commonjs模块
This is not meant to be a comprehensive overview of ES modules and CommonJS modules — I do not go into depth about what modules are and how they work. Rather, it is meant to highlight the differences between ES modules and CommonJS modules and how you can use them together.
这并不是要对ES模块和CommonJS模块进行全面的概述—我不会深入探讨什么是模块以及它们如何工作。 而是要强调ES模块和CommonJS模块之间的差异以及如何一起使用它们。
This is part two of a four part series.
这是四部分系列的第二部分。
- Using ES modules with CommonJS modules in Node.js 将ES模块与Node.js中的CommonJS模块一起使用
Check out full code examples here: https://github.com/arcticmatt/javascript_modules/tree/master/node.
在此处查看完整的代码示例: https : //github.com/arcticmatt/javascript_modules/tree/master/node 。
什么是模块? (What is a module?)
A module is just a single JavaScript file.
模块只是一个JavaScript文件。
package.json
“type
”字段 (package.json
“type
” field)
This field determines whether JavaScript files, i.e. files ending in .js
, are treated as ES modules or CommonJS modules.
该字段确定JavaScript文件(即以.js
结尾的文件)是否被视为ES模块或CommonJS模块。
ExampleSee the last field. Valid values are:
示例请参见最后一个字段。 有效值为:
"module"
"module"
"commonjs"
"commonjs"
If it is not specified, it implicitly defaults to "commonjs"
.
如果未指定,则默认为"commonjs"
。
{
"name": "es_module_playground",
"version": "1.0.0",
"description": "Testing ES Modules",
"main": "module1.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"author": "",
"license": "ISC",
"type": "commonjs"
}
"commonjs"
Behavior
"commonjs"
行为
.cjs
files are treated as CommonJS modules.cjs
文件被视为CommonJS模块.js
files are treated as CommonJS modules.js
文件被视为CommonJS模块.mjs
files are treated as ES modules.mjs
文件被视为ES模块
"module"
Behavior
"module"
行为
.cjs
files are treated as CommonJS modules.cjs
文件被视为CommonJS模块.js
files are treated as ES modules.js
文件被视为ES模块.mjs
files are treated as ES modules.mjs
文件被视为ES模块
As you can see, the difference is how .js
files are treated.
如您所见,区别在于如何处理.js
文件。
混合ES模块和CommonJS模块 (Mixing ES modules and CommonJS modules)
Here are the general rules regarding what doesn’t work, accompanied by relevant errors. “Doesn’t work” means that it results in an error if you run the code with node
from the command line.
以下是有关无效内容的一般规则, 并伴有相关错误。 “不起作用”表示如果在命令行中使用node
运行代码,则会导致错误。
Remember from above that, if "type": "module"
, then an ES module is any .js
or .mjs
file. If "type": "commonjs"
, then an ES module is any .mjs
file.
从上面记住,如果"type": "module"
,则ES模块是任何.js
或.mjs
文件。 如果"type": "commonjs"
,则ES模块是任何.mjs
文件。
You can only use
import
andexport
in an ES module. Specifically, this means you can only useimport
andexport
in a.mjs
file, or in a.js
file if"type": "module"
.您只能在ES模块中使用
import
和export
。 具体来说,这意味着您只能在.mjs
文件或.js
文件(如果为"type": "module"
使用import
和export
。You can only use
import
andexport
in an ES module. Specifically, this means you can only useimport
andexport
in a.mjs
file, or in a.js
file if"type": "module"
.Cannot use import statement outside a moduleUnexpected token ‘export’
您只能在ES模块中使用
import
和export
。 具体来说,这意味着您只能在.mjs
文件或.js
文件(如果为"type": "module"
使用import
和export
。Cannot use import statement outside a moduleUnexpected token 'export'
You cannot use
require
in an ES module, you must useimport
.您不能在ES模块中使用
require
,而必须使用import
。You cannot use
require
in an ES module, you must useimport
.ReferenceError: require is not defined
您不能在ES模块中使用
require
,而必须使用import
。ReferenceError: require is not defined
You cannot use
require
to load an ES module.您不能使用
require
加载ES模块。You cannot use
require
to load an ES module.Error [ERR_REQUIRE_ESM]: Must use import to load ES Module
您不能使用
require
加载ES模块。Error [ERR_REQUIRE_ESM]: Must use import to load ES Module
So, what does work?
那么, 什么工作呢?
- An ES module can import exports from a CommonJS module. As far as mixing goes, that’s it. ES模块可以从CommonJS模块导入导出。 就混合而言,就是这样。
For example, if "type": "commonjs"
, then this example works, i.e. node module1.mjs
will run without any errors.
例如,如果"type": "commonjs"
,则此示例有效,即, node module1.mjs
将运行而没有任何错误。
// module1.mjsconsole.log("require module1");import obj from "./module2.js";
console.log(`module2 = ${obj.module2}`);// module2.jsconsole.log("require module2");
exports.module2 = "require module2";
If "type": "module"
, then this example works, i.e. node module1
will run without any errors.
如果"type": "module"
,则此示例有效,即, node module1
将运行而没有任何错误。
// module1.jsconsole.log("require module1");import obj from "./module2.cjs";
console.log(`module2 = ${obj.module2}`);// module2.cjsconsole.log("require module2");
exports.module2 = "require module2";
As a reminder, full code examples for these rules can be found here: https://github.com/arcticmatt/javascript_modules/tree/master/node.
提醒一下,这些规则的完整代码示例可以在以下位置找到: https : //github.com/arcticmatt/javascript_modules/tree/master/node 。
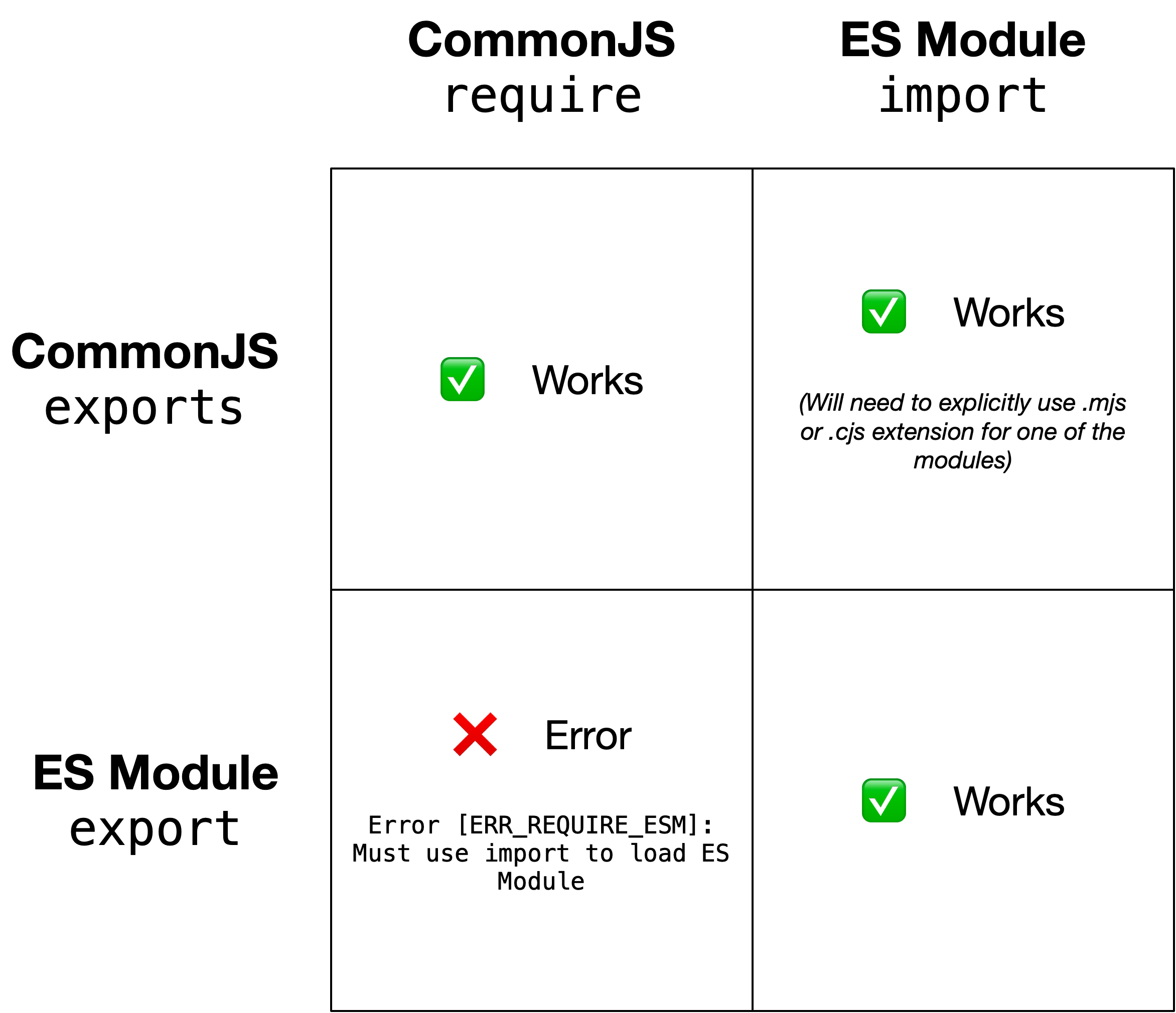
资源资源 (Resources)
翻译自: https://medium.com/@pencilflip/using-es-modules-with-commonjs-modules-in-node-js-1015786dab03
commonjs模块