PSP
任务 | 开始时间 | 结束时间 | 中断时间 | delta时间 |
---|
画素材 | 12.07 18:13 | 12.07 18:28 | 0min | 15min |
设计接口 | 12.08 15:13 | 12.08 15:38 | 0min | 25min |
写代码 | 12.08 15:40 | 12.08 17:31 | 0min | 101min |
写代码 | 12.08 18:40 | 12.08 19:42 | 0min | 62min |
WBS
任务 | 估计用时 | 实际用时 |
---|
搜集素材 | 25min | 15min |
设计分析 | 50min | 25min |
编码显示图片 | 30min | 63min |
编码使蛇运动 | 60min | 38min |
编码碰撞检测 | 30min | 62min+ |
演示
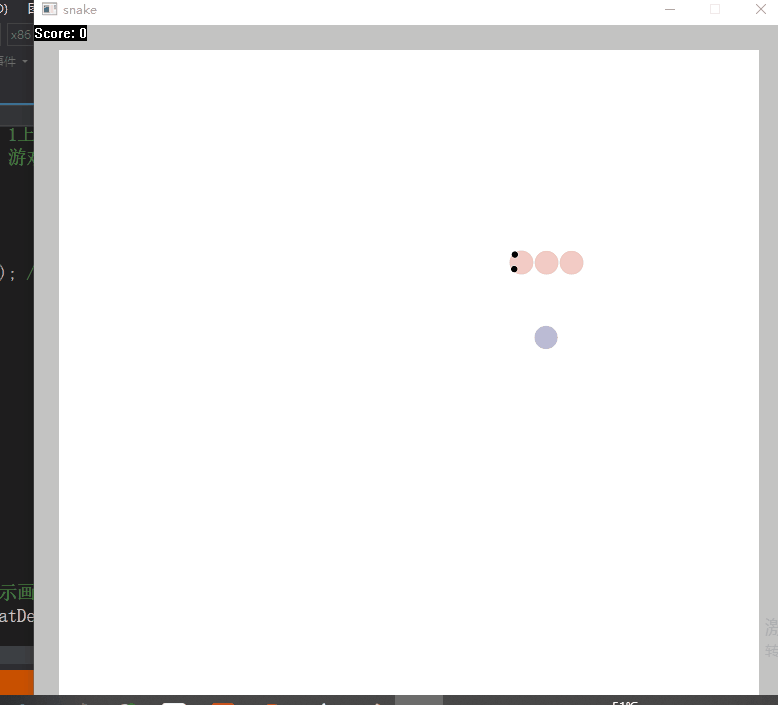
代码(已完成)
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <time.h>
#include <windows.h>
#include <math.h>
#include <graphics.h>
#include <easyx.h>
#pragma comment(lib,"Winmm.lib")
struct node {
int x;
int y;
struct node* next;
};
typedef struct node node_t;
typedef struct node* nodeptr_t;
#define High 1000
#define Width 750
IMAGE img_bk;
IMAGE img_wall;
int head_x, head_y;
int targetx, targety;
IMAGE img_target, img_headup, img_headdown, img_headleft, img_headright, img_body;
int score = 0;
nodeptr_t snakelist;
int station;
int isGameOver = 0;
void Init();
void UpdateWhthoutEat();
void Show();
void Input();
void GenerateTarget();
bool EatDetect();
bool TargetDetect();
void UpdateWhthEat();
int main() {
Init();
GenerateTarget();
while (1)
{
Show();
bool iseat = EatDetect();
if (iseat)
UpdateWhthEat();
else
UpdateWhthoutEat();
bool isover = TargetDetect();
if (isover)
isGameOver = 1;
Input();
}
return 0;
}
void Init()
{
initgraph(Width, High);
loadimage(&img_bk, L"sources/background.png");
loadimage(&img_headup, L"sources/head_up.png");
loadimage(&img_headdown, L"sources/head_down.png");
loadimage(&img_headleft, L"sources/head_left.png");
loadimage(&img_headright, L"sources/head_right.png");
loadimage(&img_body, L"sources/body.png");
loadimage(&img_target, L"sources/target.png");
loadimage(&img_wall, L"sources/wall.png");
head_x = Width / 2;
head_y = Width / 2;
station = 1;
targetx = 0;
targety = 0;
snakelist = (nodeptr_t)malloc(sizeof(node_t));
snakelist->next = nullptr;
snakelist->x = 0;
snakelist->y = 0;
int newbody_x = head_x;
int newbody_y = head_y;
nodeptr_t temptail = snakelist;
for (int i = 0; i < 3; ++i)
{
nodeptr_t temp = (nodeptr_t)malloc(sizeof(node_t));
temp->x = newbody_x;
temp->y = newbody_y;
newbody_y = newbody_y + 25;
temptail->next = temp;
temptail = temptail->next;
}
temptail->next = nullptr;
BeginBatchDraw();
}
void Input()
{
if (_kbhit())
{
char keyboard = _getch();
if (keyboard == 'w' && station != 2) {
station = 1;
}
if (keyboard == 's' && station != 1)
{
station = 2;
}
if (keyboard == 'a' && station != 4)
{
station = 3;
}
if (keyboard == 'd' && station != 3)
{
station = 4;
}
}
}
void UpdateWhthoutEat()
{
if (station == 1)
{
head_y = head_y - 25;
}
if (station == 2)
{
head_y = head_y + 25;
}
if (station == 3)
{
head_x = head_x - 25;
}
if (station == 4)
{
head_x = head_x + 25;
}
nodeptr_t temptail = snakelist;
while (temptail->next->next != nullptr)
temptail = temptail->next;
temptail->next->next = snakelist->next;
snakelist->next = temptail->next;
temptail->next = nullptr;
snakelist->next->x = head_x;
snakelist->next->y = head_y;
return;
}
void UpdateWhthEat()
{
if (station == 1)
{
head_y = head_y - 25;
}
if (station == 2)
{
head_y = head_y + 25;
}
if (station == 3)
{
head_x = head_x - 25;
}
if (station == 4)
{
head_x = head_x + 25;
}
nodeptr_t temp = (nodeptr_t)malloc(sizeof(node_t));
temp->next = snakelist->next;
snakelist->next = temp;
temp->x = head_x;
temp->y = head_y;
GenerateTarget();
return;
}
void Show()
{
putimage(0, 0, Width, High, &img_bk, 0, 0);
for (int i = 0; i < 30; ++i)
putimage(0, 25 * i, 25, 25, &img_wall, 0, 0);
for (int i = 1; i < 30; ++i)
putimage(25 * i, 0, 25, 25, &img_wall, 0, 0);
for (int i = 1; i < 30; ++i)
putimage(725, 25 * i, 25, 25, &img_wall, 0, 0);
for (int i = 1; i < 29; ++i)
putimage(25 * i, 725, 25, 25, &img_wall, 0, 0);
if (isGameOver == 0)
{
nodeptr_t templist = snakelist->next;
putimage(targetx, targety, 25, 25, &img_target, 0, 0);
if (station == 1)
putimage(templist->x, templist->y, 25, 25, &img_headup, 0, 0);
if (station == 2)
putimage(templist->x, templist->y, 25, 25, &img_headdown, 0, 0);
if (station == 3)
putimage(templist->x, templist->y, 25, 25, &img_headleft, 0, 0);
if (station == 4)
putimage(templist->x, templist->y, 25, 25, &img_headright, 0, 0);
templist = templist->next;
while (templist != nullptr)
{
putimage(templist->x, templist->y, 25, 25, &img_body, 0, 0);
templist = templist->next;
}
}
else
{
wchar_t str[50];
swprintf(str, 100, L"Game Over");
outtextxy(Width / 2, Width / 2, str);
}
wchar_t s[50];
swprintf(s, 100, L"Score: %d", score);
outtextxy(0, 0, s);
FlushBatchDraw();
Sleep(200);
}
void GenerateTarget()
{
srand((unsigned)time(NULL));
targetx = rand() % ((Width - 75) / 25) * 25 + 25;
targety = rand() % ((Width - 75) / 25) * 25 + 25;
nodeptr_t templist = snakelist;
while (1)
{
while (templist->next != nullptr)
{
if (templist->next->x == targetx && templist->next->x == targety)
{
targetx = rand() % ((Width - 75) / 25) * 25 + 25;
targety = rand() % ((Width - 75) / 25) * 25 + 25;
break;
}
return;
}
}
}
bool EatDetect()
{
nodeptr_t snakehead = snakelist->next;
if (snakehead->x == targetx && snakehead->y == targety)
{
score = score + 1;
return 1;
}
return 0;
}
bool TargetDetect()
{
nodeptr_t temp = snakelist->next;
int headx = temp->x;
int heady = temp->y;
if (headx == 0 || headx == Width - 25 || heady == 0 || heady == Width - 25)
return 1;
temp = temp->next;
while (temp != nullptr)
{
if (temp->x == headx && temp->y == heady)
return 1;
temp = temp->next;
}
return 0;
}