//
// Created by cynthia on 2021/11/25.
//
#include <sys/types.h>
#include <sys/stat.h>
#include "unistd.h"
#include "dirent.h"
#include <iostream>
#include <iostream>
#include <direct.h>
#include <opencv2/opencv.hpp>
using namespace std;
using namespace cv;
//读入指定文件夹下的所有文件
void GetFileNames(string inpath, string outpath, vector<string>& infilenames, vector<string>& outfilenames)
{
DIR *pDir;
struct dirent* ptr;
if(!(pDir = opendir(inpath.c_str())))
return;
while((ptr = readdir(pDir))!=0) {
if (strcmp(ptr->d_name, ".") != 0 && strcmp(ptr->d_name, "..") != 0)
{
infilenames.push_back(inpath + "/" + ptr->d_name);
outfilenames.push_back(outpath+ "/" + ptr->d_name);
}
}
closedir(pDir);
}
int main(int argc, char * argv[])
{
std::string inPath="C:/Users/Seizet/Desktop/Data/Add_Down_2020_11_30_xukang";
std::string outPath="C:/Users/Seizet/Desktop/Data/Add_Down_2020_11_30_TwoChannel";
vector<string> inFiles;
vector<string> outFiles;
GetFileNames(inPath, outPath, inFiles, outFiles);
if (0 != access(outPath.c_str(), 0))
{
if (0 != mkdir(outPath.c_str()))
{
return -1;
}
}
for(int i = 0; i < inFiles.size(); i++)
{
string suffixStr = inFiles[i].substr(inFiles[i].find_last_of('.') + 1); //获取文件后缀
if(!suffixStr.compare("json")) //字符串值相同返回0
{
CopyFile(inFiles[i].c_str(), outFiles[i].c_str(), FALSE); //false代表覆盖,true不覆盖
}
if(!suffixStr.compare("bmp"))
{
cv::Mat srcImg = cv::imread(inFiles[i], cv::IMREAD_COLOR);
std::vector<cv::Mat> splitImg;
split(srcImg, splitImg);
cv::Mat depthImg(srcImg.cols, srcImg.rows, CV_8UC1, cv::Scalar(0));
splitImg[2] = Scalar(0);
Mat dstImg;
merge(splitImg, dstImg);
cv::imwrite(outFiles[i], dstImg);
cv::namedWindow("No depth Image", 0);
cv::resizeWindow("No depth Image", cv::Size(960, 600)); //创建窗口,自动大小
cv::imshow("No depth Image", dstImg); //显示图像到指定的窗口
cv::waitKey(0);
}
}
return 0;
}
Opencv读取图像,图像通道分离,图像通道融合
最新推荐文章于 2024-05-05 12:02:26 发布
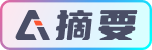