react扩展程序
重点 (Top highlight)
When starting a new React project, it’s always a good idea to put together some guidelines that you and your team will follow to make the code scalable.
在开始一个新的React项目时,最好将您和您的团队遵循的一些准则放在一起,以使代码具有可伸缩性。
In this post, I will share with you a handful of insights from my years of using React that will help you to determine your own project guideline.
在这篇文章中,我将与您分享我使用React多年的一些见解,这些见解将帮助您确定自己的项目准则。
1。 了解如何在本地和全局状态之间组织状态 (1. Learn how to organize state between local and global state)
React is a library that manages the UI based on its current state. As a developer, it’s your job to organize where to keep the state that makes up your application. Some developers prefer to keep every single piece of data inside the redux store to keep track of all available states.
React是一个基于UI的当前状态来管理UI的库。 作为开发人员,组织组成应用程序状态的位置是您的工作。 一些开发人员更喜欢将每个数据保留在redux存储中,以跟踪所有可用状态。
But do you really need to dispatch an action to your state manager just to open or close a simple dropdown menu? And do other parts of your application need to know about the value of that contact form? Form values tend to be short-lived and only used by the component that renders the form.
但是,您是否真的需要仅向打开或关闭简单的下拉菜单的状态管理者发送操作? 您的应用程序的其他部分是否需要了解该联系表的价值? 表单值往往是短暂的,并且仅由呈现表单的组件使用。
Rather than using Redux to keep track of every single state inside your application, it’s better to keep some state local to avoid over-engineering your application.
与其使用Redux来跟踪应用程序中的每个状态,不如将其保留在本地,以避免对应用程序进行过度设计,这更好。
As a rule of thumb, you can ask these questions:
根据经验,您可以提出以下问题 :
- Do other parts of the application care about this data? 应用程序的其他部分是否关心此数据?
- Do you need to be able to create further derived data based on this original data? 您是否需要能够基于此原始数据创建其他派生数据?
- Is the same data being used to drive multiple components? 是否使用相同的数据来驱动多个组件?
- Is there value to you in being able to restore this state to a given point in time (ie, time travel debugging)? 能够将状态恢复到给定的时间点(例如,时间旅行调试)对您来说是否有价值?
- Do you want to cache the data (ie, use what’s in state if it’s already there instead of re-requesting it)? 您是否要缓存数据(即,使用已经存在的状态而不是重新请求)?
- Do you want to keep this data consistent while hot-reloading UI components (which may lose their internal state when swapped)? 您是否想在热重载UI组件时保持这些数据的一致性(交换时可能会丢失其内部状态)?
Components using local states are more independent and predictable.
使用局部状态的组件更加独立和可预测。
学习测试的好处,并从一开始就做 (Learn the benefits of testing and do it from the start)
The thing about writing automation tests is that at a certain point, it’s impossible to test your React project manually without spending a major amount of time and resources.
关于编写自动化测试的事情是,在某个时刻,如果不花费大量时间和资源,就无法手动测试您的React项目。
When starting out a project, it’s very easy to justify skipping writing test code because your codebase is relatively small. If you only have five to ten components in your React application, writing automation does feel like a chore with no clear benefit. But when you have more than fifty components and you have multiple higher-order components, testing your project manually might take a whole day, and even then there could be bugs creeping in without anyone noticing.
开始一个项目时,很容易证明跳过编写测试代码是合理的,因为您的代码库相对较小。 如果您的React应用程序中只有5到10个组件,那么编写自动化的感觉确实很琐碎,没有明显的好处。 但是,当您有五十多个组件并且有多个高阶组件时,手动测试项目可能需要一整天的时间,即使如此,也可能会出现漏洞,而没有任何人注意到。
Yes, writing test code will help you make your code more modular. Yes, it will help you find errors faster and safeguard against crashing in production. But automation testing is ultimately about helping you grow your project when manual testing can no longer verify the code is working as expected.
是的,编写测试代码将帮助您使代码更具模块化。 是的,它将帮助您更快地发现错误并防止生产崩溃。 但是,自动化测试最终是要在手动测试无法再验证代码是否按预期工作时帮助您扩展项目。
But you can’t suddenly write test code when you’re not used to it. That’s why you have to start at the beginning. If you don’t know where to start, then start from integration test because the most important part about testing is that you verify that your components work together properly.
但是,如果您不习惯测试代码,就不能突然编写它。 这就是为什么您必须从头开始。 如果您不知道从哪里开始,那么请从集成测试开始,因为有关测试的最重要部分是验证组件是否可以正常工作。
采用工具来帮助您扩展 (Adopt tools to help you scale)
Normally, you don’t need to add many tools to your React project at the beginning of your application. But since we’re talking about scaling React application to a large codebase, I’d say you need to adopt all the good tools to help you out.
通常,您不需要在应用程序开始时向您的React项目添加许多工具。 但是由于我们正在谈论将React应用程序扩展到大型代码库,所以我想说您需要采用所有好的工具来帮助您。
Prettier and ESLint will be needed to give a consistent code pattern between team members and reduce syntax errors. Powerful utility libraries like React Router, date-fns, and react-hook-form are always good to include.
将需要更漂亮和ESLint,以在团队成员之间提供一致的代码模式并减少语法错误。 强大的实用程序库(如React Router , date-fns和react-hook-form)总是很好地包含在内。
- Adding TypeScript and Redux may be delayed until your app is prone to typing errors and parts of your application require the same state over and over again that you need to make it globally available. TypeScript和Redux的添加可能会延迟,直到您的应用程序容易出现键入错误,并且您的应用程序的某些部分需要一遍又一遍地需要相同的状态才能使它全局可用。
- Implementing state management from the start is not needed because React itself already thinks of the best way to manage state without making you crazy. 不需要从一开始就执行状态管理,因为React本身已经在考虑使状态疯狂的最佳方法。
Bit (Github) to manage and share your components as independent building blocks. That means you test and render each component in isolation. That will guarantee an easier time maintaining and reusing it, later on.
位 ( Github )作为独立的构建块来管理和共享您的组件。 这意味着您将单独测试和渲染每个组件。 这样可以保证以后维护和重用它的时间更加轻松。
Moreover, when using Bit in your project, Bit source-controls each component independently (alongside your project’s SCM), which means a component (shared to
此外,在项目中使用Bit时,Bit源代码独立控制每个组件(与项目的SCM一起),这意味着一个组件(共享给
Bit.dev) can be maintained completely independent of its project (i.e by “importing” a component from Bit.dev, changing it and “pushing” it back to its shared collection)
Bit.dev )可以完全独立于其项目进行维护(例如,通过从Bit.dev中 “导入”组件,进行更改并将其“推回”其共享集合)。
Oh, and you can also use Next.js instead of Create React App to start your project.
哦,您还可以使用Next.js代替Create React App来启动您的项目。
These tools will help you maintain a large React codebase, but be aware that each tool you add will increase the complexity level of your project. Please do your research before deciding to adopt the tool into your stack.
这些工具将帮助您维护大型的React代码库,但是请注意,添加的每个工具都会增加项目的复杂度。 在决定采用此工具之前,请先进行研究。
很好地组织项目文件 (Organize your project files well)
One of the best tips that I learned on scaling React application is that organizing your project files and naming them well can speed up your progress. Some developers tend to write index.js
as the main file in a component directory, like this:
我在扩展React应用程序方面学到的最好的技巧之一是组织项目文件并对其进行良好命名可以加快进度。 一些开发人员倾向于将index.js
编写为组件目录中的主文件,如下所示:

That seems reasonable because when you import the components into other files, the statement becomes simply this:
这似乎是合理的,因为当您将组件导入到其他文件中时,该语句将变成以下形式:
import Button from '../components/Button';
But consider when you open them side by side on the code editor:
但是请考虑在代码编辑器中并排打开它们时:

Honestly, all those index.js
will make anyone confused. But if you rename those index.js files into the component name, your import statement will look a bit ugly:
老实说,所有这些index.js
都会使任何人感到困惑。 但是,如果将这些index.js文件重命名为组件名称,则import语句将看起来有些难看:
import Button from '../components/Button/Button';
My team finally settled on having both the file named after the component and an index.js
file that exports the component:
我的团队最终决定同时拥有以该组件命名的文件和一个导出该组件的index.js
文件:

We also put the CSS and unit test file inside the component directory. This way, each component directory can be a self-contained component.
我们还将CSS和单元测试文件放在component目录中。 这样,每个组件目录可以是一个独立的组件。

建立您的UI /逻辑组件库 (Build your UI/logic component library)
You should not wait to build a component library only when your project has reached its large proportions. You can continuously share components as-you-go. Whenever a new component is built, use Bit to track it and share it to your team’s component collection on Bit.dev, or on your own server.
您不应该仅在项目达到很大比例时才等待构建组件库。 您可以随时随地共享组件。 每当构建新组件时,请使用Bit对其进行跟踪,并将其共享给您在Bit.dev或您自己的服务器上的团队的组件集合。
As mentioned earlier, (truly) independent components are much easier to maintain and when shared and documented, are much easier to reuse.
如前所述,(真正的)独立组件易于维护,并且在共享和记录文档时,也易于重用。
A component library is not only for UI components. Logic should also be included — in the case of React, as custom hooks (by and large).
组件库不仅适用于UI组件。 逻辑也应包括在内-在React的情况下,作为自定义钩子(大体上)。
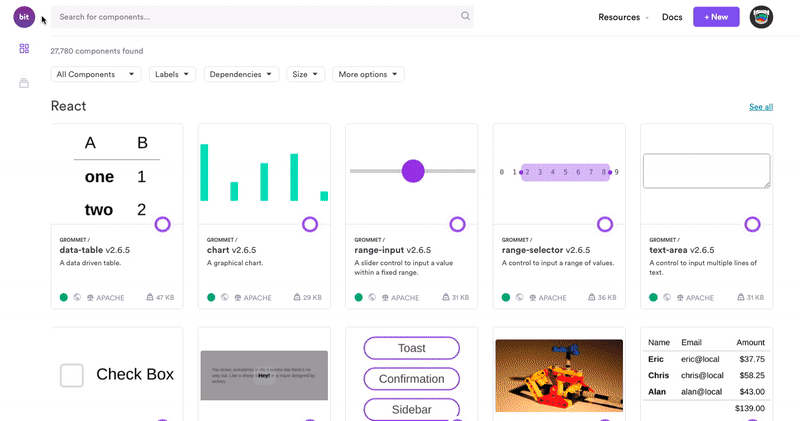
Read more about it here:
在这里阅读更多关于它的信息:
使用钩子将逻辑与组件分离 (Decouple your logic from your component with hooks)
As your project grows, you might notice that some of your component’s logic seems to get used over and over again. To share your component’s logic, you need to write a custom hook.
随着项目的发展,您可能会注意到,组件的某些逻辑似乎一遍又一遍地被使用。 要共享组件的逻辑,您需要编写一个自定义钩子。
For example, let’s say you have an application that counts the score of a Basketball match:
例如,假设您有一个用于计算篮球比赛得分的应用程序:
As you can see in the example, HomeTeam.js
and AwayTeam.js
used the same logic for incrementing the counter. When you have the same logic responsible for driving UI changes, you can decouple the logic from your component and put it in a separate file.
在示例中可以看到, HomeTeam.js
和AwayTeam.js
使用相同的逻辑来增加计数器。 当您具有负责驱动UI更改的相同逻辑时,可以将逻辑与组件分离,并将其放在单独的文件中。
In the example below, I removed the counter state and increment logic into a separate util.js
file and import it into the components:
在下面的示例中,我将计数器状态和增量逻辑删除到一个单独的util.js
文件中,并将其导入到组件中:
Hooks are simply functions that return certain values back into its caller, that’s why you can implement the same pattern to reuse logic between your components.
挂钩只是将某些值返回给其调用者的函数,这就是为什么您可以实现相同的模式以在组件之间重用逻辑的原因。
结论 (Conclusion)
Always remember that building React application at scale is a complicated task that requires you to consider the best decision for both the consumers and developers. In the end, the best practice is the one that works for your users and your team.
永远记住,大规模构建React应用程序是一项复杂的任务,需要您为消费者和开发人员考虑最佳决策。 最后,最佳实践是对您的用户和团队有效的实践。
Although you will always have to experiment with tools and methods for scaling your React project, I hope the tips I’ve shared above will be useful for you.
尽管您始终必须尝试使用工具和方法来扩展React项目,但我希望以上分享的技巧对您有用。
学到更多 (Learn More)
翻译自: https://blog.bitsrc.io/best-practices-and-tips-for-a-scalable-react-application-db708ae49227
react扩展程序