swift mvvm
I’m glad to present in this article well-known for software engineers MVVM design pattern which was invented by Microsoft architects Ken Cooper and Ted Peters specifically to simplify event-driven programming of user interfaces. Let’s move on and consider the class diagram below for more details and further clarification during this example.
我很高兴在本文中介绍著名的软件工程师MVVM设计模式,该模式是由Microsoft架构师Ken Cooper和Ted Peters发明的,目的是简化事件驱动的用户界面编程 。 让我们继续来看下面的类图,以获取更多详细信息,并在此示例中进一步阐明。
Model-View-ViewModel (MVVM) is a structural design pattern that separates objects into three distinct groups:
Model-View-ViewModel(MVVM)是一种结构设计模式,可将对象分为三个不同的组:
• Model holds application data - it’s usually structs or simple classes.
•模型保存应用程序数据-它通常是结构或简单类。
• View/ViewController display visual elements and controls on the screen such as buttons, labels, images, text fields etc.
•View / ViewController在屏幕上显示视觉元素和控件,例如按钮,标签,图像,文本字段等。
• ViewModel responsible for presentation logic, in other words transform model information into values that can be displayed on a view and serves as a bridge between the model and view.
•ViewModel负责 对于表示逻辑,换句话说,将模型信息转换为可以在视图上显示的值,并充当模型和视图之间的桥梁。
For more information you can check out this book.
有关更多信息,您可以查看本书。
Cool, now it makes sense, doesn’t it?😎
酷,现在很有意义,不是吗?😎
Go ahead and create our model:
继续创建我们的模型 :
import Foundation
struct Credentials {
var username: String = ""
var password: String = ""
}
Yeah pretty easy, so let’s create our view. I used storyboard for it but you also can implement it programmatically, it’s up to you.
是的,非常简单,因此让我们创建视图 。 我使用了情节提要,但您也可以以编程方式实现,这取决于您。
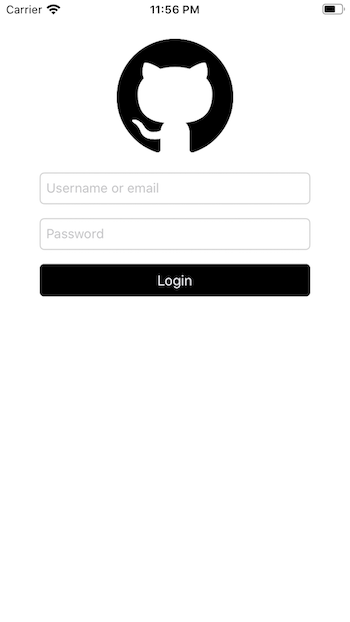
As you can see, in this sample I decided to implement GitHub authorization screen although ne