meter,
and 1 <= < repeat> <= 100 is the number of times the robot should perform this single move.
Output
Output one line for each test case:
Only the first crash is to be reported.
- Robot i crashes into the wall, if robot i crashes into a wall. (A robot crashes into a wall if Xi = 0, Xi = A + 1, Yi = 0 or Yi = B + 1.)
- Robot i crashes into robot j, if robots i and j crash, and i is the moving robot.
- OK, if no crashing occurs.
Only the first crash is to be reported.
Sample Input
4 5 4 2 2 1 1 E 5 4 W 1 F 7 2 F 7 5 4 2 4 1 1 E 5 4 W 1 F 3 2 F 1 1 L 1 1 F 3 5 4 2 2 1 1 E 5 4 W 1 L 96 1 F 2 5 4 2 3 1 1 E 5 4 W 1 F 4 1 L 1 1 F 20
Sample Output
Robot 1 crashes into the wall Robot 1 crashes into robot 2 OK Robot 1 crashes into robot 2
咳咳,模拟题。这一类的题呢没什么算法就是写程序麻烦一些,另外一个就是要有耐心慢慢来,想明白点才行,一次成功才行,不然找错会很麻烦有可能只是一个很小的点,你以为是大的错就大改程序。像我就是第一次WA我只改了一个地方就是%4忘记了,差一点就要大改来着QAQ
#include <stdio.h>
#include <string.h>
int d[110];
char s[2];
int x[110],y[110];
int flag[110][110];
int main()
{
int i;
int n,m;
int hang,lie;
int t;
scanf("%d",&t);
while(t--)
{
memset(flag,0,sizeof(flag));
scanf("%d%d",&lie,&hang);
scanf("%d%d",&n,&m);
for(i=1; i<=n; ++i)
{
scanf("%d %d %s",&y[i],&x[i],s);
flag[x[i]][y[i]]=i;
if(s[0]=='E')d[i]=0;
if(s[0]=='N')d[i]=1;
if(s[0]=='W')d[i]=2;
if(s[0]=='S')d[i]=3;
}
int f=1;
while(m--)
{
int num,p;
scanf("%d %s %d",&num,s,&p);
if(f)
{
if(s[0]=='L')
{
d[num]=(d[num]+p)%4;
}
if(s[0]=='R')
{
d[num]=(d[num]+(4-p%4))%4;
}
if(s[0]=='F')
{
if(d[num]==0)
{
for(i=y[num]+1; i<=y[num]+p; ++i)
{
if(i>lie)
{
printf("Robot %d crashes into the wall\n",num);
f=0;
break;
}
if(flag[x[num]][i])
{
printf("Robot %d crashes into robot %d\n",num,flag[x[num]][i]);
f=0;
break;
}
}
if(f)
{
flag[x[num]][y[num]]=0;
y[num]+=p;
flag[x[num]][y[num]]=num;
}
}
if(d[num]==3)
{
for(i=x[num]-1; i>=x[num]-p; --i)
{
if(i<1)
{
printf("Robot %d crashes into the wall\n",num);
f=0;
break;
}
if(flag[i][y[num]])
{
printf("Robot %d crashes into robot %d\n",num,flag[i][y[num]]);
f=0;
break;
}
}
if(f)
{
flag[x[num]][y[num]]=0;
x[num]-=p;
flag[x[num]][y[num]]=num;
}
}
if(d[num]==2)
{
for(i=y[num]-1; i>=y[num]-p; --i)
{
if(i<1)
{
printf("Robot %d crashes into the wall\n",num);
f=0;
break;
}
if(flag[x[num]][i])
{
printf("Robot %d crashes into robot %d\n",num,flag[x[num]][i]);
f=0;
break;
}
}
if(f)
{
flag[x[num]][y[num]]=0;
y[num]-=p;
flag[x[num]][y[num]]=num;
}
}
if(d[num]==1)
{
for(i=x[num]+1; i<=x[num]+p; ++i)
{
if(i>hang)
{
printf("Robot %d crashes into the wall\n",num);
f=0;
break;
}
if(flag[i][y[num]])
{
printf("Robot %d crashes into robot %d\n",num,flag[i][y[num]]);
f=0;
break;
}
}
if(f)
{
flag[x[num]][y[num]]=0;
x[num]+=p;
flag[x[num]][y[num]]=num;
}
}
}
}
}
if(f)printf("OK\n");
}
}