javascripts手册
This week I’ll cover one way of creating an algorithm to calculate whether two strings are anagrams.
本周,我将介绍一种创建算法以计算两个字符串是否为字谜的方法。
In this algorithm, you are given two strings and are required to find whether or not they are anagrams of each other.
在此算法中,将为您提供两个字符串,并且要求您查找它们是否是彼此的字谜。
An anagram is a word or phrase that is formed by rearranging the letters of another word. An example is “cinema” which can be formed using the letters of “iceman”. Another example is “listen” which can form “silent”. Note that these words have the exact same number of letters as their counterparts. If a word has an extra letter (take “reduce” and “reducer” for instance), then it will not be considered an anagram even though the letters of reducer are capable of forming the word reduce.
字谜是通过重新排列另一个单词的字母而形成的单词或短语。 一个示例是“电影院”,可以使用“ iceman”字母形成。 另一个例子是“听”,它可以形成“沉默”。 请注意,这些单词与对应单词的字母数完全相同。 如果一个单词有一个额外的字母(例如,以“ reduce”和“ reducer”为例),那么即使reducer的字母能够构成reduce单词,也不会将其视为一个字谜。
If you need further clarification, a quick google search will be able to give you all the information you require.
如果您需要进一步的说明,快速的Google搜索将能够为您提供所需的所有信息。
With this algorithm, what we are trying to achieve is this:
使用此算法,我们试图实现的是:
Example 1: Input: “iceman”, “cinema” Output: trueExample 2: Input: “abcd”, “fghi” Output: falseExample 3: Input: “reduce”, “reducer” Output: false
For the sake of simplicity, we will assume that all inputs are strings that possess no uppercase letters. In addition, you are not allowed to use the .sort() method.
为了简单起见,我们将假定所有输入都是不包含大写字母的字符串。 此外,不允许使用.sort()方法。
So, to begin with we have an empty function:
因此,首先我们有一个空函数:
function isAnagram(a, b){}
Before moving forward, I am of the opinion that it is vital to create an action plan detailing what you need to achieve in order to achieve your result.
在继续前进之前,我认为至关重要的是制定一项行动计划,详细说明您需要实现的目标,以实现目标。
With that being said, what we essentially need to do is compare two strings to check if they each have both the same characters as well as the same number of characters WITHOUT using the .sort() method.
话虽如此,我们基本上需要做的是比较两个字符串,以检查它们是否都具有相同的字符以及相同数量的字符,而无需使用.sort()方法。
In order to compare the two strings without using .sort() we need to either re-create the .sort() method or find another way to manipulate the data such that we are able to compare the information from both strings.
为了在不使用.sort()的情况下比较两个字符串,我们需要重新创建.sort()方法,或者找到另一种方式来处理数据,以便我们能够比较两个字符串中的信息。
I have chosen to proceed by manipulating the data without creating my own sorting method.
我选择继续操作数据而不创建自己的排序方法。
At this point, you may be wondering how it is possible to modify a string without using .sort(). I will be doing so by creating two objects with keys being letters and values being the frequency that these letters are found within a string.
此时,您可能想知道如何在不使用.sort()的情况下修改字符串。 我将通过创建两个对象来实现此目的,其中键是字母,值是在字符串中找到这些字母的频率。
For example:
例如:
“apple” = {“a”: 1, “p”: 2”, “l”: 1, “e”: 1}
Going back to the empty function I wrote earlier, we can now move forward with the process of turning our strings into objects.
回到我之前写的空函数,现在我们可以继续将字符串转换为对象的过程。
You could now say that we have reworded this problem into something that makes more sense from a coding perspective. In addition to checking to see if two strings are anagrams, we are also comparing two objects and checking for equivalency.
您现在可以说,我们已将此问题改写为从编码角度来看更有意义的问题。 除了检查两个字符串是否为字谜之外,我们还比较了两个对象并检查其等效性。
Inside that function, we can also potentially increase its speed (provided the small increase in memory is not a concern) by first checking to see if both strings are the same length. If not, return false.
在该函数内部,我们还可以通过首先检查两个字符串的长度是否相等来潜在地提高其速度(前提是不考虑内存的小幅增加)。 如果不是,则返回false。
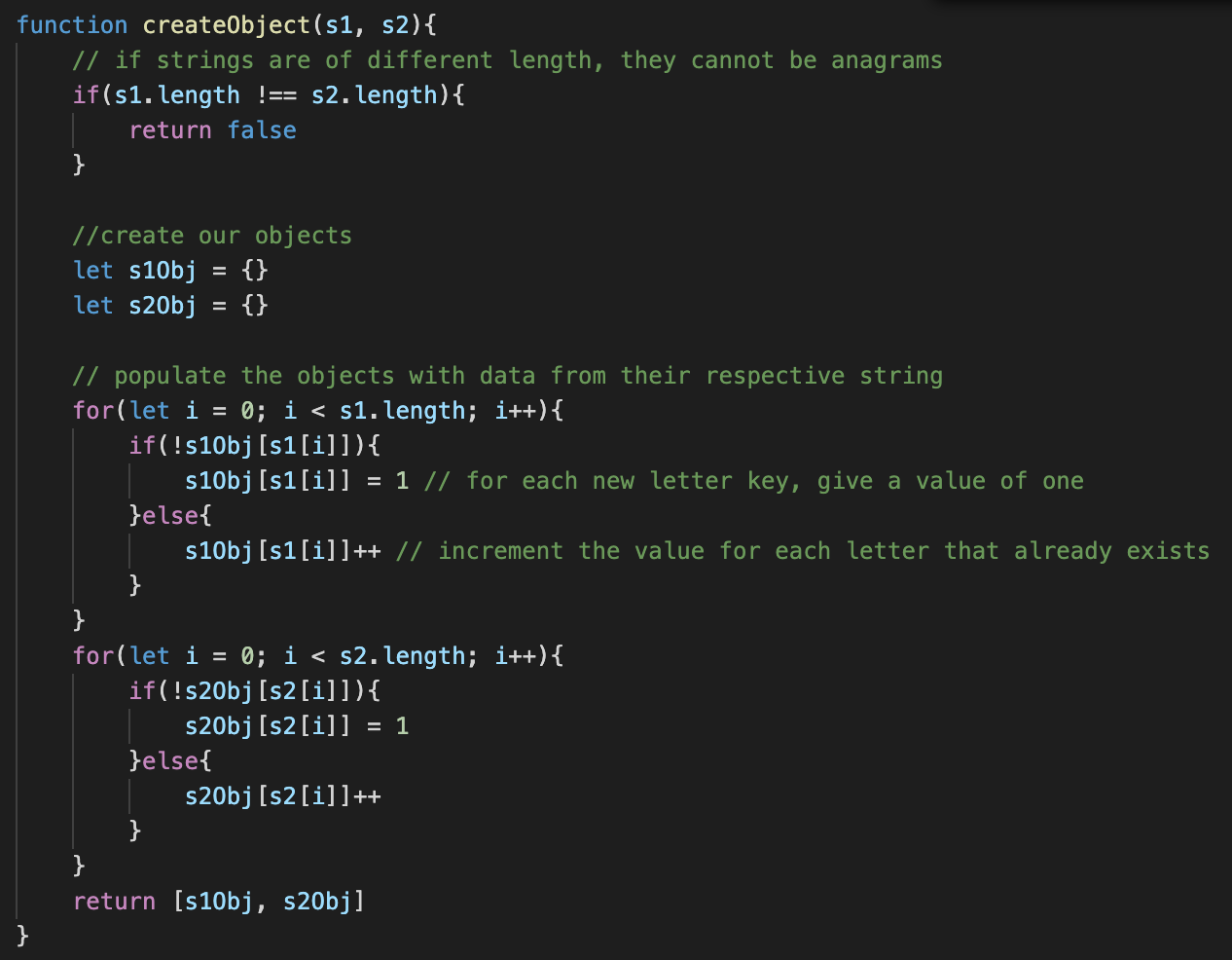
Running this function by itself should grant the following output:
单独运行此功能应授予以下输出:
createObject(“iceman”, “battery”)[ { i: 1, c: 1, e: 1, m: 1, a: 1, n: 1 }, { b: 1, a: 1, t: 2, e: 1, r: 1, y: 1 } ]
Now that we have our objects as well as validation to check if both strings are the same length, we can create a function which compares them and checks for equivalence.
现在我们有了对象和验证来检查两个字符串的长度是否相同,我们可以创建一个函数来比较它们并检查是否相等。
function isAnagram(a, b){}
We should also refactor the code of the first function so that it calls the isAnagram function at the end rather than returning an array of objects.
我们还应该重构第一个函数的代码,以便它在最后调用isAnagram函数,而不是返回对象数组。
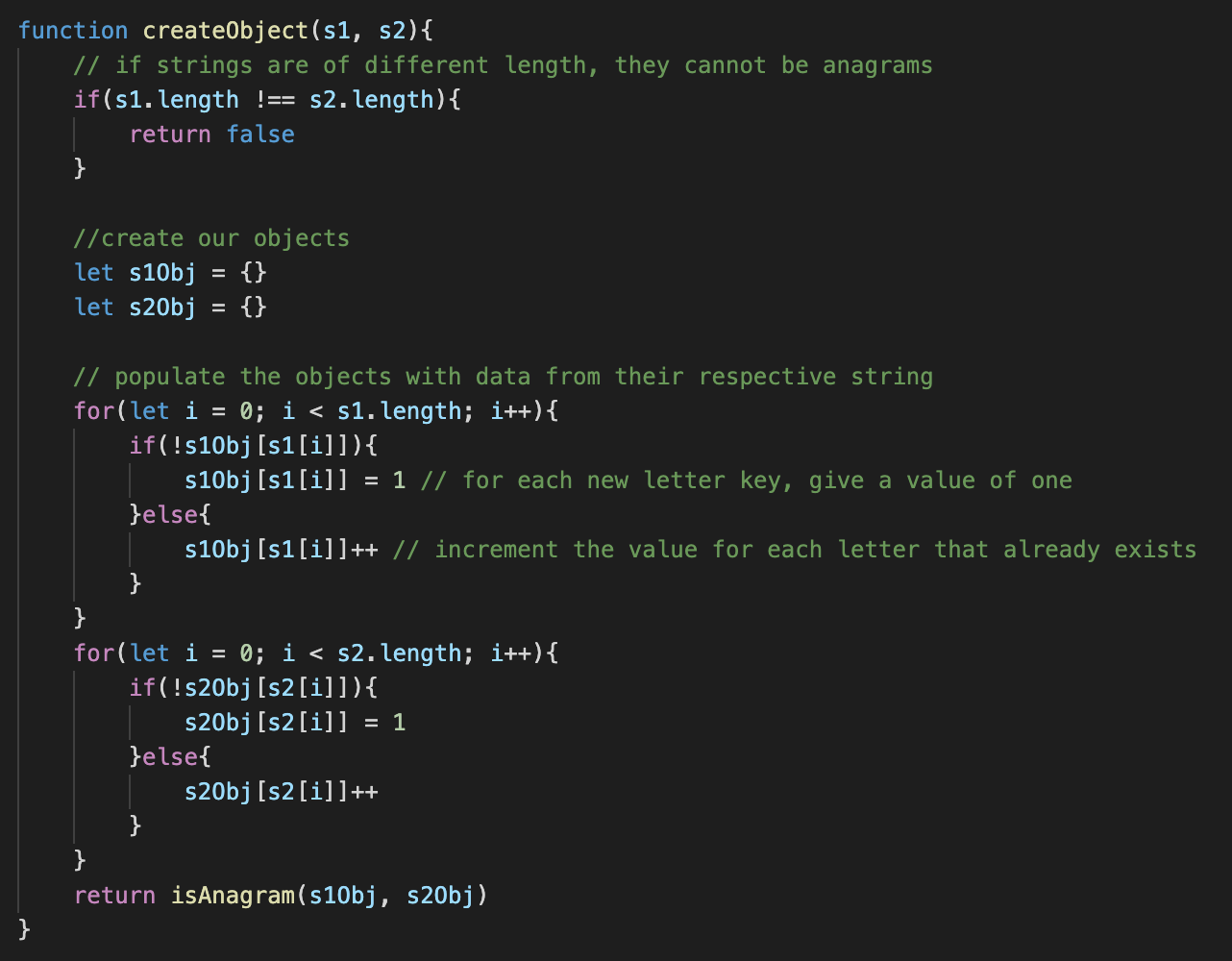
Going back to the isAnagram function, we now need to figure out how to compare both objects. For those of you newer to programming, you might think that all we need to do is:
回到isAnagram函数,我们现在需要弄清楚如何比较两个对象。 对于那些刚接触编程的人,您可能认为我们需要做的是:
return s1Obj === s2Obj // if equal, will return true. Else returns false
return s1Obj === s2Obj //如果相等,将返回true。 否则返回假
Unfortunately, this is not possible as two objects or arrays (even if their contents are the same) will never be equal to one another.
不幸的是,这是不可能的,因为两个对象或数组(即使它们的内容相同)也永远不会彼此相等。
We can now check to see if both objects contain the same characters. There is more than one way to do this. I will merely show you one method of comparing object keys. After that it is only a simple matter of comparing the lengths of both resulting arrays to see if they possess the same letters.
现在我们可以检查两个对象是否包含相同的字符。 有多种方法可以做到这一点。 我将仅向您展示一种比较对象键的方法。 之后,只需比较两个结果数组的长度以查看它们是否具有相同的字母,这很简单。
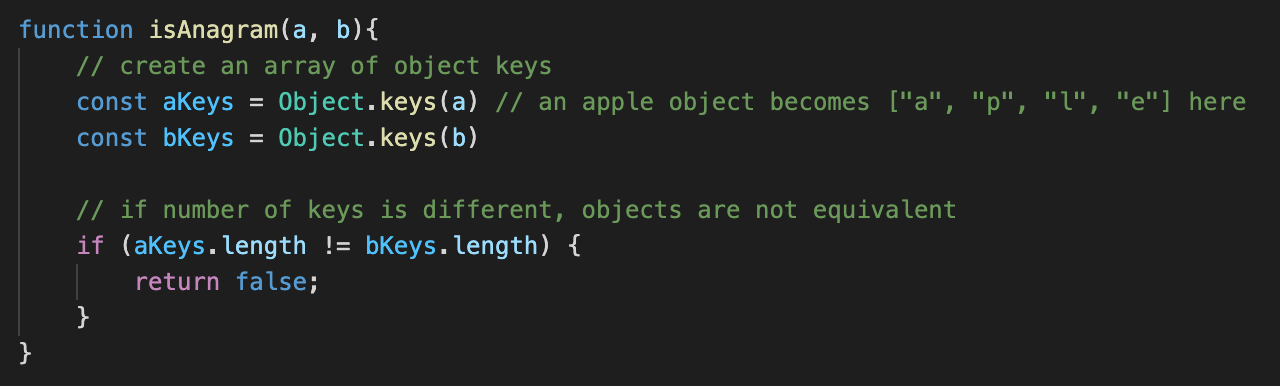
If you have been coding along, you will now be able to check if strings are of equal length and find out if they have the same letters.
如果您一直在编码,则现在可以检查字符串的长度是否相等,并找出它们是否具有相同的字母。
Next we will check if strings of equal lengths possess the same combination of characters. A for loop will be utilized for this.
接下来,我们将检查相等长度的字符串是否具有相同的字符组合。 for循环将用于此目的。
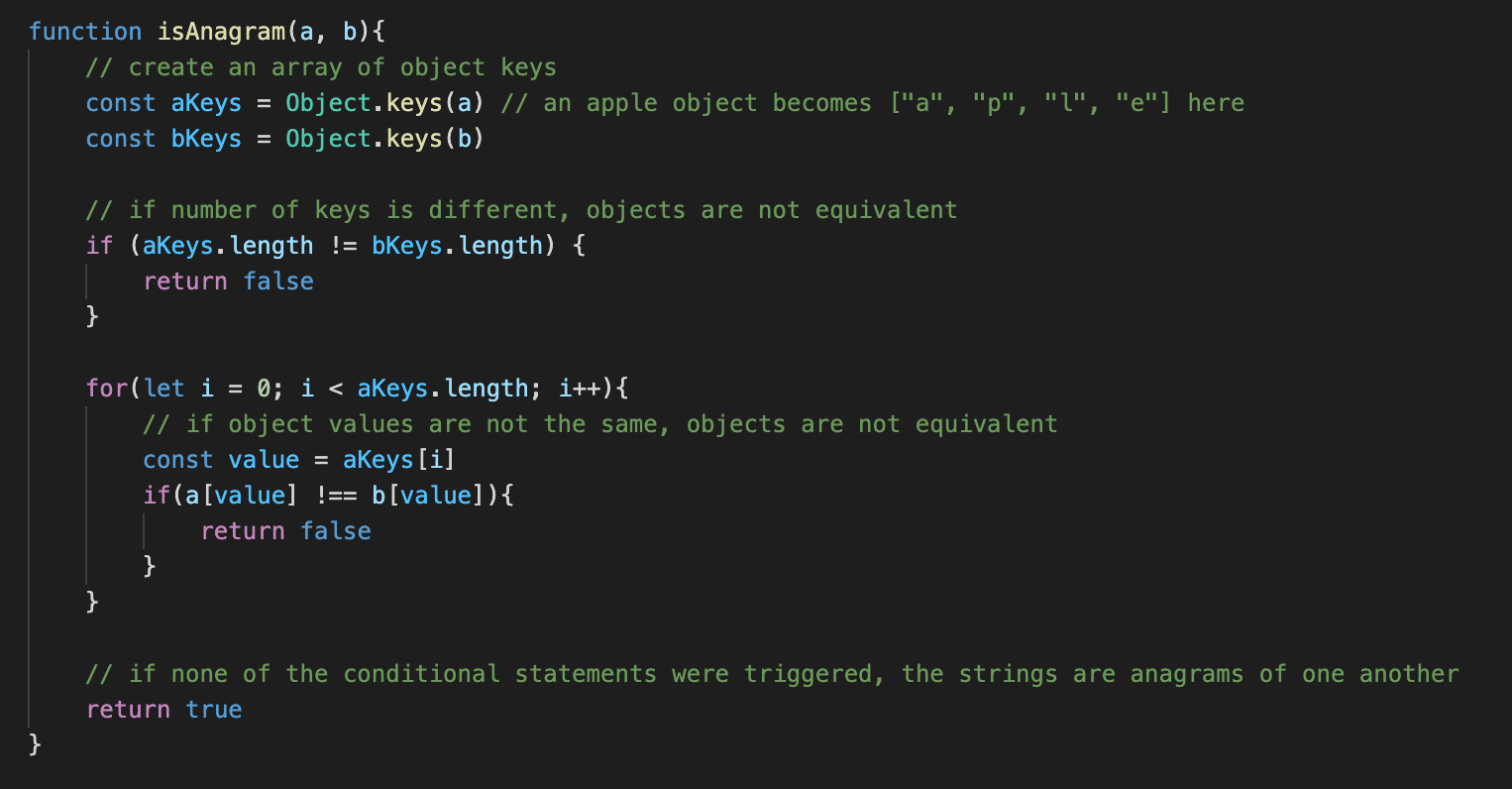
As you can see above, we are able to access object values by entering in their keys. If a certain key-value pair is not equal to its counterpart, then the function returns false. If none of the conditional statements in either functions are triggered, then it is assumed that the strings are anagrams.
如上所示,我们可以通过输入对象的键来访问对象值。 如果某个键值对不等于对应的键值对,则该函数返回false。 如果两个函数中的任何条件语句均未触发,则假定字符串为字谜。
createObject(“apple”, “mapple”) // falsecreateObject(“apple”, “maple”) // falsecreateObject(“apple”, “paple”) // truecreateObject(“apple”, “apple”) // truecreateObject(“iceman”, “cinema”) // truecreateObject(“through”, “driveway”) // falsecreateObject(“misc”, “derc”) // falsecreateObject(“miscellaneous”, “misc”) // falsecreateObject(“thru”, “urth”) // truecreateObject(“reduce”, “reducr”) // false
And there you have it! I just guided you through a way to create an algorithm that checks if two strings are anagrams of one other.
在那里,您拥有了! 我只是引导您完成一种创建算法的方法,该算法可以检查两个字符串是否彼此相同。
Here is the GitHub repository for this algorithm:
这是此算法的GitHub存储库:
javascripts手册