网络编程异步
重点(Top highlight)
Asynchronous programming is not a new concept and has been around for several years and it is nowadays a new standard in modern frameworks like AspNetCore. This framework is now fully asynchronous and it's not easy to avoid using its async keyword.
异步编程并不是一个新概念,它已经存在了好几年,如今它已成为AspNetCore等现代框架中的新标准。 现在,该框架是完全异步的,要避免使用其async关键字并非易事。
I think there is a certain cloud of fear when it comes to asynchronism, developers sometimes feel afraid of using it because they don’t understand how to use it and its best practices.
我认为异步方面存在一定的恐惧感,开发人员有时会害怕使用它,因为他们不了解如何使用它及其最佳实践。
Another reason for this sense of fear is due to the fact that in the past asynchronous programming was not easy at all indeed. Since the introduction of async/await keywords in C# 5 asynchronous programming has become much easier to work with.
这种恐惧感的另一个原因是,在过去,异步编程的确根本不容易。 自从C#5中引入async / await关键字以来,异步编程就变得更加容易使用。
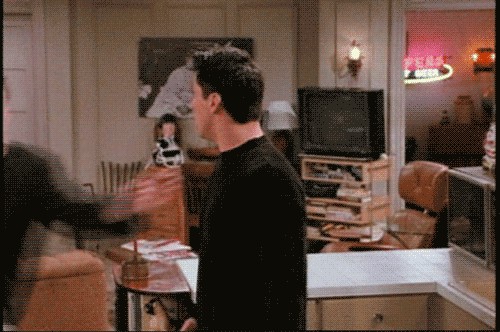
If you are afraid of asynchronism you have come to the right place, there is no need to jump up to me! In this article, I’ll present you with some of the best practices when it comes to async programming in .NET and you’ll see that's not that big of a deal you might think it is.
如果您担心异步问题,那么您来对地方了,您无需跳到我身边! 在本文中,我将向您介绍有关.NET中异步编程的一些最佳实践,并且您会发现这并不是您想的那么重要。
什么是异步编程? (What is Asynchronous Programming?)
“Asynchronous programming is a means of parallel programming in which a unit of work runs separately from the main application thread and notifies the calling thread of its completion, failure or progress.” — Source
“ 异步编程是并行编程的一种方式,其中一个工作单元与主应用程序线程分开运行,并向调用线程通知其完成,失败或进度。” —来源
The core of asynchronous programming are theTask
objects, which model asynchronous operations. They are supported by the async
and await
keywords.
异步编程的核心是Task
对象,它们对异步操作进行建模。 它们由async
和await
关键字支持。
The await keyword is where the magic happens. It yields control to the caller of the method that performed await, and it ultimately allows a service to be elastic.
await关键字是神奇的地方。 它将控制权交给执行await的方法的调用者,最终使服务具有弹性。
一路异步 (Async all the way)
When using asynchronous programming you should use it all the way, this means that all your callers should be async. The effort in asynchronous programming can be worthless if you don’t apply it all the way in your code.
当使用异步编程时,应该完全使用它,这意味着所有调用者都应该是异步的。 如果您不完全在代码中应用异步编程,那么付出的努力可能是毫无价值的。
In some cases partially asynchronism can outcome worst results that fully synchronous programming, therefore my advice is to go asynchronous all over your entire stack.
在某些情况下,部分异步可能会导致比完全同步编程更糟糕的结果,因此,我的建议是在整个堆栈中都进行异步处理。
This next piece of code is a good example where sync is preferable over async. Here the use of Task.Result
blocks the current thread to wait for the result.
下一段代码是一个很好的示例,其中,同步比异步更可取。 这里使用Task.Result
阻止当前线程等待结果。
public int MyMethodAsync()
{
var result = OtherMethodAsync().Result;
return result + 1;
}
The right way to do this is by using the await keyword to get the result:
正确的方法是使用await关键字来获取结果:
public async Task<int> MyMethodAsync()
{
var result = await OtherMethodAsync();
return result + 1;
}
只是不要返回异步虚空,相信我 (Just don’t return async void, trust me)
It’s never a good option to use to return void in an async method in .Net, never.
永远不要在异步方法中使用void返回void,这绝对不是一个好选择。
The most common situation where people feel like async void is a good idea is when implementing a fire-and-forget, it isn’t at all. If any exception is thrown in an async void method the process will be stoped.
人们通常认为异步无效是一个好主意,这是在实施“一劳永逸”时,它根本不是。 如果异步void方法中引发了任何异常,则该过程将停止。
So instead of using async void you can use the Task.Run
since if any unhandled exception happens the TaskScheduler.UnobservedTaskException
will be triggered.
因此,可以使用Task.Run
而不是使用异步void,因为如果发生任何未处理的异常,则会触发TaskScheduler.UnobservedTaskException
。
public void RunFireAndForgetOperation()
{
Task.Run(FireAndForgetAsync);
}public async Task FireAndForgetAsync()
{
var result = await RunOperationAsync();
ParseResult(result);
}
异步/等待任务返回 (Async/await over Task return)
Please take this one with a pinch of salt because when it comes to performance, returning directly a Task
is faster because there is no computation done in the async state machine but with this, you can end up not taking advantage of the async state machine benefits. The StateMachine is a struct created when using the async/await keywords.
请花一点时间,因为在性能方面,直接返回一个Task
会更快,因为异步状态机中没有进行任何计算,但是这样做最终可能无法利用异步状态机的优势。 StateMachine是使用async / await关键字创建的结构。
So there are several benefits when using the async/await keywords:
因此,使用async / await关键字有几个好处:
- Asynchronous and synchronous exceptions are normalized to always be asynchronous; 异步和同步异常被规范化为总是异步的。
Exceptions thrown will be automatically wrapped in the returned
Task
instead of surprising the caller with an actual exception;抛出的异常将自动包装在返回的
Task
而不会使调用者感到实际异常。- The code is easier to modify; 该代码更易于修改;
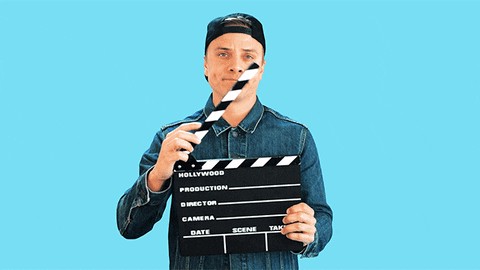
To wrap up, these are some practices that you should follow when it comes to asynchronous programming and there are lots of great resources over the internet that you can use to know more about this subject and enhance your skills.
总结起来,这些是异步编程时应遵循的一些做法,并且互联网上有很多很棒的资源,您可以用来了解更多有关该主题的知识并提高技能。
Asynchronous programming can simultaneously be your best ally along with being your worst enemy, the key to success is to study and understand how to use it properly.
异步编程既可以成为最大的敌人,也可以同时成为您的最佳盟友,成功的关键是学习并了解如何正确使用它。
Remember to always look up to understanding how things really work on a deeper level and don’t try to just follow the patterns or you can end up in a Cargo Cult Anti-Pattern.
请记住,始终要了解事物在更深层次上的真实运作方式,不要试图仅仅遵循模式,否则您可能会遇到“货邪教反模式” 。
Nelson out!
尼尔森出去!
翻译自: https://medium.com/@nelsonparente/net-async-programming-in-a-nutshell-dc01c2e71a20
网络编程异步