思维导图
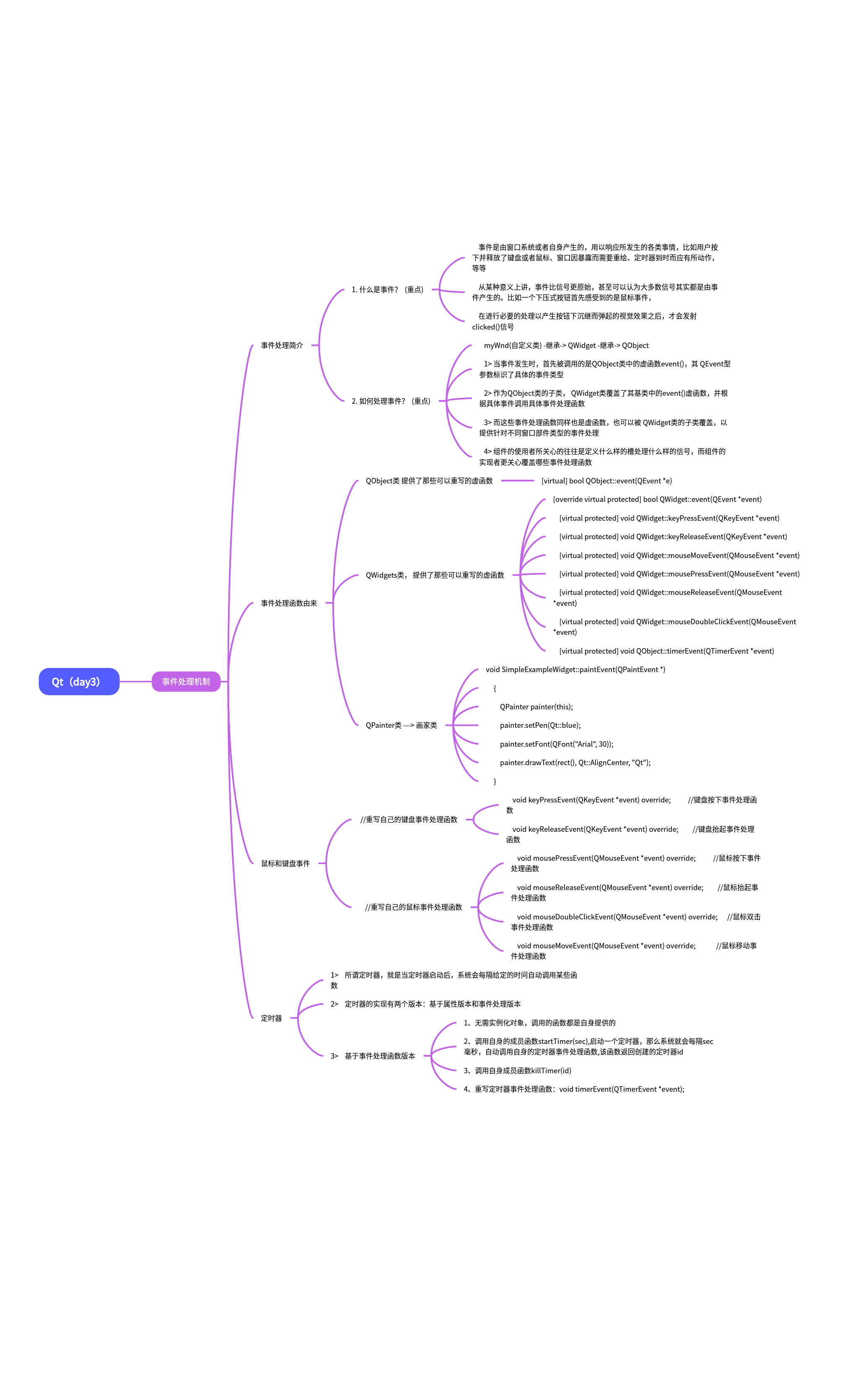
小练习
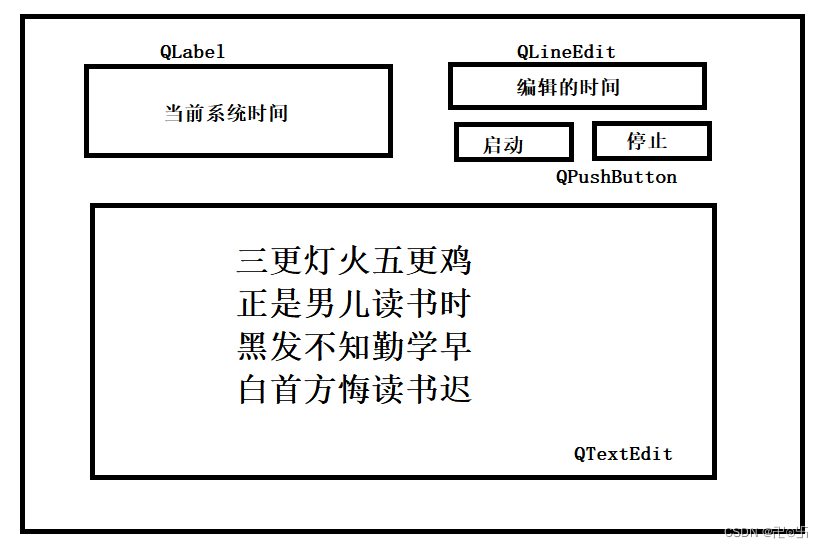
second.h
#ifndef SECOND_H
#define SECOND_H
#include <QWidget>
#include<QLabel>
#include<QLineEdit>
#include<QPushButton>
#include<QTimerEvent>
#include<QTime>
#include<QTextEdit>
#include<QTextToSpeech>
namespace Ui {
class Second;
}
class Second : public QWidget
{
Q_OBJECT
public slots:
void jump_slot();
void startbtn_slot();
void endbtn_slot();
public:
explicit Second(QWidget *parent = nullptr);
~Second();
void timerEvent(QTimerEvent *event) override;
private:
Ui::Second *ui;
QLabel *lab;
QLineEdit *ledit;
QPushButton *startbtn;
QPushButton *endbtn;
QTextEdit *tedit;
int timer_id; //定时器id
QTextToSpeech *speecher;
};
#endif // SECOND_H
second.cpp
#include "second.h"
#include "ui_second.h"
Second::Second(QWidget *parent) :
QWidget(parent),
ui(new Ui::Second)
{
ui->setupUi(this);
speecher =new QTextToSpeech(this); //为播报员实例化空间
this->setFixedSize(QSize(800,700)); //固定文件框的大小
this->setWindowTitle("定时器"); //设置文件的标题
lab=new QLabel(this); //创建一个lab
lab->resize(300,220); //设置lab大小
lab->move(50,50); //设置lab的位置
lab->setStyleSheet("background-color:skyblue;font-weight:bold;font-size:60px;");
ledit=new QLineEdit(this); //创建一个ledit
ledit->resize(300,100); //设置ledit大小
ledit->move(lab->x()+400,lab->y()); //设置ledit的位置
startbtn=new QPushButton(this); //创建一个startbtn
startbtn->resize(140,100); //设置startbtn大小
startbtn->move(ledit->x(),ledit->y()+120); //设置startbtn的位置
startbtn->setText("start"); //设置startbtn的文本
endbtn=new QPushButton(this); //创建一个endbtn
endbtn->resize(140,100); //设置endbtn大小
endbtn->move(ledit->x()+160,ledit->y()+120); //设置endbtn的位置
endbtn->setText("end"); //设置endbtn的文本
tedit=new QTextEdit(this); //创建一个tedit
tedit->resize(700,300);
tedit->move(lab->x(),lab->y()+300);
connect(startbtn,&QPushButton::clicked,this,&Second::startbtn_slot);
connect(endbtn,&QPushButton::clicked,this,&Second::endbtn_slot);
}
Second::~Second()
{
delete ui;
}
void Second::jump_slot(){
this->show();
}
void Second::startbtn_slot(){
timer_id=this->startTimer(1000);
}
void Second::endbtn_slot(){
this->killTimer(timer_id);
}
void Second::timerEvent(QTimerEvent *event){
QTime sys_t=QTime::currentTime();
QString t=sys_t.toString("hh:mm:ss");
if(event->timerId()==timer_id){
lab->setText(t);
}
if(t==ledit->text()){
speecher->say(tedit->toPlainText());
}
}
效果图:
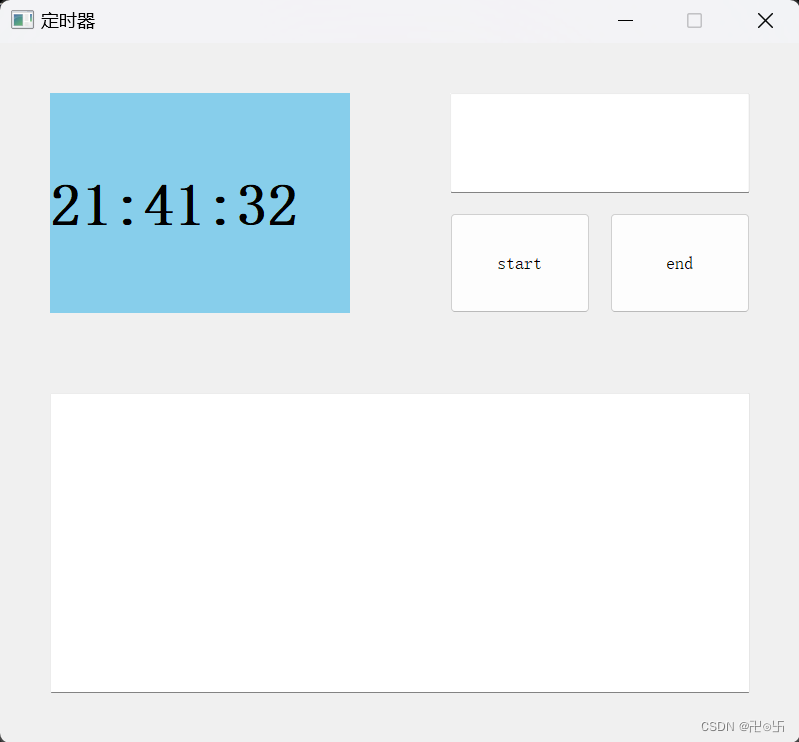
文本编辑器的保存工作
widget.h
#ifndef WIDGET_H
#define WIDGET_H
#include <QWidget>
#include<QFontDialog> //字体对话框类
#include<QFont> //字体类
#include<QMessageBox>
#include<QColorDialog> //颜色对话框类
#include<QColor> //颜色类
#include<QFileDialog> //文件对话框类
#include<QDebug>
#include<QFile> //文件类
QT_BEGIN_NAMESPACE
namespace Ui { class Widget; }
QT_END_NAMESPACE
class Widget : public QWidget
{
Q_OBJECT
public:
Widget(QWidget *parent = nullptr);
~Widget();
private slots:
void on_fontBtn_clicked();
void on_colorBtn_clicked();
void on_openBtn_clicked();
void on_saveBtn_clicked();
private:
Ui::Widget *ui;
};
#endif // WIDGET_H
weight.cpp
#include "widget.h"
#include "ui_widget.h"
Widget::Widget(QWidget *parent)
: QWidget(parent)
, ui(new Ui::Widget)
{
ui->setupUi(this);
}
Widget::~Widget()
{
delete ui;
}
//字体按钮对应的槽函数
void Widget::on_fontBtn_clicked()
{
bool ok; //返回用户是否选中字体的变量
//直接调用静态成员函数调出字体对话框
QFont f = QFontDialog::getFont(&ok, //返回是否选中字体
QFont("隶书", 2, 10, false), //初始字体
this, //父组件
"字体对话框"); //对话框标题
//要对ok进行判断,如果用户点击的取消,则ok为false,否则为true
if(ok)
{
//说明用户选中了字体,直接使用字体即可
//将该字体加载到文本上面
//ui->textEdit->setFont(f); //将该组件中的全部字体进行设置新字体
ui->textEdit->setCurrentFont(f); //将组件中当前选中的字体进行设置,其余字体不设置
}else
{
//说明用户没有选中字体
QMessageBox::information(this,"提示","用户没有选中字体");
}
}
//颜色按钮对应的槽函数
void Widget::on_colorBtn_clicked()
{
QColor c = QColorDialog::getColor(QColor(0,255,255), //初始颜色
this, //父组件
"选择颜色"); //窗口标题
//有没有选中颜色
if(c.isValid())
{
//说明该颜色合肥,直接使用
//ui->textEdit->setTextColor(c); //设置字体的颜色,前景色
ui->textEdit->setTextBackgroundColor(c); //设置字体背景色
}else
{
//说明颜色没有选中
QMessageBox::information(this,"提示","用户没有选中颜色");
}
}
//打开文件按钮对应的槽函数
void Widget::on_openBtn_clicked()
{
QString fileName = QFileDialog::getOpenFileName(this, //父组件
"选中文件", //对话框标题
"./", //起始路径
"all(*.*);; Img(*.png *.jpg *.xpm);; 文本(*.txt)");//过滤器
qDebug()<<fileName;
//文件操作
//1、实例化一个文件对象
QFile file(fileName); //使用有参构造构造一个文件对象
if(!file.exists()) //判断文件是否存在
{
return;
}
//2、打开文件
if(!file.open(QFile::ReadWrite)) //判断文件是否打开成功
{
return;
}
//3、从文件中读取数据
QByteArray msg = file.readAll();
//4、关闭文件
file.close();
//将从文件中读取的内容展示到ui界面上
ui->textEdit->setText(QString::fromLocal8Bit(msg));
}
//保存按钮对应的槽函数
void Widget::on_saveBtn_clicked()
{
//获取路径
QString fileName = QFileDialog::getSaveFileName();
//实例化文件对象
QFile file(fileName);
//打开文件
if(!file.open(QFile::WriteOnly))
{
return;
}
//将ui界面上的数据读取下来
QString msg = ui->textEdit->toPlainText();
//将信息写入到文件中
file.write(msg.toLocal8Bit());
//关闭文件
file.close();
}
效果图: