map、unordered_map与multimap
map
简介
- 关联容器。
- 键值对的映射(key - value)。key作为唯一标识,不能重复,但是value可以。
- 按照键值有序排列(默认从小到大)。底层实现为红黑树,查询效率为O(logN)。
常用操作
- clear():删除所有元素
- empty():当map为空时返回真
- begin():返回一个指向map头部的迭代器
- end():返回一个指向map尾部的迭代器
- rbegin():返回一个指向map尾部的逆向迭代器
- rend():返回一个指向map头部的逆向迭代器
- size():返回元素的个数
- count():返回指定元素的个数
- erase():删除一个元素
- find():查找一个元素
- insert():插入一个元素
#include<cstdio>
#include<cstdlib>
#include<string>
#include<iostream>
#include<map>
using namespace std;
map<int, string> pa;
int main(){
pa.insert(make_pair<int, string>(2, "小红"));
pa.insert(make_pair<int, string>(3, "小明"));
pa.insert(make_pair<int, string>(1, "小王"));
cout << "------------map的顺序遍历-------------" << endl;
for(map<int, string>::iterator it = pa.begin(); it != pa.end(); it++){
cout << it -> first << " " << it -> second << endl;
}
cout << "--------------------------------------" << endl;
cout << "map的所含元素个数为:" << pa.size() << endl;
cout << "map中键值等于2的元素个数为:" << pa.count(2) << endl;
pa.erase(2);
cout << "map中键值等于2的元素个数为:" << pa.count(2) << endl;
pa.insert(make_pair<int, string>(2, "小红"));
cout << "------------map的逆向遍历-------------" << endl;
for(map<int, string>::reverse_iterator it = pa.rbegin(); it != pa.rend(); it++){
cout << it -> first << " " << it -> second << endl;
}
cout << "--------------------------------------" << endl;
pa.clear();
cout << "map所含元素个数为:" << pa.size() << endl;
pa[2] = "xiaohong";
pa[3] = "xiaoming";
pa[1] = "xiaowang";
map<int, string>::iterator it = pa.find(3);
cout << it -> first << " " << it -> second << endl;
cout << "3 " << pa[3] << endl;
return 0;
}
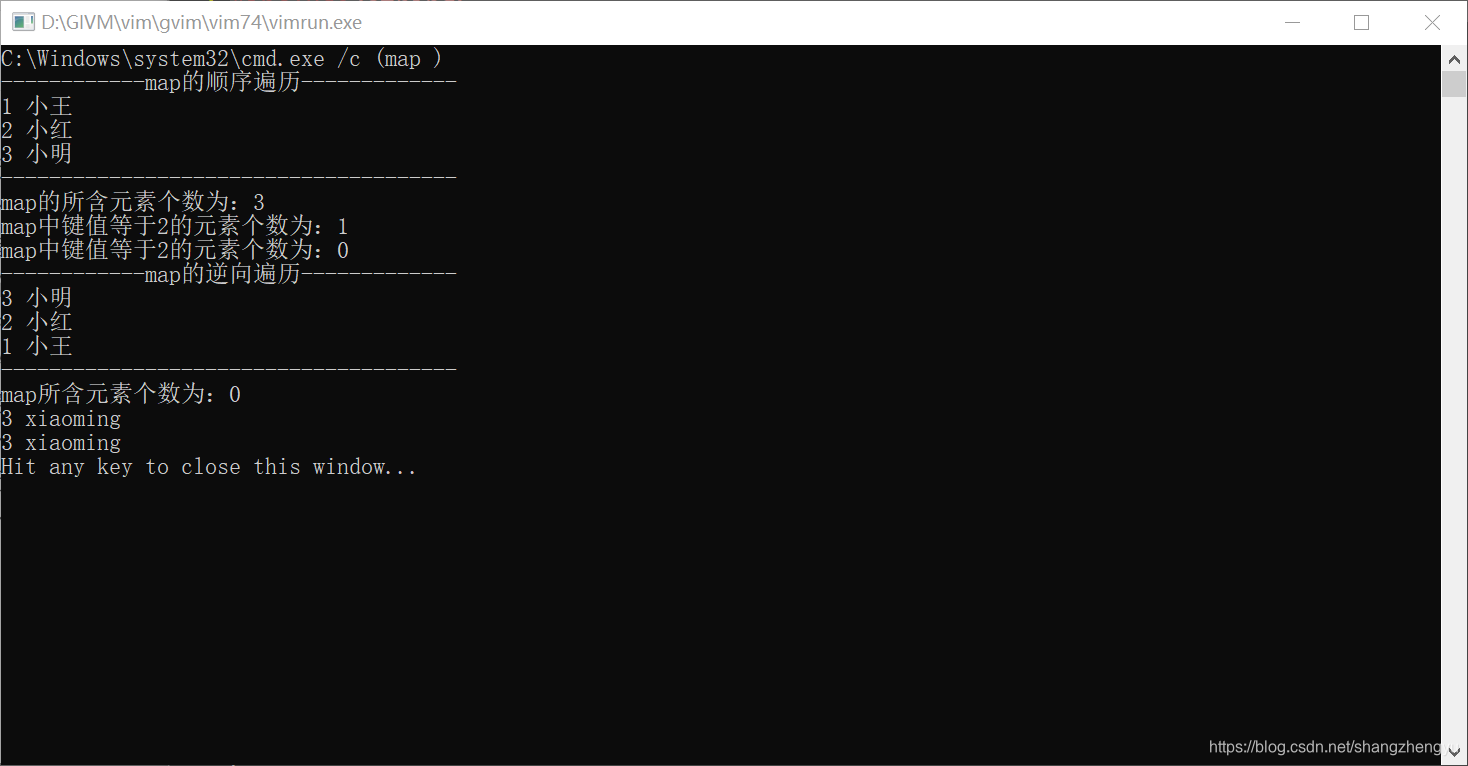
unordered_map
简介
- 无序映射,底层实现为哈希表,查询效率为O(1)。
- 空间复杂度比map高。
常用操作
- clear():删除所有元素
- empty():当map为空时返回真
- begin():返回一个指向map头部的迭代器
- end():返回一个指向map尾部的迭代器
- cbegin():返回一个指向map头部的常量迭代器
- cend():返回一个指向map尾部的常量迭代器
- size():返回元素的个数
- count():返回指定元素的个数
- erase():删除一个元素
- find():查找一个元素
- insert():插入一个元素
#include<cstdio>
#include<cstdlib>
#include<string>
#include<iostream>
#include<unordered_map>
using namespace std;
unordered_map<int, string> pa;
int main(){
pa.insert(make_pair<int, string>(2, "小红"));
pa.insert(make_pair<int, string>(3, "小明"));
pa.insert(make_pair<int, string>(1, "小王"));
for(auto it = pa.begin(); it != pa.end(); it++){
cout << it -> first << " " << it -> second << endl;
}
cout << "unordered_map的所含元素个数为:" << pa.size() << endl;
cout << "unordered_map中键值等于2的元素个数为:" << pa.count(2) << endl;
pa.erase(2);
cout << "unordered_map中键值等于2的元素个数为:" << pa.count(2) << endl;
pa.insert(make_pair<int, string>(2, "小红"));
for(auto it = pa.cbegin(); it != pa.cend(); it++){
cout << it -> first << " " << it -> second << endl;
}
pa.clear();
cout << "unordered_map的所含元素个数为:" << pa.size() << endl;
pa[2] = "xiaohong";
pa[3] = "xiaoming";
pa[1] = "xiaowang";
auto it = pa.find(3);
cout << it -> first << " " << it -> second << endl;
cout << "3 " << pa[3] << endl;
return 0;
}
multimap
简介
- 与map基本上很相似,只是multimap允许键值重复。
- 没有重载operator[],不支持像数组一样进行取值和访问。
- 按照键值有序排列(默认从小到大)。底层实现为红黑树,查询效率为O(logN)。
#include<cstdio>
#include<cstdlib>
#include<string>
#include<iostream>
#include<map>
using namespace std;
multimap<int, string> pa;
int main(){
pa.insert(make_pair<int, string>(2, "小红"));
pa.insert(make_pair<int, string>(2, "小红"));
pa.insert(make_pair<int, string>(3, "小明"));
pa.insert(make_pair<int, string>(1, "小王"));
cout << "------------map的顺序遍历-------------" << endl;
for(map<int, string>::iterator it = pa.begin(); it != pa.end(); it++){
cout << it -> first << " " << it -> second << endl;
}
cout << "--------------------------------------" << endl;
cout << "map的所含元素个数为:" << pa.size() << endl;
cout << "map中键值等于2的元素个数为:" << pa.count(2) << endl;
pa.erase(2);
cout << "map中键值等于2的元素个数为:" << pa.count(2) << endl;
pa.insert(make_pair<int, string>(2, "小红"));
cout << "------------map的逆向遍历-------------" << endl;
for(map<int, string>::reverse_iterator it = pa.rbegin(); it != pa.rend(); it++){
cout << it -> first << " " << it -> second << endl;
}
cout << "--------------------------------------" << endl;
pa.clear();
cout << "map所含元素个数为:" << pa.size() << endl;
pa.insert(make_pair<int, string>(2, "小红"));
pa.insert(make_pair<int, string>(2, "小红"));
pa.insert(make_pair<int, string>(3, "小明"));
pa.insert(make_pair<int, string>(1, "小王"));
map<int, string>::iterator it = pa.find(3);
cout << it -> first << " " << it -> second << endl;
return 0;
}
map与unordered_map的比较
- 底层实现为红黑树,因此是有序的键值对映射,查找的效率为O(logN)。
- 空间占用率高,因为map底层用红黑树进行实现的,所以每个节点会保存一些红黑树的性质,导致占用大量的空间。
- 底层实现为哈希表,所以是无序的键值对映射,查找的效率为O(1)。
- 哈希表的建立需要耗费大量的时间。