I was so inspired by Spike Jonze’s live Documentary “Beastie Boys Story” that I wanted to find a way to feature them in my programming blog. After I spent some time thinking about a topic that might combine the two, I came up with the idea to write an article about OOP (Object Oriented Programming) and the Beastie Boys. In this article, we will look at OOP and using JavaScript, and how OOP relates to real-world examples (mine being the Beastie Boys). We will also build a (very) barebones web app to demonstrate these concepts.
Spike Jonze的现场纪录片《 野兽男孩的故事 》( Beastie Boys Story )给我很大的启发,我想找到一种在我的编程博客中展示它们的方法。 在花了一些时间考虑可能将两者结合在一起的主题之后,我想到了写一篇有关OOP(面向对象编程)和Beastie Boys的文章的想法。 在本文中,我们将研究OOP和使用JavaScript,以及OOP如何与实际示例(例如Beastie Boys)相关联。 我们还将构建一个(非常)准系统的Web应用程序来演示这些概念。
Now, let’s get into it — or, as the Beastie Boys say, “kick it!”
现在,让我们开始讨论吧,或者就像Beastie Boys所说的那样,“踢吧!”
对象和类 (Objects and Classes)
As mentioned above, OOP can be conceived of in the context of real-life examples. In this case, we want to use the Beastie Boys. Essentially, there are many characteristics that could be used to define members of the Beastie Boys; these are things that all members have in common (including their band name, clothing, vocals, and so on).
如上所述,可以在实际示例中构思OOP。 在这种情况下,我们要使用Beastie Boys。 本质上,可以使用许多特征来定义“野兽男孩”的成员。 这些是所有成员的共同点(包括乐队名称,服装,人声等)。
Alongside these features, there are also actions that define them. They all like to party, drink beer, and dance. The process of translating and representing some of these characteristics into the context of OOP is known as abstraction. All of the features above, including the actions, can be defined as their properties:
除了这些功能,还有定义它们的动作。 他们都喜欢参加聚会,喝啤酒和跳舞。 将这些特征中的某些特征转换并表示为OOP上下文的过程称为抽象 。 可以将以上所有功能(包括操作)定义为它们的属性:
Name[realName, emceeName]
Clothing
Vocals
Drink{ "[emceeName] goes glug glug..." }
Now we’ve got four properties (Name, Clothing, Vocals, and Drink). The “Name” property holds another two properties (realName and emceeName). The others could possess singular or multiple data.
现在我们有了四个属性(名称,服装,人声和饮料)。 “名称”属性包含另外两个属性(realName和emceeName)。 其他可能拥有单个或多个数据。
The only actions-based property is Drink
which is a type of property that performs a function. Rather than holding key-pair data like the others, it performs a function.
唯一基于动作的属性是Drink
,它是一种执行功能的属性。 它不像其他密钥对那样保存密钥对数据,而是执行功能。
A property that performs a function is known as a method:
执行功能的属性称为方法:
mca.drink() // "MCA goes glug glug..."
adRock.drink() // "Ad Rock goes glug glug..."
All of the properties belong to a class with the name BeastieBoy
:
所有属性都属于一个名为BeastieBoy
的类 :
class BeastieBoy
Name[realName, emceeName]
Clothing
Vocals
Drink{ "[emceeName] goes glug glug..." }
A class is like a simple template to group our data together. It is used to create objects for the program. When we create objects using our class by providing specific values, this is known as instantiation:
类就像一个简单的模板,将我们的数据分组在一起。 它用于为程序创建对象。 当我们通过提供特定值使用我们的类创建对象时,这称为实例化:
Object: beastieBoyMca
Name[Adam Yauch, MCA]
Clothing: Leather jacket
Vocals: bass
Drink{ "[emceeName] goes glug glug..." }
程序概念 (A Concept for a Program)
Let’s take a further look at OOP by building a small web app with the purpose of showing how each member of the Beastie Boys likes to party. Here are the steps we need to take:
让我们通过构建一个小型Web应用程序来进一步研究OOP,目的是展示Beastie Boys的每个成员都喜欢如何聚会。 这是我们需要采取的步骤:
- Create a form to select each of the members of the Beastie Boys 创建一个表单以选择Beastie Boys的每个成员
- Dynamically change the heading based on the form’s input 根据表单的输入动态更改标题
- Update the text using a button 使用按钮更新文本
Here’s a GIF showing the app in action:
这是显示正在运行的应用程序的GIF:
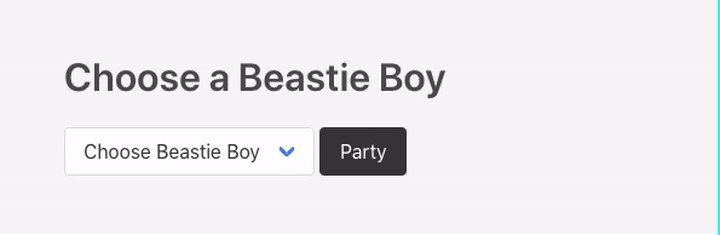
We are going to implement the functionality by using an OOP approach. The complete code example for this is available here.
我们将通过使用OOP方法来实现该功能。 此处提供了完整的代码示例。
创建课程 (Creating the Class)
We’ll use declaration to create a class with the name BeastieBoy
in our JavaScript file:
我们将使用声明在我们JavaScript文件中创建一个名称为BeastieBoy
的类:
class BeastieBoy { } // declare the Beastie Boy Class
class BeastieBoy { } // declare the Beastie Boy Class
We’ll then use a constructor method which will help initialize the objects in the program.
然后,我们将使用构造函数方法来帮助初始化程序中的对象。
class BeastieBoy {
constructor(real, emcee, style, party)
}
We’ll add our properties inside the constructor. The property names should describe their purpose: real
is their real name, emcee
is their emcee name, style
is clothes and party
is a line for how they like to party.
我们将属性添加到构造函数中。 属性名称应描述其用途: real
是其真实名称, emcee
是其主持人名称, style
是衣服, party
是他们喜欢聚会的方式。
We’ll also create a partyTime
method which will be used to replace the HTML of the element with the ID of headline
and the value of the party
property.
我们还将创建一个partyTime
方法,该方法将用于使用headline
ID和party
属性值替换元素HTML。
class BeastieBoy {
constructor(real, emcee, style, party) {
this.name = {
real : real,
emcee : emcee
}
this.emcee = emcee
this.style = style
this.party = party
this.partyTime = function() {
return document.getElementById('headline').innerHTML =
this.name.emcee + " is " + party
}
}
}
实例化对象 (Instantiating the Objects)
Next in our code, we’ll instantiate our objects by assigning values for each of the properties in the constructor.
接下来,在代码中,我们将通过为构造函数中的每个属性分配值来实例化对象。
const mca = new BeastieBoy
(
real = 'Adam Yauch',
emcee = 'MCA',
style = 'Leather Jacket',
party = 'smashing the TV!'
)
const adRock = new BeastieBoy
(
real = 'Adam Horovitz',
emcee = 'Ad Rock',
style = ['baseball cap', 'red t-shirt'],
party = 'starting a pie fight!'
)
const mikeD = new BeastieBoy
(
real = 'Michael Diamond',
emcee = 'Mike D',
style = ['Denim Jacket', 'Fedora', 'VW Chain'],
party = 'spiking the punch!'
)
Now that we’ve initiated our objects, we can access these properties using dot notation syntax:
现在我们已经启动了对象,我们可以使用点符号语法访问这些属性:
mca.name // Object { real: "Adam Yauch", emcee: "MCA" }mca.name.emcee // "Adam Yauch"mikeD.name // Object { real: "Michael Diamond", emcee: "Mike D" }mikeD.name.emcee // "Mike D"adRock.name // Object { real: "Adam Horovitz", emcee: "Ad Rock" }adRock.name.emcee // "Ad Rock"
如何聚会 (How to Party)
Each of the Beastie Boys holds different strings for the party
property because just as much as they party together, they also like to celebrate in their own unique way:
每个“野兽男孩”对于party
财产持有不同的要求,因为就像他们一起聚会一样,他们也喜欢以自己独特的方式庆祝:
Let’s demonstrate this by finishing the rest of the app. In the app’s index HTML file, we’ll create a select element with the ID of beastie-boys
and the name select-beastie-boy
. Within select, we’ll add option elements with values for each of our objects.
让我们通过完成应用程序的其余部分来演示这一点。 在应用程序的索引HTML文件中,我们将创建一个select元素,其ID为beastie-boys
,名称为select-beastie-boy
。 在select中,我们将为每个对象添加带有值的option元素。
We’ll also add a button with an ID of party
and a heading element with an ID of headline
which we’ll use to dynamically change the content of using our script.
我们还将添加一个ID为party
的按钮和一个标题为headline
的标题元素,我们将使用它们来动态更改使用脚本的内容。
<h1 id="headline" class="title"><span style="inline">Choose a Beastie Boy</span></h1>
<div class="select">
<select id="beastie-boys" name="select-beastie-boy">
<option value = "default">Choose Beastie Boy</option>
<option value = "MCA">MCA</option>
<option value = "Mike D">Mike D</option>
<option value = "Ad Rock"> Ad Rock</option>
</select>
</div>
<button id="party" class="button is-dark">Party</button>
Back in our JS, underneath the code for initiating our objects, we’ll add an event listener for changes to the select element, and assign the value to the selected
variable.
回到我们的JS中,在用于初始化对象的代码下,我们将添加一个事件侦听器以对select元素进行更改,然后将值分配给selected
变量。
selectElement.addEventListener('change', (event) => {
selected = event.target.value
})
Finally, we’ll create an “on click” function for the button, and use a switch statement to call our method on the selected object.
最后,我们将为按钮创建一个“单击时”函数,并使用switch语句在所选对象上调用我们的方法。
document.getElementById("party").onclick = function changeContent() {
switch(selected) {
case 'MCA':
mca.partyTime()
break;
case 'Ad Rock':
adRock.partyTime()
break;
case 'Mike D':
mikeD.partyTime()
break;
case 'default':
return document.getElementById('headline').innerHTML =
"Choose a Beastie Boy"
default:
}
}
Here’s an example of the final app in action:
这是运行中的最终应用程序的示例:
结论 (Conclusion)
OOP can be quite hard to initially understand so looking at real-life, entertaining examples can be fun and lend clarity. From our Beastie Boys example, you’ve learned how we can compare objects in real life to those in programming. You’ve also seen how the syntax of OOP using JS can be extended to other projects.
OOP最初可能很难理解,所以看一下现实生活中有趣的示例可能会很有趣,而且很清晰。 从我们的Beastie Boys示例中,您已经了解了如何将现实生活中的对象与编程中的对象进行比较。 您还已经了解了如何将使用JS的OOP语法扩展到其他项目。
Thank you for reading and please let me know in the comments if you enjoyed this article or if you have any suggestions for future posts.
感谢您的阅读,如果您喜欢本文或对以后的帖子有任何建议,请在评论中让我知道。
翻译自: https://codeburst.io/an-introduction-to-object-oriented-programming-in-javascript-b0cb6f65999c