字节
日常逛 xhs 看到一篇吐槽贴,表示被公司恶心到了:
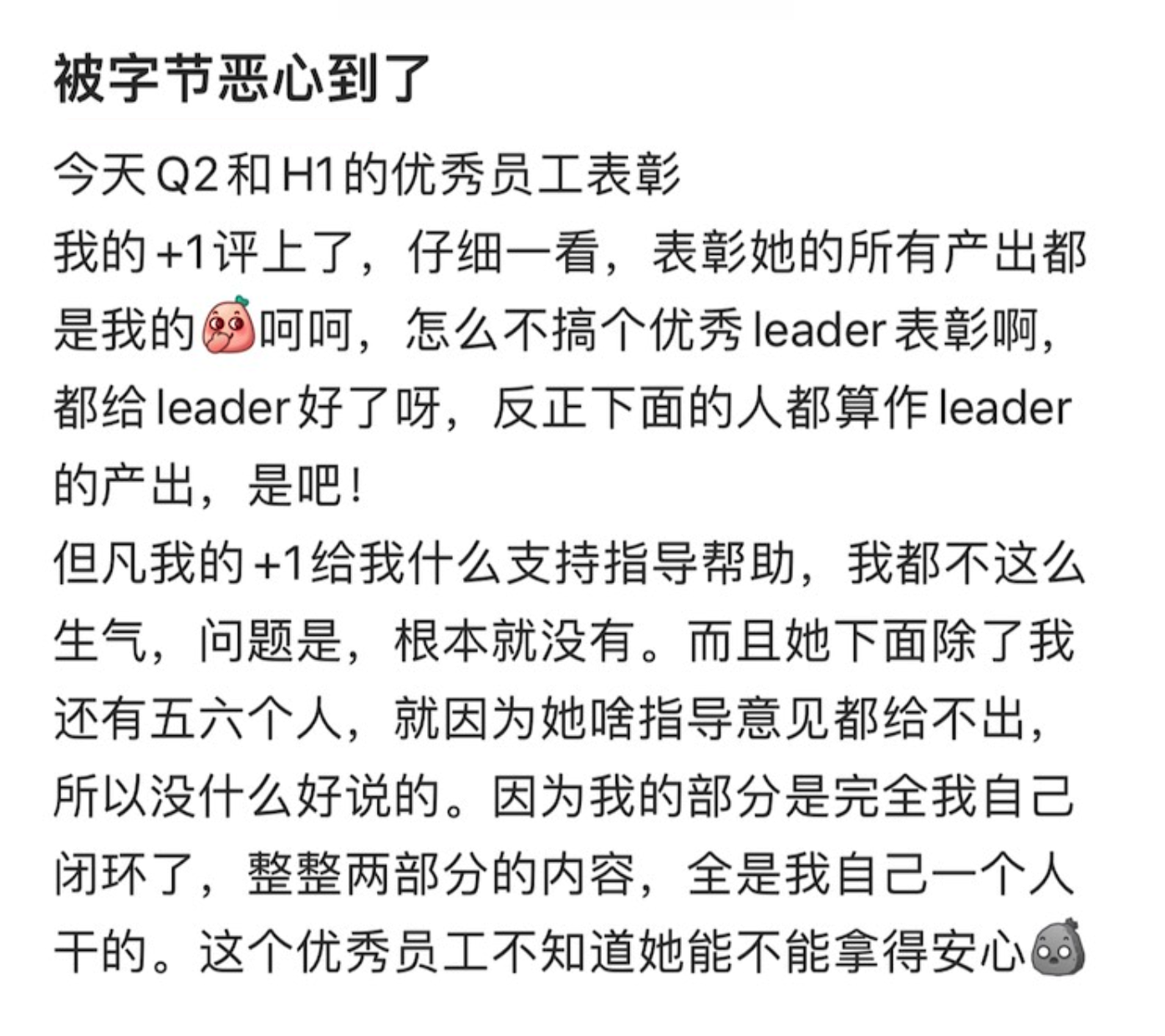
这位网友表示,最近是公司举办了 Q2 和 H1 的优秀员工表彰,自己的 +1(直属领导)评上了,但仔细一看,+1 获奖的所有产出都是自己的,对此表示十分生气。
重点在于,该网友认为自己的 +1 没有提供过指导帮助(对其他下属也是),自己的产出完全是靠自己闭环,直言不知道这个优秀员工 +1 能不能拿到安心。
这事儿怎么说呢,其实在职场十分常见,也不好评这是不是对的。
有句话不知道大家听过没有:完成本职工作其实就是完成领导的 KPI。
至于"+1 没有提供指导帮助",这个属实不应该,那不是纯纯成了邀功了吗。
对此你怎么看?你有过类似经历吗?你在公司算是"嫡系"吗?欢迎评论区交流。
...
回归主题。
来一道和「唠嗑」高度贴合的算法题。
题目描述
平台:LeetCode
题号:690
给定一个保存员工信息的数据结构,它包含了员工唯一的 id ,重要度和直系下属的 id 。
比如,员工 1 是员工 2 的领导,员工 2 是员工 3 的领导。他们相应的重要度为 15 , 10 , 5 。
那么员工 1 的数据结构是 [1, 15, [2]]
,员工 2的 数据结构是 [2, 10, [3]]
,员工 3 的数据结构是 [3, 5, []]
。
注意虽然员工 3 也是员工 1 的一个下属,但是由于并不是直系下属,因此没有体现在员工 1 的数据结构中。
现在输入一个公司的所有员工信息,以及单个员工 id,返回这个员工和他所有下属的重要度之和。
示例:
输入:[[1, 5, [2, 3]], [2, 3, []], [3, 3, []]], 1
输出:11
解释:
员工 1 自身的重要度是 5 ,他有两个直系下属 2 和 3 ,而且 2 和 3 的重要度均为 3 。因此员工 1 的总重要度是 5 + 3 + 3 = 11 。
提示:
-
一个员工最多有一个直系领导,但是可以有多个直系下属 -
员工数量不超过 2000
递归 / DFS
一个直观的做法是,写一个递归函数来统计某个员工的总和。
统计自身的 importance
值和直系下属的 importance
值。同时如果某个下属还有下属的话,则递归这个过程。
Java 代码:
class Solution {
Map<Integer, Employee> map = new HashMap<>();
public int getImportance(List<Employee> employees, int id) {
int n = employees.size();
for (int i = 0; i < n; i++) map.put(employees.get(i).id, employees.get(i));
return getVal(id);
}
int getVal(int id) {
Employee master = map.get(id);
int ans = master.importance;
for (int oid : master.subordinates) {
Employee other = map.get(oid);
ans += other.importance;
for (int sub : other.subordinates) ans += getVal(sub);
}
return ans;
}
}
C++ 代码:
class Solution {
public:
unordered_map<int, Employee*> map;
int getImportance(vector<Employee*>& employees, int id) {
for (auto employee : employees) map[employee->id] = employee;
return getVal(id);
}
int getVal(int id) {
Employee* master = map[id];
int ans = master->importance;
for (int oid : master->subordinates) {
Employee* other = map[oid];
ans += other->importance;
for (int sub : other->subordinates) ans += getVal(sub);
}
return ans;
}
};
Python 代码:
class Solution:
def __init__(self):
self.mapping = {}
def getImportance(self, employees, id):
for emp in employees:
self.mapping[emp.id] = emp
return self.getVal(id)
def getVal(self, id):
master = self.mapping[id]
ans = master.importance
for oid in master.subordinates:
other = self.mapping[oid]
ans += other.importance
for sub in other.subordinates:
ans += self.getVal(sub)
return ans
TypeScript 代码:
map: Map<number, Employee>;
function getImportance(employees: Employee[], id: number): number {
this.map = new Map();
employees.forEach(emp => this.map.set(emp.id, emp));
return getVal(id);
};
function getVal(id: number): number {
const master = this.map.get(id);
let ans = master.importance;
for (const oid of master.subordinates) {
const other = this.map.get(oid);
ans += other.importance;
for (const sub of other.subordinates) ans += getVal(sub);
}
return ans;
}
-
时间复杂度: -
空间复杂度:
迭代 / BFS
另外一个做法是使用「队列」来存储所有将要计算的 Employee
对象,每次弹出时进行统计,并将其「下属」添加到队列尾部。
class Solution {
public int getImportance(List<Employee> employees, int id) {
int n = employees.size(), ans = 0;
Map<Integer, Employee> map = new HashMap<>();
for (int i = 0; i < n; i++) map.put(employees.get(i).id, employees.get(i));
Deque<Employee> d = new ArrayDeque<>();
d.addLast(map.get(id));
while (!d.isEmpty()) {
Employee poll = d.pollFirst();
ans += poll.importance;
for (int oid : poll.subordinates) d.addLast(map.get(oid));
}
return ans;
}
}
C++ 代码:
class Solution {
public:
int getImportance(vector<Employee*> employees, int id) {
int n = employees.size(), ans = 0;
unordered_map<int, Employee*> map;
for (int i = 0; i < n; i++) map[employees[i]->id] = employees[i];
deque<Employee*> d;
d.push_back(map[id]);
while (!d.empty()) {
Employee* poll = d.front();
d.pop_front();
ans += poll->importance;
for (int oid : poll->subordinates) d.push_back(map[oid]);
}
return ans;
}
};
Python 代码:
class Solution:
def getImportance(self, employees: List['Employee'], id: int) -> int:
n, ans = len(employees), 0
mapping = {emp.id: emp for emp in employees}
d = []
d.append(mapping[id])
while d:
poll = d.pop(0)
ans += poll.importance
for oid in poll.subordinates:
d.append(mapping[oid])
return ans
TypeScript 代码:
function getImportance(employees: Employee[], id: number): number {
const map = new Map(employees.map(emp => [emp.id, emp]));
let ans = 0;
const d: Employee[] = [];
d.push(map.get(id));
while (d.length) {
const poll = d.shift();
ans += poll.importance;
for (const oid of poll.subordinates) d.push(map.get(oid));
}
return ans;
};
-
时间复杂度: -
空间复杂度:
最后
巨划算的 LeetCode 会员优惠通道目前仍可用 ~
使用福利优惠通道 leetcode.cn/premium/?promoChannel=acoier,年度会员 有效期额外增加两个月,季度会员 有效期额外增加两周,更有超大额专属 🧧 和实物 🎁 福利每月发放。
我是宫水三叶,每天都会分享算法知识,并和大家聊聊近期的所见所闻。
欢迎关注,明天见。
更多更全更热门的「笔试/面试」相关资料可访问排版精美的 合集新基地 🎉🎉