本文主要是实现卷积神经网络进行手写字体的识别,利用TensorFlow对卷积过程中的提取特征进行了可视化。具体代码如下:
# -*- coding: utf-8 -*-
"""
Created on Thu Apr 26 14:37:30 2018
@author: Administrator
"""
#导入所需要的库
import numpy as np
import tensorflow as tf
import matplotlib.pyplot as plt
from tensorflow.examples.tutorials.mnist import input_data
print ("库已准备好")
mnist = input_data.read_data_sets('mnist_data', one_hot=True)#第一个参数是存放数据集的地址
train_X = mnist.train.images
train_Y = mnist.train.labels
test_X = mnist.test.images
test_Y = mnist.test.labels
print ("数据集已准备好")
"""
这里可以选用CPU/GPU,当然也可以不选用
device_type = "/cpu:1"
with tf.device(device_type):
"""
n_input = 784 #输入图像大小是28*28*1=784
n_output = 10 #输出类别是10
weights = {
'wc1': tf.Variable(tf.random_normal([3, 3, 1, 64], stddev=0.1)), #第一卷积核是3*3*64,利用高斯分布初始化
'wd1': tf.Variable(tf.random_normal([14*14*64, n_output], stddev=0.1)) #第二层卷积核是14*14*64
}
biases = {
'bc1': tf.Variable(tf.random_normal([64], stddev=0.1)), #偏置,高斯分布初始化
'bd1': tf.Variable(tf.random_normal([n_output], stddev=0.1))
}
def conv_model(_input, _w, _b):
#把输入图像变成28*28*1
_input_r = tf.reshape(_input, shape=[-1, 28, 28, 1])
#第一层卷积:步长是1,padding='SAME'表示输出与输入大小一样
_conv1 = tf.nn.conv2d(_input_r, _w['wc1'], strides=[1, 1, 1, 1], padding='SAME')
#添加偏置
_conv2 = tf.nn.bias_add(_conv1, _b['bc1'])
#relu线性函数,max(0,x)
_conv3 = tf.nn.relu(_conv2)
#最大池化层,步长是2,padding='SAME'表示输出与输入大小一样
_pool = tf.nn.max_pool(_conv3, ksize=[1, 2, 2, 1], strides=[1, 2, 2, 1], padding='SAME')
#pool层输出压缩
_dense = tf.reshape(_pool, [-1, _w['wd1'].get_shape().as_list()[0]])
#全连接层
_out = tf.add(tf.matmul(_dense, _w['wd1']), _b['bd1'])
# Return everything
out = {
'input_r': _input_r, 'conv1': _conv1, 'conv2': _conv2, 'conv3': _conv3
, 'pool': _pool, 'dense': _dense, 'out': _out
}
return out
print ("CNN准备好")
#定义x,y,占位符
x = tf.placeholder(tf.float32, [None, n_input])
y = tf.placeholder(tf.float32, [None, n_output])
learning_rate = 0.001 #学习率
training_epochs = 10 #迭代次数
batch_size = 100 #batch是100
display_step = 1 #显示
prediction = conv_model(x, weights, biases)['out']
#交叉熵计算
cost = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits(logits = prediction,labels= y))
#优化cost,使cost达到最小
optm = tf.train.AdamOptimizer(learning_rate=learning_rate).minimize(cost)
corr = tf.equal(tf.argmax(prediction,1), tf.argmax(y,1)) # Count corrects
accr = tf.reduce_mean(tf.cast(corr, tf.float32)) # Accuracy
init = tf.initialize_all_variables() #变量初始化
# Saver
save_step = 1;
savedir = "tmp/"
saver = tf.train.Saver(max_to_keep=3)
print ("Network Ready to Go!")
do_train = 1
sess = tf.Session(config=tf.ConfigProto(allow_soft_placement=True))
sess.run(init)
if do_train == 1:
for epoch in range(training_epochs):
avg_cost = 0. #平均损失初始化
total_batch = int(mnist.train.num_examples/batch_size)
#遍历所有的batch
for i in range(total_batch):
batch_xs, batch_ys = mnist.train.next_batch(batch_size)
# 计算optm,数据来自于batch_xs,batch_ys
sess.run(optm, feed_dict={x: batch_xs, y: batch_ys})
#计算平均损失
avg_cost += sess.run(cost, feed_dict={x: batch_xs, y: batch_ys})/total_batch
# 显示
if epoch % display_step == 0:
print ("Epoch: %03d/%03d cost: %.9f" % (epoch, training_epochs, avg_cost))
train_acc = sess.run(accr, feed_dict={x: batch_xs, y: batch_ys})
print (" Training accuracy: %.3f" % (train_acc))
test_acc = sess.run(accr, feed_dict={x: test_X, y: test_Y})
print (" Test accuracy: %.3f" % (test_acc))
print ("Optimization Finished.")
if do_train == 0:
epoch = training_epochs-1
saver.restore(sess, "/tmp/cnn_mnist_simple.ckpt-" + str(epoch))
print ("NETWORK RESTORED")
conv_out = conv_model(x, weights, biases)
input_r = sess.run(conv_out['input_r'], feed_dict={x: train_X[0:1, :]})
conv1 = sess.run(conv_out['conv1'], feed_dict={x: train_X[0:1, :]})
conv2 = sess.run(conv_out['conv2'], feed_dict={x: train_X[0:1, :]})
conv3 = sess.run(conv_out['conv3'], feed_dict={x: train_X[0:1, :]})
pool = sess.run(conv_out['pool'], feed_dict={x: train_X[0:1, :]})
dense = sess.run(conv_out['dense'], feed_dict={x: train_X[0:1, :]})
out = sess.run(conv_out['out'], feed_dict={x: train_X[0:1, :]})
# 显示'input_r'
print ("Size of 'input_r' is %s" % (input_r.shape,))
label = np.argmax(train_Y[0, :])
print ("Label is %d" % (label))
# 画图显示
plt.matshow(input_r[0, :, :, 0], cmap=plt.get_cmap('gray'))
plt.title("Label of this image is " + str(label) + "")
plt.colorbar()
plt.show()
print ("Size of 'conv1' is %s" % (conv1.shape,))
# 画图显示
for i in range(3):
plt.matshow(conv1[0, :, :, i], cmap=plt.get_cmap('gray'))
plt.title(str(i) + "th conv1")
plt.colorbar()
plt.show()
# 画图显示
for i in range(3):
plt.matshow(conv2[0, :, :, i], cmap=plt.get_cmap('gray'))
plt.title(str(i) + "th conv2")
plt.colorbar()
plt.show()
print ("Size of 'conv3' is %s" % (conv3.shape,))
# 画图显示
for i in range(3):
plt.matshow(conv3[0, :, :, i], cmap=plt.get_cmap('gray'))
plt.title(str(i) + "th conv3")
plt.colorbar()
plt.show()
print ("Size of 'pool' is %s" % (pool.shape,))
# 画图显示
for i in range(3):
plt.matshow(pool[0, :, :, i], cmap=plt.get_cmap('gray'))
plt.title(str(i) + "th pool")
plt.colorbar()
plt.show()
print ("Size of 'dense' is %s" % (dense.shape,))
# Let's see 'out'
print ("Size of 'out' is %s" % (out.shape,))
# 显示weight!
wc1 = sess.run(weights['wc1'])print ("Size of 'wc1' is %s" % (wc1.shape,))
# 画图显示
for i in range(3):
plt.matshow(wc1[:, :, 0, i], cmap=plt.get_cmap('gray'))
plt.title(str(i) + "th conv filter")
plt.colorbar()
plt.show()
代码截图如下:
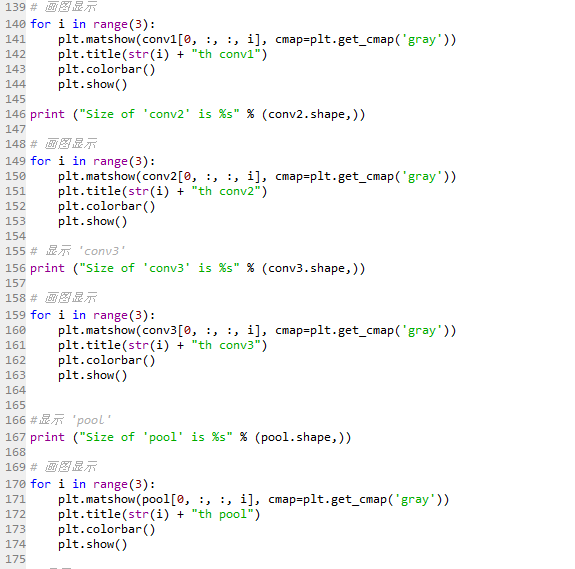
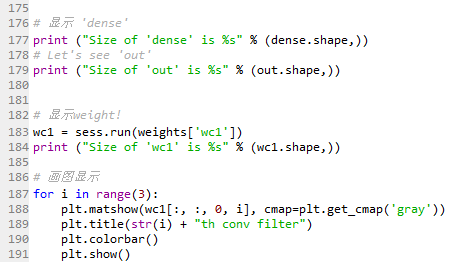
参考文献: http://blog.csdn.net/u013719780?viewmode=contents