//#pragma GCC system_header
#ifndef lhd_stl__
#define lhd_stl__
namespace lhd_stl {
template<typename T> struct LinkNode {
T data;
LinkNode* next;
LinkNode* prev;
inline void del() {
delete next;
delete prev;
}
inline void operator = (LinkNode x) {
next = x.next;
prev = x.prev;
data = x.data;
}
};
template<typename T> class LinkList {
unsigned int linkNodeNum = 0;
LinkNode<T> head ;
LinkNode<T> finish;
public:
LinkList() {
head.next = finish.next = &finish;
head.prev = finish.prev = &head;
}
bool push_back(T data) {
LinkNode<T>x;
x.data = data;
x.prev = finish.prev;
x.next = &finish;
x.prev->next = &x;
finish.prev = &x;
return ++linkNodeNum;
}
bool push_front(T data) {
LinkNode<T> x;
x.data = data;
x.next = head.next;
x.next->prev = head.next = &x;
x.prev = &head;
return ++linkNodeNum;
}
bool pop_front() {
if (linkNodeNum == 0)throw "pop of list cannot be emtpy";
head.next = head.next->next;
head.next->prev->del();
head.next->prev = &head;
return --linkNodeNum;
}
bool pop_back() {
if (linkNodeNum == 0)throw "pop of list cannot be emtpy";
finish.prev = finish.prev->prev;
finish.prev->next->del();
finish.prev->next = &finish;
return --linkNodeNum;
}
unsigned int getSize() {
return linkNodeNum;
}
inline void clear() {
while (linkNodeNum)pop_back();
}
LinkList(unsigned size, T* x) {
head.next = finish.next = &finish;
head.prev = finish.prev = &head;
clear();
for (int i = 0; i < size; ++i) {
push_front(x[i]);
}
}
LinkList(unsigned size) {
head.next = finish.next = &finish;
head.prev = finish.prev = &head;
clear();
while (size--)push_back(0);
}
inline T front() {
return head.next->data;
}
inline T back() {
return finish.prev->data;
}
};
#ifndef SIZE_THREAD_OF_VECTOR
#define SIZE_THREAD_OF_VECTOR 1000
#endif
#if SIZE_THREAD_OF_VECTOR < 8
#warning size of vector should be over than 8.
#endif
template<typename ty>
class vector {
struct nod {
ty v[SIZE_THREAD_OF_VECTOR];
int shen;
inline void operator = (nod other) {
int i = 0;
for (i = 0; i <= SIZE_THREAD_OF_VECTOR / 8 * 8; i += 8) {
v[i + 0] = other.v[i + 0];
v[i + 1] = other.v[i + 1];
v[i + 2] = other.v[i + 2];
v[i + 3] = other.v[i + 3];
v[i + 4] = other.v[i + 4];
v[i + 5] = other.v[i + 5];
v[i + 6] = other.v[i + 6];
v[i + 7] = other.v[i + 7];
}
while (i < SIZE_THREAD_OF_VECTOR) {
v[i] = other.v[i++];
}
}
};
LinkList<nod>val;
unsigned s = 0;
protected:
inline void push(ty x) {
if (val.back().shen) {
val.back().v[SIZE_THREAD_OF_VECTOR - val.back().shen] = x;
--val.back().shen;
} else {
nod a;
a.shen = SIZE_THREAD_OF_VECTOR - 1;
a.v[0] = x;
val.push_back(a);
}
++s;
}
inline void pop(void) {
if (val.back().shen == 1) {
val.pop_back();
} else {
val.back().v[SIZE_THREAD_OF_VECTOR - val.back().shen] = 0;
val.back().shen++;
}
--s;
}
inline void push1(ty x) {
if (val.front().shen) {
val.front().v[SIZE_THREAD_OF_VECTOR - val.back().shen] = x;
--val.front().shen;
} else {
nod a;
a.shen = SIZE_THREAD_OF_VECTOR - 1;
a.v[0] = x;
val.push_front(a);
}
++s;
}
inline void pop1(void) {
if (val.front().shen == 1) {
val.pop_front();
} else {
val.front().v[SIZE_THREAD_OF_VECTOR - val.front().shen] = 0;
val.front().shen++;
}
--s;
}
public:
inline void push_back(ty x) {
push(x);
}
inline void pop_back(void) {
pop();
}
inline void push_front(ty x) {
push1(x);
}
inline void pop_front(void) {
pop1();
}
inline ty operator [] (int x) {
if (x >= s)throw "(in vector of lhd)idx >= size";
int t = x / 100;
LinkNode<nod>xx;
xx = val.front();
if (t)while (--t) {
xx = *(xx.next);
}
return xx.v[x % 100];
}
inline int size() {
return s;
}
};
#if SIZE_THREAD_OF_VECTOR < 8
template<typename ty>
class queue {
LinkList<ty> val;
public:
inline void push(ty data) {
val.push_front(data);
}
inline void pop() {
val.pop_back();
}
inline ty front() {
return val.front();
}
inline unsigned size() {
return val.getSize();
}
};
#elif SIZE_THREAD_OF_VECTOR >= 8
template<typename ty>class queue {
vector<ty> x;
public:
inline void pop() {
x.pop_back();
}
inline void push(ty x) {
x.push_front();
}
inline int size() {
return x.size();
}
inline ty front() {
return x[0];
}
};
#endif
template<typename ty>
class deque {
vector<ty>val;
public:
inline void pop_back() {
val.pop_back();
}
inline void push_back(ty x) {
val.pop_back(x);
}
inline void pop_front() {
val.pop_front();
}
inline void push_front(ty x) {
val.push_back(x);
}
inline int size() {
return val.size();
}
inline ty operator[](int x) {
return val[x];
}
inline ty front() {
return val[0];
}
inline ty back() {
return val[val.size() - 1];
}
};
}
#endif
手写stl,C语言请删namespace lhd_stl和最后一个‘}‘
于 2023-05-13 13:50:45 首次发布
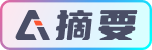