创建进程
#include <pthread.h>
int pthread_create(pthread_t *thread, const pthread_attr_t *attr,
void *(*start_routine) (void *), void *arg);
功能:在进程中创建子线程(原来的进程可以称为主线程)
参数:thread–创建成功的线程的ID号
attr–线程的属性,一般设置为NULL
start_routine-指向线程处理函数的指针
arg–传给线程处理函数的参数
线程处理函数:子线程的任务就是在这个函数中完成的该函数我们只需要传给pthread_create函数就好,只要线程创建成功,该函数就会被调用 线程就会参与系统的调度
返回值:成功 0, 失败 -1
#include <pthread.h>
int pthread_join(pthread_t thread, void **retval);
功能:主线程等待并回收子线程的退出状态(task_struct结构体)
参数:thread–要等待的线程的id号
retval–子线程的返回值
返回值:成功 0 失败 -1
信号量
#include <semaphore.h>
int sem_init(sem_t *sem,int pshared,unsigned int value);
/*信号量示例代码*/
#include<stdio.h>
#include <pthread.h>
#include <unistd.h>
#include <semaphore.h>
sem_t sem1, sem2;
void *theadFunc1(void *arg)
{
while(1)
{
//p
sem_wait(&sem1);
printf("thread1....\n");
sleep(1);
//v
sem_post(&sem2);
}
}
void *theadFunc2(void *arg)
{
while(1)
{
//p
sem_wait(&sem2);
printf("thread2....\n");
sleep(1);
//v
sem_post(&sem1);
}
}
int main()
{
//创建信号量,并初始化
sem_init(&sem1, 0, 1);
sem_init(&sem2, 0, 0);
//创建子线程
pthread_t thId1, thId2;
int ret = pthread_create(&thId1, NULL, theadFunc1, NULL);
if(ret < 0)
{
printf("pthread_create error!\n");
return -1;
}
ret = pthread_create(&thId2, NULL, theadFunc2, NULL);
if(ret < 0)
{
printf("pthread_create error!\n");
return -1;
}
//主线程
pthread_join(thId1, NULL);
pthread_join(thId2, NULL);
return 0;
}
互斥锁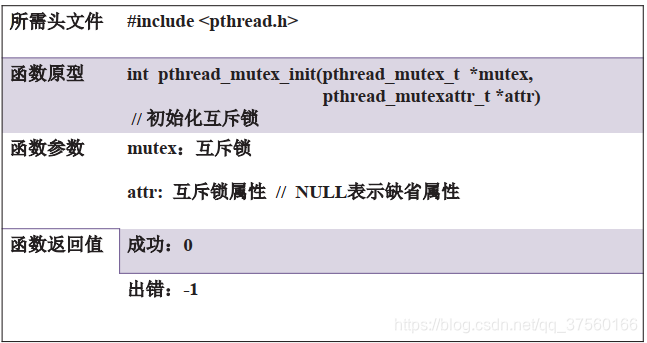
/*上锁解锁示例代码*/
#include<stdio.h>
#include <pthread.h>
#include <unistd.h>
char buf[100];
//锁的id
pthread_mutex_t mutex;
void *theadFunc(void *arg)
{
char *p = (char *)arg;
int i = 0;
//加锁
pthread_mutex_lock(&mutex);
while(p[i])
{
buf[i] = p[i];
i++;
usleep(500);
}
puts(buf);
//解锁
pthread_mutex_unlock(&mutex);
}
int main()
{
//得到锁
pthread_mutex_init(&mutex, NULL);
char buf1[100] = "12345678";
char buf2[100] = "abcdefgh";
//创建子线程
pthread_t thId1, thId2;
int ret = pthread_create(&thId1, NULL, theadFunc, buf1);
if(ret < 0)
{
printf("pthread_create error!\n");
return -1;
}
ret = pthread_create(&thId2, NULL, theadFunc, buf2);
if(ret < 0)
{
printf("pthread_create error!\n");
return -1;
}
//主线程
pthread_join(thId1, NULL);
pthread_join(thId2, NULL);
return 0;
}
生产者与消费者实例
/*
* File Name: pthread.c
* Author:lanyxs
* Mail:lanyxs@126.com
* Created Time: 2019年08月15日 星期四 03时27分53秒
*/
#include <stdio.h>
#include <pthread.h>
#include <unistd.h>
#include <semaphore.h>
#include <stdlib.h>
#include <time.h>
#define SUM 10
int value = 1; /*商品数量*/
char buf[30];
pthread_mutex_t mutex; /*初始化锁*/
sem_t sem_prod,sem_cons; /*信号量*/
/*生产者*/
void *pthreadFun_prod(void *arg)
{
//pthread_mutex_lock(&mutex); /*上锁*/
while(1)
{
sem_wait(&sem_prod);
puts("生产产品中...");
while(value <= SUM)
{
printf("%d\n",value);
value++;
sleep(1);
}
sem_post(&sem_cons);
}
//pthread_mutex_unlock(&mutex); /*解锁*/
}
/*消费者*/
void *pthreadFun_cons(void *arg)
{
while(1)
{
srand((unsigned)time(NULL));
int cons_sum = rand() % 10; /*产生随机数,随机消费*/
//pthread_mutex_lock(&mutex); /*上锁*/
sem_wait(&sem_cons);
puts("消费产品中...");
sleep(2);
printf("消费< %d >个产品\n",cons_sum);
value -= cons_sum;
printf("*****************************************\n\n");
sem_post(&sem_prod);
}
//pthread_mutex_unlock(&mutex); /*解锁*/
}
int main(int argc,char *argv[])
{
int ret = pthread_mutex_init(&mutex,NULL); /*初始化锁*/
if(ret < 0)
{
puts("pthread_mutex_init error.");
return -1;
}
/*创建信号量*/
sem_init(&sem_prod,0,1); /*生产者信号量*/
sem_init(&sem_cons,0,0); /*消费者信号量*/
/*创建进程1*/
pthread_t thid_prod,thid_cons;
ret = pthread_create(&thid_prod,NULL,pthreadFun_prod,NULL);
if(ret < 0)
{
puts("pthread error.");
return -1;
}
/*创建进程2*/
ret = pthread_create(&thid_cons,NULL,pthreadFun_cons,NULL);
if(ret < 0)
{
puts("pthread error.");
return -1;
}
/*主进程等待*/
pthread_join(thid_prod,NULL);
pthread_join(thid_cons,NULL);
return 0;
}