ios音频硬编码和软编码
Difficulty: Beginner | Easy | Normal | Challenging<br/>
难度:初学者| 容易| 普通 | 具有挑战性的<br/>
This article has been developed using Xcode 11.4.1, and Swift 5.2.2
本文是使用Xcode 11.4.1和Swift 5.2.2开发的。
先决条件: (Prerequisites:)
You will be expected to be aware how to make a Single View Application in Swift.
您应该知道如何在Swift中制作Single View应用程序 。
术语 (Terminology)
Dark mode: A darker color visual palette for users
深色模式:用户的深色视觉调色板
该方法 (The Approach)
Light and dark mode puts your app at the mercy of users. They can easily change the look and feel of the app. So how can you make sure that you App will be prepared for this brave new world? There is just one way of doing this:
亮和暗模式将您的应用置于用户的摆布之下。 他们可以轻松更改应用程序的外观。 那么,如何确保您的App为这个勇敢的新世界做好准备? 只有一种方法可以做到这一点:
- Avoid hard-coded colors 避免硬编码的颜色
So how would we do that? How would we make a `UILabel` change for both dark mode and light mode — so label has a default color for each?
那么我们该怎么做呢? 我们如何在暗模式和亮模式下都更改UILabel,因此标签的每种颜色都有默认颜色?
Well you could choose one of the following colors *semantic colors*
好吧,您可以选择以下颜色之一* 语义颜色 *
- label 标签
- systemGroupedBackground systemGroupedBackground
- systemBlue systemBlue
- systemBackground 系统背景
- secondarySystemBackground secondarySystemBackground
- tertiarySystemBackground 第三系统背景
So we should think about implementing UI picking an appropriate semantic color. UIKit does the work for you, don’t need to know the mode and when changes the work is done for you. Dark Mode is not a simple inversion of light colours, and we are often given versions of color that fall into one of the following iOS color categories:
因此,我们应该考虑实现UI选择合适的语义颜色。 UIKit可以为您完成工作,不需要了解模式以及何时进行更改即可为您完成工作。 深色模式不是简单的浅色反转,我们经常得到的颜色版本属于以下iOS颜色类别之一:
- Primary 主
- Secondary 次要的
- Tertiary 第三
- Quaternary 第四纪
Views and controls are designed to fit together
视图和控件旨在整合在一起
为黑暗模式设计 (Designing for Dark Mode)
- Use UIKit colors and controls 使用UIKit颜色和控件
- Customize colors and images where necessary 必要时自定义颜色和图像
- Decide on the color and appearance of the App 确定应用程序的颜色和外观
Each color can have a value for dark and light mode, the color is set one time only.
每种颜色可以有一个用于暗和亮模式的值,该颜色只能设置一次。
在情节提要中切换到黑暗模式 (Switching to dark mode in the Storyboard)
Still using the Storyboard? We can view a dark or light interface style! The requisite option is highlighted below
还在使用情节提要吗? 我们可以查看深色或浅色界面样式! 必需的选项在下面突出显示

Which means we can switch from a light view:
这意味着我们可以从明亮的视图切换:
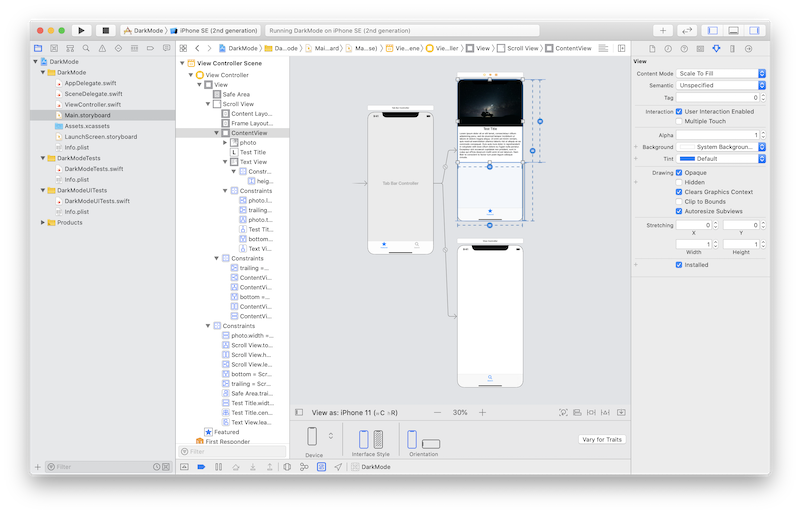
to a dark view
到黑暗的景色
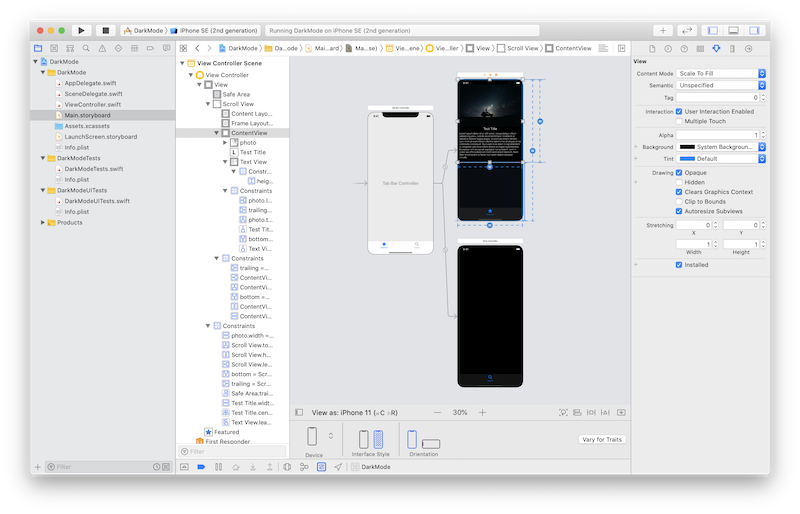
If you look at the demo in the Repo System Background color
has already been used for the background, and Label color
for the label colour — these are semantic colors so automatically has a light and a dark variant!
如果您在回购System Background color
查看演示,则System Background color
已用作背景, Label color
用于标签颜色-这些是语义颜色,因此自动具有浅色和深色变体!
在Xcode中动态切换到暗模式 (Switching to dark mode dynamically in Xcode)
From iOS13 we have had a rather attractive Environment Overrides
button, and can switch between dark and light mode in real time.
从iOS13开始,我们有了一个非常吸引人的“ Environment Overrides
按钮,可以实时在暗和亮模式之间切换。
We must first run our App, and then select the Environment Overrides
button on the bottom toolbar (highlighed with a red rectangle below)
我们必须首先运行我们的应用程序,然后选择底部工具栏上的“ Environment Overrides
按钮(在下面用红色矩形高亮显示)

from there we can select the Environment Overrides
slider (once again highlighted with a red rectangle)
从那里我们可以选择“ Environment Overrides
滑块(再次用红色矩形突出显示)
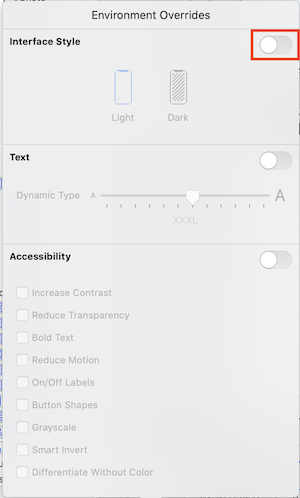
which then allows us to select either dark or light mode
然后,我们可以选择暗或亮模式
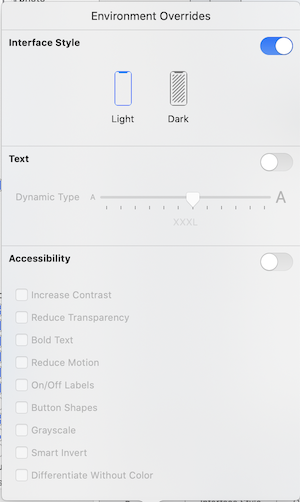
If you look at the demo in the Repo System Background color
has already been used for the background, and Label color
for the label colour — these are semantic colors so automatically has a light and a dark variant!
如果您在回购System Background color
查看演示,则System Background color
已用作背景, Label color
用于标签颜色-这些是语义颜色,因此自动具有浅色和深色变体!
动态影像 (Dynamic images)
I’ve noticed that my image doesn’t really match the light mode. Xcode has me covered here, and can switch the image according to the light or dark mode.
我注意到我的图像与发光模式不完全匹配。 Xcode在这里介绍了我,可以根据亮或暗模式切换图像。
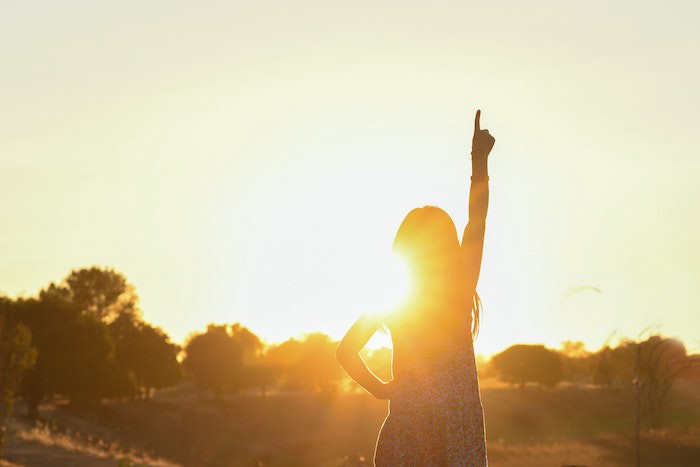
I (luckily perhaps) put my image in the Assets
folder. Now we can select an alternative appearance (highlighted in red)
我(幸运的是)将我的图像放在Assets
文件夹中。 现在我们可以选择其他外观(以红色突出显示)
Here I select Any, Dark
在这里我选择Any, Dark
This is all sorts of awesome!
这真是太棒了!
If you want to this in code, you can!
如果您想在代码中做到这一点,可以!
let image = UIImage(named: "photo")let asset = image?.imageAssetlet resolvedImage = asset?.image(with: traitCollection)
制作自己的动态色彩 (Make your own dynamic colors)
In Xcode you can add your own color set in the asset catalog. The colors can also be written in code!
在Xcode中,您可以在资产目录中添加自己的颜色集。 颜色也可以用代码编写!
let myColor = UIColor { (traitCollection: UITraitCollection) -> UIColor in
if traitCollection.userInterfaceStyle == .dark {
return .black
} else {
return .white
}
}
which can then be accessed through code
然后可以通过代码访问
print (myColor.cgColor.components)
which will be then drawn again when the user switches between light and dark mode.
当用户在明暗模式之间切换时,将再次绘制该图。
however cgColor
can’t be dynamic. We will need to get a trait collection from a view — and we can ask the color to resolve itself using a trait collection.
但是cgColor
不能是动态的。 我们将需要从视图中获取特征集-并且我们可以要求颜色使用特征集来解决自身问题。
There are few options for this, so let us explore them all!
很少有选择,所以让我们一起探索吧!
1. resolve the color
1.解决颜色
// 1let traitCollection = view.traitCollectionlet resolvedColor = myColor.resolvedColor(with: traitCollection
2. performAsCurrent
2. performAsCurrent
// 2traitCollection.performAsCurrent { // set border color, or similar Core Graphics color}
3. set the current UITraitCollection
3.设置当前的UITraitCollection
// 3UITraitCollection.current = traitCollection// set border color, or similar Core Graphics color
The first one is tricky for many colors, however. Setting UITraitCollection
only affects the current thread. For the third option you probably want to change the UITraitCollection
back again once you have finished, so should save this as a temporary property.
但是,第一个对于许多颜色来说都是棘手的。 设置UITraitCollection
仅影响当前线程。 对于第三个选项,您可能想要在UITraitCollection
后再次将UITraitCollection
改回,因此应将其保存为临时属性。
When we override traitCollectionDidChange
we might have the following function, and we only want to resolve dymanic colours when the appearance has changed. There is no problem, we can do this using traitCollection.hasDifferentColorAppearance
, which is called when the trait collection changes.
当我们覆盖traitCollectionDidChange
我们可能具有以下功能,并且我们只想在外观更改时解决动态色。 没问题,我们可以使用traitCollection.hasDifferentColorAppearance
进行此操作,当trait集合更改时会调用它。
override func traitCollectionDidChange(_ previousTraitCollection: UITraitCollection?) {
super.traitCollectionDidChange(previousTraitCollection)
if traitCollection.hasDifferentColorAppearance(comparedTo: previousTraitCollection) {
// reset dynamic colors
}
}
Did you kno* you can enable debug logging with the launch argument -UITraitCollectionChangeLoggingEnabled YES
which can be set through Edit Scheme…>Arguments
which you can then press the addition button (highlighted in red):
您是否知道 *可以使用启动参数-UITraitCollectionChangeLoggingEnabled YES
启用调试日志记录,可以通过Edit Scheme…>Arguments
进行设置,然后可以按添加按钮(红色突出显示):
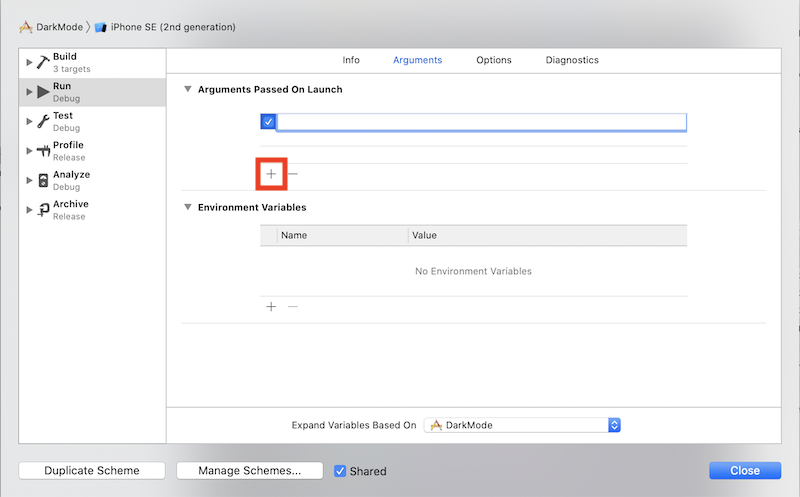
and then enter the argument, which then will log changes to the console as you would expect.
然后输入参数,然后将更改记录到控制台中,如您所愿。
Traits are updated before layout occurs — meaning that the right colors are used at any time.
在进行布局之前会更新特性,这意味着可以随时使用正确的颜色。
使您的整个应用处于亮或暗模式 (Make your whole App light or dark mode)
You can set, in the info.plist
, UIUserInterfaceStyle
to either light or dark.
您可以在info.plist
UIUserInterfaceStyle
设置为亮或暗。
Done!
做完了!
结论 (Conclusion)
Dark Mode has been around for a while, and users expect this. Therefore you should be implementing this in your own applications to give uses that choice and flexibility.
黑暗模式已经存在了一段时间,用户对此表示期望。 因此,您应该在自己的应用程序中实现此目的,以便使用该选择和灵活性。
Happy users mean good reviews, and ultimately this means more users.
满意的用户意味着好评,最终意味着更多的用户。
This has to be good for everyone!
这必须对所有人都有好处!
If you’ve any questions, comments or suggestions please hit me up on Twitter
如果您有任何疑问,意见或建议,请在Twitter上打我
翻译自: https://medium.com/@stevenpcurtis.sc/stop-hard-coding-colors-in-ios-apps-18f96f3b30e0
ios音频硬编码和软编码