P3
P3 - 1 C++ 映射输入
P3 - 1 - 1 Melee....h
#pragma once
#include "CoreMinimal.h"
#include "GameFramework/Character.h"
#include "MeleeCombatSystemCPPCharacter.generated.h"
UCLASS(config=Game)
class AMeleeCombatSystemCPPCharacter : public ACharacter
{
GENERATED_BODY()
//弹簧臂
UPROPERTY(VisibleAnywhere, BlueprintReadOnly, Category = Camera, meta = (AllowPrivateAccess = "true"))
class USpringArmComponent* CameraBoom;
//相机
UPROPERTY(VisibleAnywhere, BlueprintReadOnly, Category = Camera, meta = (AllowPrivateAccess = "true"))
class UCameraComponent* FollowCamera;
public:
AMeleeCombatSystemCPPCharacter();
protected:
void MoveForward(float Value);
void MoveRight(float Value);
void TurnRight(float Value);
void LookUp(float Value);
virtual void SetupPlayerInputComponent(class UInputComponent* PlayerInputComponent) override;
virtual void BeginPlay();
public:
FORCEINLINE class USpringArmComponent* GetCameraBoom() const { return CameraBoom; }
FORCEINLINE class UCameraComponent* GetFollowCamera() const { return FollowCamera; }
private:
UPROPERTY(meta = (AllowPrivateAccess))
class APlayerController* MeleeCombatSystemPC;
};
P3 - 1 - 2 Melee....cpp
#include "MeleeCombatSystemCPPCharacter.h"
#include "Camera/CameraComponent.h"
#include "Components/CapsuleComponent.h"
#include "Components/InputComponent.h"
#include "GameFramework/CharacterMovementComponent.h"
#include "GameFramework/SpringArmComponent.h"
#include "Kismet/GameplayStatics.h"
#include "Kismet/KismetMathLibrary.h"
AMeleeCombatSystemCPPCharacter::AMeleeCombatSystemCPPCharacter()
{
//设置胶囊体大小
GetCapsuleComponent()->InitCapsuleSize(42.f, 96.0f);
//取消控制器控制角色旋转
bUseControllerRotationPitch = false;
bUseControllerRotationYaw = false;
bUseControllerRotationRoll = false;
//朝向移动方向旋转
GetCharacterMovement()->bOrientRotationToMovement = true;
//设置角速度
GetCharacterMovement()->RotationRate = FRotator(0.0f, 500.0f, 0.0f);
//设置速度
GetCharacterMovement()->JumpZVelocity = 700.f;
GetCharacterMovement()->AirControl = 0.35f;
GetCharacterMovement()->MaxWalkSpeed = 500.f;
GetCharacterMovement()->MinAnalogWalkSpeed = 20.f; //用于设置角色在模拟行走时的最小速度
GetCharacterMovement()->BrakingDecelerationWalking = 2000.0f; //制动加速度比率,这里我调小了,停止输入之后直接就冰面滑行了
//弹簧臂
CameraBoom = CreateDefaultSubobject<USpringArmComponent>(TEXT("CameraBoom"));
CameraBoom->SetupAttachment(RootComponent);
CameraBoom->TargetArmLength = 400.0f;
CameraBoom->bUsePawnControlRotation = true; //用控制器控制角色旋转
FollowCamera = CreateDefaultSubobject<UCameraComponent>(TEXT("FollowCamera"));
FollowCamera->SetupAttachment(CameraBoom, USpringArmComponent::SocketName);
FollowCamera->bUsePawnControlRotation = false;
//获取PlayerController
MeleeCombatSystemPC = UGameplayStatics::GetPlayerController(GetWorld(),0);
}
void AMeleeCombatSystemCPPCharacter::BeginPlay()
{
Super::BeginPlay();
}
void AMeleeCombatSystemCPPCharacter::SetupPlayerInputComponent(class UInputComponent* PlayerInputComponent)
{
PlayerInputComponent->BindAction(TEXT("Jump"),IE_Pressed,this,&ThisClass::Jump);
PlayerInputComponent->BindAxis(TEXT("MoveForward"),this,&ThisClass::MoveForward);
PlayerInputComponent->BindAxis(TEXT("MoveRight"),this,&ThisClass::MoveRight);
PlayerInputComponent->BindAxis(TEXT("TurnRight"),this,&ThisClass::TurnRight);
PlayerInputComponent->BindAxis(TEXT("LookUp"),this,&ThisClass::LookUp);
}
//前后移动
void AMeleeCombatSystemCPPCharacter::MoveForward(float Value)
{
if(MeleeCombatSystemPC)
{
FRotator PlayerControllerRotation = {0,MeleeCombatSystemPC->GetControlRotation().Yaw,0};
auto ControllerForwardVector = UKismetMathLibrary::GetForwardVector(PlayerControllerRotation);
AddMovementInput(ControllerForwardVector,Value);
}
}
//左右移动
void AMeleeCombatSystemCPPCharacter::MoveRight(float Value)
{
if(MeleeCombatSystemPC)
{
FRotator PlayerControllerRotation = FRotator{0,MeleeCombatSystemPC->GetControlRotation().Yaw,0};
auto PlayerControllerRightVector = UKismetMathLibrary::GetRightVector(PlayerControllerRotation);
AddMovementInput(PlayerControllerRightVector,Value);
}
}
//摄像机 偏航角
void AMeleeCombatSystemCPPCharacter::TurnRight(float Value)
{
AddControllerYawInput(Value);
}
//摄像机 俯仰角
void AMeleeCombatSystemCPPCharacter::LookUp(float Value)
{
AddControllerPitchInput(-1 * Value);
}
P3 - 2 动画蓝图 - Control Rig 节点
- 在第三人称模板默认的动画蓝图中 从目前的效果来看 这个节点的作用是 是否开启 FootIK.
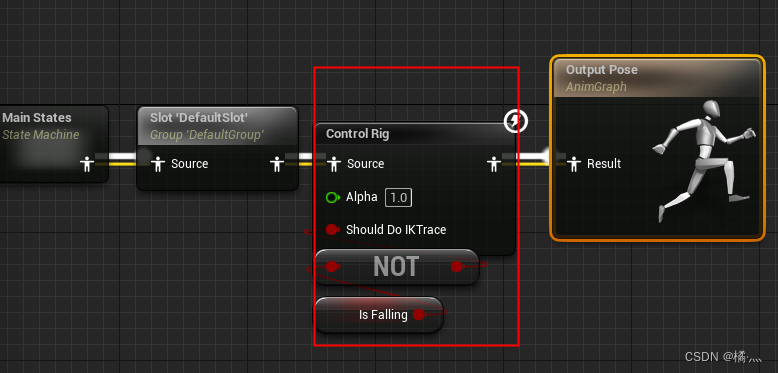
P4
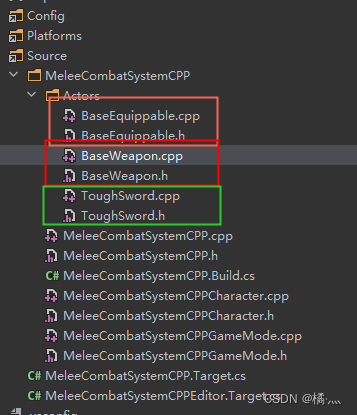
P4 - 1 关于 Attachment 节点 Weld Simulated Bodies
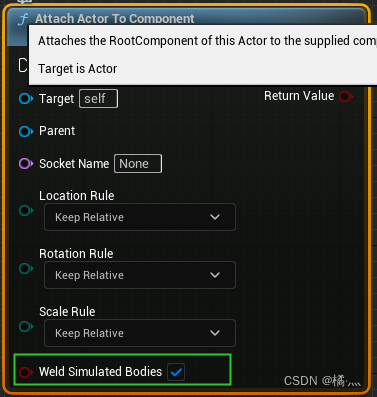
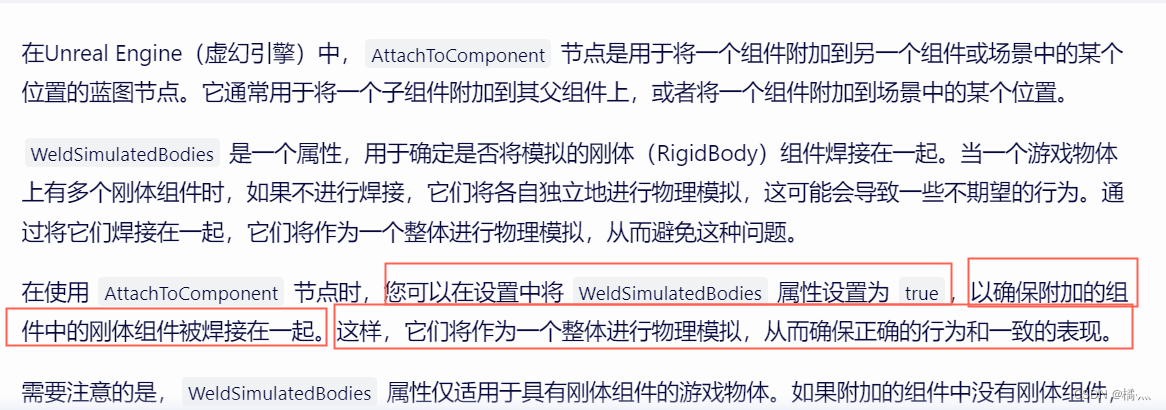
P4 - 2 AttachToComponent - 附加 - C++
void ABaseEquippable::AttachActor(const FName SocketName)
{
//获取 Owner 的 Mesh
auto CharacterMesh = Cast<ACharacter>(GetOwner())->GetMesh();
//创建 附加的规则
FAttachmentTransformRules TransformRules ={
EAttachmentRule::SnapToTarget,
EAttachmentRule::SnapToTarget,
EAttachmentRule::SnapToTarget
,true};
//附加
AttachToComponent(CharacterMesh,TransformRules,SocketName);
}
P4 - X - 3 SpawnActor
void AMeleeCombatSystemCPPCharacter::BeginPlay()
{
Super::BeginPlay();
//创建 Tough Sword
FActorSpawnParameters SpawnParameters;
SpawnParameters.SpawnCollisionHandlingOverride = ESpawnActorCollisionHandlingMethod::Undefined;
SpawnParameters.Owner = this;
SpawnParameters.Instigator = this;
ABaseEquippable* EqToughSword = GetWorld()->SpawnActor<AToughSword>(AToughSword::StaticClass(),GetActorTransform(),SpawnParameters);
//调用 BaseEq 的 Eq 方法
EqToughSword->OnEquipped();
}
P5
P5 - 1 Anim Notify
P5 - 1 - 1 Notify() override;
- 在蓝图里面的重载 叫 Received Notify
class MELEECOMBATSYSTEMCPP_API UAttachWeaponActorAN : public UAnimNotify
{
GENERATED_BODY()
protected:
virtual void Notify(USkeletalMeshComponent* MeshComp, UAnimSequenceBase* Animation, const FAnimNotifyEventReference& EventReference) override;
};
P5 - 1 - 2 GerOwner();
- 获取 执行动画的 角色 , 一般需要 Cast 成自己的PlayerCharacter.
void UAttachWeaponActorAN::Notify(USkeletalMeshComponent* MeshComp, UAnimSequenceBase* Animation,
const FAnimNotifyEventReference& EventReference)
{
Super::Notify(MeshComp, Animation, EventReference);
//Get Player Character
AMeleeCombatSystemCPPCharacter* PlayerCharacter = Cast<AMeleeCombatSystemCPPCharacter>( MeshComp->GetOwner());
}
P5 - 2 AnimBP - Layered blend per bone
- 之前也提到过 , 这里只是重新提一下,就不过多赘述了.
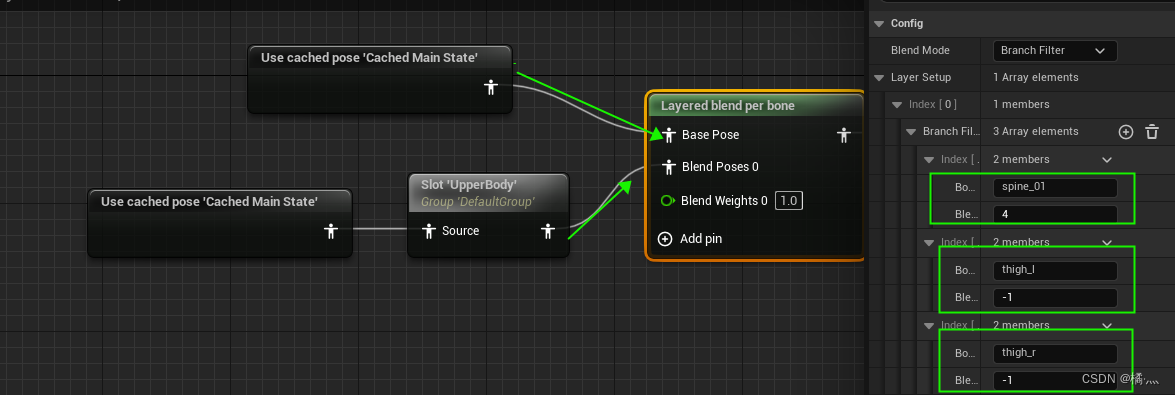