Hello there, In this article, we are going to look for the best practice and simple steps to create a Restful API using Node.js and MySQL. The stuff we are going to use in this Restful API is Node.js, Express js, MySQL server, MySQL workbench.
大家好 ,在本文中,我们将寻找最佳实践和使用Node.js和MySQL创建Restful API的简单步骤。 我们将在此Restful API中使用的东西是Node.js,Express js,MySQL服务器,MySQL工作台。
What Is Restful API?
什么是Restful API?
Before getting into a Restful API. Let us know what is an API?
在进入Restful API之前。 让我们知道什么是API?
Application Program Interface (API) is a code used as an intermediator between two Software programs to communicate with each other. Rest API is the architectural style, that uses HTTP request to GET, PUT, POST and DELETE data in Database
应用程序接口(API)是用作两个软件程序之间相互通信的中介的代码。 Rest API是一种体系结构样式,它使用HTTP请求来对数据库中的GET,PUT,POST和DELETE数据进行处理
Installations
装置
Download and install the node.js from https://nodejs.org/en/
从https://nodejs.org/en/下载并安装node.js
MySQL server, MySQL workbench from https://dev.mysql.com/downloads/ After the installation follows the below step to execute.
MySQL服务器,来自https://dev.mysql.com/downloads/的 MySQL工作台,安装后,请执行以下步骤。
1. The First Step to Install npm Packages
1.安装npm软件包的第一步
Create a folder where you going to work on API creation. Then open a command terminal of that folder.
创建一个用于创建API的文件夹。 然后打开该文件夹的命令终端。
After that, enter the npm init and press enter. It will ask for certain details about the project if you needed to mention anything you can mention it otherwise keep it default and after it will create a package.json file.
之后,输入npm init,然后按Enter。 如果您需要提及任何可以提及的内容,它将询问有关该项目的某些详细信息,否则将其保留为默认值,然后它将创建一个package.json文件。
package.json
package.json
It is a JSON file used for managing the project dependencies, scripts, version, and a lot more.
这是一个JSON文件,用于管理项目依赖项,脚本,版本等。
Then install npm packages express, body-parser, Mysql by entering the following command in a command terminal
然后通过在命令终端中输入以下命令来安装npm软件包express,body-parser,Mysql
npm install — save express@4.17.1 mysql@2.18.1 body-parser@1.19.0
npm install-保存express@4.17.1 mysql@2.18.1 body-parser@1.19.0
After the installation checks, the packages are installed in package.json file it holds the data of installed packages.
安装检查后,软件包将安装在package.json文件中,其中包含已安装软件包的数据。
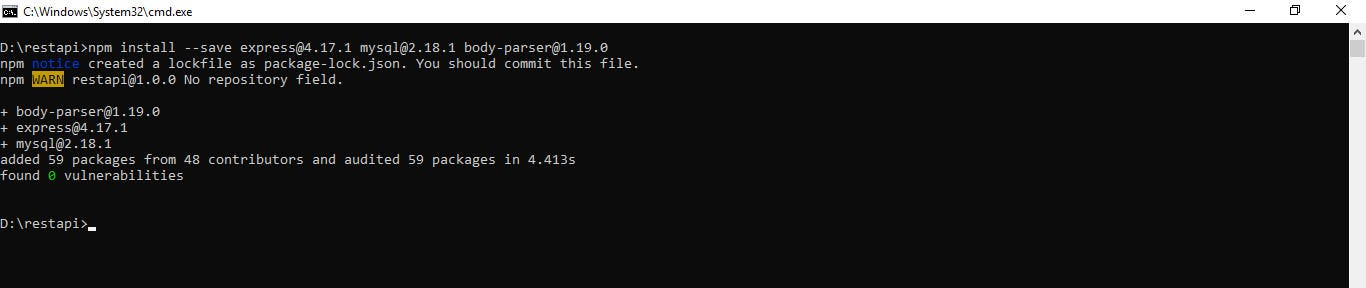
2. Create MySql Database(use Mysql Workbench)
2.创建MySql数据库(使用Mysql Workbench)
To create a database right-click on the schema panel and click on creating a schema. And provide a database and click apply. After that action database is created.
要创建数据库,请在模式面板上单击鼠标右键,然后单击创建模式。 并提供一个数据库,然后单击“应用”。 此后,将创建数据库。
And create a table click on the SQL query tab above the schema panel. And pass the below query to create an employee table.
然后在架构面板上方SQL查询选项卡上创建一个表。 并传递以下查询以创建一个雇员表。


Use employeedb;
使用employeedb;
CREATE TABLE `employee` (
创建表`employee`(
`EmpId` int(11) NOT NULL AUTO_INCREMENT,
`EmpId` int(11)不是NULL AUTO_INCREMENT,
`Name` varchar(45) DEFAULT NULL,
`Name` varchar(45)默认为NULL,
`EmpCode` varchar(45) DEFAULT NULL,
EmpCode varchar(45)默认为NULL,
PRIMARY KEY (`EmpId`)
主键(`EmpId`)
) ENGINE = InnoDB AUTO_INCREMENT=0 DEFAULT CHARSET=utf8mb4 COLLATE =utf8mb4_0900_ai_ci;
)ENGINE = InnoDB AUTO_INCREMENT = 0 DEFAULT CHARSET = utf8mb4 COLLATE = utf8mb4_0900_ai_ci;
Before getting into an database connection let us add an dummy data in database to get an structure. For that execute the following query
在进入数据库连接之前,让我们在数据库中添加虚拟数据以获取结构。 为此,执行以下查询
Use employeedb;
使用employeedb;
INSERT INTO `employee` VALUES (1, ‘Jhon Doe’, ‘EMP001’),(2, ‘Peter Parker’, ‘EMP002’),(3, ‘Kevin’, ‘EMP003’);
将值插入(employee)值中(1,'Jhon Doe','EMP001'),(2,'Peter Parker','EMP002'),(3,'Kevin','EMP003');

After that check whether a data is added in a table using the query
之后,使用查询检查是否在表中添加了数据
SELECT * FROM employeedb.employee
选择* FROM employeedb.employee
3. Database Connection
3.数据库连接
Create a root and main javascript file index.js. After that, to connect with the database we needed to import the MySQL package by calling require function and save it in a variable to access it anywhere in the file.
创建一个根目录和主要的javascript文件index.js。 之后,要连接数据库,我们需要通过调用require函数导入MySQL包,并将其保存在变量中以在文件中的任何位置访问它。
And give a login database details. After that execute a connect function to connect with the database. Use a CallBack function to throw an error if the connection does not execute.
并给出登录数据库的详细信息。 之后,执行连接功能以连接数据库。 如果连接未执行,请使用CallBack函数引发错误。
Important note: Do Reconfigure Authentication Method, there you select legacy type password support for 5.1(MySQL installer).
重要说明:请执行“重新配置身份验证方法”,在此选择5.1(MySQL安装程序)的旧式密码支持。
The below image shows the above-mentioned coding practice.
下图显示了上述编码实践。

4. API CREATION
4. API创建
Before getting into an API let us know about the express js. It is a well-known node js framework. It will provide more flexibility for the coder to develop a code in node js. Express js is a third party module.
在进入API之前,请让我们了解Express js。 这是一个著名的节点js框架。 这将为编码人员在节点js中开发代码提供更大的灵活性。 Express js是第三方模块。
To use the express js, initially import the express js and store it in a variable as you decide. We have now only imported the express js but we need to initialize the express. For that, we need to execute the express function as mentioned below.
要使用express js,请首先导入Express js并将其存储在您决定的变量中。 现在,我们仅导入了express js,但是我们需要初始化express。 为此,我们需要执行如下所述的express函数。
Then import a body-parser third-party module in index.js file and it is also called as a middleware. It means body-parser is work in between the post and get method of HTTP API. It will watch the CRUD function. And all the API requests are passed through a middleware. By using a body-parser(middleware) we can achieve which method is calling, whether GET or POST method is requested by a user. For that purpose only we are using body-parser.
然后将主体分析器第三方模块导入index.js文件,它也称为中间件。 这意味着body-parser在HTTP API的post和get方法之间工作。 它将监视CRUD功能。 并且所有API请求都通过中间件传递。 通过使用body-parser(middleware),我们可以实现正在调用哪个方法,无论用户是请求GET还是POST方法。 仅出于此目的,我们正在使用body解析器。
To use body-parser in API we need to call a middleware inside the express function. As we have mentioned below.
要在API中使用body-parser,我们需要在express函数内部调用中间件。 正如我们下面提到的。

Now we need to start a server for API to use. For that, we give a port number and we have the callback function to find the server is existing or not.
现在我们需要启动服务器以供API使用。 为此,我们提供一个端口号,并具有回调函数以查找服务器是否存在。
To fetch the data inside the database we needed to call the get function. we are going to pass the route value and callback function as a parameter. Inside the callback function, we are going to pass two parameters, they are request and response. This callback function invoked when the user requests the route. As we have mentioned below.
为了获取数据库内部的数据,我们需要调用get函数。 我们将传递路由值和回调函数作为参数。 在回调函数内部,我们将传递两个参数,它们是请求和响应。 用户请求路由时调用此回调函数。 正如我们下面提到的。

Inside the callback function, we are going to pass a query to fetch the data inside the database. Inside that, we are going to pass the SELECT query to fetch data. To save data from the database we are using another callback function. Inside the callback function, we are going to pass three parameters they are, err, row, fields.
在回调函数内部,我们将传递一个查询以获取数据库内部的数据。 在其中,我们将传递SELECT查询以获取数据。 为了从数据库保存数据,我们使用了另一个回调函数。 在回调函数中,我们将传递三个参数,即err,row,fields。
err throws the error and row shows the data inside the database. To print, the data(row) from the database we have to call the res.send() method. Inside the res.send method, we are going to pass the parameter in which one has data from the database. After that, it will show data(JSON) in the URL. To get that response please follow the code as mentioned below.
err引发错误,并且行显示数据库内部的数据。 要打印,数据库中的数据(行)我们必须调用res.send()方法。 在res.send方法内部,我们将传递参数,其中该参数具有来自数据库的数据。 之后,它将在URL中显示data(JSON)。 要获得该响应,请遵循以下代码。

cmd node index.js
cmd 节点index.js
After entering the coding in a command terminal then run the command by pressing the button Enter. Then we get a console message server is running in port number 3000 and DB is connected. It represents the database and the port is working properly.
在命令终端中输入编码后,请按Enter键运行命令。 然后,我们获得一个控制台消息服务器,该服务器在端口号3000中运行,并且DB已连接。 它代表数据库,端口正常运行。
Then pass the URL http://localhost:3000/employees in the browser. As a response, we get the array of an object which holds the data in the database. In other words, the data will be in JSON format. By using the JSON file we can get all data from a database to display.
然后传递URL http:// localhost:3000 / employees 在浏览器中。 作为响应,我们获得了一个对象数组,该数组将数据保存在数据库中。 换句话说,数据将为JSON格式。 通过使用JSON文件,我们可以从数据库中获取所有数据以进行显示。

I hope you enjoyed the small article of API Creations. Thank you.
希望您喜欢API Creations的小文章。 谢谢。
By Keerthi, Software Developer @ ABI-Tech Solution
作者: ABI-Tech Solution的软件开发人员Keerthi