在My_string类的基础上,完成运算符重载
算术运算符:+
赋值运算符:+=
下标运算符:[]
关系运算符:>、<、==、!=、>=、<=
插入提取运算符:<<、>>
要求:注意数据的保护(const)
my_string.cpp
#include <iostream>
#include<string.h>
#include<my_string.h>
using namespace std;
//无参构造默认长度为15
My_string:: My_string():size(15)
{
data = new char[size];
data[0]='\0';
}
//有参构造
My_string::My_string(const char *str):size(15)
{
size_t len=strlen(str);
//判断字符串是否大于默认大小
//大于则增加默认大小
if((len+1)/15==0)
{
data = new char[size];
}
else if(15/(len+1)==0)
{
int num=(len+1)/15;
data=new char[size*=(num+1)];
}
strcpy(data,str);
}
My_string::My_string(int n, char ch):size(15)
{
//判断字符串是否大于默认大小
//大于则增加默认大小
if((n+1)/15==0)
{
data = new char[size];
}
else if(15/(n+1)==0)
{
int num=(n+1)/15;
data=new char[size*=num];
}
for(int i=0;i<n;i++)
{
data[i]=ch;
}
}
//析构函数
My_string::~My_string()
{
delete []data;
}
//拷贝构造函数
My_string::My_string(const My_string &my):size(15)
{
size_t len=strlen(my.data);
//判断字符串是否大于默认大小
//大于则增加默认大小
if((len+1)/15==0)
{
data = new char[size];
}
else if(15/(len+1)==0)
{
int num=(len+1)/15+1;
data=new char[size*=(num+1)];
}
strcpy(data,my.data);
}
//拷贝赋值函数相当于赋值运算符重载
My_string &My_string::operator=(const My_string &my)
{
//先释放原来的空间
delete []data;
data=nullptr;
//在申请与my一样大的空间
data=new char[my.size];
//最后在复制
strcpy(data,my.data);
size=my.size;
return *this;
}
//算术运算符重载:+
My_string My_string:: operator+(const My_string &my)const
{
My_string temp;
//先复制后拼接
strcpy(temp.data,this->data);
strcat(temp.data,my.data);
return temp;
}
// 赋值运算符:+=
My_string & My_string::operator+=(const My_string &my)
{
//先保存原来空间的内容
char arr[this->size];
strcpy(arr,data);
//先释放原来的空间
delete []data;
data=nullptr;
//在申请与my加原来一样大的空间
data=new char[my.size+this->size];
//最后在先复制后拼接
strcpy(data,arr);
strcat(data,my.data);
//更改最大容量
size=my.size;
return *this;
}
//下标运算符:[]
char & My_string::operator[](int m)
{
return data[m];
}
//关系运算符:>
bool My_string::operator>(const My_string m)const
{
char *p=this->data;
char *q=m.data;
int len=-1;
while(*p==*q&&*p!='\0'&&*q!='\0')
{
p++;
q++;
}
len=*p-*q;
cout<<len<<endl;
if(len<0)
{
return 0;
}
else if(len>=0)
{
return 1;
}
}
//关系运算符:<
bool My_string::operator<(const My_string m)const
{
char *p=this->data;
char *q=m.data;
int len=-1;
while(*p==*q&&*p!='\0'&&*q!='\0')
{
p++;
q++;
}
len=*p-*q;
cout<<len<<endl;
if(len<0)
{
return 1;
}
else if(len>=0)
{
return 0;
}
}
//关系运算符:==
bool My_string::operator==(const My_string m)const
{
char *p=this->data;
char *q=m.data;
int len=-1;
while(*p==*q&&*p!='\0'&&*q!='\0')
{
p++;
q++;
}
len=*p-*q;
cout<<len<<endl;
if(len!=0)
{
return 0;
}
else if(len==0)
{
return 1;
}
}
//关系运算符:!=
bool My_string::operator!=(const My_string m)const
{
char *p=this->data;
char *q=m.data;
int len=-1;
while(*p==*q&&*p!='\0'&&*q!='\0')
{
p++;
q++;
}
len=*p-*q;
cout<<len<<endl;
cout<<"len=="<<len<<endl;
if(len!=0)
{
return 1;
}
else if(len==0)
{
return 0;
}
}
//关系运算符:>=
bool My_string::operator>=(const My_string m)const
{
char *p=this->data;
char *q=m.data;
int len=-1;
while(*p==*q&&*p!='\0'&&*q!='\0')
{
p++;
q++;
}
len=*p-*q;
cout<<len<<endl;
if(len<=0)
{
return 0;
}
else if(len>0)
{
return 1;
}
}
//关系运算符:<=
bool My_string::operator<=(const My_string m)const
{
char *p=this->data;
char *q=m.data;
int len=-1;
while(*p==*q&&*p!='\0'&&*q!='\0')
{
p++;
q++;
}
len=*p-*q;
cout<<len<<endl;
if(len<=0)
{
return 0;
}
else if(len>0)
{
return 1;
}
}
//插入提取运算符:<<
ostream & operator<<(ostream &L, My_string &R)
{
L<<R.data;
return L;
}
//插入提取运算符:>>
istream & operator>>(istream &L, My_string &R)
{
L>>R.data;
return L;
}
//c_str函数
char* My_string::cc_s_str()
{
return data;
}
//size函数
int My_string::cc_size()
{
return strlen(data);
}
//empty函数
bool My_string::cc_empty()
{
return strlen(data)==0;
}
//at函数
char My_string::cc_at(size_t n)
{
//判断n是否合法
if(n<0||n>strlen(data))
{
cout<<"数组没有这个下标"<<endl;
return -1;
}
return data[n];
}
void My_string::show()
{
cout<<data<<endl;
}
my_string.h
#include <iostream>
#include<cstring>
#ifndef MY_STRING_H
#define MY_STRING_H
using namespace std;
class My_string
{
private:
char *data;
int size;
public:
//无参构造默认长度为15
My_string();
//有参构造
My_string(const char *str);
My_string(int n, char ch);
//析构函数
~My_string();
//拷贝构造函数
My_string(const My_string &my);
//拷贝赋值函数相当于赋值运算符重载
My_string & operator=(const My_string &my);
//算术运算符重载:+
My_string operator+(const My_string &my)const;
// 赋值运算符:+=
My_string & operator+=(const My_string &my);
//下标运算符:[]
char & operator[](int x);
//关系运算符:>
bool operator>(const My_string m)const;
//关系运算符:<
bool operator<(const My_string m)const;
//关系运算符:==
bool operator==(const My_string m)const;
//关系运算符:!=
bool operator!=(const My_string m)const;
//关系运算符:>=
bool operator>=(const My_string m)const;
//关系运算符:<=
bool operator<=(const My_string m)const;
//插入提取运算符:<<
friend ostream & operator<<(ostream &L, My_string &R);
//插入提取运算符:>>
friend istream & operator>>(istream &L, My_string &R);
//c_str函数
char* cc_s_str();
//size函数
int cc_size();
//empty函数
bool cc_empty();
//at函数
char cc_at(size_t n);
void show();
};
#endif // MY_STRING_H
main.cpp
#include <iostream>
#include<cstring>
#include<my_string.h>
using namespace std;
int main()
{
// //调用有参构造
// My_string m(5,'w');
// m.show();
// //调用有参构造
// My_string m1("zhangs");
// m1.show();
// //调用拷贝构造
// My_string m2=m1;
// m2.show();
// //拷贝赋值
// My_string m3;
// m3=m;
// m3.show();
// //c_str函数:返回c风格的字符串指针
// My_string m4("hhhhhh");
// printf("%s\n",m4.cc_s_str());
// //size函数:返回字符串实际长度
// My_string m5(5,'*');
// printf("m5的长度:%d\n",m5.cc_size());
// //empty函数:判断是否是空串
// My_string m6;
// if(m6.cc_empty())
// {
// cout<<"是"<<endl;
// }
// else if(m6.cc_empty())
// {
// cout<<"否"<<endl;
// }
// //at函数
// My_string m7=m1;
// m7.show();
// cout<<m7.cc_at(-1)<<endl;
// cout<<m7.cc_at(8)<<endl;
// cout<<m7.cc_at(3)<<endl;
算术运算符重载:+
My_string p1("ads");
My_string p2="ads";
My_string p3;
p3=p1+p2;
cout<<p3<<endl;
// 赋值运算符:+=
p3+=p1;
cout<<p1<<endl;
//下标运算符:[]
cout<<p1[3]<<endl;
//关系运算符:>
if(p1>p2)
{
cout<<"p1大"<<endl;
}
else
{
cout<<"p2大"<<endl;
}
if(p1!=p2)
{
cout<<"p1!=p2"<<endl;
}
else
{
cout<<"p1=p2"<<endl;
}
return 0;
}
运行结果
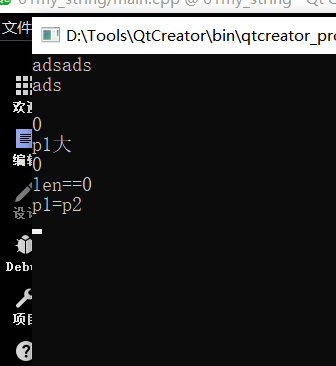