面向对象编程语言和面向过程
Most software engineers are taught the principles of object-oriented programming early on in their education. It is a way of thinking about abstract problems in a tactile way.
大多数软件工程师在学习初期就被教导了面向对象编程的原理。 这是一种以触觉方式思考抽象问题的方法。
Many languages exist — Java, C++, and even Python — that provide first-class constructs for object-oriented programming. They streamline the process of writing software “by the book”.
存在许多语言(Java,C ++甚至Python),它们为面向对象的编程提供了一流的构造。 他们简化了“按书”编写软件的过程。
However, developers occasionally find themselves in a position where they must use a language that does not provide such amenities. Perhaps they are writing embedded software for a platform for which only a C compiler exists, or they are creating a video game using a scripting language like GML. What then?
但是,开发人员有时会发现自己处在必须使用不提供此类便利性的语言的位置。 也许他们正在为仅C编译器存在的平台编写嵌入式软件,或者正在使用GML之类的脚本语言来创建视频游戏。 然后怎样呢?
The goal of this article is to convince software engineers that object-oriented programming is a mindset and approach — rather than just a language feature — that can be adopted regardless of the constraints of the development environment at hand.
本文的目的是使软件工程师相信,面向对象的编程是一种思维方式和方法,而不仅仅是一种语言功能,可以在不考虑开发环境约束的情况下采用。
类图 (A Class Diagram)
In order to achieve its goal, this article is going to give an example of object-oriented programming in a language that has no first-class constructs for such an approach. The following UML class diagram visually shows the “classes” that will be implemented and their relationships to one another.
为了实现其目标,本文将提供一种使用这种方法的一流结构的语言进行面向对象编程的示例。 下面的UML 类图直观地显示了将要实现的“类”以及它们之间的关系。
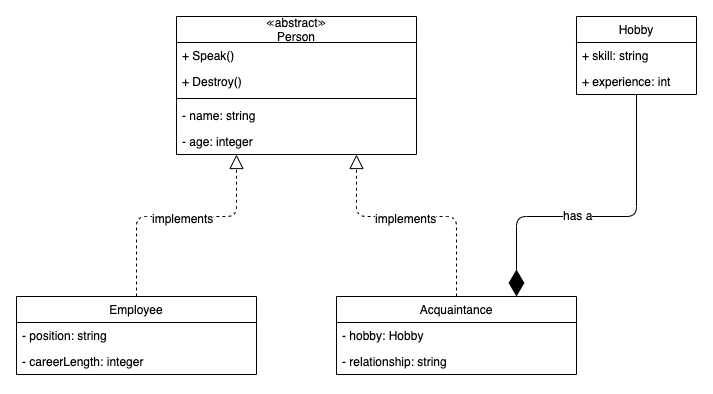
使它发生 (Making it Happen)
Based on the above diagram, consider the following example of a very basic (and nearly useless) program written in C — a language with no first-class constructs for object-oriented programming. Try to get a general feel for the semantics — or what the “intention” of the code is — even if the syntax is unfamiliar.
根据上图,考虑以下用C语言编写的非常基本(几乎无用)程序的示例,C是一种没有用于面向对象编程的一流构造的语言。 即使语法不熟悉,也应尝试大致了解语义或代码的“意图”。
The example above shows many concepts of object oriented programming.
上面的示例显示了许多面向对象编程的概念。
A Person
abstract class is defined, and two other concrete classes – Employee
and Acquaintance
– inherit from it and implement its members in different ways. Said members are truly a part of each class' structure and are thus bound to object instances of each – a demonstration of encapsulation.
定义了Person
抽象类 ,另外两个具体类 – Employee
和Acquaintance
–从该类继承并以不同方式实现其成员。 所说的成员确实是每个类结构的一部分,因此绑定到每个类的对象实例– 封装的演示。
Further, Acquaintance
has another concrete class – Hobby
– as a member, which is a demonstration of composition (or, arguably aggregation – some people do not have any hobbies).
此外, Acquaintance
还有一个具体的班级- Hobby
-作为成员,这是构图的演示(或者可以说是聚合 -有些人没有任何爱好)。
Down in the main()
program body, polymorphism is shown. Because functions are bound to each class via a virtual method table, they can be called on an object instance with only a simple reference to the base class – Person
– regardless of whether the referenced instance is an Employee
or an Acquaintance
. When a method in the virtual method table is called, the implementation associated with the underlying object instance's concrete class is executed.
在main()
程序主体中,显示了多态性 。 因为函数是通过虚拟方法表绑定到每个类的,所以可以在对象实例上调用它们,而只需简单地引用基类Person
,无论引用的实例是Employee
还是Acquaintance
。 调用虚拟方法表中的方法时,将执行与基础对象实例的具体类关联的实现。
可能的改进 (Possible Improvements)
With a basic mental image of how object-oriented programming is possible even when first-class support for it is not available, one can begin to ponder how to implement various “advanced” mechanisms.
有了即使没有一流的支持也无法实现面向对象编程的基本思想,人们就可以开始思考如何实现各种“高级”机制。
Need to support calling a super’s version of a method? Enhance the virtual method table implementation to support implementation chains.
是否需要支持调用方法的超级版本? 增强虚拟方法表实现以支持实现链。
Looking for a way to perform a “type of” operation on a base reference, or support for a this
or self
reference to help make open recursion feel more natural? Add some decorators to the base structure and initialize them appropriately in the children.
寻找一种在基本参考上执行“类型”操作的方法,还是对this
或self
参考的支持以使开放递归更加自然? 在基础结构中添加一些装饰器,并在子代中适当地初始化它们。
Many languages — such as C — even support some concept of static
functions and variables, allowing for class variables and methods to be realized as opposed to just instance variables and methods.
许多语言(例如C)甚至支持static
函数和变量的某些概念,从而允许实现类变量和方法,而不仅仅是实例变量和方法。
And finally, many languages provide mechanisms for data hiding. In fact, C allows for header files — which simply declare struct and method signatures — and separate implementation files — where said structs and methods are actually implemented. Leveraging such a feature can allow for the concept of public
and private
members to be realized.
最后,许多语言提供了数据隐藏机制。 实际上,C允许头文件(仅声明结构和方法签名)以及单独的实现文件(在其中实际实现所述结构和方法)。 利用这种功能可以实现public
和private
成员的概念。
The sky really is the limit. Arguably, there are more design options with languages that do not enforce an object-oriented approach. But, like anything, the freedom comes with responsibility.
天空真的是极限。 可以说,使用不强制执行面向对象方法的语言的设计选项更多。 但是,就像任何事物一样,自由伴随着责任。
警示语 (Word of Caution)
Like most formal sources, this article champions object-oriented programming. However, it is necessary to put an asterisk next to everything written thus-far.
像大多数正式资源一样,本文拥护面向对象编程。 但是,有必要在迄今为止编写的所有内容旁边加上星号。
There are cases where object-oriented programming makes things much more complicated than necessary. It lends itself to a development culture heavily focused on design patterns and principles. While extremely valuable in their own right, too much of anything can cause more harm than good.
在某些情况下,面向对象的编程会使事情变得比必要的复杂得多。 它使自己非常注重设计模式和原理的开发文化。 尽管它们本身具有极其宝贵的价值,但任何东西太多都可能造成弊大于利。
Be careful to not over-engineer nor under-engineer a solution. Solve each problem elegantly, and then move onto the next one. Often, an object-oriented approach leads to an elegant and maintainable solution. Sometimes, however, it is a sledgehammer when a screwdriver is really what is needed.
注意不要过度解决方案或过度解决方案。 优雅地解决每个问题,然后继续进行下一个。 通常,面向对象的方法会导致一种优雅且可维护的解决方案。 但是,有时候确实需要螺丝刀时,这是一把大锤。
翻译自: https://medium.com/swlh/object-oriented-programming-in-any-language-cb65788cc0bc
面向对象编程语言和面向过程