I found data structure and algorithms are daunting and it is like something I can never be reached to as a self-taught. However, from some point I wanted to become a better programmer who can code with efficiency and with ability of considering memory spaces and time length of code execution. I am sharing what I learnt as I am learning this topic from a view of a self-taught individual.
我发现数据结构和算法令人生畏,这就像我自学成才一样。 但是,从某个角度来看,我想成为一个更好的程序员,可以高效地进行编码,并能够考虑内存空间和代码执行的时间长度。 我从一个自学成才的人的角度分享我在学习这个主题时所学到的知识。
什么是数组? (What is an Array?)
Array is like a list of data. It has its own address for each space.
数组就像数据列表。 每个空间都有自己的地址。
Example above is an array called arr that contains 5 integers from 1 to 5, each data space has own address which begins from 0, as computers count from 0. We need address to go somewhere, likewise computer needs address to go to check if the data is in the box. So to get 3, for example, it can be expressed as arr[2]. This example above can be expressed in C as following:
上面的示例是一个名为arr的数组,其中包含5个从1到5的整数,每个数据空间都有自己的地址,该地址从0开始,因为计算机从0开始计数。我们需要将地址放到某个地方,同样,计算机也需要地址去检查是否数据在框中。 因此,例如,要获得3 ,可以将其表示为arr [2] 。 上面的示例可以用C表示如下:
int arr[5] = {1, 2, 3, 4, 5}
int arr [5] = {1,2,3,4,5}
This means I am creating an array length of 5 and its name is arr, and it will contain integer numbers by declaring int before its name.
这意味着我要创建一个长度为5的数组,其名称为arr ,并且通过在其名称之前声明int来包含整数。
更改数组中的数据 (Changing datas in array)
Let’s change data in arr[3] where 4 lives in to 13.
让我们将arr [3]中的数据更改为4到13。
arr[3] = 13;
arr [3] = 13;
Now, our array arr look like as following:
现在,我们的数组arr如下所示:
{1, 2, 3, 13, 5}
{1,2,3,13,5}
记忆 (Memory)
To understand how data structures work behind the scene, we need to have some knowledge of memory, which is basically storage for computer.
要了解数据结构如何在后台工作,我们需要具有一些内存知识,而内存基本上是计算机的存储内容。
There are two mechanisms to store data on your computer. One is memory that handles temporal data that is called RAM, another one is storage that handles permanent data. So if your computer is switched off while you were working on something that you did not save, the progress will be all gone.
有两种机制可以在计算机上存储数据。 一种是处理临时数据的内存 ,称为RAM ,另一种是处理永久数据的存储 。 因此,如果您在处理未保存的内容时关闭了计算机,则进度将全部消失。
RAM和存储的区别 (Difference of RAM and Storage)
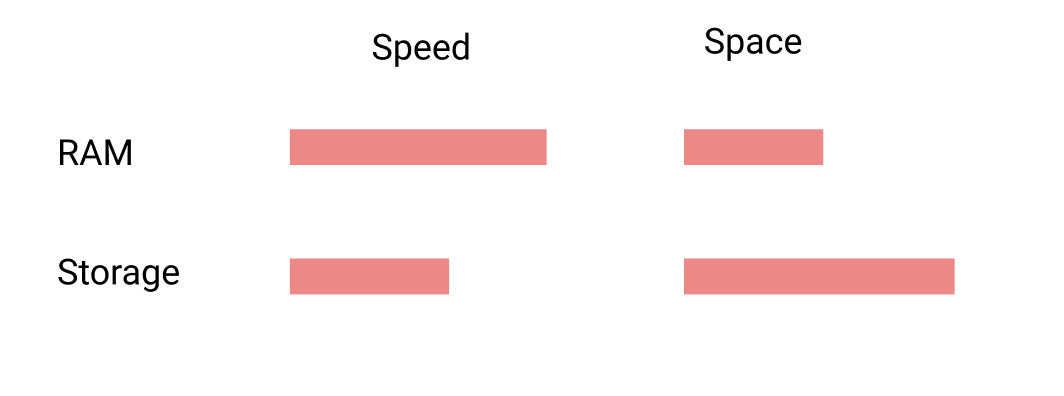
As you can see from above graph, RAM(memory) is much faster to get and read data but its space is a lot smaller. Storage, on the other hand, has much more spaces but it is slower to get data from.
从上图可以看出,RAM(内存)获取和读取数据的速度要快得多,但其空间要小得多。 另一方面,存储具有更多的空间,但获取数据的速度较慢。
深入了解阵列和内存 (Array and Memory in-depth)
Memory is like a box of spaces in our computer, each box size is a byte.
内存就像我们计算机中的一堆空格,每个箱子的大小都是一个 字节 。
Also, it has got arbitrary numbers of address and store datas in binary numbers that is kind of “language a computer understands”
此外,它具有任意数量的地址,并且以二进制数存储数据,这是“计算机可以理解的语言”
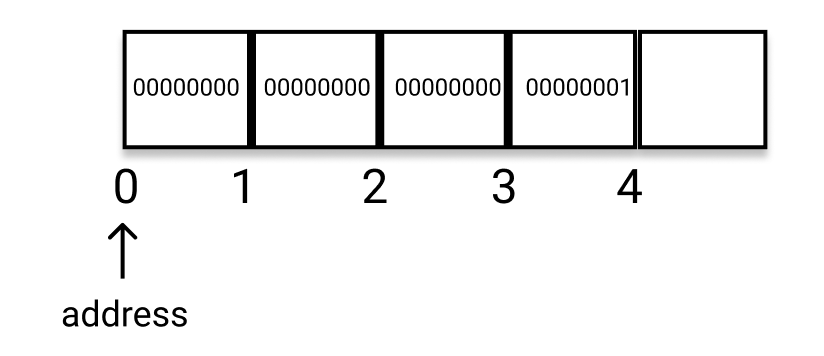
字节和位 (Byte and Bit)
Let’s say you are going to store integer number to variable a, you can store other types of datas like decimals, strings, for the sake of simplicity, we are going to only store integer for this time. Computers can only read 1 and 0, these are called binary numbers and that is how datas looks like under the hood. integer numbers are 32 bits of 1 and 0 and can be expressed like so.
假设您将整数存储到变量a,可以存储其他类型的数据,例如小数,字符串,为简单起见,我们这次仅存储整数。 计算机只能读取1和0 ,它们被称为二进制数 ,这就是数据在幕后的样子。 整数是32位的1和0,可以这样表示。
1 = 00000000000000000000000000000001 ( 0 * 31, 1*1)
1 = 00000000000000000000000000000001(0 * 31,1 * 1)
1 bit = 1 or 0
1位= 1或0
8 bits = a byte
8位=一个字节
Therefore, integer takes up 4 bytes of memory (32 bits).
因此,整数占用4个字节的内存(32位)。
使用数组的缺点 (Disadvantage of using array)
Array is a sequence of data as I mentioned earlier, so this means, datas in an array should be stored contiguously. Let’s store our array, arr, we created earlier. int arr[5] = {1, 2, 3, 4, 5}
正如我前面提到的, 数组是数据序列,因此这意味着数组中的数据应连续存储。 让我们存储我们之前创建的数组arr 。 int arr [5] = {1,2,3,4,5}
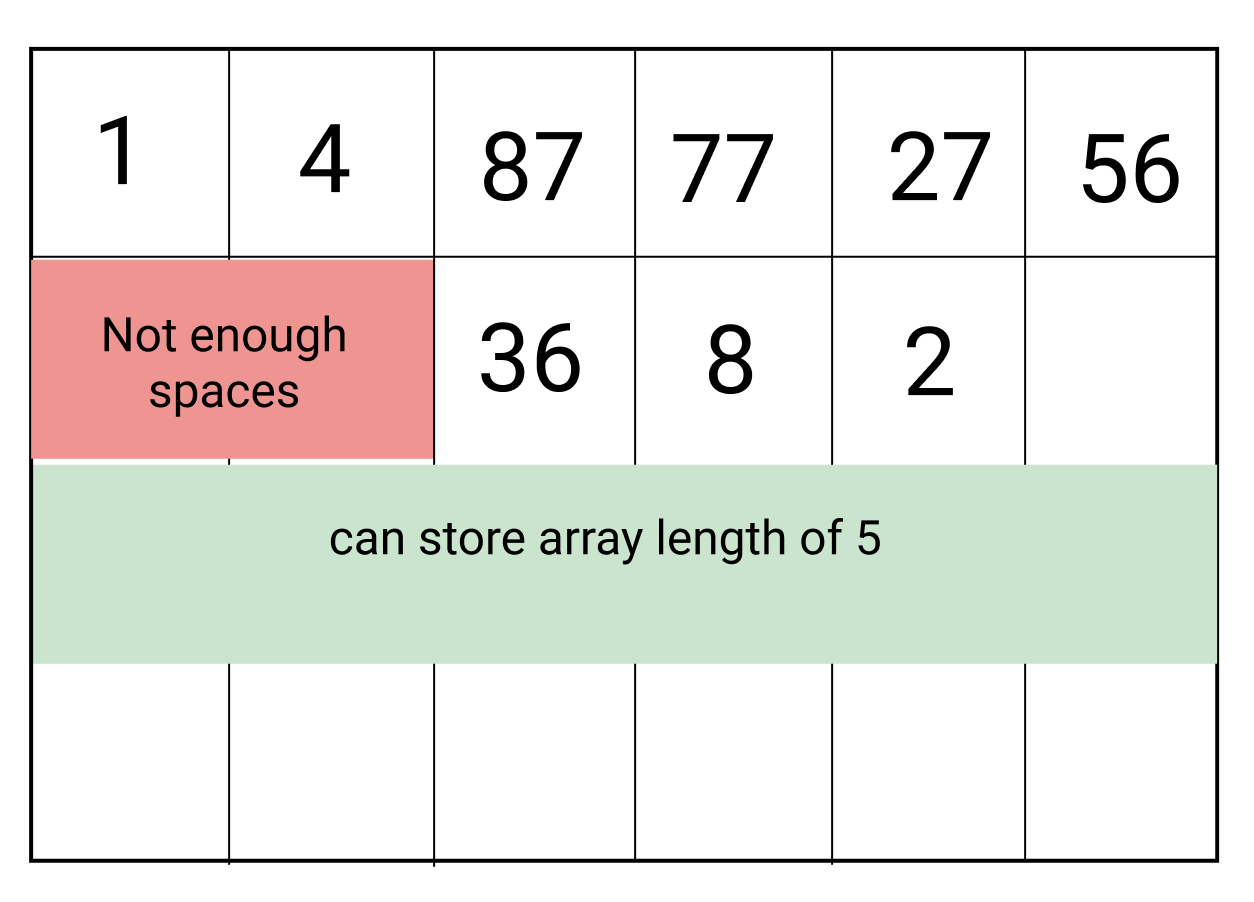
There are few disadvantages of array as far as I know.
据我所知,数组的缺点很少。
Firstly, as you can see, we can not store our array in red spaces because it is small, but we can store it on green spaces. This means that array could be inefficiency in managing memory.
首先,如您所见,由于数组很小,因此无法将其存储在红色空间中,但可以将其存储在绿色空间中。 这意味着,阵列可以在 管理存储器是低效率 。
Secondly, when you add new data to an array, array would not be able to create or extend new space for the new data, as the length is fixed already, so new length of array will be newly created, which has to take more spaces.
其次,当您向数组中添加新数据时,由于长度已固定,因此数组将无法为新数据创建或扩展新空间,因此将重新创建数组的新长度,该数组必须占用更多空间 。
So that is where Linked List comes into a play. I will talk about Linked list on next post.
这就是链接列表的作用所在。 我将在下一篇文章中讨论链接列表。
翻译自: https://medium.com/swlh/learning-data-structure-01-array-6bc37fc37c54