C++ STL算法篇(一) day17
查找类算法
基本查找
- find:区间查找
- find_if:条件查找
- find_first_of:查找区间第一次出现的值
- adjacent_find:查找第一次重复的值
- search:子序列查找
- search_n:子序列查找出现的次数
#include <iostream>
#include <algorithm>
#include <vector>
class student
{
public:
student(std::string name = "", int age = 0) :name(name), age(age) {}
std::string getName() { return name; }
int getAge() { return age; }
bool operator==(const std::string& name)
{
return this->name == name;
}
bool operator==(const int& age)
{
return this->age == age;
}
protected:
std::string name;
int age;
};
void basicSerch()
{
std::vector<int> vec{ 1,3,4,6,2,8,9,5,2,2,1,1,1};
auto it = find(vec.begin(), vec.end(), 2);
if (it != vec.end())
{
std::cout << *it << std::endl;
}
std::vector<student> vec2{ {"小瓜",21},{"大瓜",23},{"傻瓜",22} };
auto it2 = find(vec2.begin(), vec2.end(), "小瓜");
if (it2 != vec2.end())
{
std::cout << it2->getName() << " " << it2->getAge() << std::endl;
}
it2 = find(vec2.begin(), vec2.end(), 23);
if (it2 != vec2.end())
{
std::cout << it2->getName() << " " << it2->getAge() << std::endl;
}
it = find_if(vec.begin(), vec.end(), [](int& data) {return data == 2; });
if (it != vec.end())
{
std::cout << *it << std::endl;
}
int inData;
std::cin >> inData;
it = find_if(vec.begin(), vec.end(), [&](int& data) {return data == inData; });
if (it != vec.end())
{
std::cout << *it << std::endl;
}
it2 = find_if(vec2.begin(), vec2.end(), [](student& object) {return object.getName() == "小瓜"; });
if (it2 != vec2.end())
{
std::cout << it2->getName() << " " << it2->getAge() << std::endl;
}
int arr[]{ 7,8,3 };
std::cout << *find_first_of(vec.begin(), vec.end(), arr, arr + 3) << std::endl;
std::vector<int> vec3{ 1,23,3,2,3,4,6,5,5,8,9 };
std::cout << *adjacent_find(vec3.begin(), vec3.end()) << std::endl;
std::vector<int>vec4{ 5,2,2 };
auto ifSearch = search(vec.begin(), vec.end(), vec4.begin(), vec4.end());
if (ifSearch != vec.end())
{
std::cout << "searchc查找:" << std::endl;
std::cout << *ifSearch << *(ifSearch + 1) << *(ifSearch + 2) << std::endl;
}
else
{
std::cout << "未找到" << std::endl;
}
auto iter = search_n(vec.begin(), vec.end(), 2, 2);
std::cout << *iter << *(iter + 1) << *(iter + 2) << std::endl;
}
int main()
{
basicSerch();
return 0;
}
- 运行结果
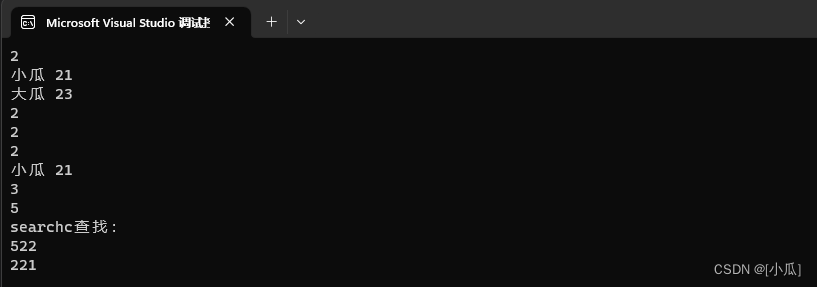
统计查找
- count:区间统计
- count_if:条件统计
- equal:相等比较
#include <iostream>
#include <algorithm>
#include <vector>
class student{
public:
student(std::string name = "", int age = 0) :name(name), age(age) {}
std::string getName() { return name; }
int getAge() { return age; }
bool operator==(const std::string& name)
{
return this->name == name;
}
bool operator==(const int& age)
{
return this->age == age;
}
protected:
std::string name;
int age;
};
void statisticSearch()
{
std::vector<student> vec2{ {"小瓜",21},{"大瓜",23},{"傻瓜",22} };
std::vector<int> vec = { 1,3,4,6,2,8,9,5,2,2,1,1,1 };
std::cout << count(vec.begin(), vec.end(), 1) << std::endl;
std::cout <<"大于8的数字有多少个:" <<
count_if(vec.begin(), vec.end(), [](int& score) {return score > 8; }) << std::endl;
std::cout << "大于20岁的有多少个:" <<
count_if(vec2.begin(), vec2.end(), [](student& object) {return object.getAge() > 20; }) << std::endl;
std::vector<int> one = { 1,2,3,1,2 };
std::vector<int> two = { 1,2,3,1,2 };
std::cout << std::endl << std::boolalpha <<
equal(one.begin(), one.end(), two.begin(), two.end()) << std::endl;;
}
int main()
{
statisticSearch();
return 0;
}
- 运行结果
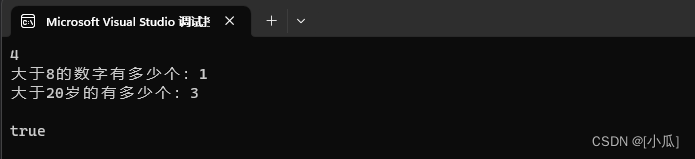
有序查找
- binary_search:二分查找
- upper_bound:查找最后一个大于查找值
- lowe_bound:大于等于查找值
- equal_range:区间比较
#include <iostream>
#include <algorithm>
#include <vector>
void orderedSearch()
{
std::vector<int> vec = { 1,2,3,4,5,6,7,7,8,9 };
std::cout << std::boolalpha << binary_search(vec.begin(), vec.end(), 5) << std::endl;
std::cout << "大于:" << *upper_bound(vec.begin(), vec.end(), 8) << std::endl;
std::cout << "大于等于:" << *lower_bound(vec.begin(), vec.end(), 8) << std::endl;
std::cout << "first等于:" << *equal_range(vec.begin(), vec.end(), 8).first << std::endl;
std::cout << "second大于:" << *equal_range(vec.begin(), vec.end(), 8).second << std::endl;
}
int main()
{
orderedSearch();
return 0;
}
- 运行结果
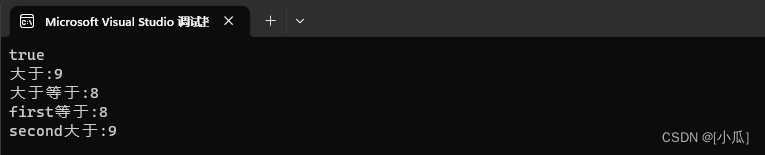
集合算法
- set_union:并集
- set_intersetction:交集
- set_difference:差集
- set_symmetric_difference:对称差集
#include <iostream>
#include <algorithm>
#include <vector>
void print(std::vector<int>& data)
{
for (auto v : data)
{
std::cout << v << " ";
}
std::cout << std::endl;
}
void setLookup()
{
std::vector<int> one{ 1,3,4,5 };
std::vector<int> tow{ 2,4,6,8 };
std::vector<int> unionSet;
std::set_union(one.begin(), one.end(), tow.begin(), tow.end(), std::back_inserter(unionSet));
print(unionSet);
std::vector<int> intersection;
std::set_intersection(one.begin(), one.end(), tow.begin(), tow.end(), std::back_inserter(intersection));
print(intersection);
std::vector<int> difference;
std::set_difference(one.begin(), one.end(), tow.begin(), tow.end(), std::back_inserter(difference));
print(difference);
std::vector<int> symmetric;
std::set_symmetric_difference(one.begin(), one.end(), tow.begin(), tow.end(), std::back_inserter(symmetric));
print(symmetric);
}
int main()
{
setLookup();
return 0;
}
- 运行结果
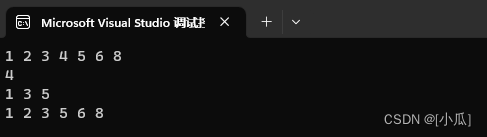
排序和通用类算法
- sort:排序
- stable_sort:保持相对顺序排序
- merge:归并排序
- inplace_merge:归并排序,直接作用在原容器上
- nth_element:关键字排序
- partition:分类处理,把条件下的数据分为两部分
- stable_partion:保持数据相对顺序,分类处理
- partial_sort:局部排序
- partial_sort_copy:局部排序结果放到新容器中
- random_shuffle:乱序算法
- reverse:逆序
- reverse_copy:逆序排序另存一个容器
- rotate:移动元素到末尾
- rotate_copy:移动元素到末尾的结果另存
#include <iostream>
#include <algorithm>
#include <vector>
#include <list>
#include <ctime>
class student
{
public:
student(std::string name = "", int age = 0) :name(name), age(age) {}
std::string getName()const { return name; };
int getAge()const { return age; };
protected:
std::string name;
int age;
};
void universalSort()
{
std::vector<int> vec1{ 1,2,3,5,7,8,4,6,10,9 };
std::sort(vec1.begin(), vec1.end(), std::less<int>());
copy(vec1.begin(), vec1.end(), std::ostream_iterator<int>(std::cout, " "));
std::cout << std::endl;
std::sort(vec1.begin(), vec1.end(), std::greater<int>());
copy(vec1.begin(), vec1.end(), std::ostream_iterator<int>(std::cout, " "));
std::cout << std::endl;
std::list<int> li = { 1,3,5,7,9,2,4,6,8,10 };
li.sort(std::less<int>());
copy(li.begin(), li.end(), std::ostream_iterator<int>(std::cout, " "));
std::cout << std::endl;
std::vector<student> xiaogua = { {"1小瓜",21},{"3小傻",23},{"2小姐",22} };
std::sort(xiaogua.begin(), xiaogua.end(), [](const student& one, const student& two)
{return one.getAge() < two.getAge(); });
for (auto v : xiaogua)
{
std::cout << v.getName() << "\t" << v.getAge() << std::endl;
}
std::cout << std::endl;
std::sort(xiaogua.begin(), xiaogua.end(), [](const student& one, const student& two)
{return one.getName() < two.getName(); });
for (auto v : xiaogua)
{
std::cout << v.getName() << "\t" << v.getAge() << std::endl;
}
std::vector<double> dNum = { 1.11,3.22,3.21,1.23,1.45,4.55,5.43 };
stable_sort(dNum.begin(), dNum.end(), [](const double& a, const double& b){return int(a) < int(b); });
std::cout << std::endl;
copy(dNum.begin(), dNum.end(), std::ostream_iterator<double>(std::cout, " "));
std::cout << std::endl;
std::vector<int> first = { 1,3,5 };
std::vector<int> second = { 2,4,6,8 };
std::vector<int> order;
merge(first.begin(), first.end(), second.begin(), second.end(), std::back_inserter(order));
copy(order.begin(), order.end(), std::ostream_iterator<int>(std::cout, " "));
std::cout << std::endl;
std::vector<int> value = { 1,3,5,7,9,2,4,6,8,10 };
inplace_merge(value.begin(), value.begin() + 5, value.end());
copy(value.begin(), value.end(), std::ostream_iterator<int>(std::cout, " "));
std::cout << std::endl;
std::vector<int> element = { 1,3,5,7,9,2,4,6,8,0 };
nth_element(element.begin(), element.begin() + 4, element.end());
copy(element.begin(), element.end(), std::ostream_iterator<int>(std::cout, " "));
std::cout << std::endl;
std::vector<int> e = { 1,3,5,7,9,0,2,4,6,8 };
partition(e.begin(), e.end(), [](int data) {return data < 6; });
copy(e.begin(), e.end(), std::ostream_iterator<int>(std::cout, " "));
std::cout << std::endl;
std::vector<double> dNum1 = { 1.11,3.22,3.21,1.23,1.45,4.55,5.43 };
stable_partition(dNum1.begin(), dNum1.end(), [](const double& a)
{return int(a) < 2; });
std::cout << std::endl;
copy(dNum1.begin(), dNum1.end(), std::ostream_iterator<double>(std::cout, " "));
std::cout << std::endl;
std::vector<int> testData = { 88,77,45,18,19,65,34 };
partial_sort(testData.begin(), testData.begin() + 3, testData.end());
std::cout << std::endl;
copy(testData.begin(), testData.end(), std::ostream_iterator<int>(std::cout, " "));
std::cout << std::endl;
std::vector<int> presult(3);
partial_sort_copy(testData.begin(), testData.begin() + 3,
presult.begin(), presult.end());
std::cout << std::endl;
copy(presult.begin(), presult.end(), std::ostream_iterator<int>(std::cout, " "));
std::cout << std::endl;
std::string str = "XiaoGua";
random_shuffle(str.begin(), str.end());
std::cout << str << std::endl;
reverse(str.begin(), str.end());
std::cout << str << std::endl;
std::string strresult;
reverse_copy(str.begin(), str.end(), std::back_inserter(strresult));
std::cout << str << std::endl;
std::cout << strresult << std::endl;
std::string info = { "XiaoGua" };
rotate(info.begin(), info.begin() + 4, info.end());
std::cout << info << std::endl;
std::string rotateStr;
rotate_copy(info.begin(), info.begin() + 4, info.end(), std::back_inserter(rotateStr));
std::cout << rotateStr << std::endl;
}
int main()
{
srand((unsigned int)time(NULL));
universalSort();
return 0;
}
- 运行结果
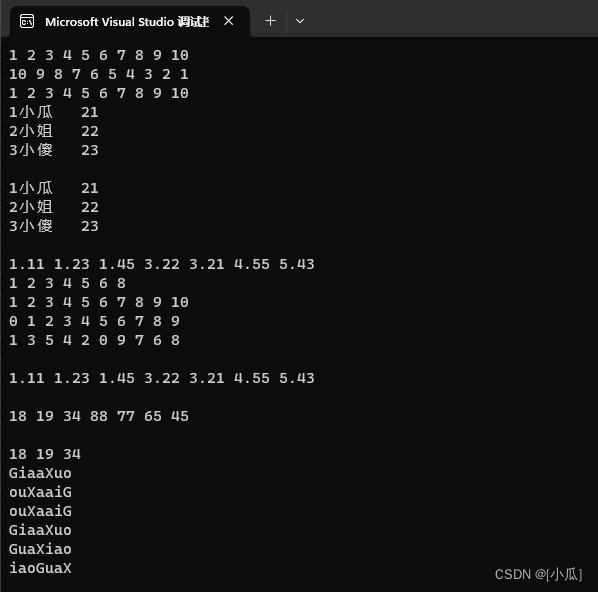