angle2quat
It’s 2020, and Angular is on version 10 with over 1200 contributors and 18,500 commits. I think it’s safe to say that this project has picked up some momentum over the years.
现在是2020年,Angular的版本为10,拥有1200多个贡献者和18,500个提交。 我认为可以肯定地说,这个项目在过去的几年中取得了一些进展。
But when I started using Angular (it was on version 3 when I started), these things didn’t matter to me. Heck, I probably couldn’t have explained what open source software was and definitely couldn’t have explained what Typescript was. To me, Angular was just the popular framework that everybody was using — and that was plenty of a reason to start using it.
但是当我开始使用Angular时(当我开始使用版本3时),这些东西对我来说并不重要。 哎呀,我可能无法解释什么是开源软件,也绝对不能解释什么是Typescript。 对我来说,Angular只是每个人都在使用的流行框架-这是开始使用它的充分理由。
As I learned more about software engineering, I tried out frameworks like React and Vue, and there were even a few months where I truly thought that Angular “wasn’t powerful enough for my needs” because it didn’t have an easy state management system like React (talk about ignorance). But time kept passing by, I kept learning more, and with each successive project that I completed using Angular, it became harder and harder to switch away from the framework I had come to know so well. I had officially become an Angular developer.
随着我对软件工程的更多了解,我尝试了React和Vue之类的框架,甚至有几个月我真的认为Angular“不够强大,无法满足我的需求”,因为它没有简单的状态管理功能像React这样的系统(谈论无知)。 但是随着时间的流逝,我不断学习更多的知识,并且在使用Angular完成的每个后续项目中,越来越难以脱离原来熟悉的框架。 我已经正式成为Angular开发人员。
In the last few years, I’ve built production-ready apps using Angular and have come miles and miles from where I started. That’s probably not saying much considering I started “using” (or more accurately, stumbling around in) Angular at the same time I was learning how to write basic JavaScript.
在过去的几年中,我已经使用Angular构建了可用于生产环境的应用程序 ,并且距我开始的路途遥遥。 考虑到我在学习如何编写基本JavaScript的同时开始“使用”(或更准确地说是在Angular中),这可能并没有说太多。
In this post, I want to share 3 things that I now understand about Angular that I wish I would have much earlier.
在这篇文章中,我想分享我现在希望对Angular有所了解的3件事。
第1课:那叫TypeScript的东西是什么? (Lesson #1: So what’s this thing called TypeScript?)
For a guy who could barely write JavaScript at the time, using TypeScript was more of a burden than anything. Back when I started using Angular, TypeScript was a “necessary evil” that I needed to learn if I wanted to use the Angular framework. Like most beginners, I started off by learning the C language and I had always found the “static typing” concept annoying since it added extra keystrokes to my day. At the time, I thought statically typed languages like C were languages of the past.
对于当时几乎无法编写JavaScript的人来说,使用TypeScript比什么都负担更大。 回到我开始使用Angular时,TypeScript是一个“必不可少的恶魔”,如果我想使用Angular框架,我需要学习它。 像大多数初学者一样,我从学习C语言开始,并且一直觉得“静态键入”概念很烦人,因为它给我的生活增加了额外的击键。 当时,我认为像C这样的静态类型语言是过去的语言。
I thought to myself — Why couldn’t every language be like Javascript without the concept of “types”?
我心想一想-如果没有“类型”的概念,为什么每种语言都不能像Javascript?
To me, Javascript was easier because I didn’t have to keep track of all my variable types. A variable was a variable, and that was that. I now know that this is exactly why someone would avoid writing JavaScript altogether.
对我来说,使用Javascript更容易,因为我不必跟踪所有变量类型。 变量就是变量,仅此而已。 现在我知道,这就是为什么有人会完全避免编写JavaScript。
Typescript is “statically typed” while JavaScript is “dynamically typed”. In other words, with TypeScript, all variable types are known at compile-time as opposed to run-time.
Typescript是“静态类型”,而JavaScript是“动态类型”。 换句话说,使用TypeScript,所有变量类型在编译时(而不是运行时)都是已知的。
Why does this fact excite so many Angular developers? Some common answers to this question might include:
为什么这个事实激发了这么多Angular开发人员? 该问题的一些常见答案可能包括:
- All the features of JavaScript plus the benefit of “types” JavaScript的所有功能以及“类型”的好处
- Enhanced IDE experiences 增强的IDE体验
- Browser Compatibility 浏览器兼容性
But these don’t really excite me. What excites me about TypeScript is twofold — it makes my coding process more efficient, and it makes my thinking more disciplined.
但是这些并没有真正让我兴奋。 TypeScript 让我兴奋的是双重方面的- 它使我的编码过程更高效,并且使我的思维更加规范。
My IDE (Vscode) catches my coding errors as I make them, which in turn forces me to be more disciplined when writing the arguments and return types of my functions. And the cycle repeats.
我的IDE(Vscode)在编写错误时会捕捉到我的编码错误,这又迫使我在编写函数的参数和返回类型时要更加严格。 并且循环重复。
Let’s say you’re writing an Angular component that fetches some data from a backend API (we can use JSONPlaceholder for this example), and the data that you will receive looks something like this:
假设您正在编写一个Angular组件,该组件从后端API中获取一些数据(在此示例中,我们可以使用JSONPlaceholder ),并且您将收到的数据如下所示:
{
"userId": 1,
"id": 1,
"title": "delectus aut autem",
"completed": false
}
So you write your component, carelessly place your HTTP client within the component itself (don’t do this for your projects — put it in a service), and render the return data in the template.
因此,您编写组件时,会不小心将HTTP客户端放到组件本身内(不要对您的项目这样做-将其放在服务中),然后将返回数据呈现在模板中。
/**
* app.component.ts
*/
import { Component, OnInit } from '@angular/core';
import { HttpClient } from '@angular/common/http';
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent implements OnInit {
todo: any;
constructor(private http: HttpClient){}
ngOnInit() {
this.getUser();
}
getUser() {
this.http.get('https://jsonplaceholder.typicode.com/todos/1').subscribe(todo => {
this.todo = todo;
})
}
}
<!-- app.component.html -->
<div *ngIf="todo">
<p>Todo name: {{ todo.title }}</p>
<p>Is todo completed?: {{ todo.completed ? 'YES' : 'NO' }}</p>
</div>
This all works, and it’s not hard to follow because of how simple the project is. But once your APIs get bigger and you’re editing hundreds of different files in the same project, it gets difficult to remember the return types of your API.
所有这些都有效,并且由于项目的简单性,因此不难理解。 但是,一旦您的API变大并且您在同一项目中编辑数百个不同的文件,就很难记住API的返回类型。
At the moment, your Angular project has no idea what the API is going to return and will accept pretty much anything. Let’s make a few improvements to these files and save our future selves a few headaches.
目前,您的Angular项目不知道API将返回什么,并且几乎可以接受任何东西。 让我们对这些文件进行一些改进,以免我们以后为自己烦恼。
/**
* app.component.ts
*/
import { Component, OnInit } from '@angular/core';
import { HttpClient } from '@angular/common/http';
// ADDED THIS
interface Todo {
userId: number;
id: number;
title: string;
completed: boolean;
}
@Component({
selector: 'my-app',
templateUrl: './app.component.html',
styleUrls: [ './app.component.css' ]
})
export class AppComponent implements OnInit {
// ADDED type for variable
todo: Todo;
constructor(private http: HttpClient){}
ngOnInit(): void {
this.getUser();
}
getUser(): void {
// ADDED get<Todo>
this.http.get<Todo>('https://jsonplaceholder.typicode.com/todos/1').subscribe(todo => {
this.todo = todo;
})
}
}
The file doesn’t look all that different, but now, our IDE knows exactly what to expect from our API. This allows for all the following things to happen:
该文件看起来并没有什么不同,但是现在,我们的IDE完全知道从我们的API中可以获得什么。 这允许发生以下所有事情:
- If the backend model changes, all we need to do is update the interface in our Angular app and then our IDE will tell us if this change caused any errors in our code 如果后端模型发生变化,我们需要做的就是更新Angular应用中的界面,然后我们的IDE会告诉我们此变化是否导致代码中的任何错误
When we start typing
this.todo.
, our IDE will suggest the known properties on the object当我们开始输入
this.todo.
,我们的IDE将建议对象的已知属性
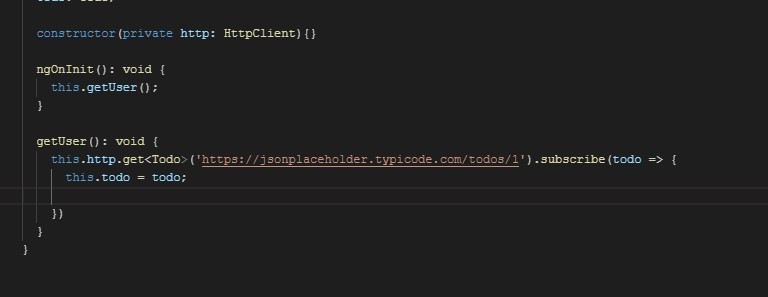
This may not seem like a big deal, but when you’ve got hundreds or even thousands of types defined within a project, having the IntelliSense from your IDE can save you a lot of time.
这似乎没什么大不了,但是当您在项目中定义了数百甚至数千个类型时,从IDE中获取IntelliSense可以节省大量时间。
第2课:Angular有主见,所以不要争论 (Lesson #2: Angular is opinionated, so don’t argue)
Not only was I trying to wrap my head around the usefulness of Typescript when I first started learning Angular, but I was also trying to figure out what a class was, how decorators worked, what dependency injection did, and many more concepts (that’s what happens when your first coding language is JavaScript rather than Java or C++).
刚开始学习Angular时,我不仅试图将Typescript的实用性广为人知,而且还试图弄清一个类是什么,装饰器如何工作,依赖项注入做了什么以及更多概念(这就是当您的第一种编码语言是JavaScript而不是Java或C ++时,就会发生这种情况。
In a sense, I was coding with design patterns and object-oriented-programming before I knew what either of these concepts meant. I later would read Head First Design Patterns and parts of Elements of Reusable Object-Oriented Software to discover that Angular was using several of the design patterns already.
从某种意义上说,在我不了解这两个概念的含义之前,我一直在使用设计模式和面向对象的编程进行编码。 稍后,我将阅读Head First设计模式和可重用的面向对象软件的元素部分,以发现Angular已经在使用几种设计模式。
One of the major benefits you’ll find using an opinionated framework like Angular is that you will be forced to learn and write clean code (although no guarantees — there is definitely a way to write sloppy Angular code).
使用像Angular这样的自以为是的框架会发现的主要好处之一是,您将被迫学习和编写简洁的代码(尽管不能保证-肯定有一种编写草率的Angular代码的方法)。
So here are a few “opinions” that Angular has that I wish I would have taken more seriously from the start:
因此,我希望Angular从一开始就认真对待一些“意见”:
The concept of “feature modules” — I think it took me at least 4 full Angular projects to realize that not all code should be placed in
app.component
. Angular has a robust modules system, and once I started learning about feature modules and how to actually apply SOLID principles in my apps, I finally realized the true power of the Angular framework.“功能模块”的概念 -我认为我至少花了4个完整的Angular项目才意识到,并非所有代码都应放置在
app.component
。 Angular具有强大的模块系统,一旦我开始学习功能模块以及如何在我的应用程序中实际应用SOLID原理,我终于意识到Angular框架的真正威力。Typescript — Kind of beating a dead horse here, but Angular “strongly suggests” that you use Typescript, and I think it’s an opinion worth embracing. And yes, when I say “strongly suggests”, I just mean that using vanilla JavaScript in Angular makes your job much harder and you would not be smart to use it in large capacities.
Typescript —在这里打败一匹老马,但是Angular“强烈建议”您使用Typescript,我认为这是值得接受的一种观点。 是的,当我说“强烈建议”时,我的意思是说在Angular中使用香草JavaScript会使您的工作更加困难,并且大容量使用它并不明智。
The Observer Pattern (aka rxjs) — Angular pairs really well with Observables and functional programming. These concepts take some time to learn, but once you get the hang of it you’ll never turn back. Oh, and learn how to use the async pipe, it will save you a lot of time, clean up your code, and automatically manage your Observables.
观察者模式(aka rxjs ) —与Observables和函数式编程的角度对非常好。 这些概念需要花费一些时间来学习,但是一旦掌握了这些概念,就永远不会回头。 哦,并学习如何使用异步管道 ,它将为您节省大量时间,清理代码并自动管理Observable。
Inputs and Outputs: The Component Pattern — This plays into that “separation of concerns” software principle, and if you take a day to really learn it, your code will be 10x cleaner. Not sure what I’m talking about? Read through this part of the Tour of Heroes tutorial. Using Inputs and Outputs can be confusing at first, but by using them, you can create what we call “dumb components” that can function entirely from the inputs that it receives. This can be really useful if you are creating component libraries of common UI elements such as buttons, modals, etc. It can also be great when using
*ngFor
directive with complex objects as the iterables.输入和输出:组件模式 —这体现了“关注点分离”软件的原理,如果您花一天的时间真正学习它,您的代码将比原来的代码干净10倍。 不知道我在说什么? 通读《英雄之旅》教程的这一部分 。 首先使用输入和输出可能会造成混乱,但是通过使用它们,您可以创建所谓的“哑组件”,这些组件可以完全从接收到的输入中发挥作用。 如果要创建包含按钮,模式等常用UI元素的组件库,这将非常有用。当将
*ngFor
指令与复杂对象作为可迭代对象一起使用时,这也非常*ngFor
。
Angular has many more “opinions”, which for most developers, is a great reason to use Angular over a less opinionated framework like React. If you’re a superstar software engineer that lives and breathes design patterns, Angular’s “opinions” may not be so inviting, and I’m not sure why you’re still reading this post. But for someone like myself who used Angular as a vehicle to learn software engineering, it’s a lifesaver and has pushed me to write cleaner, more modular code.
Angular有更多的“意见”,对于大多数开发人员来说,这是在诸如React之类的观点不强的框架上使用Angular的重要原因。 如果您是一位生活并享受着设计模式的超级软件工程师,那么Angular的“观点”可能不会那么吸引人,我不确定您为什么仍在阅读这篇文章。 但是对于像我这样使用Angular作为学习软件工程工具的人来说,这是一种救命稻草,促使我编写更简洁,更模块化的代码。
第3课:Angular的学习曲线陡峭,但不要让它灰心 (Lesson #3: Angular has a steep learning curve, but don’t let it discourage you)
This point encompasses everything else that I’ve talked about, but I think it’s worth repeating.
这一点涵盖了我已经讨论过的所有其他内容,但我认为值得重复。
For at least the first couple of years I used Angular, it was confusing. Really confusing.
至少在我使用Angular的头几年,它令人困惑。 真令人困惑。
On top of that, I kept hearing people talk about how “front end development is the easy type of development”. Because of this, I felt like I needed to push myself “past the easy stuff” and on to better things.
最重要的是,我不断听到人们谈论“前端开发是简单的开发类型”。 因此,我觉得我需要推动自己“过去简单的事情”,并继续追求更好的事情。
I wish I would have scrapped this mindset much earlier.
我希望我早点放弃这种想法。
In yesterday’s world, front end development involved HTML, CSS, and some clunky JavaScript. In today’s world, front end developers can write robust, Single Page Applications. Long story short? Front end development is much more powerful than it used to be, and it can certainly be a challenge even for a seasoned developer!
在当今世界,前端开发涉及HTML,CSS和一些笨拙JavaScript。 在当今世界,前端开发人员可以编写强大的单页应用程序。 长话短说? 前端开发比以前强大得多,即使对于经验丰富的开发人员而言,这无疑也是一个挑战!
So if you’ve just started using Angular and haven’t quite gotten the hang of it, keep staring at that confusing code in front of you. Eventually, those class decorators, Observables, and directives will make sense. And once they do, you’ll really start to see what Angular has to offer.
因此,如果您刚刚开始使用Angular并没有完全掌握它,请继续盯着面前的那些令人困惑的代码。 最终,这些类装饰器,Observables和指令将变得有意义。 一旦他们这样做了,您将真正开始看到Angular提供的功能。
— — —
— — —
Liked this post? Here are a few more:
喜欢这篇文章吗? 这里还有一些:
Imperative vs. Declarative Programming
翻译自: https://medium.com/swlh/3-things-i-wish-i-knew-when-i-started-using-angular-2-e64652d9a59
angle2quat