使用DeepZoom打造变形金刚SHOW
DeepZoom技术是Silverlight 2中加入的全新特性,你可以把DeepZoom简单的理解为一项基于位图的局部加载和缩放的技术,
http://memorabilia.hardrock.com/就是这项技术的典型应用,如图所示。
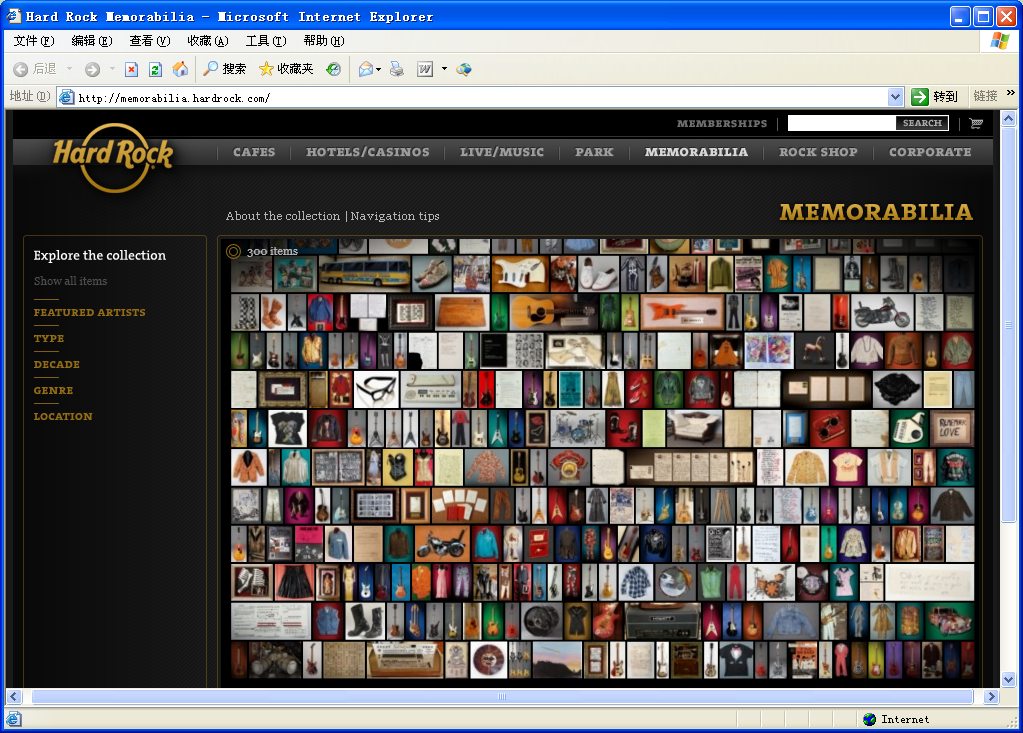
这个页面中包括300幅高分辩率的位图,可想而知,如果将这些图片按顺序全部都下载到客户端浏览器将需要耗费大量的时间和带宽,并且用户关心的内容也不能够在第一时间查看,但是DeepZoom技术可以把一幅或一组图片分割成多个部分,根据用户浏览图像的位置来进行选择性的加载,当用户查看一幅清晰度很高、容量较大的图片时,DeepZoom仅仅加载用户需要浏览位置的内容,并且用户可以轻松的使用鼠标键来拖动和缩放图片,这样极大的减少了用户等待的时间,为用户带来一种很酷的交互方式和浏览体验,放大图片如图所示。
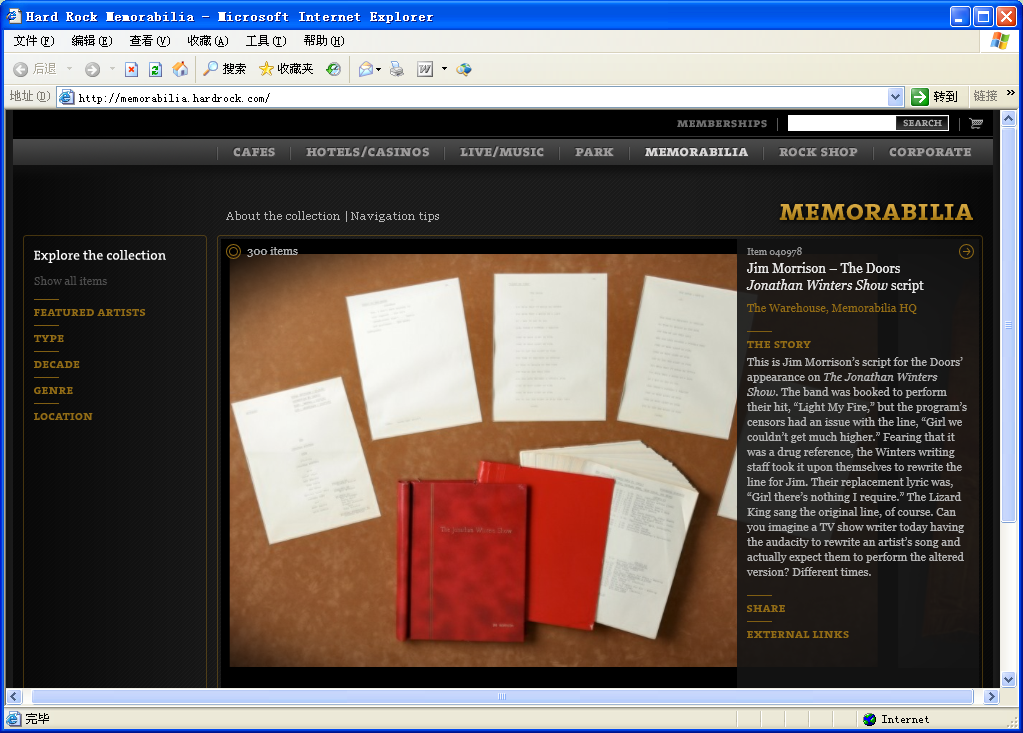
对于开发者而言,应用DeepZoom技术,你只需要下载并安装
DeepZoomComposer,DeepZoomComposer程序工作界面如图所示。
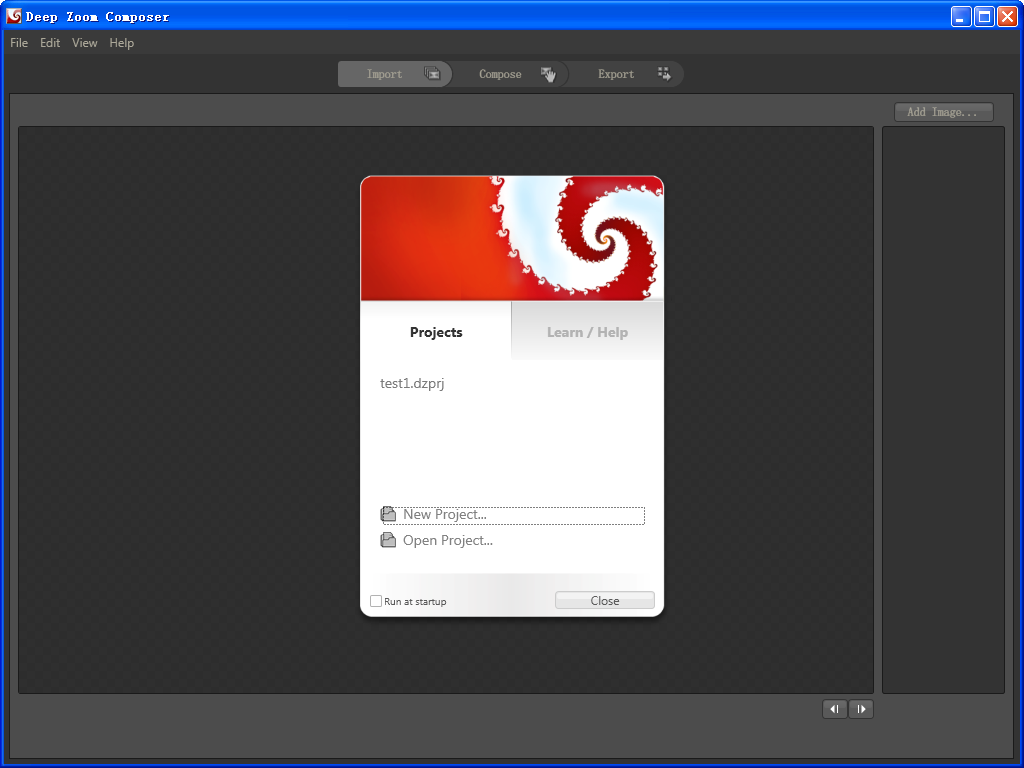
首先在DeepZoomComposer启动界面中创建一个新项目,如图所示。
DeepZoom工作界面主要分为三个工作区,同时又是制作DeepZoom的三个步骤,分别是导入、构图和输出。
导入:
在导入区中你可以将项目中所需要的图片导入到DeepZoomComposer项目中,点击Add Image按钮选择需要导入的图片,导入的过程中会出现Importing进度条窗口,导入完成后的图片会在右侧列表中显示出来。
构图:
完成图片的导入后,你就可以将对程序界面中需要用到图片的拖动到排列区中,在这里你可以使用下方的布局工具,如图所示,对图片的位置、大小和对齐方式进行布局,右侧的All Image选项是显示项目导入全部图片列表,Layer View是显示那些在构图区中用到的图片列表。
输出:
完成导入和排列后,一个简单的DeepZoom应用程序就完成了,通过DeepZoomComposer的输入功能将完成的DeepZoom应用程序输入为一个Silverlight 2应用程序项目,输出前可以预览程序运行结果,如图所示。
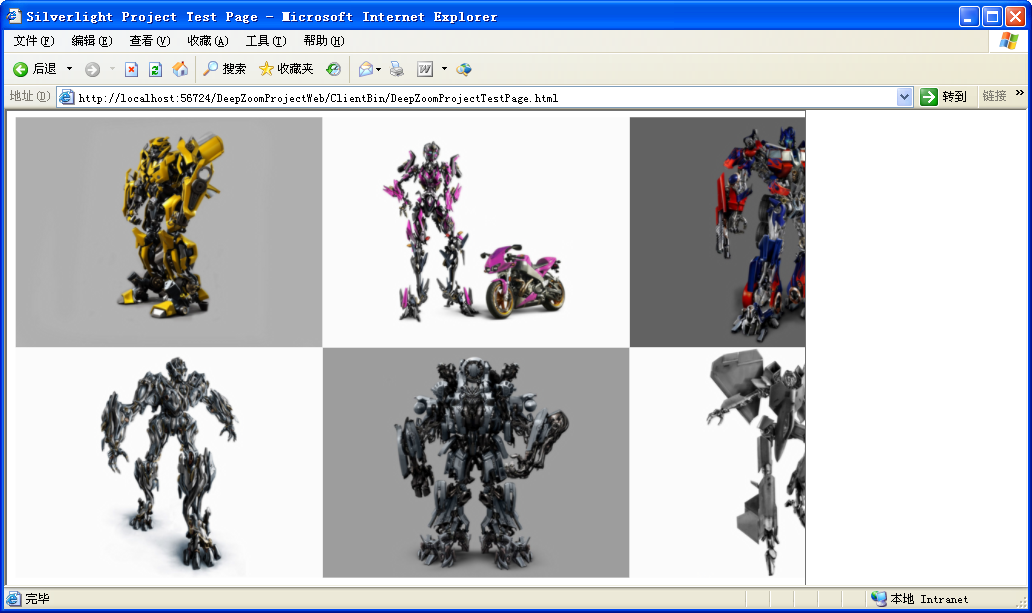
选择Silverlight Export选项,在Silverlight Export选项中,你需要输入Silverlight应用程序名称和输出文件夹,并选择输出的图片类型,点击Export(输出)会出现输出处理进度窗口,如图所示,完成后会显示输出完成窗口,如图所示,这样一个应用DeepZoom技术的Silverlight 2应用程序项目就完成了,使用Visual Studio 2008打开并运行输出的文件夹中的Silverlight 2项目,程序运行结果如图所示。
XAML代码:
<UserControl x:Class="DeepZoomProject.Page"
xmlns=" http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x=" http://schemas.microsoft.com/winfx/2006/xaml"
Width="780" Height="450">
<Grid Background="White">
<MultiScaleImage x:Name="msi" Width="780" Height="400"/>
</Grid>
</UserControl>
xmlns=" http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x=" http://schemas.microsoft.com/winfx/2006/xaml"
Width="780" Height="450">
<Grid Background="White">
<MultiScaleImage x:Name="msi" Width="780" Height="400"/>
</Grid>
</UserControl>
C#代码:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Documents;
using System.Windows.Input;
using System.Windows.Media;
using System.Windows.Media.Animation;
using System.Windows.Shapes;
namespace DeepZoomProject
{
public partial class Page : UserControl
{
//
// Based on prior work done by Lutz Gerhard, Peter Blois, and Scott Hanselman
//
Point lastMousePos = new Point();
{
public partial class Page : UserControl
{
//
// Based on prior work done by Lutz Gerhard, Peter Blois, and Scott Hanselman
//
Point lastMousePos = new Point();
double _zoom = 1;
bool mouseButtonPressed = false;
bool mouseIsDragging = false;
Point dragOffset;
Point currentPosition;
bool mouseButtonPressed = false;
bool mouseIsDragging = false;
Point dragOffset;
Point currentPosition;
public double ZoomFactor
{
get { return _zoom; }
set { _zoom = value; }
}
{
get { return _zoom; }
set { _zoom = value; }
}
public Page()
{
InitializeComponent();
{
InitializeComponent();
//
// We are setting the source here, but you should be able to set the Source property via
//
this.msi.Source = new DeepZoomImageTileSource(new Uri("GeneratedImages/dzc_output.xml", UriKind.Relative));
// We are setting the source here, but you should be able to set the Source property via
//
this.msi.Source = new DeepZoomImageTileSource(new Uri("GeneratedImages/dzc_output.xml", UriKind.Relative));
//
// Firing an event when the MultiScaleImage is Loaded
//
this.msi.Loaded += new RoutedEventHandler(msi_Loaded);
// Firing an event when the MultiScaleImage is Loaded
//
this.msi.Loaded += new RoutedEventHandler(msi_Loaded);
//
// Firing an event when all of the images have been Loaded
//
this.msi.ImageOpenSucceeded += new RoutedEventHandler(msi_ImageOpenSucceeded);
// Firing an event when all of the images have been Loaded
//
this.msi.ImageOpenSucceeded += new RoutedEventHandler(msi_ImageOpenSucceeded);
//
// Handling all of the mouse and keyboard functionality
//
this.MouseMove += delegate(object sender, MouseEventArgs e)
{
if (mouseButtonPressed)
{
mouseIsDragging = true;
}
this.lastMousePos = e.GetPosition(this.msi);
};
// Handling all of the mouse and keyboard functionality
//
this.MouseMove += delegate(object sender, MouseEventArgs e)
{
if (mouseButtonPressed)
{
mouseIsDragging = true;
}
this.lastMousePos = e.GetPosition(this.msi);
};
this.MouseLeftButtonDown += delegate(object sender, MouseButtonEventArgs e)
{
mouseButtonPressed = true;
mouseIsDragging = false;
dragOffset = e.GetPosition(this);
currentPosition = msi.ViewportOrigin;
};
{
mouseButtonPressed = true;
mouseIsDragging = false;
dragOffset = e.GetPosition(this);
currentPosition = msi.ViewportOrigin;
};
this.msi.MouseLeave += delegate(object sender, MouseEventArgs e)
{
mouseIsDragging = false;
};
{
mouseIsDragging = false;
};
this.MouseLeftButtonUp += delegate(object sender, MouseButtonEventArgs e)
{
mouseButtonPressed = false;
if (mouseIsDragging == false)
{
bool shiftDown = (Keyboard.Modifiers & ModifierKeys.Shift) == ModifierKeys.Shift;
{
mouseButtonPressed = false;
if (mouseIsDragging == false)
{
bool shiftDown = (Keyboard.Modifiers & ModifierKeys.Shift) == ModifierKeys.Shift;
ZoomFactor = 2.0;
if (shiftDown) ZoomFactor = 0.5;
Zoom(ZoomFactor, this.lastMousePos);
}
mouseIsDragging = false;
};
if (shiftDown) ZoomFactor = 0.5;
Zoom(ZoomFactor, this.lastMousePos);
}
mouseIsDragging = false;
};
this.MouseMove += delegate(object sender, MouseEventArgs e)
{
if (mouseIsDragging)
{
Point newOrigin = new Point();
newOrigin.X = currentPosition.X - (((e.GetPosition(msi).X - dragOffset.X) / msi.ActualWidth) * msi.ViewportWidth);
newOrigin.Y = currentPosition.Y - (((e.GetPosition(msi).Y - dragOffset.Y) / msi.ActualHeight) * msi.ViewportWidth);
msi.ViewportOrigin = newOrigin;
}
};
{
if (mouseIsDragging)
{
Point newOrigin = new Point();
newOrigin.X = currentPosition.X - (((e.GetPosition(msi).X - dragOffset.X) / msi.ActualWidth) * msi.ViewportWidth);
newOrigin.Y = currentPosition.Y - (((e.GetPosition(msi).Y - dragOffset.Y) / msi.ActualHeight) * msi.ViewportWidth);
msi.ViewportOrigin = newOrigin;
}
};
new MouseWheelHelper(this).Moved += delegate(object sender, MouseWheelEventArgs e)
{
e.Handled = true;
if (e.Delta > 0)
ZoomFactor = 1.2;
else
ZoomFactor = .80;
{
e.Handled = true;
if (e.Delta > 0)
ZoomFactor = 1.2;
else
ZoomFactor = .80;
Zoom(ZoomFactor, this.lastMousePos);
};
}
};
}
void msi_ImageOpenSucceeded(object sender, RoutedEventArgs e)
{
//If collection, this gets you a list of all of the MultiScaleSubImages
//
//foreach (MultiScaleSubImage subImage in msi.SubImages)
//{
// // Do something
//}
}
{
//If collection, this gets you a list of all of the MultiScaleSubImages
//
//foreach (MultiScaleSubImage subImage in msi.SubImages)
//{
// // Do something
//}
}
void msi_Loaded(object sender, RoutedEventArgs e)
{
// Hook up any events you want when the image has successfully been opened
}
{
// Hook up any events you want when the image has successfully been opened
}
public void Zoom(double zoom, Point pointToZoom)
{
Point logicalPoint = this.msi.ElementToLogicalPoint(pointToZoom);
this.msi.ZoomAboutLogicalPoint(zoom, logicalPoint.X, logicalPoint.Y);
}
{
Point logicalPoint = this.msi.ElementToLogicalPoint(pointToZoom);
this.msi.ZoomAboutLogicalPoint(zoom, logicalPoint.X, logicalPoint.Y);
}
/*
* Sample event handlerrs tied to the Click of event of various buttons for
* showing all images, zooming in, and zooming out!
*
private void ShowAllClick(object sender, RoutedEventArgs e)
{
this.msi.ViewportOrigin = new Point(0, 0);
this.msi.ViewportWidth = 1;
ZoomFactor = 1;
}
* Sample event handlerrs tied to the Click of event of various buttons for
* showing all images, zooming in, and zooming out!
*
private void ShowAllClick(object sender, RoutedEventArgs e)
{
this.msi.ViewportOrigin = new Point(0, 0);
this.msi.ViewportWidth = 1;
ZoomFactor = 1;
}
private void zoomInClick(object sender, RoutedEventArgs e)
{
Zoom(1.2, new Point(this.ActualWidth / 2, this.ActualHeight / 2));
}
{
Zoom(1.2, new Point(this.ActualWidth / 2, this.ActualHeight / 2));
}
private void zoomOutClick(object sender, RoutedEventArgs e)
{
Zoom(.8, new Point(this.ActualWidth / 2, this.ActualHeight / 2));
}
* */
}
}
{
Zoom(.8, new Point(this.ActualWidth / 2, this.ActualHeight / 2));
}
* */
}
}
放大后效果:
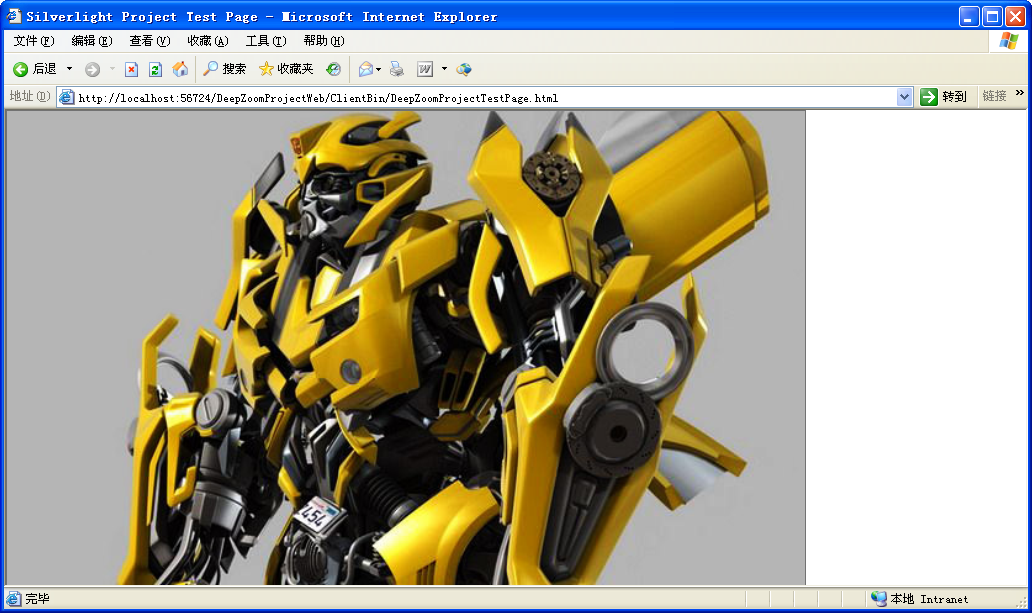
Deep Zoom Composer 下载地址:http://www.microsoft.com/downloads/details.aspx?familyid=457b17b7-52bf-4bda-87a3-fa8a4673f8bf&displaylang=en
本文转自dotfun 51CTO博客,原文链接:http://blog.51cto.com/dotfun/285830